How to use RxJS with React Hooks
Summary
TLDRThis video script delves into the integration of RxJS, a reactive extensions library for JavaScript, with React hooks. It begins by explaining observables, the core concept behind RxJS, and their advantages over promises. The script then demonstrates how to use observables with React, first through class components and then with hooks, showcasing the simplicity and conciseness of the latter approach. A real-world example of an auto-suggestion feature is implemented, illustrating the powerful capabilities of RxJS operators like debounce, filter, and mergeMap. The script highlights the benefits of RxJS, such as lazy execution and efficient handling of asynchronous data streams, making it an invaluable tool for building reactive applications.
Takeaways
- 😀 RxJS is a reactive extensions library for JavaScript that uses Observables for reactive programming.
- 🤔 Observables are an invokeabledcollection of future values or events that can emit multiple values over time, unlike Promises which resolve only once.
- 🧐 Observables are being standardized into ECMAScript, though the proposal is currently stalled at stage 1.
- 📖 Key RxJS concepts: Observables, Operators (modification functions), Subscriptions, Subjects (preset pipelines).
- 🚀 Using RxJS with React Hooks is cleaner and more concise than class components.
- ✨ Custom Hooks like useObservable can abstract away RxJS subscription logic.
- 🔑 Operators like filter, debounceTime, distinctUntilChanged, and mergeMap enable powerful async data handling.
- 📡 RxJS enables efficient querying by preventing unnecessary server requests and computations.
- 🌐 RxJS has a large community and many third-party libraries like RxJS Hooks to simplify integration.
- 🛠 Real-world use cases for RxJS include auto-suggestions, search functionalities, and handling async data streams.
Q & A
What is RxJS and its primary purpose?
-RxJS is a reactive programming library for JavaScript, focusing on using observables to handle asynchronous and event-based programs.
How do observables differ from promises?
-Unlike promises, which can only resolve once, observables can emit multiple values over time, making them suitable for handling multiple asynchronous events.
What is the current standardization status of observables in ECMAScript?
-As of the script's context, observables are in the process of being standardized in ECMAScript, but they are stuck at stage one due to some issues with the proposal.
How do you create an observable from an array using RxJS?
-You can create an observable from an array using the `from` operator in RxJS, allowing the observable to emit each element of the array over time.
What are RxJS operators and how are they used?
-RxJS operators are functions used for modifying, filtering, and transforming data streams in observables. They are used in a pipeline with the `pipe` method to operate on the items emitted by observables.
What does it mean when observables are described as lazy?
-Observables are considered lazy because they do not start emitting values until a subscriber has subscribed to them, allowing for efficient use of resources.
How can you manage observable subscriptions in React class components?
-In React class components, observable subscriptions are typically managed within the `componentDidMount` method to subscribe and `componentWillUnmount` method to unsubscribe, preventing memory leaks.
What advantage do React hooks provide when using observables in functional components?
-React hooks simplify the integration with observables in functional components by allowing subscriptions within the `useEffect` hook, making the code less verbose and easier to manage.
How can you implement an auto-suggest feature using RxJS and React?
-An auto-suggest feature can be implemented using RxJS by creating a stream of search text inputs, debouncing them to limit the rate of API calls, and then transforming these inputs into search results through observables.
Why are subjects used in RxJS, particularly for implementing a search feature?
-Subjects in RxJS act as both observers and observables. They are used for multicast communication among multiple subscribers, making them ideal for implementing features like search where user inputs need to be broadcasted to multiple observers.
