Python TIC TAC TOE Tutorial | Beginner Friendly Tutorial
Summary
TLDRIn this informative video, the creator guides viewers through the process of developing a classic tic-tac-toe game in Python, complete with a basic AI bot. The tutorial covers game board creation, player input, win/tie conditions, and switching players. It emphasizes the importance of understanding beginner programming skills before tackling more complex projects. The video also hints at potential for an advanced tutorial with a smarter AI bot and encourages viewers to explore more complex coding projects.
Takeaways
- 📝 The video focuses on creating a classic tic-tac-toe game in Python, suitable for beginners to reinforce their programming skills.
- 🎯 The project involves making a game that runs in the Python console and includes a basic AI bot.
- 📌 Before starting the project, it's important to outline the necessary features, such as creating a game board, taking player input, and checking for win or tie conditions.
- 🔲 The game board is initialized as a 3x3 list of dashes, representing empty spaces.
- ⚙️ Global variables are set for the game board, current player, winner, and game running status.
- 🖨️ A 'print board' function is created to display the game board in a user-friendly format.
- 🔄 Functions are defined to take player input, validate the input, and update the game board accordingly.
- 🏆 Functions are implemented to check for winning conditions both horizontally, vertically, and diagonally.
- 👥 A 'check tie' function is created to determine if the game has resulted in a draw.
- 🔄 A 'switch player' function is used to alternate turns between players.
- 🤖 A basic AI bot is introduced, making random moves using the 'random' module.
Q & A
What is the main topic of the video?
-The main topic of the video is creating a beginner programming project, specifically a classic tic-tac-toe game in Python, including a basic AI bot.
What are some key features that the tic-tac-toe game needs to have?
-The tic-tac-toe game should have a game board, player input functionality, a method to check for win or tie, player switching, and a loop to continuously run the game.
How does the video suggest initializing the game board?
-The video suggests initializing the game board as a 3x3 list of dashes, representing empty spaces on the tic-tac-toe grid.
What global variables are set up at the beginning of the code?
-The global variables set up at the beginning are 'board' for the game board, 'current player' to track the turn, 'winner' to determine the winning player, and 'game running' to control the game loop.
How does the video propose to handle player input for the game?
-The video proposes using Python's built-in 'input' function to get player input, then converting the input to an integer and validating it to ensure it's a number between 1 and 9 and that the chosen position on the board is not yet occupied.
What is the purpose of the 'check horizontal' function?
-The 'check horizontal' function checks each row across the game board to determine if there's a winner based on three-in-a-row for horizontal positions.
How does the 'check tie' function work?
-The 'check tie' function checks if there are any remaining empty spaces (indicated by dashes) on the board. If there are no dashes left, it means the board is full and no player has won, resulting in a tie.
What is the role of the 'switch player' function?
-The 'switch player' function updates the 'current player' variable to alternate turns between players X and O.
How does the AI bot make its moves in the game?
-The AI bot makes its moves by using the 'random' module to generate a random number between 0 and 8, representing a position on the board. It then checks if the position is unoccupied and makes a move there.
What additional feature does the video suggest for future development?
-The video suggests developing a more advanced AI bot that can strategically play the game, providing a challenge for human players.
How can viewers access the code for the tic-tac-toe game?
-Viewers can access the code by visiting the GitHub page linked in the video description.
