Learn LUA, The Basics | Episode 1
Summary
TLDRThis episode of 'Learning Lua' introduces the basics of the Lua programming language, covering its history, usage in various applications like game development, and how to write simple scripts. Topics include printing messages, variables, functions, math equations, text inputs, and if statements. The tutorial concludes with a challenge to create a sales tax calculator.
Takeaways
- 📜 Lua was created in 1993 and is versatile, used in applications like Roblox, 5M Love, and more.
- 💻 Lua can be edited in various programs, including Roblox Studio, Visual Studio Code, Atom, and any text editor.
- 📝 Comments in Lua are made using `--` for single lines or `--[[]]` for multi-line comments.
- 🖨 The `print` function in Lua is used to output text or variables to the console, enclosed in quotation marks.
- 🔑 Variables in Lua are declared with `local` to limit their scope to the script, followed by the variable name and assignment.
- 📚 Lua functions are defined using `local function functionName() end` and can be executed multiple times as needed.
- 🔢 Basic math operations in Lua include addition, subtraction, multiplication, division, and exponentiation, stored in variables or printed directly.
- 📉 Lua supports different ways to perform and print math equations, with variables for reusability and direct print for simplicity.
- 📑 Text input in Lua is handled through the command prompt, using `io.read()` to capture user input and store it in a variable.
- 🔄 Lua's `if` statements allow conditional execution of code based on comparisons like equality (`==`), inequality (`~=`), less than (`<`), and greater than (`>`).
- 🛒 The tutorial concludes with a challenge to create a sales tax calculator, demonstrating Lua's practical application in simple calculations.
Q & A
What is the history of the Lua programming language?
-Lua was born in 1993 and has been used in various applications including game development, math equations, and more.
Can Lua be used in Roblox?
-Yes, Lua can be used in Roblox, and it is one of the primary languages supported by the platform.
What are some programs where you can edit Lua code?
-Lua code can be edited in many programs such as Roblox Studio, Visual Studio Code, Atom, or any text editor like Notepad++.
What is the basic syntax for a comment in Lua?
-In Lua, a comment is made with two dashes (--) followed by the comment text. For multi-line comments, use --[[ and ]].
How do you print a message in Lua?
-In Lua, you use the 'print' function followed by parentheses containing the message in quotation marks, for example, 'print("Hello, World")'.
What does the 'local' keyword do in Lua?
-The 'local' keyword in Lua is used to declare a variable that is local to the block of code where it is defined, not accessible globally.
How do you define a variable in Lua?
-In Lua, you define a variable with 'local' followed by the variable name and then the assignment operator '=', for example, 'local hi = "Hello!"'.
What is a function in Lua and how do you define one?
-A function in Lua is a block of code that can be executed multiple times. It is defined using 'local function functionName()' followed by the function's code block enclosed in 'then' and 'end'.
How can you perform simple math operations in Lua?
-In Lua, you can perform simple math operations by using variables and arithmetic operators like '+', '-', '*', '/', and '^' for addition, subtraction, multiplication, division, and exponentiation, respectively.
How do you handle text input in Lua?
-Text input in Lua is handled using the 'io.read' function, which reads a line from the standard input and returns it as a string that can be stored in a variable.
What is an 'if' statement and how is it used in Lua?
-An 'if' statement in Lua is used for conditional execution. It checks if a condition is true and executes the code block under 'then'. If the condition is false, it executes the code block under 'else', if present.
How can you create a sales tax calculator in Lua as suggested in the tutorial?
-To create a sales tax calculator in Lua, you would define variables for the price and tax rate, perform the calculation for the tax amount, and then print the original price, price with tax, and the tax amount.
