Kotlin Flows in practice
Summary
TLDRThis script introduces the concept of reactive programming in Android development using Kotlin Flow. It explains how to model data streams, optimize for lifecycle events, and handle configuration changes. The talk uses the analogy of water infrastructure to illustrate the benefits of setting up data 'pipes' instead of manual data requests. It also covers creating, transforming, and collecting flows, as well as best practices for lifecycle-aware collection and testing strategies.
Takeaways
- π **Reactive Programming in Android**: The talk introduces reactive programming concepts to Android development, focusing on stream data modeling using Kotlin Flow.
- π **Optimizing Flows for UI Lifecycle**: Discusses how to optimize data flows for Android's unique UI lifecycle, including handling rotations and backgrounding the app.
- π§ **Flow as a Stream of Data**: Uses the analogy of water flow to explain the concept of data streams in Android, emphasizing the efficiency of 'observing' data rather than 'requesting' it.
- π§ **Building Infrastructure for Data**: Advocates for creating infrastructure (like pipes for water) in apps to manage data flow more effectively, reducing redundancy and potential errors.
- π **Unidirectional Data Flow**: Highlights the importance of keeping data flow in one direction to simplify management and reduce bugs in the application.
- π οΈ **Kotlin Flow for Data Management**: Recommends using Kotlin Flow, part of the Coroutines library, for sophisticated data combination and transformation within an app.
- π‘ **Creating and Transforming Flows**: Covers the creation of flows using flow builders and the transformation of data through intermediate operators like map and filter.
- π **Error Handling in Flows**: Introduces the catch operator for handling exceptions within a flow, ensuring robust data stream management.
- π **Collecting Flows in UI Layer**: Explains the process of collecting data from flows in the UI layer using terminal operators and the importance of doing so efficiently.
- π **Lifecycle Awareness in Flow Collection**: Discusses the use of lifecycle-aware APIs like repeatOnLifecycle and flowWithLifecycle to manage flow collection based on the UI's visibility.
- π **Testing Flows**: Provides insights into testing flows, emphasizing the need for strategies to handle the asynchronous nature of stream data.
Q & A
What is the main concept discussed in the script related to Android redevelopment?
-The main concept discussed is reactive programming, specifically using Kotlin Flow for modeling streams of data in Android redevelopment.
How does the script illustrate the idea of reactive programming in the context of fetching data?
-The script uses the analogy of Pancho fetching water from a lake, explaining that instead of repeatedly requesting data (walking to the lake), it's more efficient to set up an infrastructure (installing pipes) to observe data changes automatically.
What is the significance of keeping data flow in one direction in the script's explanation?
-Keeping data flow in one direction is highlighted as a best practice because it reduces the likelihood of errors and simplifies the management of data streams within an application.
What is the role of a producer in the context of Kotlin Flow as described in the script?
-A producer in Kotlin Flow is responsible for emitting data into the flow, which can then be collected by a consumer, typically a UI layer in an Android application.
How can intermediate operators be used to transform or filter data streams in Kotlin Flow?
-Intermediate operators like 'map' can transform data to a different type, while 'filter' can be used to select only certain items that meet specific criteria, such as containing important notifications.
What is the purpose of the 'catch' operator in handling errors within a flow in Kotlin?
-The 'catch' operator is used to handle exceptions that may occur while processing items in the upstream flow, allowing the application to either rethrow exceptions or emit alternative values.
Why is it recommended to use 'repeatOnLifecycle' or 'flowWithLifecycle' when collecting flows from the Android UI layer?
-These APIs are lifecycle-aware and help optimize the collection of flows by automatically managing the lifecycle of coroutines based on the UI's visibility, preventing unnecessary resource usage when the app is in the background.
What is the difference between 'repeatOnLifecycle' and 'flowWithLifecycle' in collecting flows?
-'repeatOnLifecycle' is a suspend function that automatically launches and cancels coroutines based on lifecycle states, suitable for collecting from multiple flows. 'flowWithLifecycle', on the other hand, is used when there is only one flow to collect, as it emits items and manages the lifecycle of the producer directly.
How does the script suggest handling configuration changes like device rotation in the context of data flows?
-The script recommends using StateFlow, which acts as a buffer holding data and can share it between multiple collectors, ensuring a smooth transition during configuration changes without restarting upstream flows.
What is the role of 'StateFlow' in the context of view models and activities with different lifecycles?
