The byte, short, and long Data Types in Java
Summary
TLDRThis educational video script delves into Java's numeric data types: byte, short, int, and long. It explains the value ranges each type can hold, with byte being the smallest and long the largest. Examples illustrate valid and invalid assignments for each type. The script also covers type conversion rules, showing how smaller types are automatically converted to larger ones during operations, and the necessity of using the letter 'L' for long literals to avoid errors. The lecture concludes with a discussion on type conversion, emphasizing the automatic conversion process and the importance of understanding these rules for error-free programming.
Takeaways
- 😀 Java provides different data types for integers: byte, short, int, and long, each with a specific range of values.
- 🔢 A byte in Java can store values ranging from -128 to 127, which is a smaller range compared to an int.
- ⚠️ Assigning a value outside the byte range, like -129, will result in a compilation error.
- 📏 The short data type has a larger range than a byte but smaller than an int, typically from -32,768 to 32,767.
- 🆚 When a value exceeds the range of a short, it must be explicitly declared as a long by appending the letter 'L'.
- 🔑 The long data type is the largest among the integer types and can store values beyond the range of an int.
- 💡 To indicate a long literal in Java, append an 'L' or 'l' to the number to differentiate it from an int.
- 🔄 Type conversion in Java is automatic when assigning a smaller data type to a larger one, like byte to int.
- ❌ Direct assignment from a larger data type to a smaller one, such as long to int, is not allowed and will cause an error.
- 🔍 The script emphasizes the importance of understanding data type ranges and conversions for error-free Java programming.
Q & A
What is the range of values that can be stored in a byte variable in Java?
-A byte variable in Java can store values ranging from -128 to 127.
Why would assigning -129 to a byte variable in Java cause an error?
-Assigning -129 to a byte variable in Java would cause an error because -129 is outside the valid range for byte data type, which is from -128 to 127.
What is the purpose of the 'short' data type in Java?
-The 'short' data type in Java is used to store integer values with a larger range than a byte but smaller than an int, specifically from -32,768 to 32,767.
How does the range of the 'short' data type compare to that of a 'byte' and an 'int'?
-The 'short' data type has a larger range than a 'byte' but a smaller range than an 'int'. It can store values from -32,768 to 32,767, which is more than a byte but less than an int.
What is the significance of the letter 'L' when declaring a long variable in Java?
-In Java, the letter 'L' is used to denote that a number is of the 'long' data type. It is necessary to append 'L' to a number when declaring a long variable to differentiate it from an 'int'.
Why is it important to use 'L' when assigning a value to a long variable that exceeds the range of an int?
-Using 'L' when assigning a value to a long variable that exceeds the range of an int is important because without 'L', Java would interpret the number as an int, which could lead to an error if the value is too large to fit in an int.
What is the range of values that can be stored in a long variable in Java?
-A long variable in Java can store values ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Can an integer variable in Java store a value that is too large for an int but fits within the range of a long?
-No, an integer variable in Java cannot store a value that is too large for an int. If a value exceeds the range of an int, it must be stored in a long variable with the 'L' suffix.
What happens during type conversion when adding a byte and a short in Java?
-During type conversion when adding a byte and a short in Java, the byte is automatically promoted to a short before the addition takes place, and the result is stored in a short variable if the result fits within the short range.
How does Java handle type conversion when assigning values across different numeric types?
-Java automatically handles type conversion by promoting smaller types to a larger type when the result of an expression requires it. However, when assigning a value to a smaller type from a larger one, explicit casting might be necessary to avoid errors.
Outlines
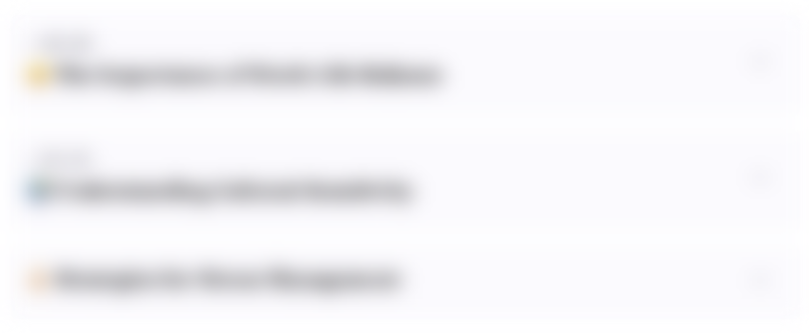
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
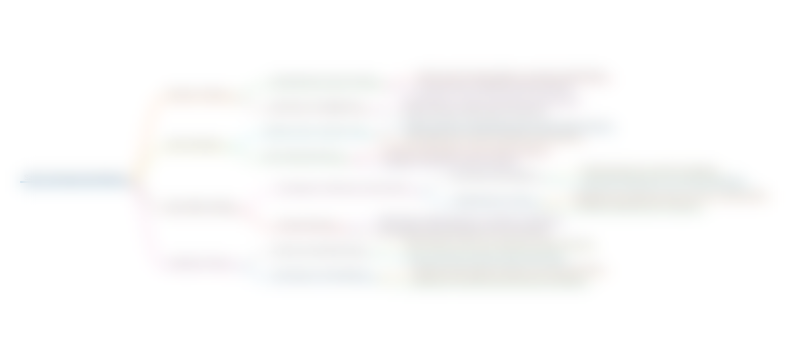
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
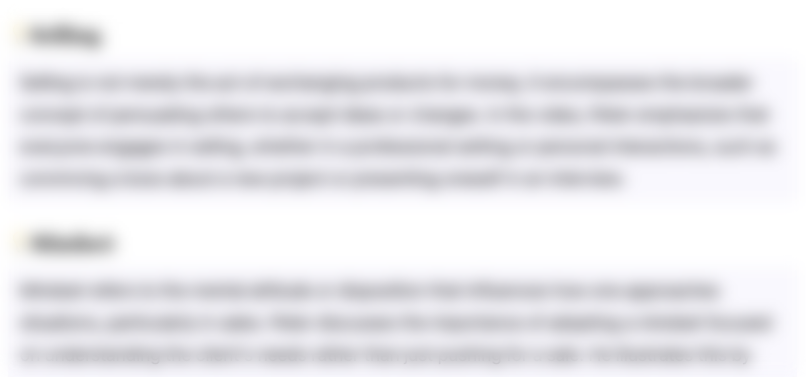
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
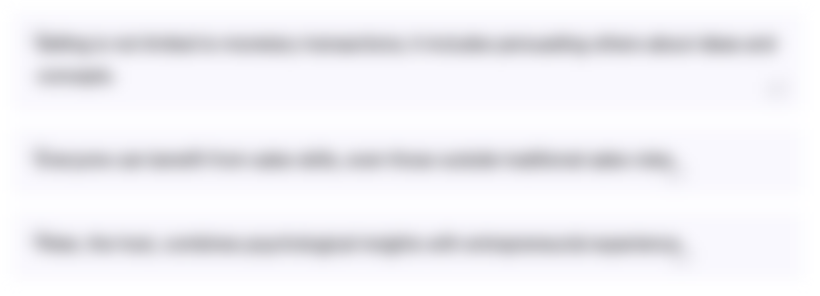
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
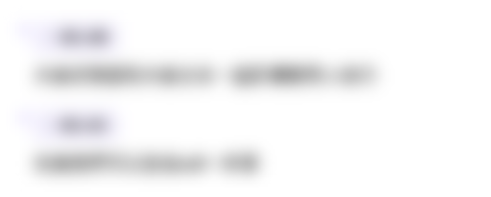
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)