Outlines
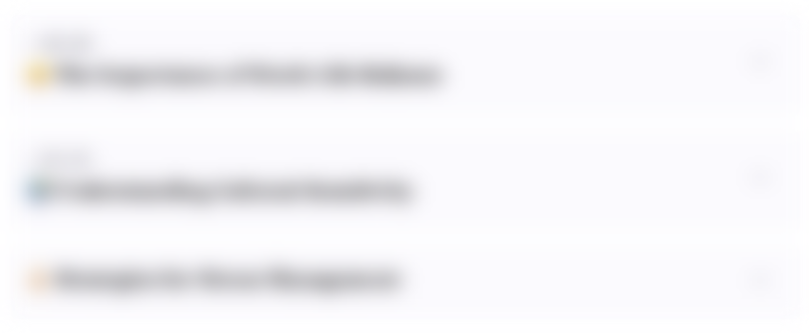
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
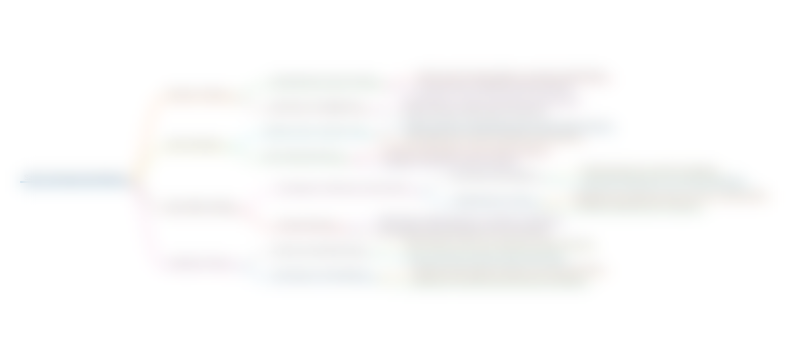
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