Outlines
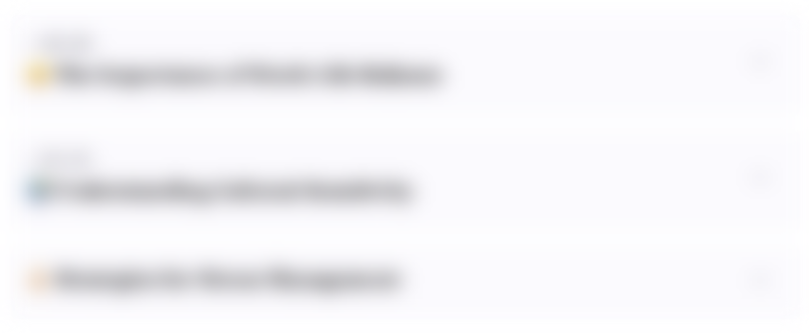
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
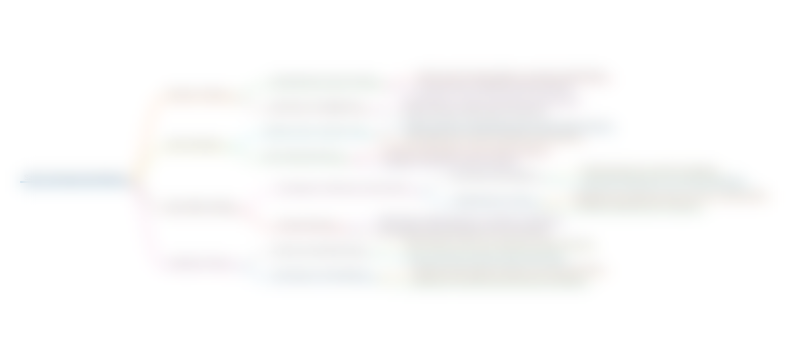
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
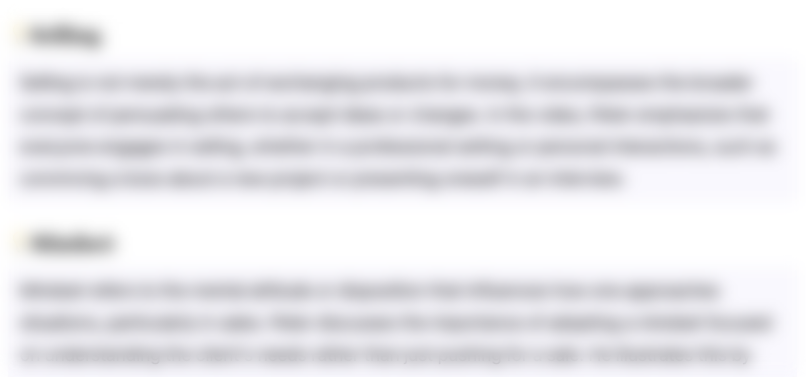
