How Events Work in React | Lecture 134 | React.JS 🔥
Summary
TLDRThis lecture delves into how React handles events and their underlying mechanisms, starting with a primer on event propagation and delegation in the DOM to lay the groundwork for understanding React's approach. It explains the process of events bubbling through the DOM tree and introduces the concept of event delegation for efficient event handling across multiple elements. The discussion transitions to React's unique handling of events, highlighting the use of synthetic events to normalize browser inconsistencies and the practice of attaching event handlers to the root container for performance gains. The lecture concludes by contrasting event handling in React with vanilla JavaScript, emphasizing differences in syntax, event object handling, and phase capturing.
Takeaways
- 👣 Events in the browser follow a propagation pattern: capturing phase (top to target) and bubbling phase (target to top).
- 🎯 By default, event handlers listen to events during the bubbling phase, allowing parent elements to handle child events.
- 🧩 Event delegation allows handling events for multiple elements in one central place, improving performance and memory usage.
- 🃏 In React, event handlers are registered to the root DOM container, not individual elements, utilizing event delegation behind the scenes.
- ⚡ React creates a synthetic event object, a wrapper around the native DOM event, to fix browser inconsistencies and ensure consistent event behavior.
- 🔀 React bubbles focus, blur, and change events, which normally don't bubble, except for the scroll event.
- 🐫 React uses camelCase for event handler prop names (e.g., onClick), unlike vanilla JavaScript (onclick or click).
- 🚫 To prevent default browser behavior in React, use event.preventDefault(), as returning false from the handler doesn't work.
- 🔍 Attach 'Capture' to the event handler name (e.g., onClickCapture) to handle events in the capturing phase, though rarely used.
- 🧠 Understanding event propagation and React's event handling mechanisms helps diagnose and fix strange event-related behaviors in applications.
Q & A
What is event propagation in the context of the DOM?
-Event propagation is the process by which an event travels through the DOM tree. It starts at the root of the document, goes down to the target element during the capturing phase, and then bubbles back up to the root during the bubbling phase.
How does event delegation work in the DOM and why is it useful?
-Event delegation is a technique where event listeners are added to a parent element instead of individual child elements. It's useful for handling events on multiple elements in one central place, improving app performance and memory usage, especially with a large number of elements.
How does React handle events differently from vanilla JavaScript?
-React handles events by registering all event handler functions to the root DOM container of the React app instead of directly on the target element. This approach allows React to perform event delegation automatically for all events in applications.
What is the significance of the capturing and bubbling phases in event propagation?
-The capturing and bubbling phases are important for understanding how events travel through the DOM tree. The capturing phase is when the event travels down to the target element, and the bubbling phase is when it travels back up. This process allows for more flexible event handling strategies.
Why might you want to prevent an event from bubbling in a React application?
-Preventing an event from bubbling can be necessary to stop the event from being handled by parent elements' event handlers when such behavior is not desired. It's done by calling the stopPropagation method on the event object, although it's rarely necessary.
What are synthetic events in React and why are they used?
-Synthetic events in React are wrappers around the browser's native event objects. They provide a consistent interface across different browsers, fixing browser inconsistencies and including some additional functionalities. This makes event handling in React more reliable and consistent.
How does React's event handling improve app performance?
-React's event handling improves app performance by using event delegation at the root DOM container. This means React only needs to register one event handler per event type, reducing the number of event listeners and simplifying event management across the application.
In React, how can you stop the default behavior triggered by an event?
-In React, to stop the default behavior triggered by an event, you must call the preventDefault method on the synthetic event object. Returning false from an event handler function, as you might in vanilla JavaScript, does not work.
What is the difference between the way event handlers are named in React and in vanilla JavaScript?
-In React, event handler props are named using camelCase, like onClick, while in HTML it would be all lowercase (onclick), and in vanilla JavaScript addEventListener method, the event is referred to simply by its type, like 'click', without the 'on' prefix.
How can you handle an event in the capturing phase instead of the bubbling phase in React?
-In React, to handle an event in the capturing phase, you can attach 'Capture' to the event handler name, for example, using onClickCapture instead of just onClick. This allows you to catch events in the capturing phase rather than the default bubbling phase.
