Data Structures and Algorithms in 15 Minutes
Summary
TLDRThis video script offers a humorous and insightful journey into the world of data structures and algorithms, essential for computer science enthusiasts. It humorously addresses the challenges and rewards of mastering these concepts, which are crucial for problem-solving efficiency and highly valued by tech companies. The script covers various data structures like arrays, linked lists, binary trees, and heaps, and algorithms including sorting, searching, and graph theory. It also touches on the importance of understanding time and space complexity, and provides learning resources for further study, emphasizing the value of a broad understanding before delving into deeper details.
Takeaways
- 😎 Being proficient in data structures and algorithms is highly valued in the tech industry, often associated with high intellect and attractive job offers.
- 🚀 The journey to mastering algorithms and data structures is challenging and can be filled with frustration and feelings of imposter syndrome.
- 💡 The motivation to learn should stem from a genuine interest in problem-solving efficiency rather than just for job prospects.
- 📈 Understanding algorithm efficiency involves recognizing the relationship between input size and the number of operations required, often described using Big O notation.
- 🔍 Different algorithms have distinct time complexities, such as constant, linear, quadratic, logarithmic, exponential, and factorial, which greatly affect performance with varying input sizes.
- 🌲 Data structures like arrays, linked lists, and binary trees, each with their own properties and use cases, are fundamental to computer science.
- 🔄 Sorting algorithms, including selection sort and merge sort, have different time complexities, with merge sort being notably efficient at O(n log n).
- 🌐 Graphs, with their vertices and edges, are used to model complex relationships and can be manipulated to find shortest paths or perform topological sorts.
- 🔑 Hash maps offer nearly constant time data retrieval and are built on arrays with a hash function to map keys to indices, making them extremely powerful for data storage and access.
- 🎓 Learning data structures and algorithms is more effective when approached with a general understanding first, followed by deeper dives into specific topics.
Q & A
Why is being proficient in data structures and algorithms considered prestigious in the computer science field?
-Proficiency in data structures and algorithms is considered prestigious because it signifies a deep understanding of efficient problem-solving in computer science. It often leads to high-paying job offers from prestigious companies and is associated with high social market value in online tech communities.
What is the significance of Big O notation in computer science?
-Big O notation is used to describe the time complexity of an algorithm, which is the relationship between the growth of input size and the growth of the number of operations required. It helps in comparing the efficiency of different algorithms and is crucial for optimizing performance.
How does the efficiency of an algorithm impact the performance of a program?
-The efficiency of an algorithm directly impacts the performance of a program by determining how the execution time and resources required scale with the input size. Inefficient algorithms can lead to excessive resource usage and slow execution times, especially with large inputs.
What is the difference between a constant time and a linear time algorithm?
-A constant time algorithm has a fixed number of operations, regardless of the input size, often represented as O(1). In contrast, a linear time algorithm's operation count grows proportionally with the input size, represented as O(n), where 'n' is the input size.
Why is it important to understand logarithms when studying algorithms?
-Logarithms are important in algorithm studies because they often represent the efficiency of algorithms that involve dividing the problem size by half with each step, such as binary search. Understanding logarithms helps in grasping the concept of logarithmic time complexity, which is significant for algorithms like binary search and merge sort.
What is a binary search and how does it work?
-A binary search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing the search interval in half. If the value of the search key is less than the item in the middle of the interval, narrow the interval to the lower half. Otherwise, narrow it to the upper half. Repeatedly check until the value is found or the interval is empty.
Why is sorting important in computer science, and what is the best time complexity we can achieve for sorting?
-Sorting is important in computer science because it is a fundamental operation that prepares data for efficient searching, processing, and analysis. The best time complexity we can achieve for sorting an arbitrary collection is O(n log n), which is the time complexity of algorithms like merge sort.
What is the primary difference between an array and a linked list?
-The primary difference is that an array is a fixed-size, contiguous block of memory where elements can be accessed directly by index, while a linked list is a flexible-size data structure composed of nodes that contain data and a reference to the next node in the sequence. Linked lists allow for efficient insertion and deletion but do not support direct access by index.
How does a binary search tree differ from a binary tree, and what are its properties?
-A binary search tree is a specific type of binary tree with the property that the value of each node is greater than or equal to any value stored in the left subtree and less than any value in the right subtree. This ordering property makes binary search trees efficient for searching, insertion, and deletion operations.
What is a heap, and how does it differ from a binary search tree?
-A heap is a specialized tree-based data structure that satisfies the heap property: either the parent node is always greater than or equal to (in a max heap) or less than or equal to (in a min heap) any of its children. Unlike binary search trees, heaps are not necessarily sorted, and the primary focus is on the priority of the root element, which is the highest or lowest value in the heap.
