L16 Unit Testing
Summary
TLDRThis tutorial by Phil Copeman delves into the intricacies of unit testing, emphasizing its importance in validating software's detailed design and implementation. It distinguishes between white box and black box testing, highlighting their respective advantages and limitations. The script underscores the significance of coverage metrics like statement, branch, and MCDC coverage, and the necessity of boundary and exceptional value testing. It also advises on best practices, including the use of a unit testing framework and the importance of thorough testing to ensure robust software development.
Takeaways
- π Unit testing is a method to verify that the detailed design and implementation of a software meet the expected outcomes by testing individual components of code.
- π A unit test checks various input combinations against an expected output, using an 'oracle' to determine correctness.
- π Unit tests should cover both structural (white box) and functional (black box) aspects of code to ensure comprehensive testing.
- π Catching boundary-based bugs and slight implementation imperfections is more feasible in unit testing than at the system testing level.
- π« Three anti-patterns in unit testing include not performing unit tests, only testing 'happy paths', and forgetting to test missing code.
- π¦ Black box testing focuses on the software's behavior without knowledge of its implementation, which helps avoid bias but may miss internal code paths.
- π White box testing, also known as structural testing, involves examining the code to ensure all paths and conditions are tested, which can help achieve high code coverage.
- π Coverage metrics are crucial for assessing the thoroughness of testing; they range from function coverage to more granular metrics like statement, branch, and MCDC coverage.
- π Achieving 100% code coverage can be challenging, especially when dealing with error handlers for unlikely events or unused code that should be removed.
- π MCDC (Modified Condition/Decision Coverage) is a sophisticated coverage metric used in safety-critical applications to ensure all possible execution paths are tested.
- π Boundary testing is essential for probing the limits of software behavior, including minimum and maximum values, and exceptional time-related events.
- π Exceptional value testing is vital for ensuring robust systems can handle unexpected inputs such as null pointers, undefined inputs, or unusual events like leap years.
Q & A
What is the primary purpose of unit testing?
-Unit testing is a method to ensure that the detailed design and implementation of a software module meet the expected outcomes by testing individual subroutines, procedures, or methods.
What are the two main types of unit tests mentioned in the script?
-The two main types of unit tests are structure or white box tests, which examine the internal workings of the code, and functionality or black box tests, which focus on the output without considering the internal implementation.
Why is it important to test both 'happy paths' and edge cases in unit testing?
-Testing both 'happy paths' and edge cases is crucial because it helps uncover bugs that may arise due to slight imperfections in the code implementation, which might not be evident during regular operation.
What are the three anti-patterns for unit testing mentioned in the script?
-The three anti-patterns for unit testing are: 1) Not performing unit tests at all, relying only on system-level testing, 2) Testing only the 'happy paths' and not exploring all possible scenarios, and 3) Forgetting to test missing code, such as exception handlers or special conditions that are not present in the code.
What is the difference between black box testing and white box testing?
-Black box testing focuses on the software's behavior without knowledge of the internal implementation, whereas white box testing, also known as structural testing, involves knowledge of the software's actual code structure and is used to ensure all paths and conditions are tested.
Why is coverage a key concept in unit testing?
-Coverage is a metric that measures the thoroughness of testing. High coverage helps ensure that all parts of the code are executed and tested, increasing the likelihood of catching bugs and ensuring the software behaves as expected under various conditions.
What is the significance of boundary testing in unit testing?
-Boundary testing is important because it checks the software's behavior at the limits of its input ranges, such as minimum and maximum values, to ensure there are no unexpected results or errors at these critical points.
What is an assertion in the context of unit testing?
-An assertion is a statement used within a test case to determine whether the test has passed or failed. It checks if the actual output of the tested code matches the expected outcome.
Why is it recommended to use a unit testing framework for unit testing?
-Using a unit testing framework simplifies the process of creating, organizing, and running test cases. It also provides built-in functionalities for assertions and reporting, making it easier to manage and understand the results of the tests.
What are some best practices for writing effective unit tests?
-Best practices for unit testing include testing every module, using a combination of white box and black box testing with granular coverage metrics, breaking tests into small, focused chunks, and ensuring both control flow and data value coverage, including boundary and exceptional value testing.
What are some pitfalls to avoid when performing unit testing?
-Pitfalls to avoid in unit testing include not creating test cases, using a debugger instead of a testing framework, testing large code blobs instead of individual units, relying solely on white box testing, and neglecting peer reviews and static analysis as part of the development process.