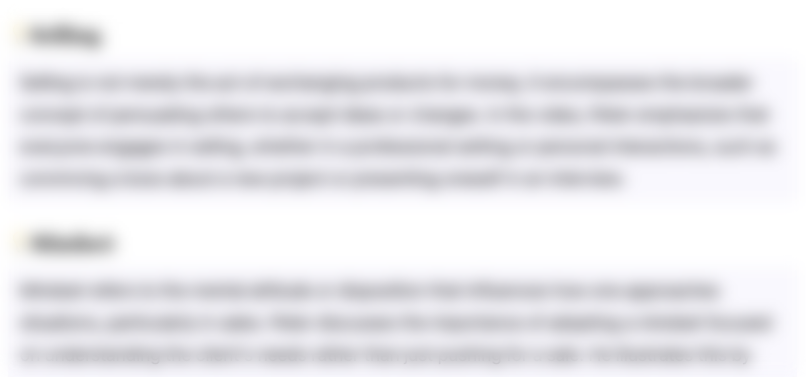
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
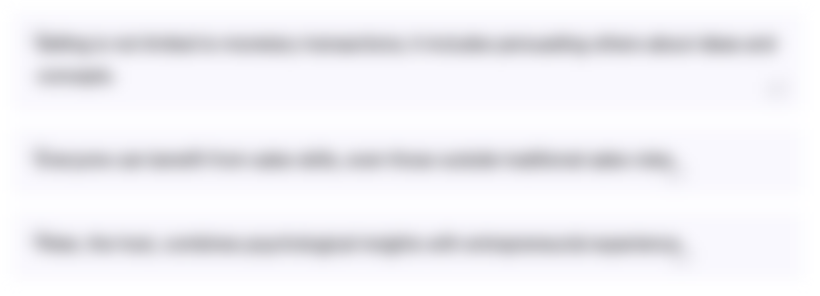
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
