This is the Only Right Way to Write React clean-code - SOLID
Summary
TLDRThis tutorial dives deep into the SOLID principles in React, focusing on how to build maintainable, testable, and scalable components. It covers each principle — Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion — with practical examples. By applying these principles, developers can create reusable, flexible components that are easier to test and extend. The video emphasizes improving code organization by abstracting logic and avoiding unnecessary dependencies, making it a valuable guide for developers looking to enhance their React applications with clean, effective code design.
Takeaways
- 😀 The SOLID principles are essential for writing maintainable, scalable, and testable React applications.
- 😀 Single Responsibility Principle (SRP) suggests that each component should only handle one responsibility to enhance readability and maintainability.
- 😀 Open/Closed Principle (OCP) emphasizes extending components instead of modifying them, allowing for flexible updates without breaking existing code.
- 😀 Liskov Substitution Principle (LSP) ensures that custom components can be substituted for their base components without affecting functionality or causing bugs.
- 😀 Interface Segregation Principle (ISP) advocates that components should only depend on the data they need, reducing unnecessary complexity and dependencies.
- 😀 Dependency Inversion Principle (DIP) encourages passing abstractions (like functions) instead of directly relying on concrete implementations, enhancing flexibility and testability.
- 😀 Refactoring large components into smaller ones that handle specific responsibilities follows SRP and increases code modularity.
- 😀 Using props to extend functionality (like passing icons to a button component) adheres to OCP and avoids modifying core components.
- 😀 Ensuring that child components only receive necessary data and props aligns with ISP, preventing over-reliance on unnecessary data.
- 😀 By abstracting functionality (like form submission), components can be reused in various contexts without altering the core logic, following DIP principles.
- 😀 Proper application of SOLID principles in React enhances the code’s scalability, reusability, and reduces complexity, making the app easier to maintain over time.
Q & A
What are the SOLID principles?
-The SOLID principles are a set of five design principles aimed at making software more understandable, maintainable, and flexible. They are: Single Responsibility Principle (SRP), Open/Closed Principle (OCP), Liskov Substitution Principle (LSP), Interface Segregation Principle (ISP), and Dependency Inversion Principle (DIP).
What does the Single Responsibility Principle (SRP) entail in React development?
-In React, the Single Responsibility Principle (SRP) means that a component should only handle one specific task. For example, data fetching, rendering UI, and filtering data should be split into different components and hooks to keep the code modular and easier to maintain.
How does SRP apply to React components?
-SRP in React is applied by breaking down large components into smaller, more focused components. For instance, creating a separate 'Product' component for rendering product details, and using custom hooks like 'useProducts' for fetching data. This separation of concerns improves reusability and clarity.
What is the Open/Closed Principle (OCP) in React?
-The Open/Closed Principle (OCP) in React states that components should be open for extension but closed for modification. This can be achieved by using props and abstraction to add new functionality without altering the existing code of a component.
Can you provide an example of applying OCP in React?
-An example of applying OCP is creating a 'Button' component that accepts props like 'icon' or 'role', allowing it to be extended in various ways (e.g., adding an icon or changing its behavior) without modifying the core button functionality.
What does the Liskov Substitution Principle (LSP) imply in React?
-LSP in React suggests that components or subcomponents should be interchangeable with their parent components without causing issues. For instance, a 'SearchInput' component should accept all the props of a basic 'input' element and behave as expected when substituted.
How can LSP be applied when creating React components?
-LSP can be applied by ensuring that your components properly handle the props of their parent components. For example, passing all the props of a standard 'input' element to a custom 'SearchInput' component using the 'rest' props technique ensures that it can substitute the original 'input' without any issues.
What is the Interface Segregation Principle (ISP), and how does it relate to React?
-ISP in React dictates that components should only depend on the props they actually use. For example, if a component only needs an image URL, it should not be passed the entire product object. This makes components more focused and reduces unnecessary dependencies.
How does Dependency Inversion Principle (DIP) improve React components?
-DIP promotes the idea that high-level components should not depend on low-level components, but both should rely on abstractions. In React, this means passing functions like 'handleSubmit' as props, rather than hard-coding them inside components, making them more flexible and reusable.
How can the Dependency Inversion Principle be implemented in React forms?