Outlines
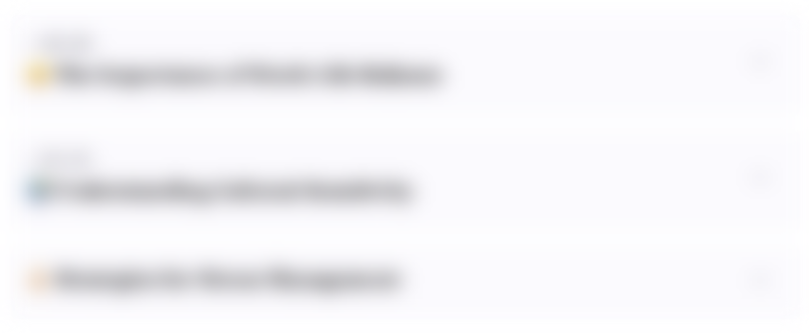
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
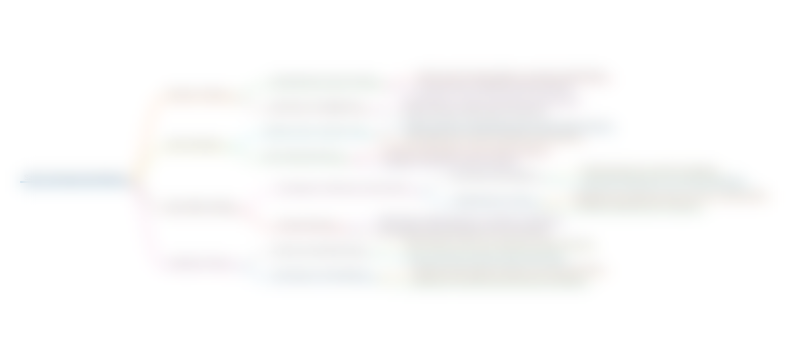
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
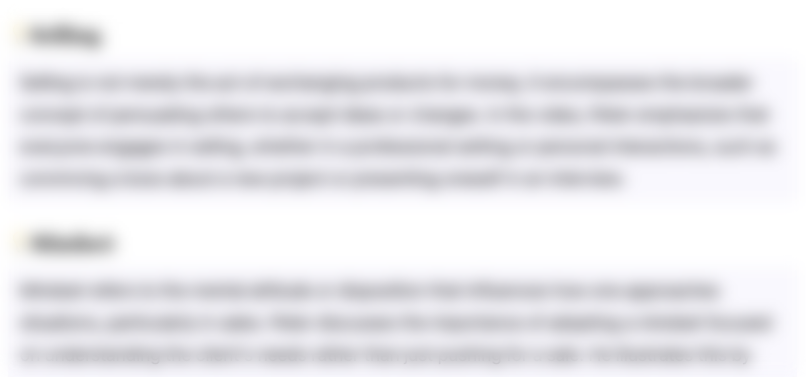
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
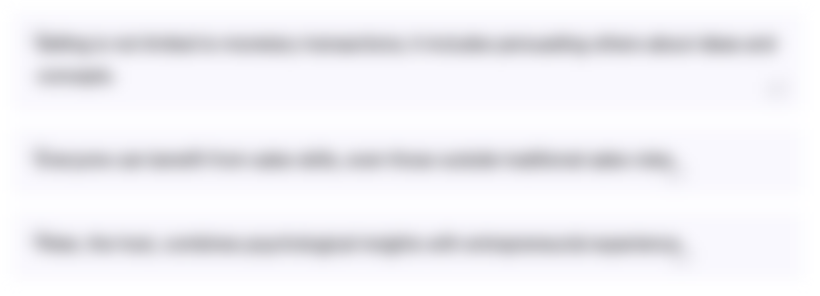
