Performance Optimization and Wasted Renders | Lecture 239 | React.JS 🔥
Summary
TLDRThe video script focuses on optimizing React applications by addressing three key areas: preventing wasted renders, improving app speed and responsiveness, and reducing bundle size. To prevent wasted renders, React provides tools like `memo`, `useMemo`, and `useCallback`. These can also enhance perceived speed, along with the `useTransition` hook. Reducing the bundle size is achieved by minimizing third-party packages and implementing code splitting and lazy loading. The script emphasizes that these tools are not always necessary but should be part of a developer's toolkit for when needed. It also clarifies common misconceptions about props and rendering, explaining that changes in props do not directly cause a component to re-render, but rather it's the parent component's re-rendering that triggers it. The concept of a 'wasted render' is introduced, highlighting its inefficiency and the importance of avoiding frequent or slow re-renders to maintain a fluid and responsive user experience.
Takeaways
- 🚦 Optimizing React apps involves preventing wasted renders, improving app speed and responsiveness, and reducing bundle size.
- 🛠️ Use `React.memo`, `useMemo`, and `useCallback` hooks to prevent unnecessary component re-renders and memorize objects and functions.
- 🔁 Passing elements as children or props to other elements can help prevent re-renders, thus optimizing performance.
- 🎢 Utilize `useMemo`, `useCallback`, and the newer `useTransition` hook to enhance the perceived speed of the application.
- 📦 Minimize the use of third-party packages and implement code splitting and lazy loading to reduce the bundle size.
- 🔍 Understand when a component re-renders: state changes, context changes, or parent component re-rendering.
- 🔄 Props change only when the parent component re-renders, which is why child components receiving the props also re-render.
- 🖥️ Rendering a component doesn't necessarily mean the DOM updates; it involves creating a new virtual DOM and performing the reconciliation process.
- 🚫 A 'wasted render' occurs when a render doesn't produce any DOM changes, leading to unnecessary computational overhead.
- 📉 Frequent or slow re-renders can make an application feel sluggish and unresponsive, impacting user experience negatively.
- 🧰 This section aims to provide an arsenal of optimization tools, to be used judiciously based on specific situations and needs.
Q & A
What are the three main areas of optimization in React applications?
-The three main areas of optimization in React applications are: preventing wasted renders, improving overall app speed and responsiveness, and reducing the bundle size.
How can we prevent wasted renders in React components?
-Wasted renders can be prevented by using React's `memo` to memorize components, `useMemo` to memorize objects, and `useCallback` hooks to memorize functions. Additionally, passing elements as children or props to other elements can help prevent unnecessary re-renders.
What is the misconception about props and re-rendering in React?
-A common misconception is that updating props directly causes a component to re-render. However, props only change when the parent component re-renders, which then causes the child components to re-render as well.
What is the role of the `useTransition` hook in optimizing perceived app speed?
-The `useTransition` hook can be used to improve the perceived speed of an application by managing state transitions and reducing visual stutter, providing a smoother user experience.
Why is it important to reduce the bundle size in a React application?
-Reducing the bundle size is important because it can lead to faster load times and better performance, especially for users with slower internet connections or on mobile devices.
How can code splitting and lazy loading help with bundle size optimization?
-Code splitting and lazy loading allow the application to load only the necessary parts of the code at a given time, rather than loading the entire application upfront, thus reducing the initial bundle size.
What is a wasted render in the context of React components?
-A wasted render is a render that does not result in any change to the DOM. It is considered wasteful because all the calculations and operations associated with rendering are performed without leading to an actual update in the user interface.
When does a React component instance get re-rendered?
-A React component instance gets re-rendered when the component's state changes, when there's a change in a context that the component is subscribed to, or when a parent component re-renders.
Why is it important to understand when and why components re-render in React?
-Understanding when and why components re-render is important for optimizing performance. It helps developers identify unnecessary renders, which can lead to performance bottlenecks and a less responsive user interface.
What are some built-in React tools for optimizing performance?
-Built-in React tools for optimizing performance include `memo`, `useMemo`, `useCallback`, and `useTransition`. These tools help in preventing wasted renders, improving app speed, and managing state transitions.
What is the significance of the virtual DOM in React's rendering process?
-The virtual DOM is a lightweight representation of the actual DOM that allows React to compute the minimal number of changes needed to update the real DOM. It helps in optimizing performance by minimizing direct manipulation of the DOM, which can be expensive.