What are the two main ways to traverse a tree, and how do they differ?
-The two main ways to traverse a tree are depth-first search (DFS) and breadth-first search (BFS). DFS explores as far as possible along each branch before backtracking, while BFS visits all the nodes at the present depth level before moving on to nodes at the next depth level.
Outlines
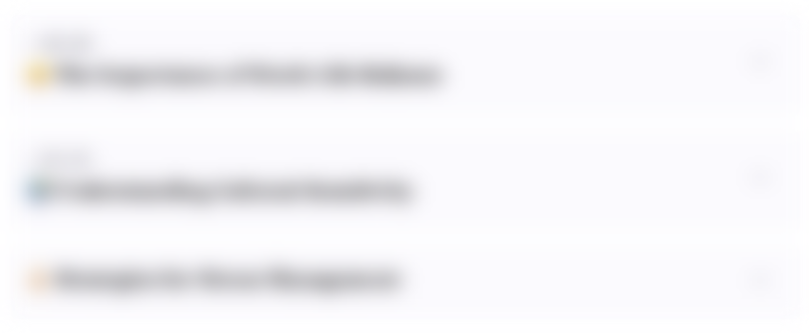
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
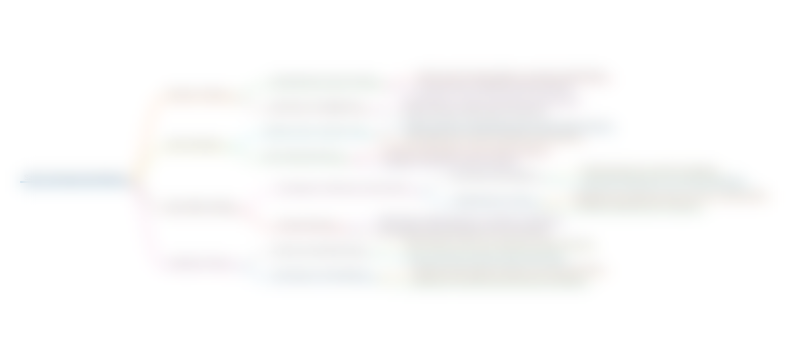
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
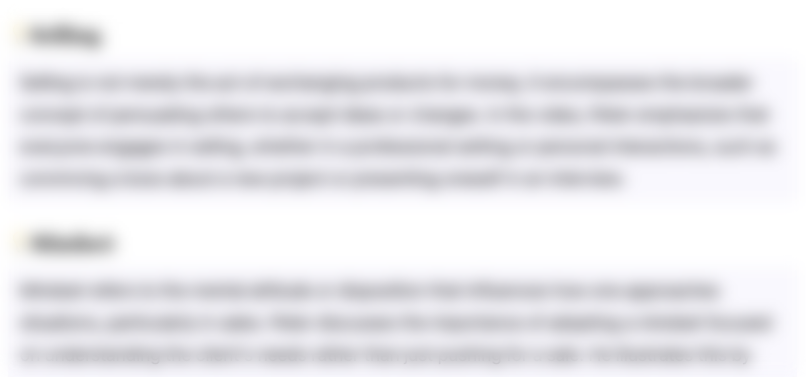
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
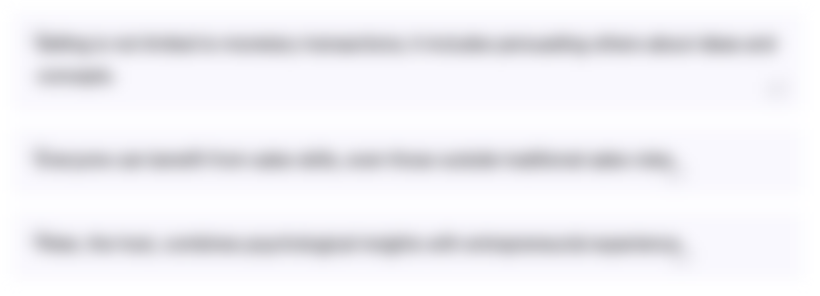
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
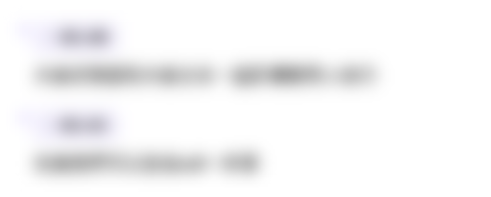
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

Data Structures & Algorithms Roadmap - What You NEED To Learn