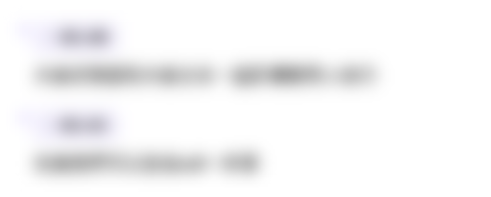
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
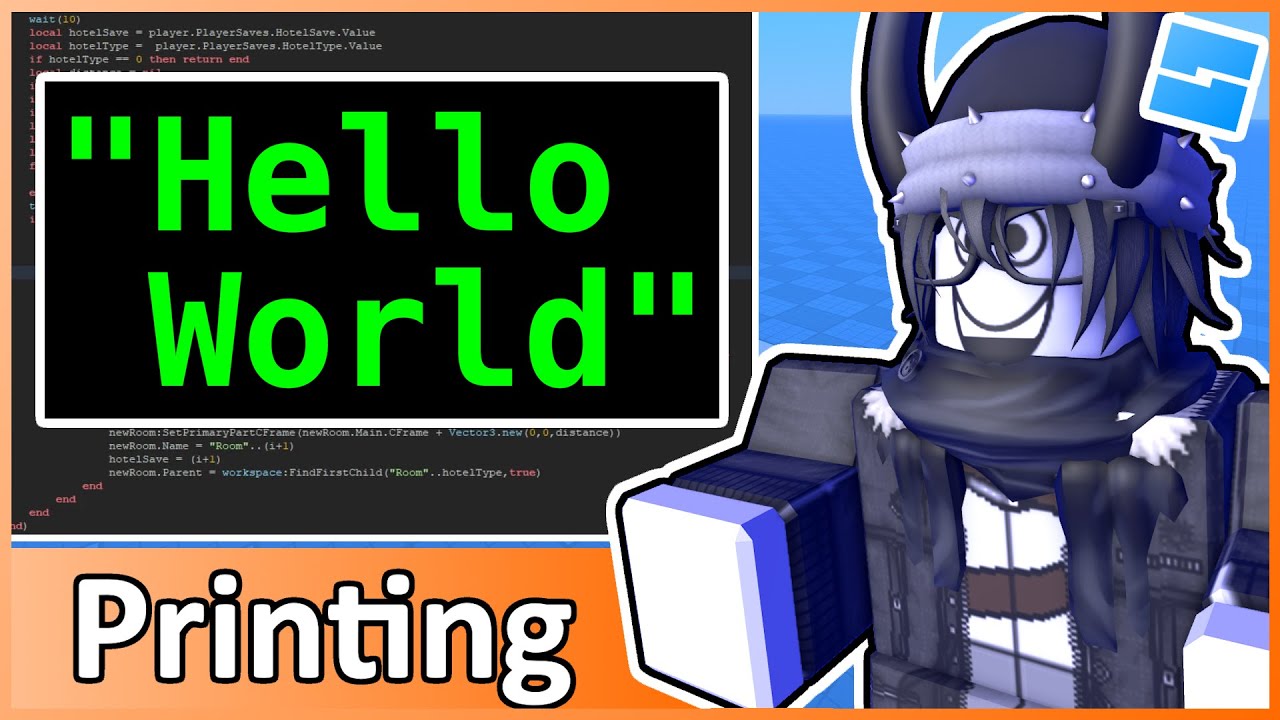
Printing - Roblox Beginners Scripting Tutorial #2 (2024)
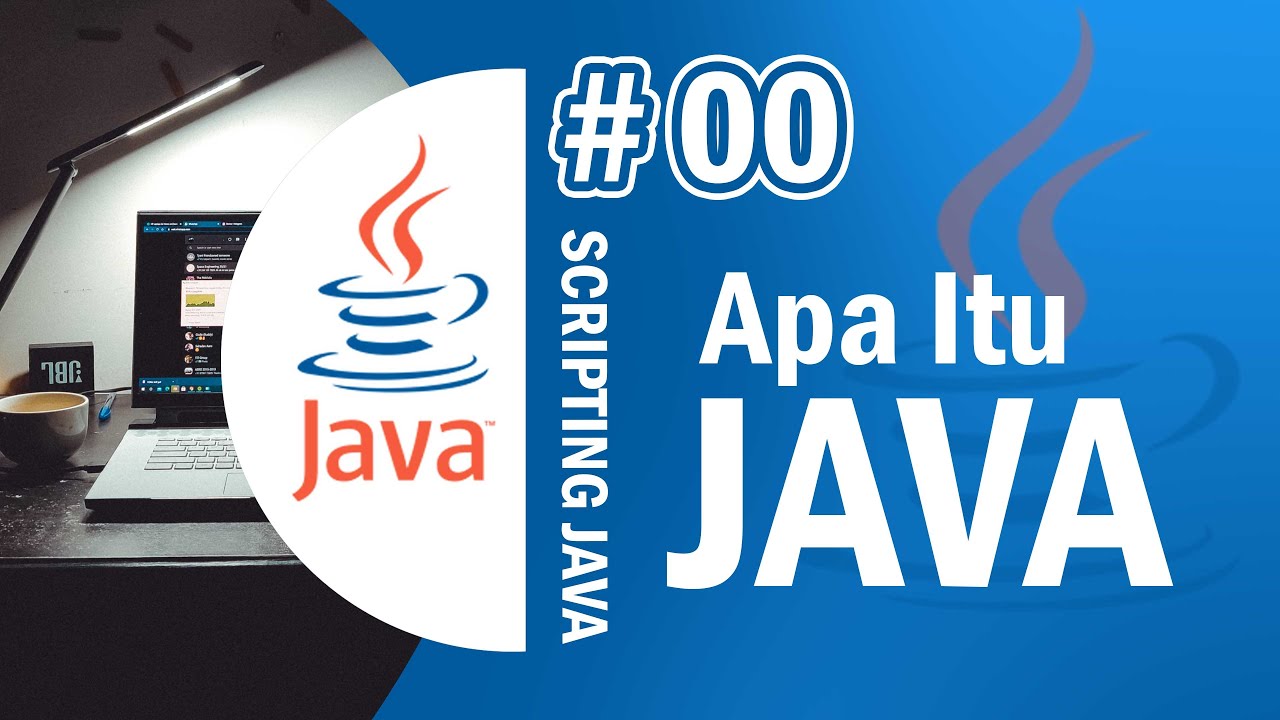