How can third-party packages affect the performance of a React application?
-Third-party packages can affect performance by increasing the bundle size, which can slow down the application load time. It's important to use them judiciously and only when necessary to maintain optimal performance.
Outlines
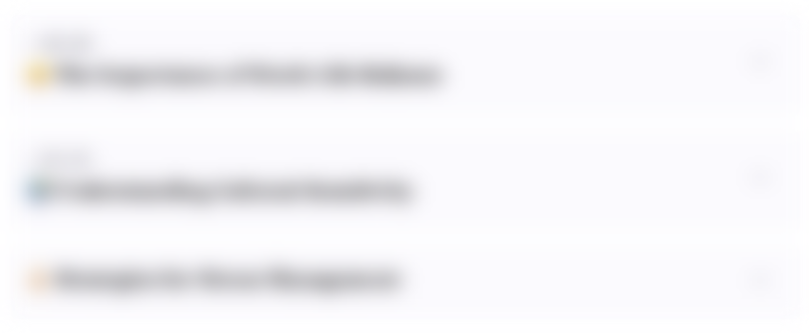
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
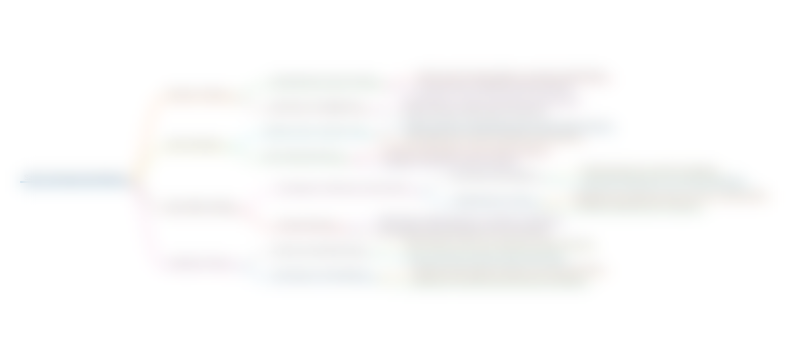
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
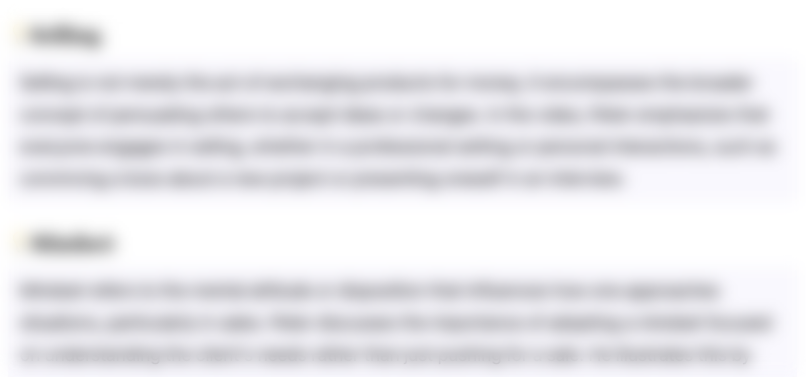
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
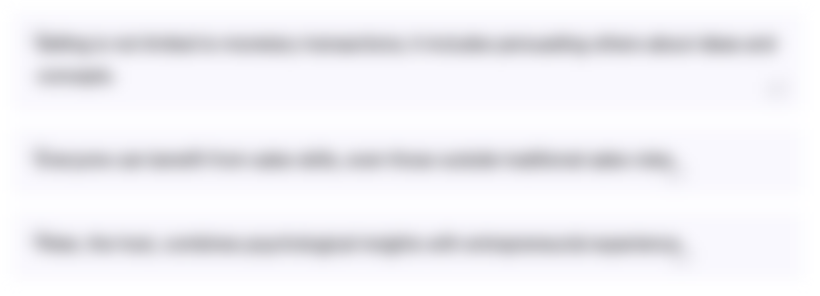
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
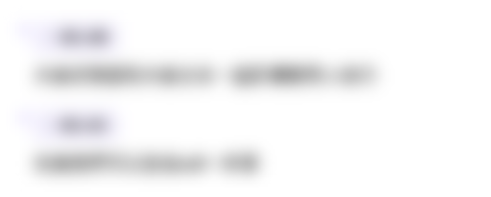
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)