When to use take() vs takeUntilDestroyed()?
Summary
TLDRThis video explores the use of RxJS operators 'take' and 'takeUntilDestroyed' with HTTP requests in Angular. It explains how 'take' limits the number of emissions from an observable and is ideal for infinite streams, while 'takeUntilDestroyed' prevents memory leaks by unsubscribing when a component or service is destroyed. The script clarifies that 'take' is unnecessary for one-time HTTP requests like GET, but 'takeUntilDestroyed' can be used to cancel pending requests on navigation. The video includes code examples and a deep dive into Angular and RxJS source code for a comprehensive understanding.
Takeaways
- π The RxJS `take` operator emits a specified number of items from an observable and then automatically completes.
- π When using `take` with an HTTP request, it doesn't serve a purpose since HTTP requests are inherently one-time operations that complete after emitting a single response.
- π οΈ The `takeUntilDestroyed` operator is introduced in Angular 16 to automatically complete the observable when a component or service is destroyed, preventing memory leaks.
- π« It's advised against using `takeUntilDestroyed` with HTTP `PUT`, `POST`, or `DELETE` requests because these operations should not be canceled when the user navigates away.
- π The script demonstrates how the order of operators affects the output, showing that `take` can behave differently depending on its position in the observable pipeline.
- π The source code of Angular and RxJS is explored to understand how HTTP requests are handled and how observables are completed after a response.
- π The `take` operator is useful for limiting the number of emissions from infinite or long-running observables, such as those created by `timer` or `subject`.
- π The script includes a practical example using a to-do list application to illustrate the effects of the `take` operator on observables.
- β For one-time HTTP `GET` requests, `take` does not change behavior, but `takeUntilDestroyed` can be used to cancel the request if the user navigates away before the response is received.
- π The video encourages viewers to like and subscribe if they find the content useful, highlighting the importance of community engagement for educational content.
Q & A
What is the primary function of the RxJS 'take' operator?
-The 'take' operator emits a specified number of items from an observable and automatically completes after taking the specified number of items. It is useful for limiting the number of emissions from an observable.
How does the 'take' operator interact with other operators in a pipeline?
-The 'take' operator takes the specified number of items from an observable, regardless of whether they pass through filters or not. Once the specified number is reached, the observable completes, even if subsequent items pass through filters.
What is the difference between using 'take' and 'takeUntil' operators with HTTP requests in Angular?
-HTTP requests in Angular are typically one-time operations that complete after emitting a single response. Using 'take(1)' with these requests has no effect since the observable completes after the first emission. 'takeUntilDestroyed' is used to automatically complete the observable when the component or service is destroyed, preventing memory leaks.
Why might 'takeUntilDestroyed' be used with an HTTP GET request?
-While 'takeUntilDestroyed' doesn't prevent the emission of the response in an HTTP GET request, it can be used to cancel the request if the user navigates away before the data is returned, preventing unnecessary processing or subscription handling when the data is eventually returned.
What is the significance of the 'takeUntilDestroyed' operator in Angular applications?
-The 'takeUntilDestroyed' operator is used to automatically unsubscribe from observables when a component or service is destroyed, which helps prevent potential memory leaks and eliminates the need for manual unsubscription.
Why should 'takeUntilDestroyed' not be used with HTTP PUT, POST, or DELETE requests?
-Since HTTP PUT, POST, or DELETE requests are intended to modify data, using 'takeUntilDestroyed' could inadvertently cancel these operations if the user navigates away, which is generally not the desired behavior for modification requests.
How does the 'take' operator handle the order of emissions in an observable sequence?
-The 'take' operator takes the specified number of items from the observable sequence in the order they are emitted, regardless of any filters applied. Once the specified count is reached, the observable completes.
What is the role of the 'filter' operator in the observable pipeline demonstrated in the script?
-The 'filter' operator in the observable pipeline is used to selectively pass items through the pipeline based on a condition. Items that do not meet the condition are not emitted by the pipeline, even if they are taken by the 'take' operator.
What does the script reveal about the internal workings of HTTP requests in Angular?
-The script reveals that Angular's HTTP client sets up an observable for each request, which emits the response and then completes. This behavior means that operators like 'take(1)' do not change the observable's behavior for HTTP requests.
How does the script demonstrate the practical use of RxJS operators in an Angular application?