Java 00 - Mengenal Bahasa Pemrograman Java - Tutorial Java Netbeans Indonesia
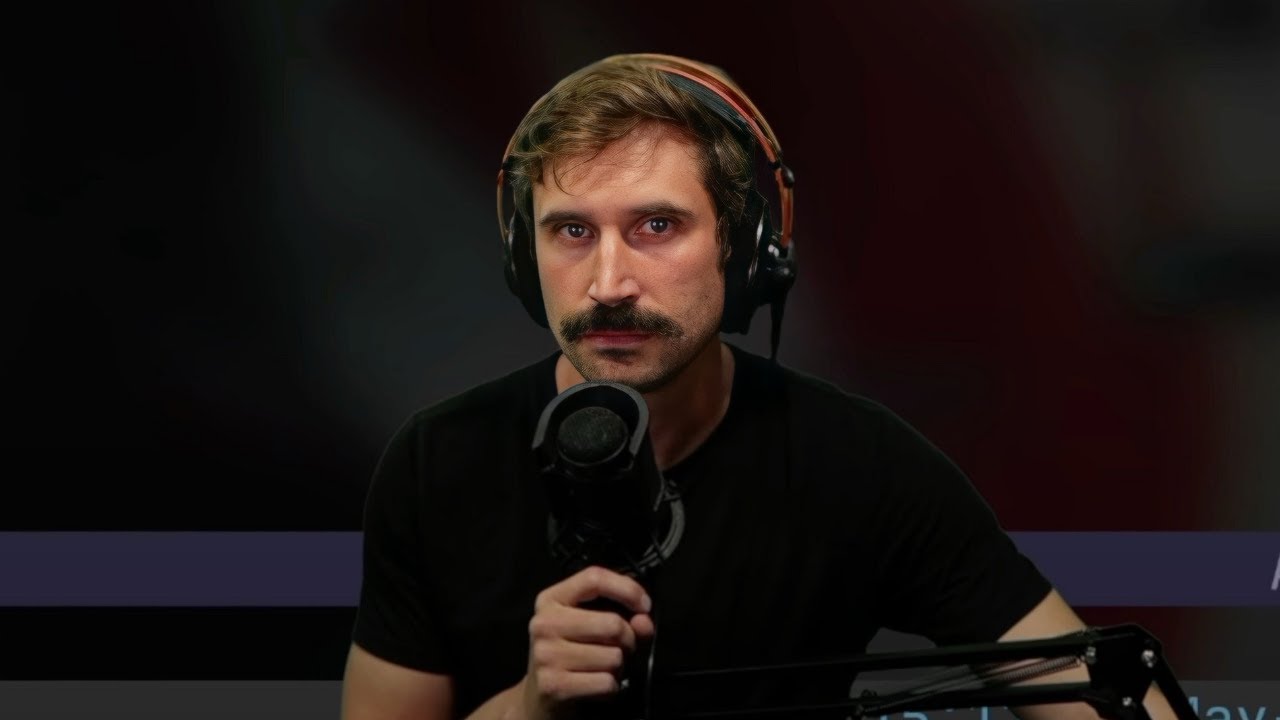
I'VE HAD ENOUGH!!!
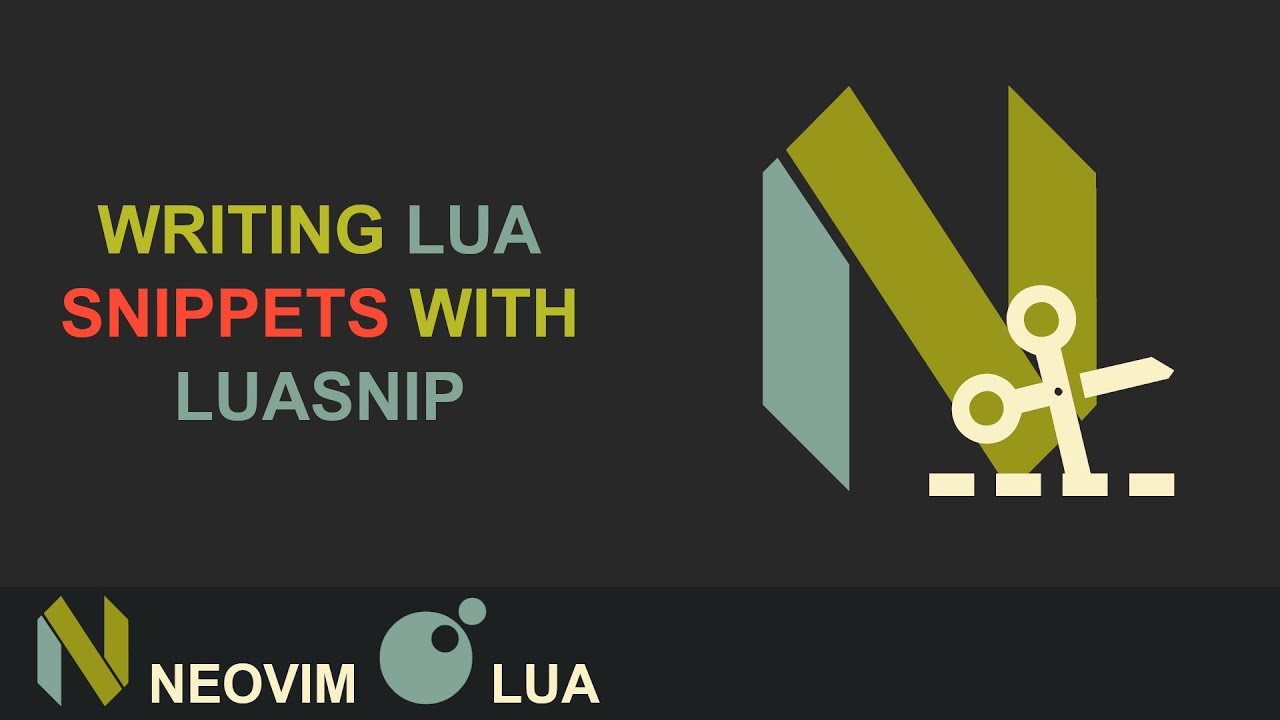