Outlines
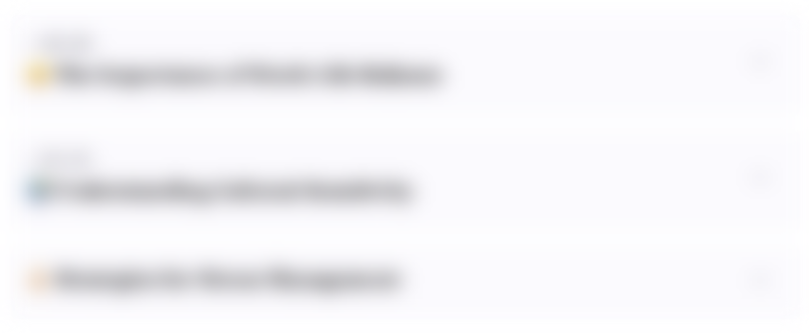
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
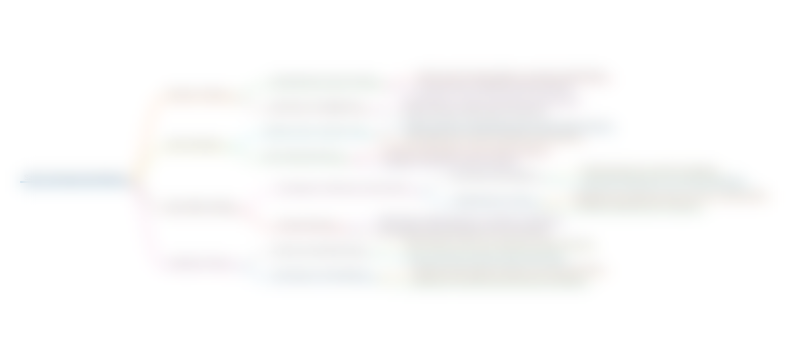
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
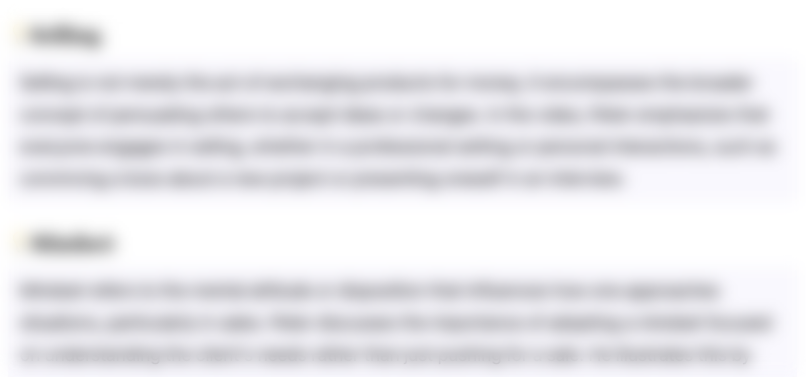
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
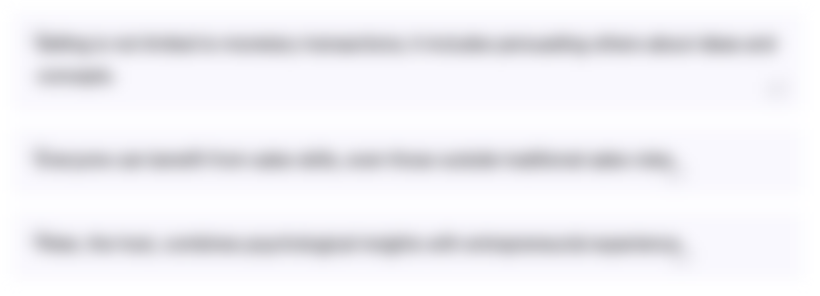
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
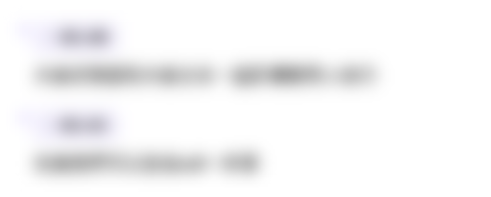
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