-StateFlow is used to safely expose flows from a view model to activities or fragments, ensuring that the data remains consistent and available even when the UI elements are recreated due to lifecycle changes.
How can testing of flows be approached as discussed in the script?
-Testing flows can involve replacing dependencies with fake producers to emit specific test data, or by using terminal operators like 'first' or 'take' to collect and verify the output of the flow under test.
Outlines
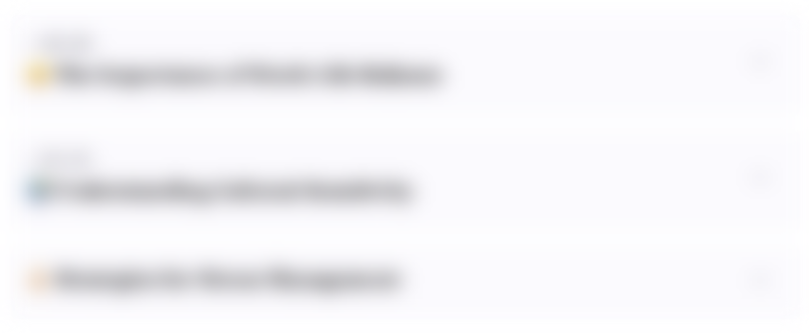
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
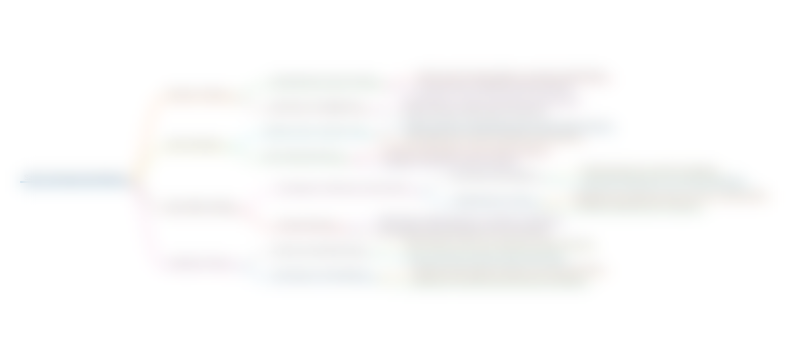
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
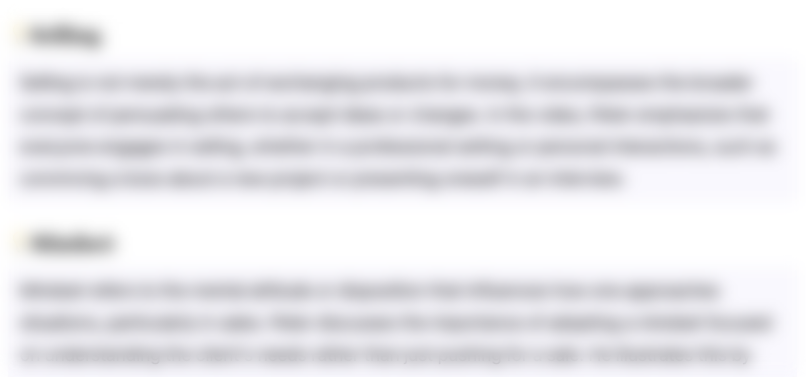
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
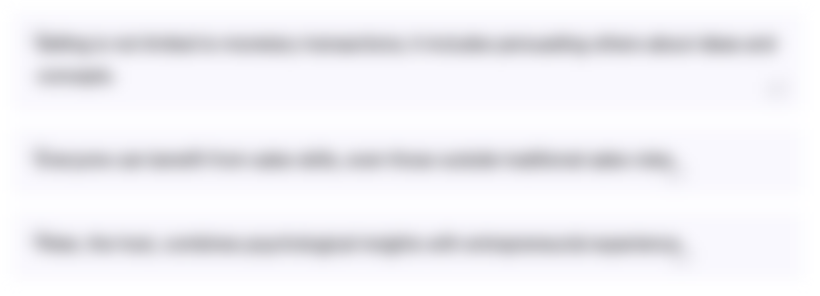
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
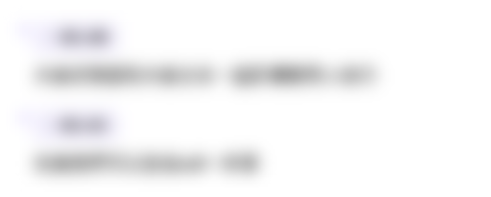
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)