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
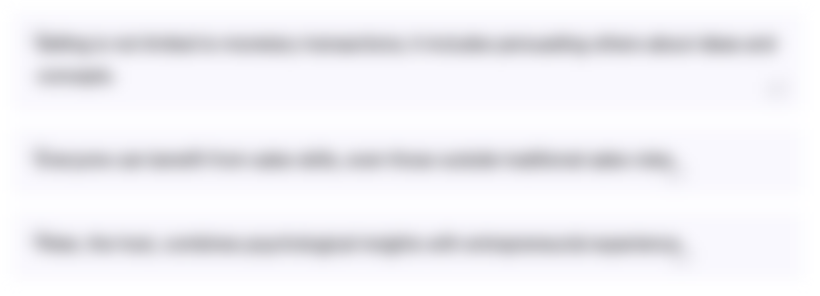
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
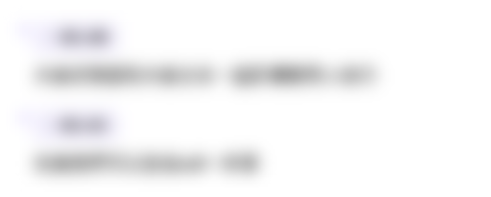
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
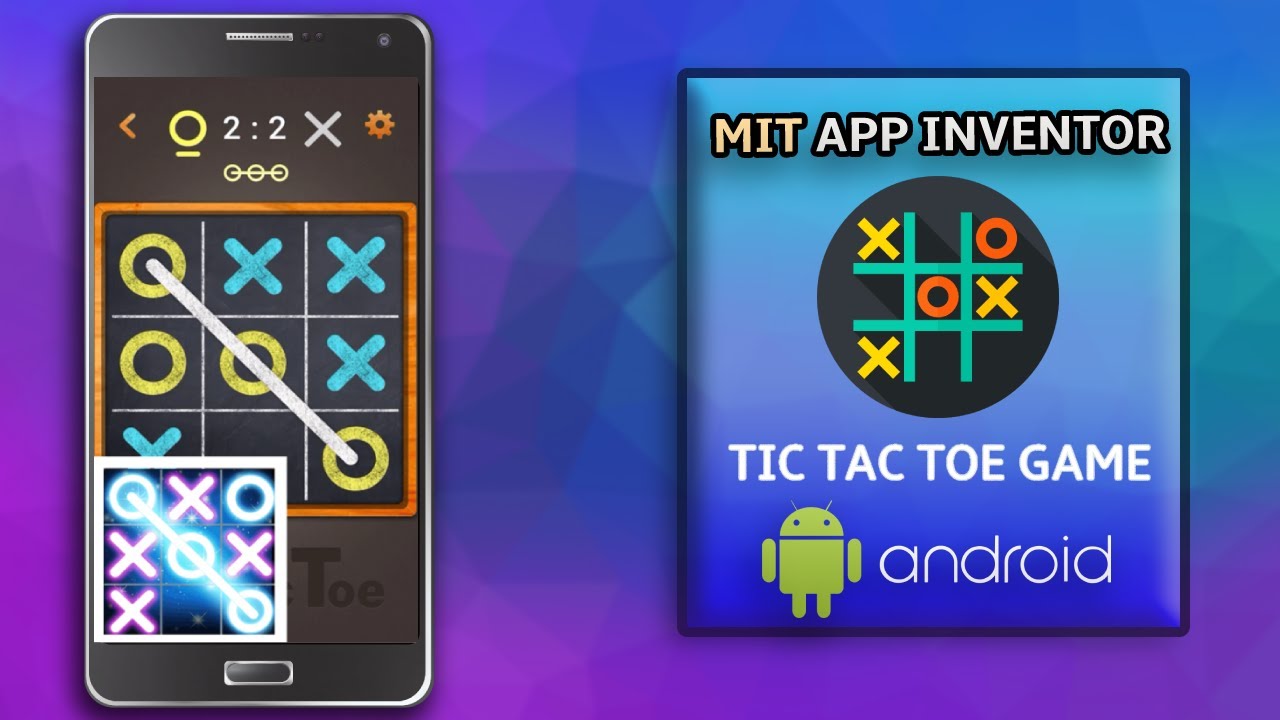