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
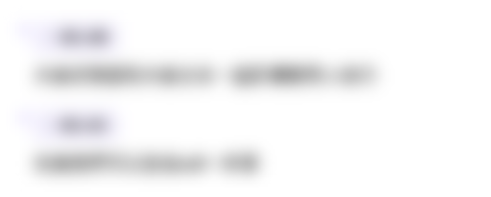
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

L17 Integration Testing
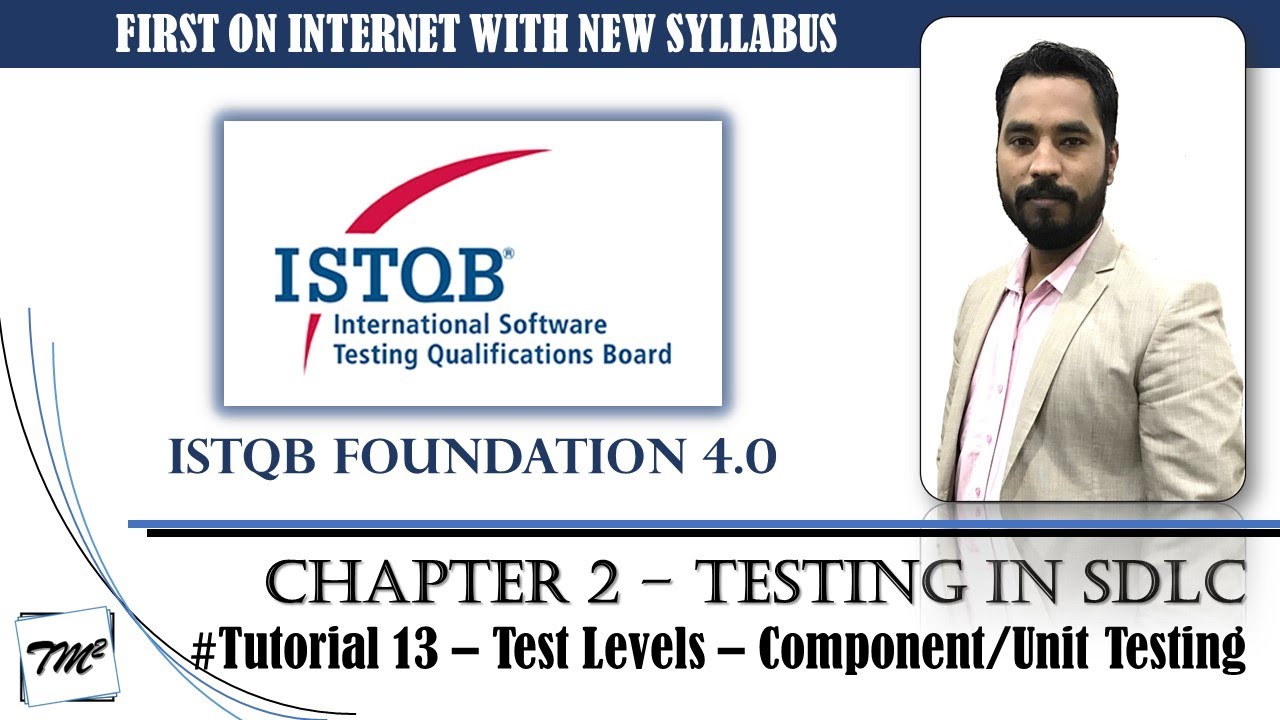
ISTQB FOUNDATION 4.0 | Tutorial 13 | 2.2.1 Test Levels & Test Types | Component Testing | CTFL

ISTQB FOUNDATION 4.0 | Tutorial 5 | 1.4 Test Activities, Testware & Test Roles (Part-1) | CTFL