Outlines
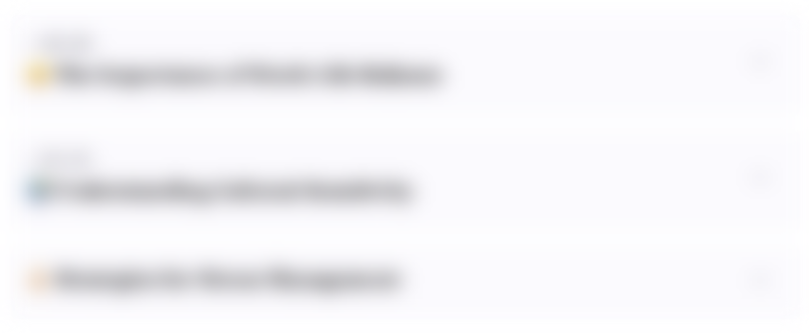
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
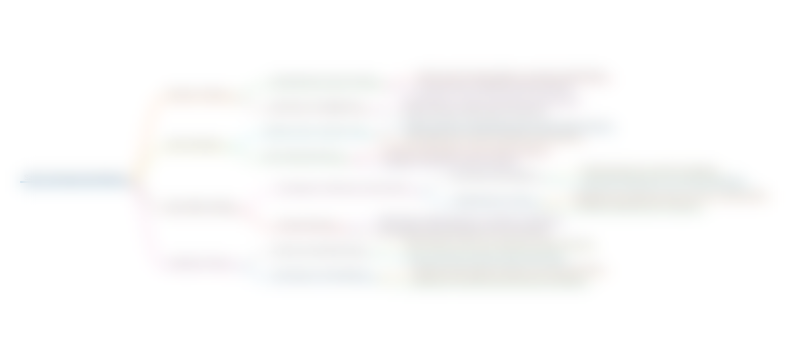
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
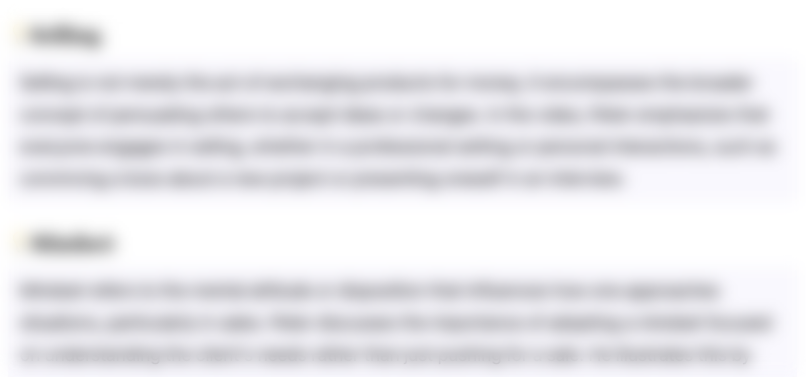