Create Tic Tac Toe Game in MIT App Inventor in Just 10 Minutes! || Free Extension || App Inventor 2

Tic Tac Toe - Down the memory Lane 02
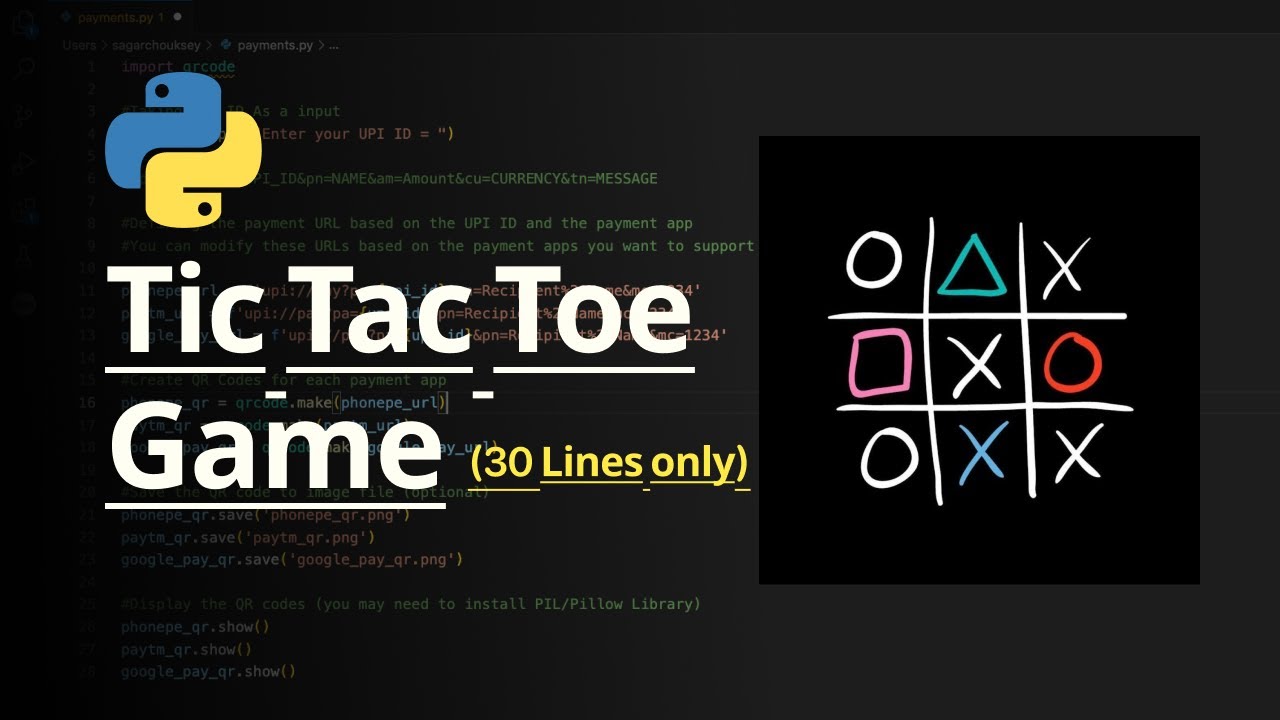
Tic Tac Toe Game In Python | Python Project for Beginners