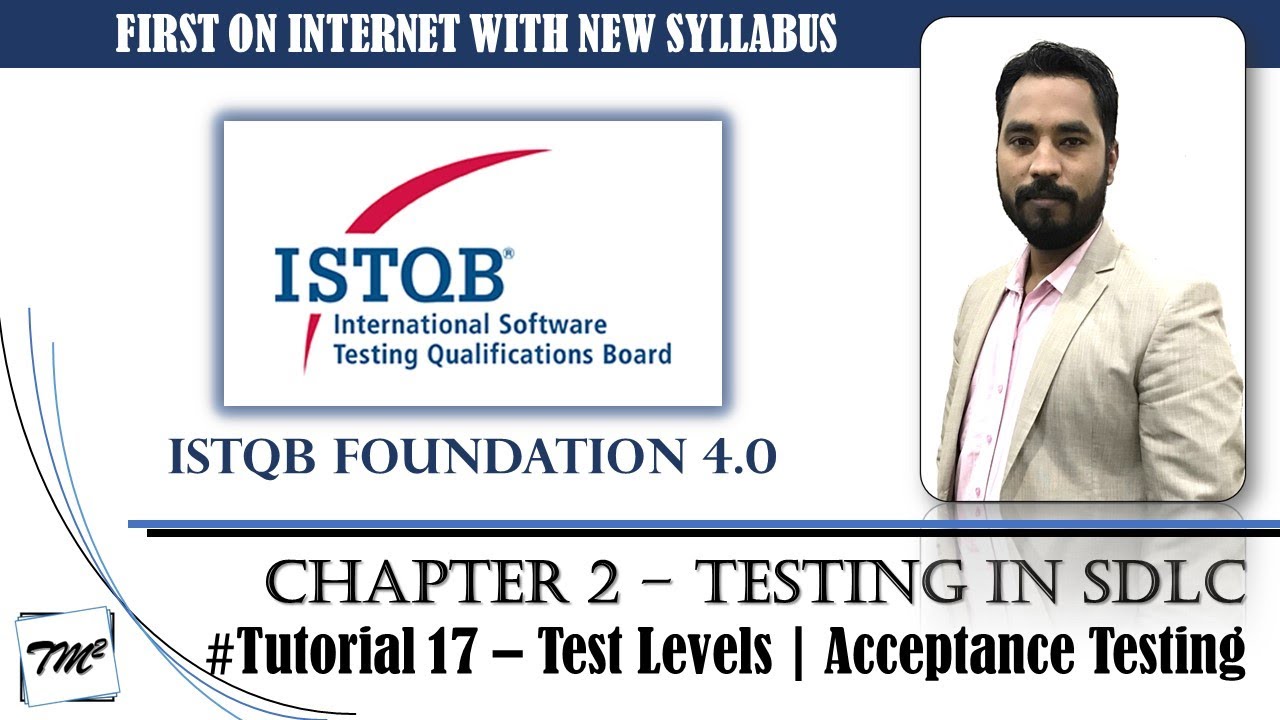
ISTQB FOUNDATION 4.0 | Tutorial 17 | Test Levels | Acceptance Testing | Alpha Testing | Beta Testing

CH04. L01. Test development process
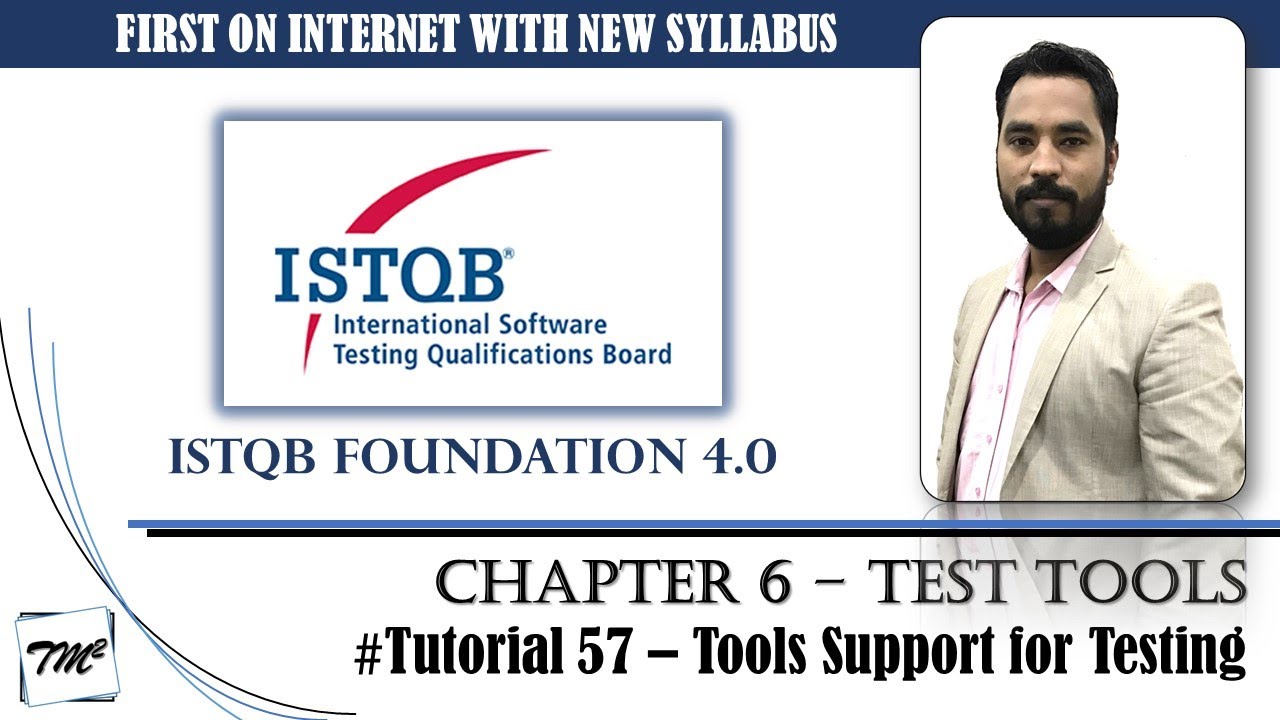
ISTQB FOUNDATION 4.0 | Tutorial 57 | Tool Support for Testing | Test Tools | ISTQB Tutorials
5.0 / 5 (0 votes)