State Update Batching in Practice | Lecture 133 | React.JS 🔥

How Rendering Works: Overview | Lecture 123 | React.JS 🔥

How Rendering Works: The Render Phase | Lecture 124 | React.JS 🔥

Multiple ways of Fetching Events in React #knowledgekeen
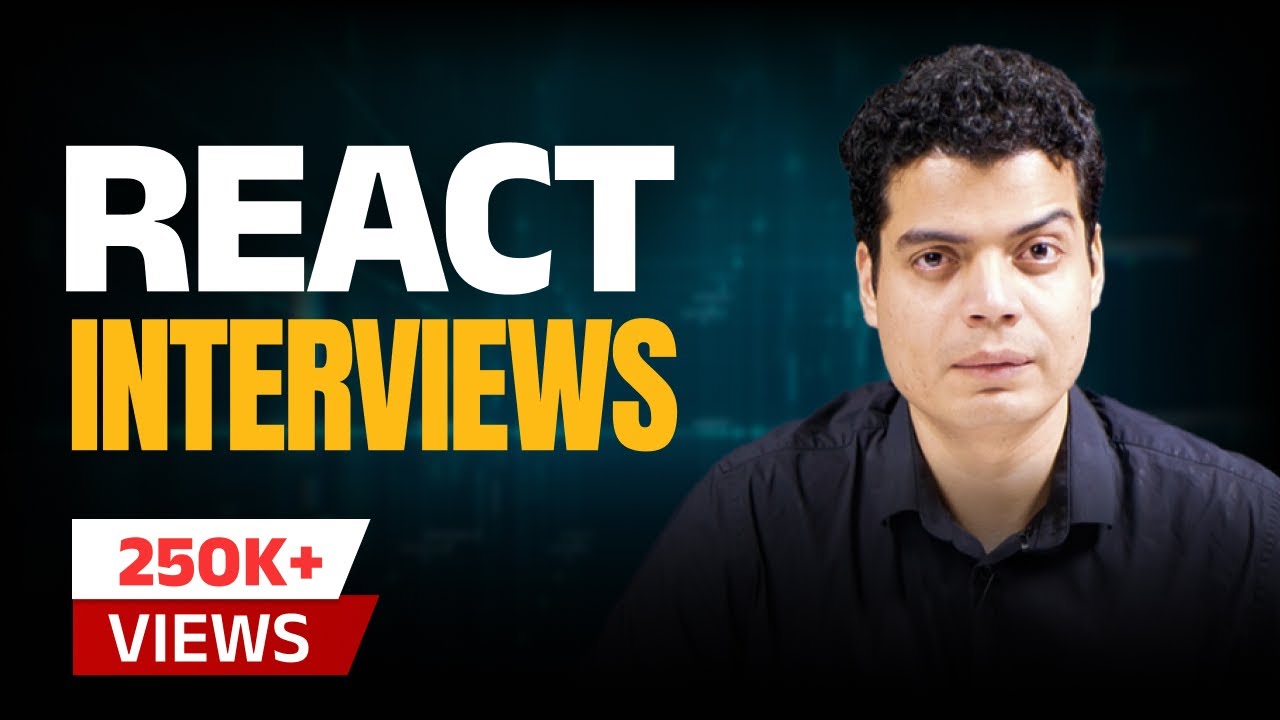
Mastering React: Decoding 09 Most Common Interview Questions | Tanay Pratap Hindi
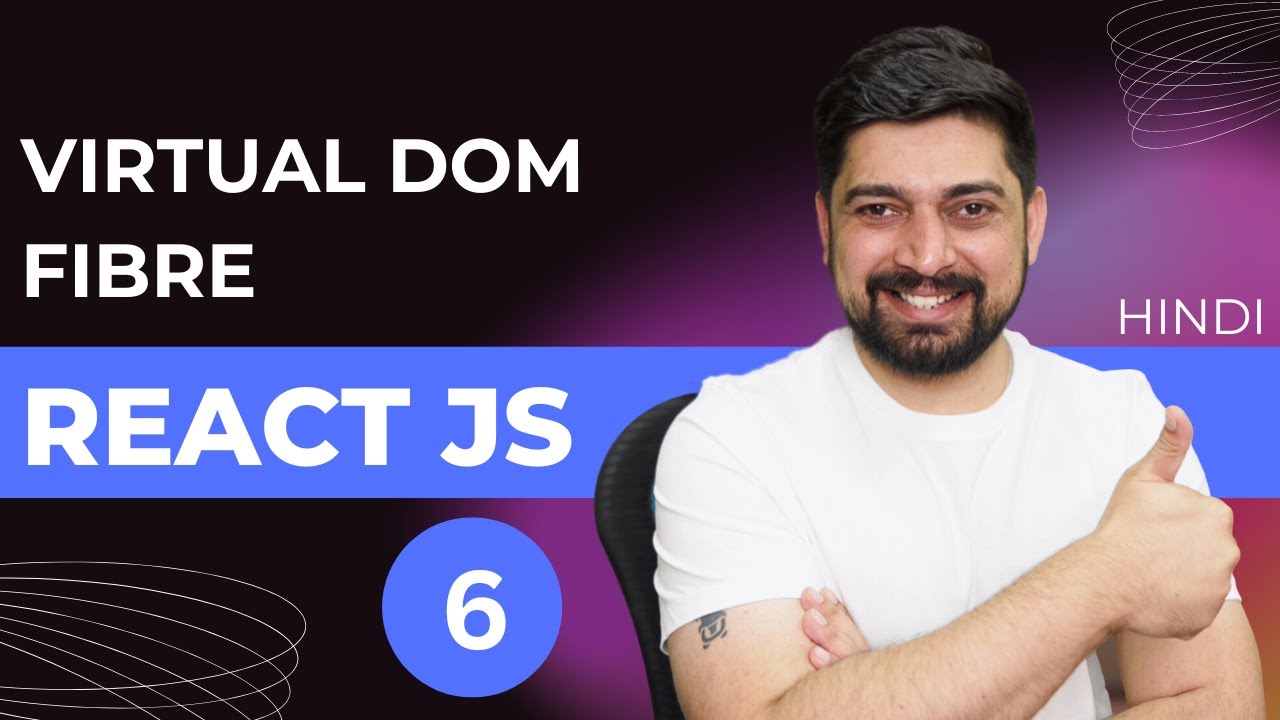
06 Virtual DOM, Fibre and reconciliation
5.0 / 5 (0 votes)