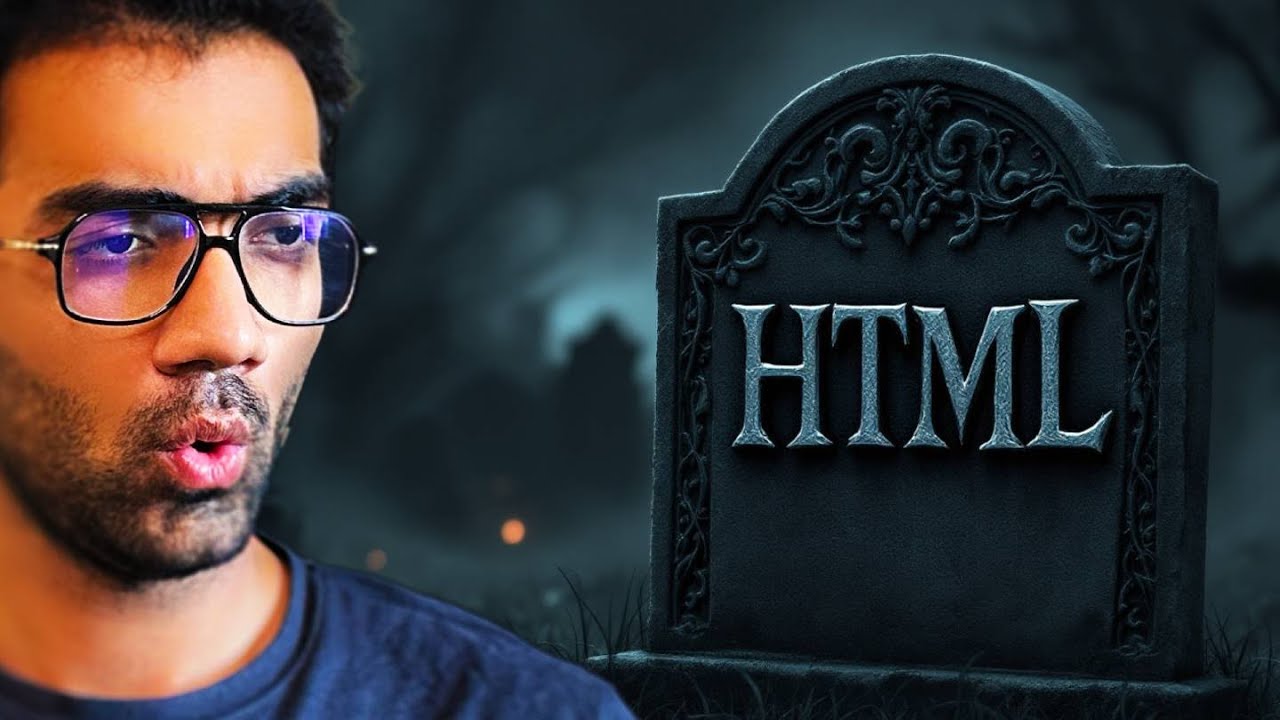
Leave HTML - You can code frontend with Python now

I Played Every SECRET GOOGLE GAME
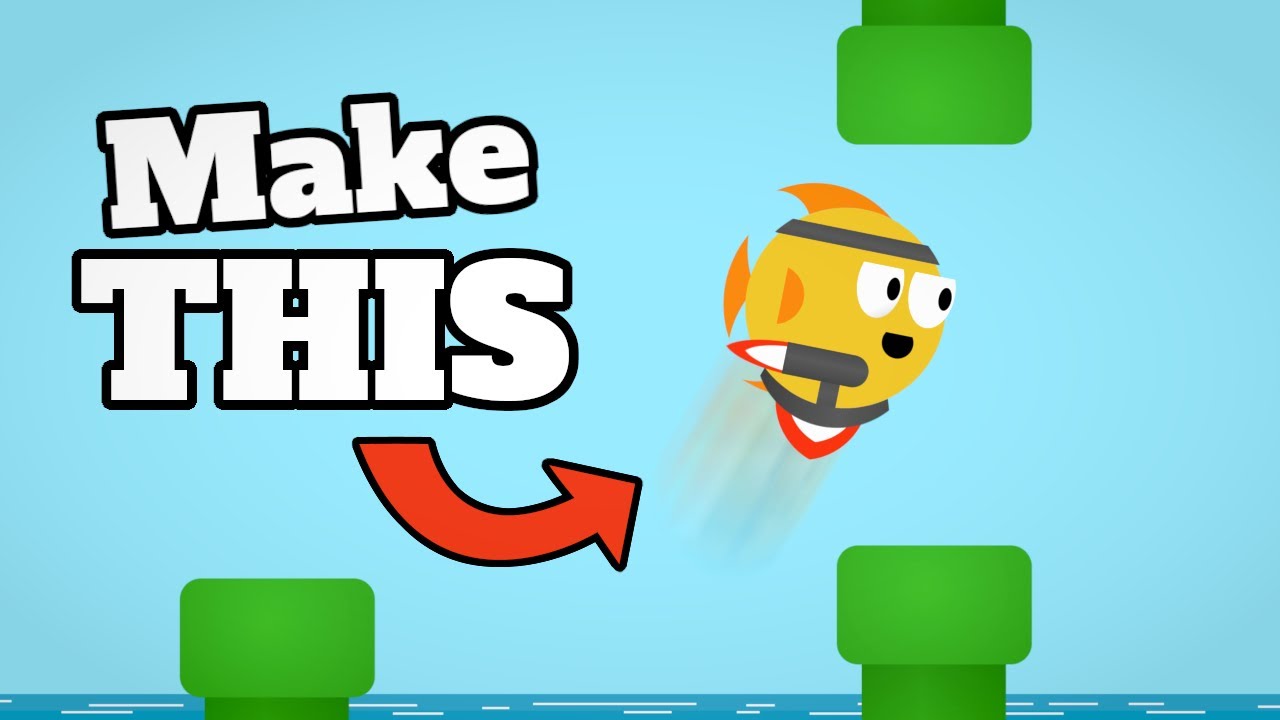
CREATE a Flappy Bird Game in Unity with CLEAN CODE Like a PRO!
5.0 / 5 (0 votes)