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
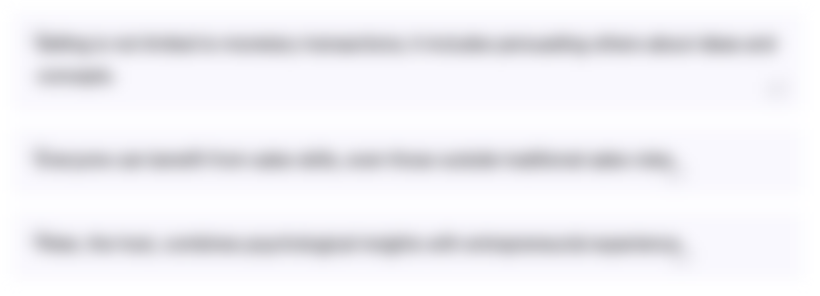
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
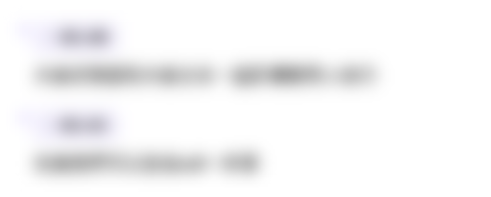
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
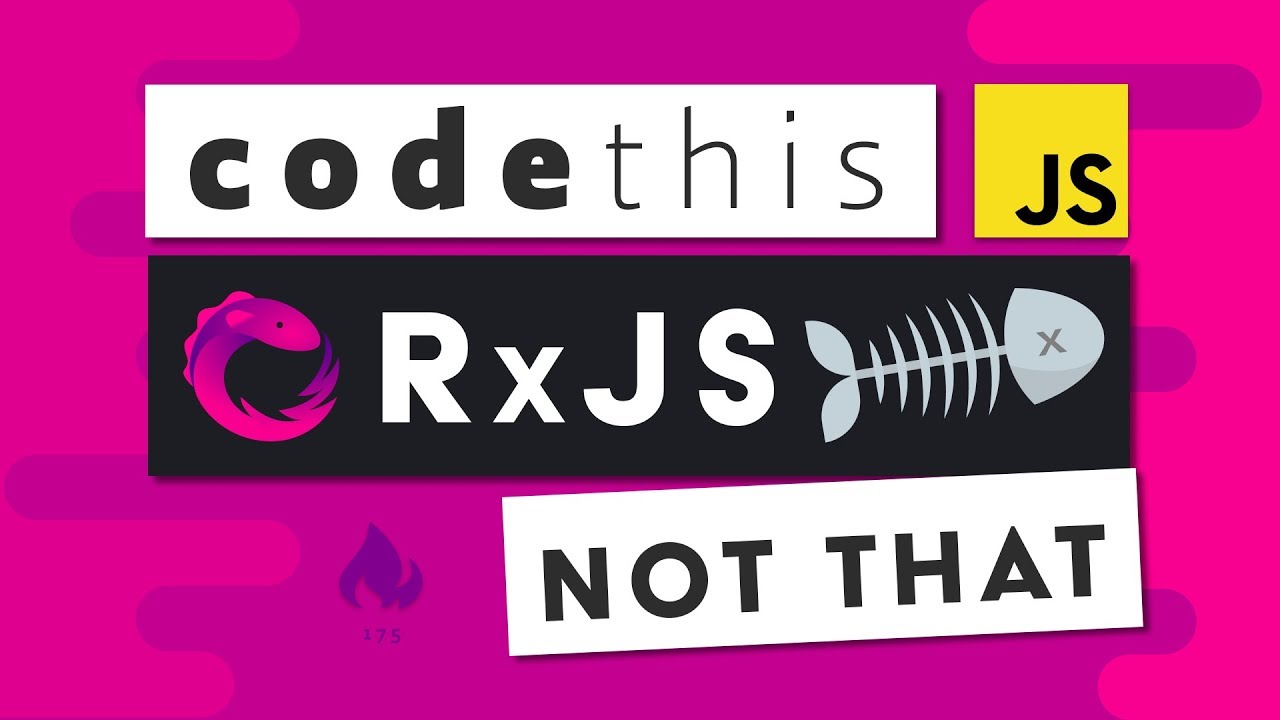