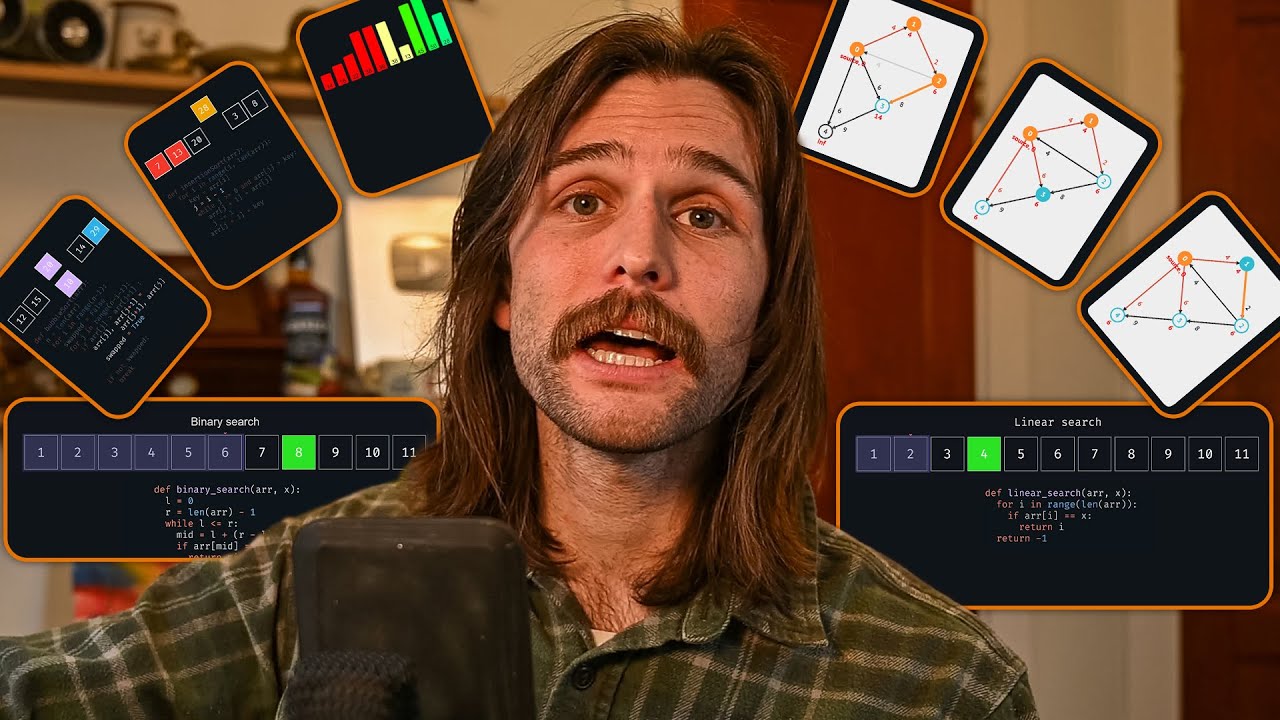
3 Types of Algorithms Every Programmer Needs to Know

8 Data Structures Every Programmer Should Know

Top 7 Data Structures for Interviews Explained SIMPLY
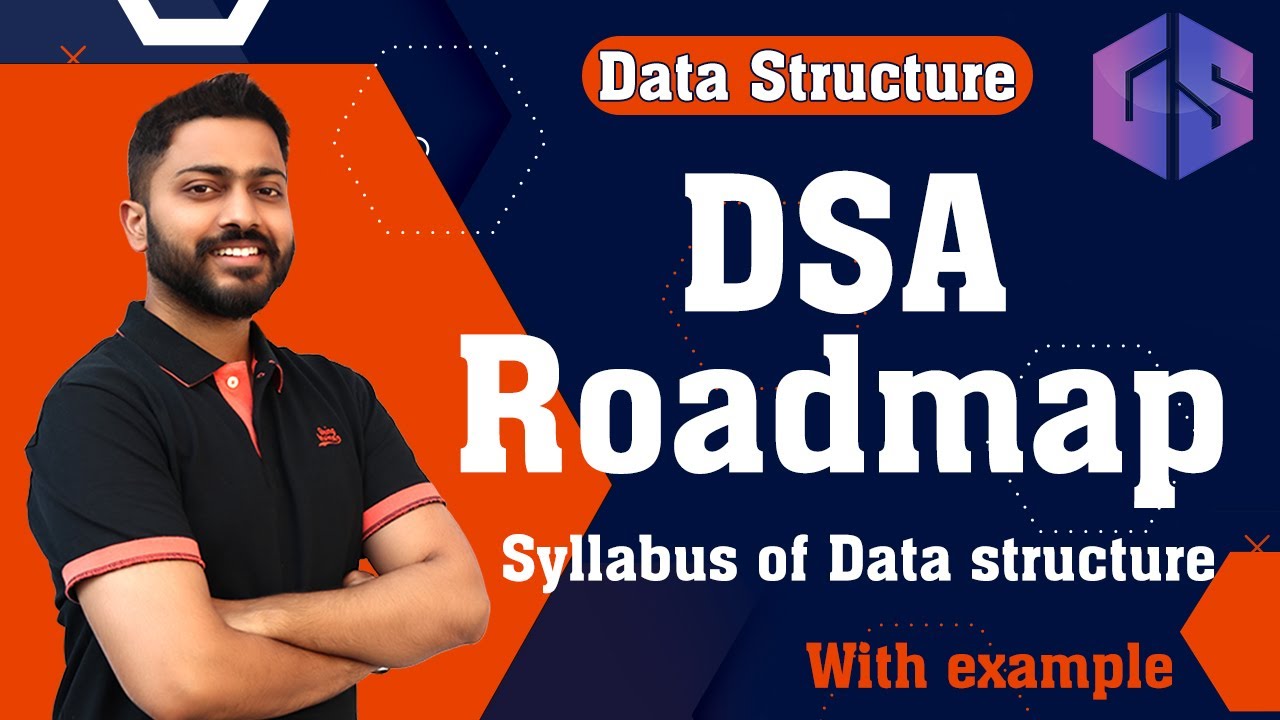
Roadmap 🛣️ of DSA | Syllabus of Data structure | Data Structure for Beginners
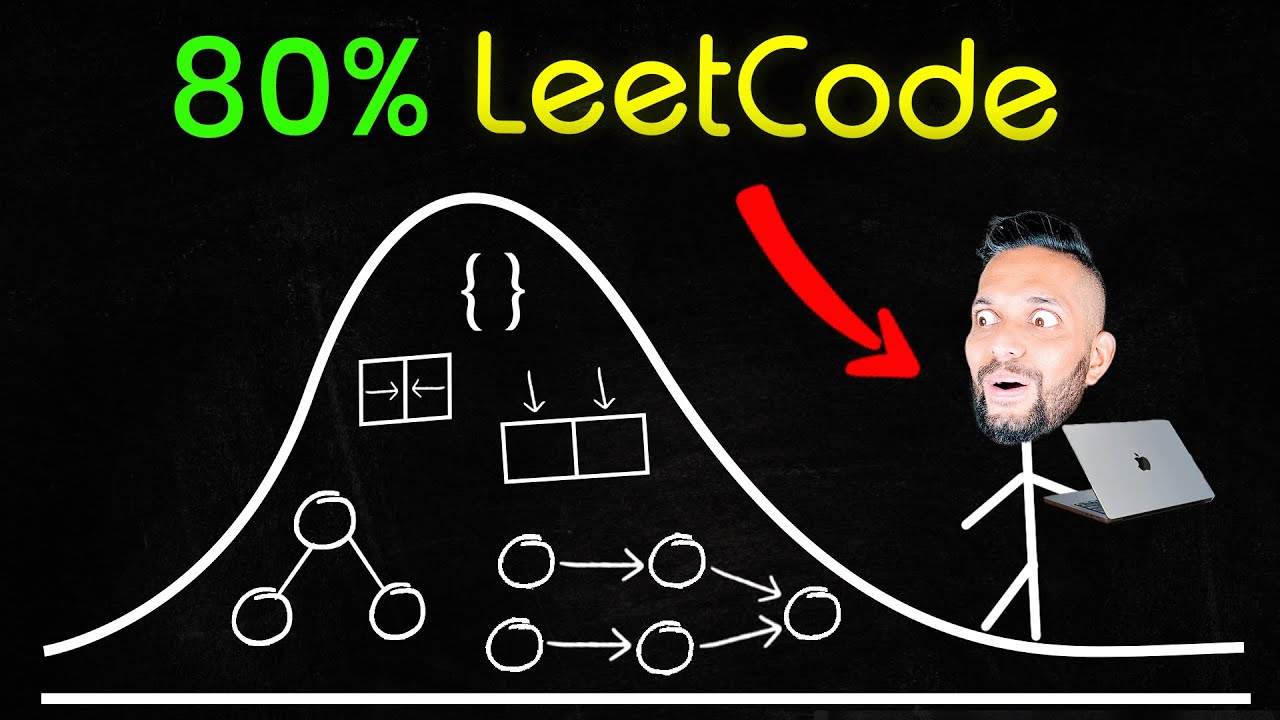
8 patterns to solve 80% Leetcode problems
5.0 / 5 (0 votes)