The Best Neovim Plugin | Writing Lua Snippets With Luasnip

MEMBUAT GAMES SEDERHANA DI SCRATCH 3.0
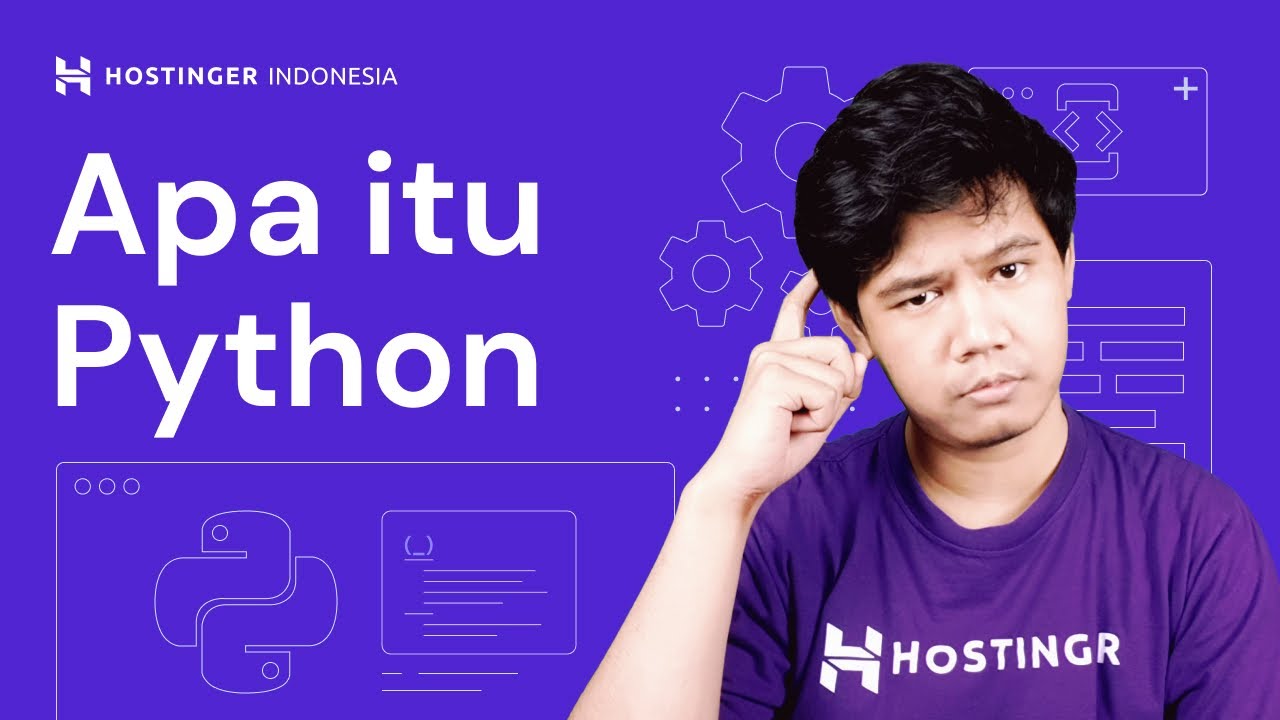
Apa itu Python? Penjelasan dan Kelebihan Beserta Fungsinya
5.0 / 5 (0 votes)