RxJS Top Ten - Code This, Not That
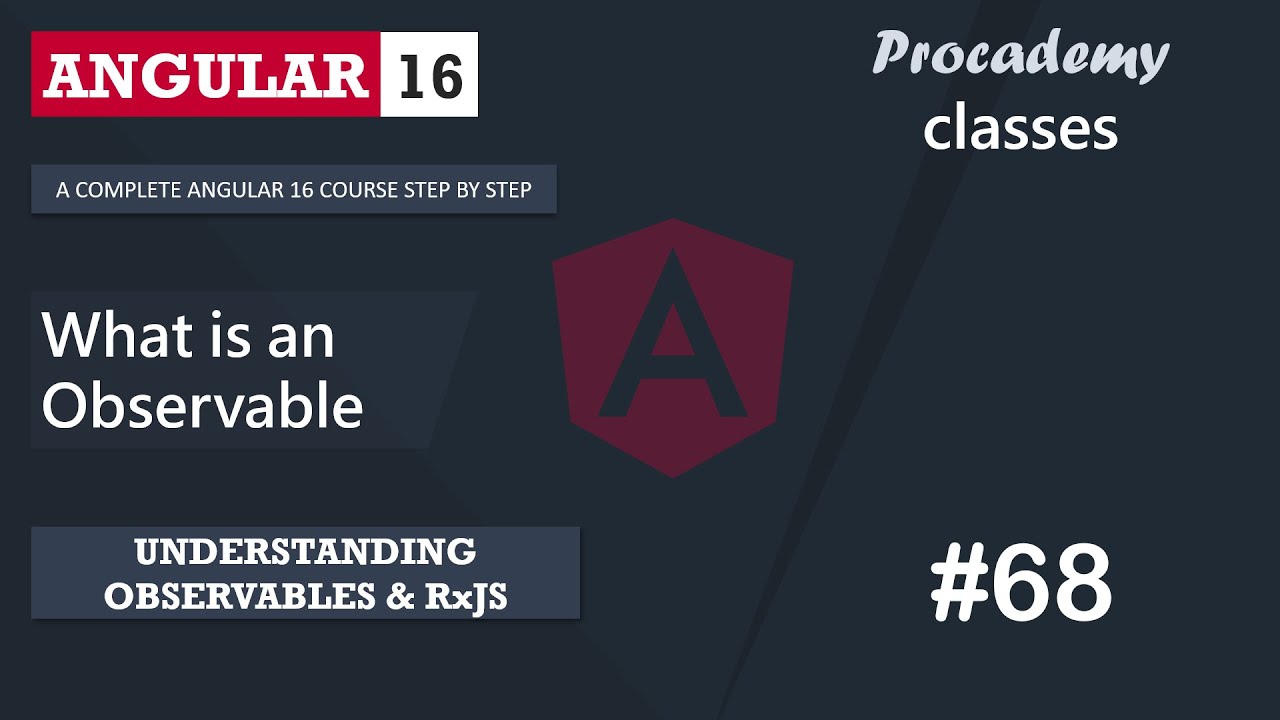
#68 What is an Observable | Understanding Observables & RxJS | A Complete Angular Course
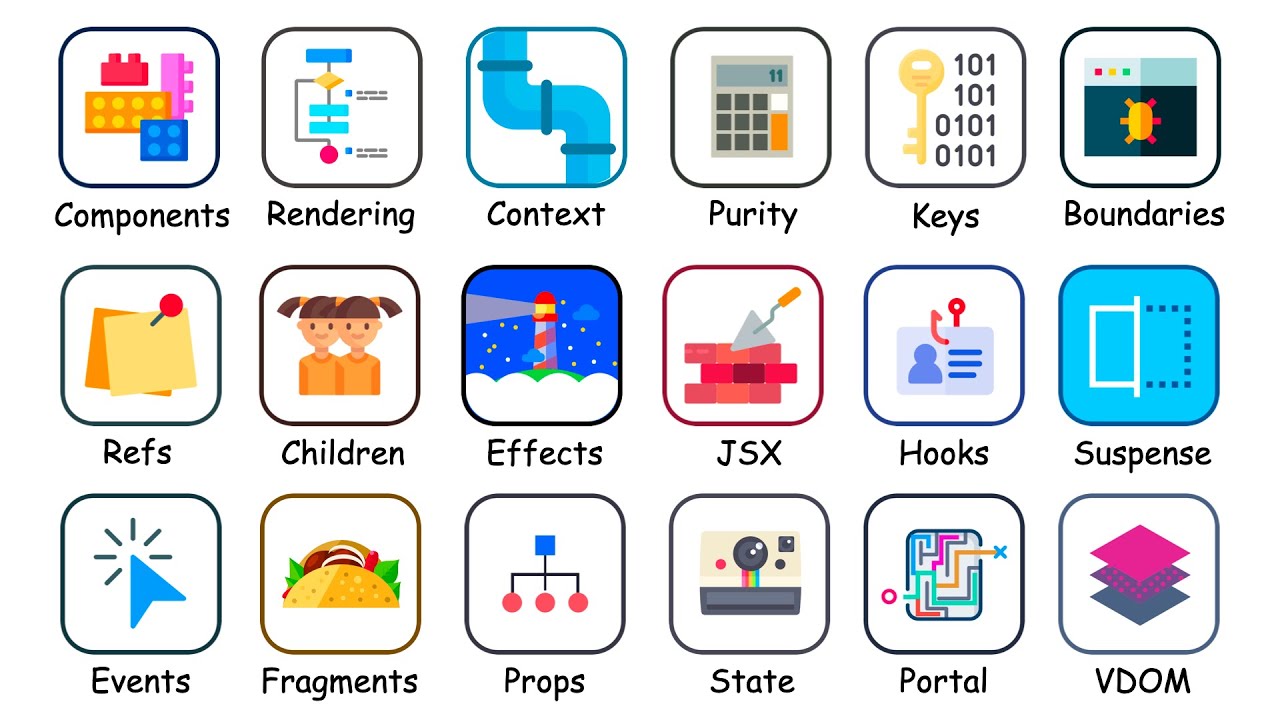
Every React Concept Explained in 12 Minutes