-The script demonstrates the use of RxJS operators in an Angular application by showing how they can be applied to a to-do list component. It shows how operators like 'take' and 'filter' can be used to control the flow and processing of data in the application.
Outlines
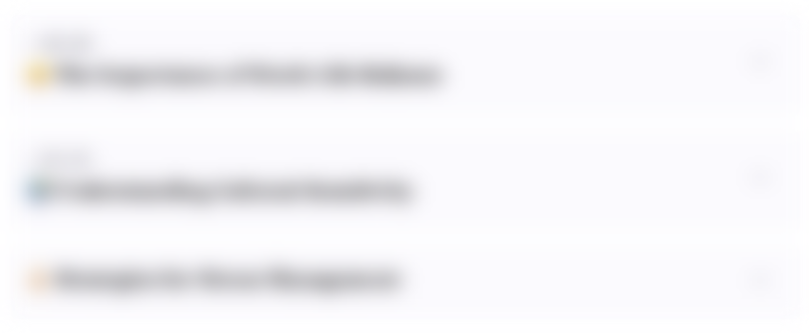
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
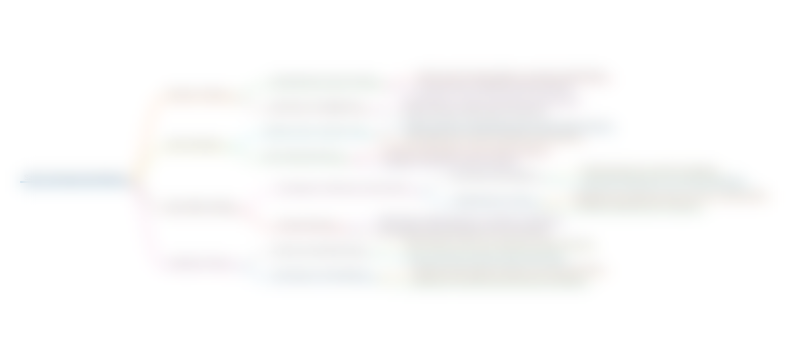
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
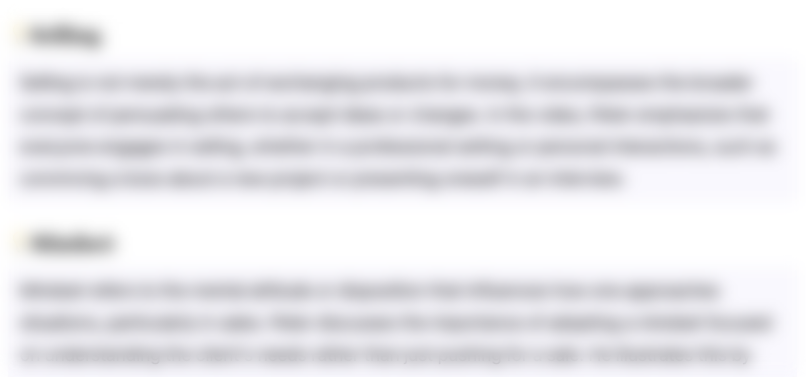
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
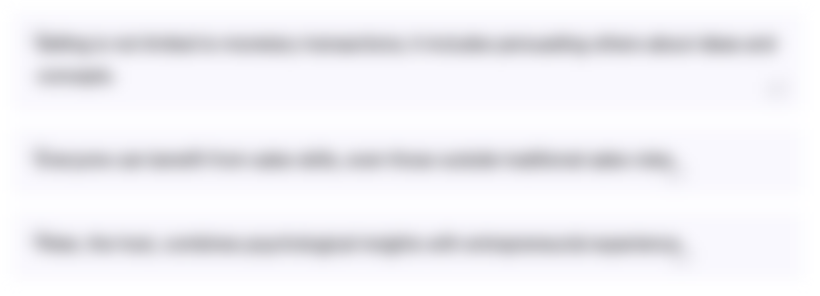
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
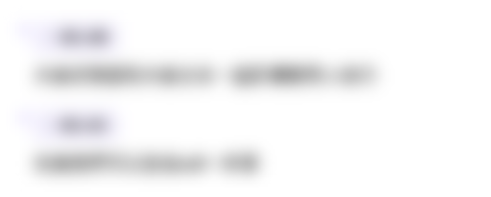
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)