-To implement DIP in React forms, instead of directly defining the submit logic inside the form component, you can pass the submit handler as a prop. This allows the form to be reused with different handlers for various purposes, such as login or registration forms.
Outlines
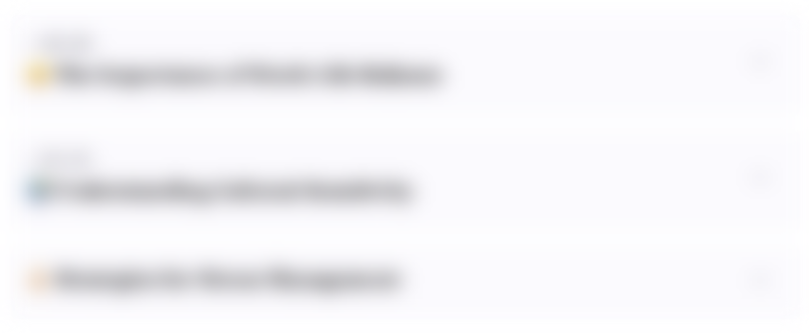
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
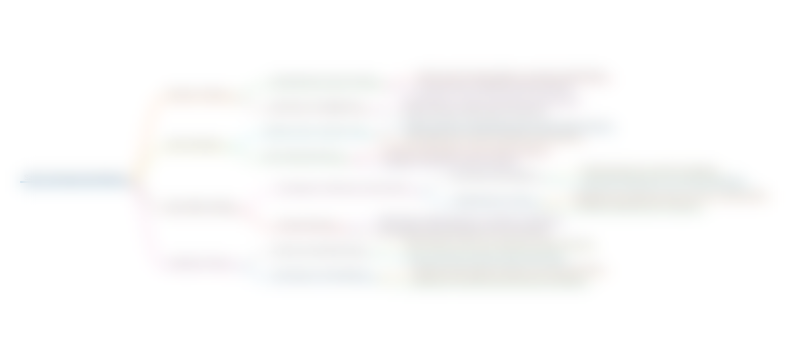
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
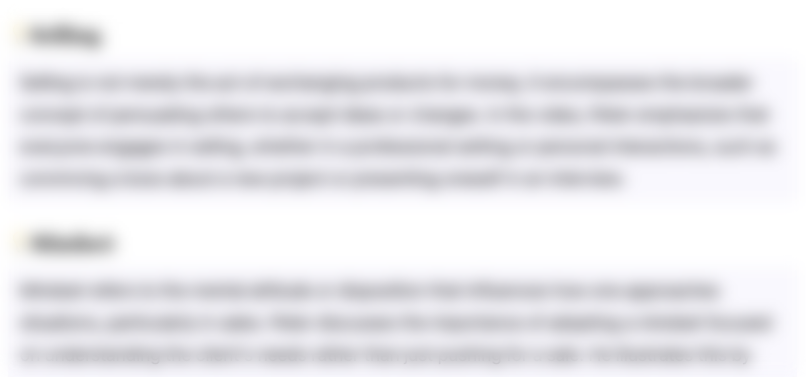
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
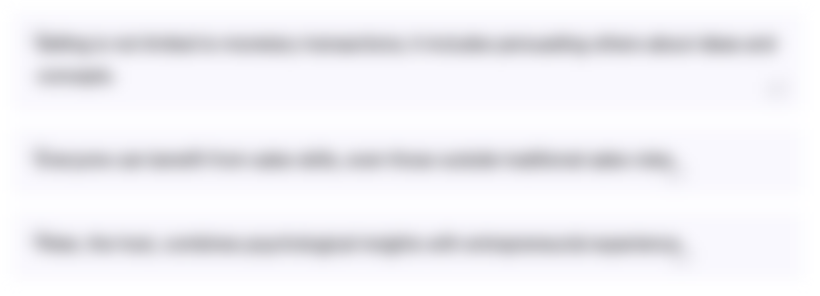
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
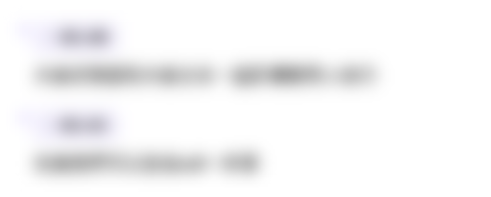
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)