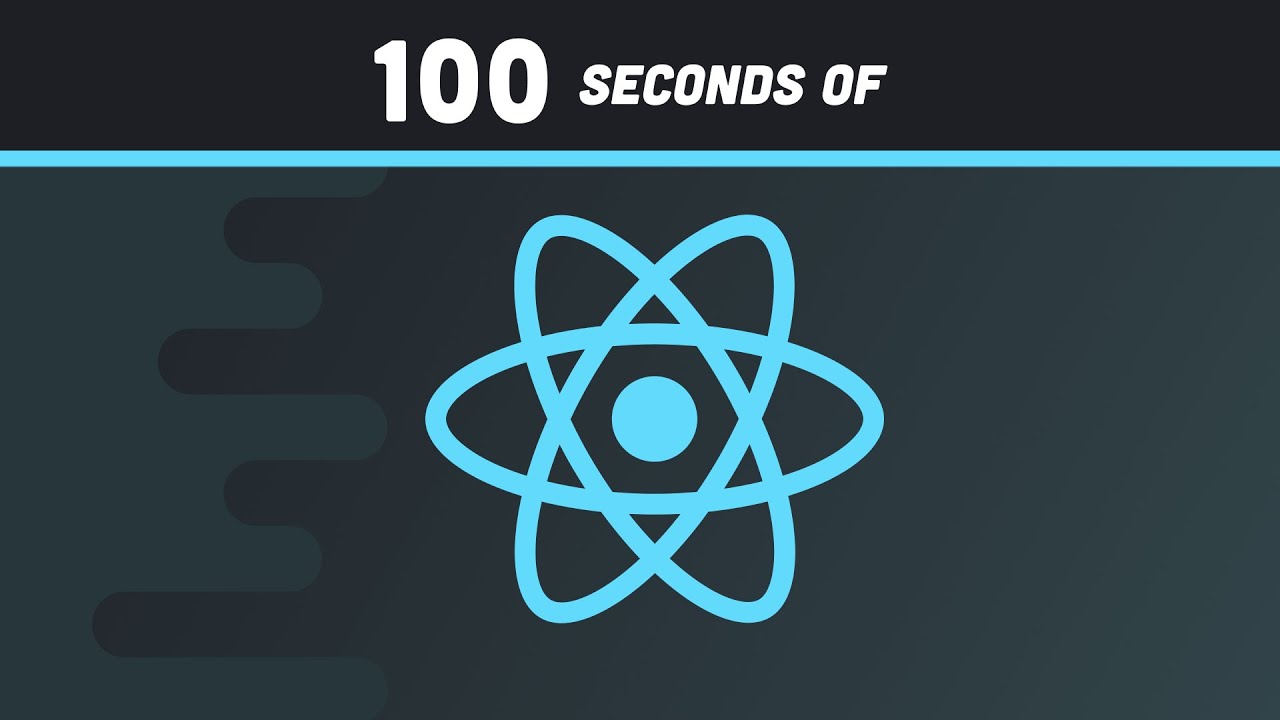
React in 100 Seconds
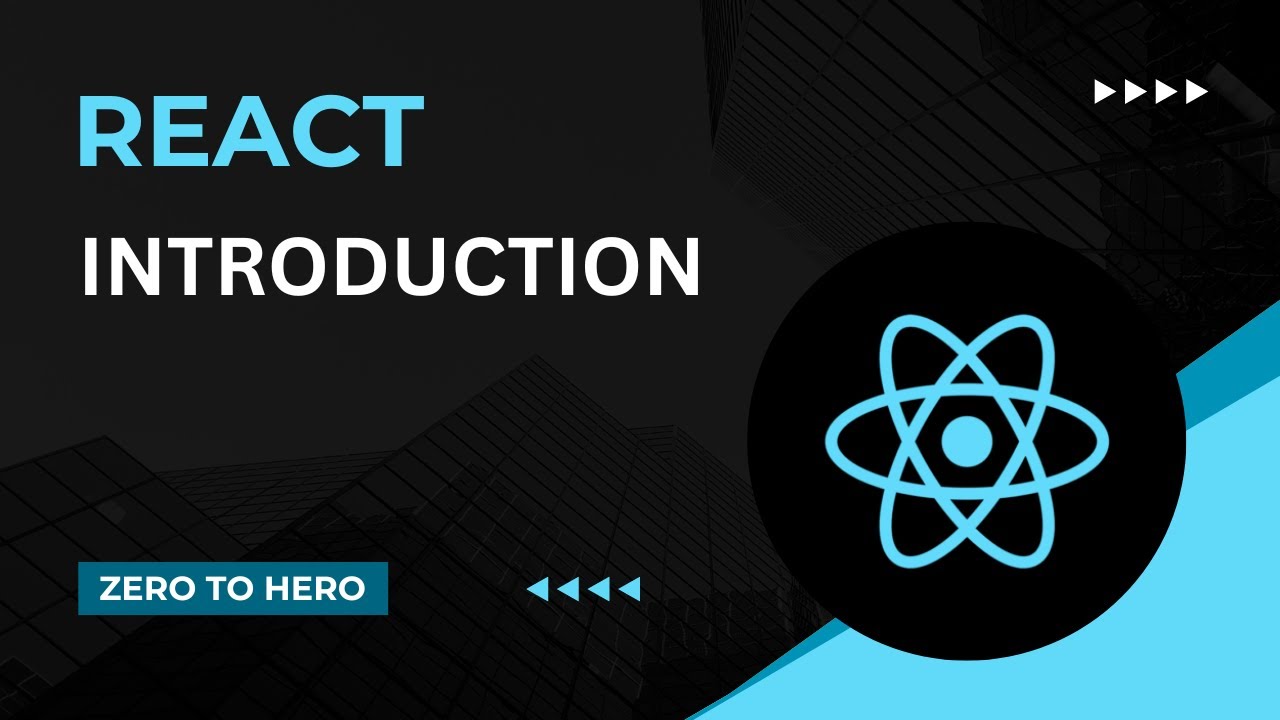
Course Introduction | Mastering React: An In-Depth Zero to Hero Video Series

Libraries vs Frameworks & The React Ecosystem | Lecture 135 | React.JS 🔥
5.0 / 5 (0 votes)