Movie Recommender System in Python with LLMs
Summary
TLDRThis video tutorial guides viewers through building a movie recommender system in Python using a local large language model and a vector store. It utilizes a Netflix dataset from Kaggle, transforming movie and TV show information into textual representations. These are then embedded into a 4,096-dimensional vector space by llama 2, allowing similar content to cluster together. The system stores vectors in a Facebook AI Similarity Search (FAISS) database for efficient similarity searches, providing movie recommendations based on user preferences or imagined scenarios.
Takeaways
- 🎬 The video is about building a movie recommender system using a large language model and a vector store in Python.
- 📈 The process involves using a dataset from Kaggle, specifically a Netflix dataset containing information about TV shows and movies.
- 🔍 Features of the dataset include the title, description, cast, release year, genre, and other details of movies and TV shows.
- 📝 The script describes creating a textual representation of each movie or TV show by combining its features into a single string.
- 🧠 A large language model, in this case, llama 2, is used to embed these textual representations into a high-dimensional vector space.
- 📊 The expectation is that similar movies will be closer in vector space, relying on the intelligence of the language model to determine similarity.
- 🛠 The embeddings are stored in a vector store called FAISS (Facebook AI Similarity Search), provided by Facebook.
- 🔄 The vector store can then be used to find the top recommendations for a given movie by comparing the movie's vector to others in the store.
- 🔧 The video includes a step-by-step guide on installing the necessary Python packages and the llama 2 model, as well as coding the recommender system.
- 🔍 The script also covers how to perform a similarity search using the vector store to recommend movies similar to a given movie.
- 💡 The video concludes by demonstrating the recommender system with examples, including creating a hypothetical movie and getting recommendations based on it.
Q & A
What is the main topic of the video?
-The main topic of the video is building a movie recommender system using a large language model and a vector store in Python.
Which dataset is used in the video for building the recommender system?
-The video uses a dataset from Kaggle, specifically the Netflix dataset containing information about TV shows and movies.
What features does the Netflix dataset contain?
-The Netflix dataset contains features such as the title, description, cast, release year, genre, and other details of movies and TV shows.
How does the large language model contribute to the recommender system?
-The large language model contributes by taking the textual representation of a movie and embedding it into a high-dimensional vector space, allowing for the identification of similar movies based on their vector proximity.
What is the name of the vector store used in the video?
-The vector store used in the video is called FAISS, which stands for Facebook AI Similarity Search.
What is the dimension of the vectors produced by the large language model in this video?
-The large language model, in this case, llama 2, produces vectors with a dimension of 4096.
How does the recommender system determine which movies are similar?
-The recommender system determines similarity by comparing the vector representations of movies in the vector space, where closer vectors indicate more similar movies.
What Python packages are needed to implement the recommender system as described in the video?
-The Python packages needed are numpy, pandas, FAISS, and requests.
How does the video demonstrate the process of creating a textual representation of a movie?
-The video demonstrates this by creating a function that formats a multi-line string containing various attributes of a movie from a data frame, such as type, title, director, cast, release year, genre, and description.
What is the purpose of the 'create_textual_representation' function in the script?
-The 'create_textual_representation' function is used to generate a textual representation of each movie from the data frame, which includes details like type, title, director, cast, release year, genre, and description.
How can the recommender system be tested with a new or hypothetical movie?
-The recommender system can be tested by creating a textual representation of a new or hypothetical movie, embedding it using the large language model, and then performing a similarity search to find the most similar existing movies in the vector store.
What is the significance of the embedding process in the context of the recommender system?
-The embedding process is significant as it translates the textual information of movies into a numerical format that can be compared and analyzed within the vector space, which is essential for the similarity search and recommendation functionality.
How does the video handle the installation and usage of the large language model llama 2?
-The video instructs viewers to install llama 2 by downloading it from the official website, using the command line to pull the model, and then utilizing it locally for generating embeddings from textual representations.
What is the final output of the recommender system when a user queries for movie recommendations?
-The final output of the recommender system is a list of the top five most similar movies to the user's query, which could be based on an existing movie from the dataset or a hypothetical movie created by the user.
Outlines
🎬 Building a Movie Recommender System
The video script introduces a project to create a movie recommender system using a large language model and a vector store in Python. The process involves using a dataset from Kaggle, specifically a Netflix dataset with features like movie titles, descriptions, cast, release year, and genres. The aim is to convert this data into a textual representation, which will then be embedded into a high-dimensional vector space by the language model llama 2. The expectation is that similar movies will be positioned closer in this vector space, facilitating a recommendation system based on vector similarity.
📝 Preparing the Data and Environment
The script details the preliminary steps for the project, which include installing the necessary Python packages like numpy, pandas, faiss (a vector store by Facebook), and requests. It also involves downloading the Netflix dataset from Kaggle and preparing the data by creating a textual representation for each movie or TV show. This textual data will be used to generate vectors using the llama 2 model, which will then be stored in the vector database for later use in the recommendation process.
🔢 Embedding Text into Vector Space
The script explains the process of sending textual representations of movies to the llama 2 model to generate embeddings. It outlines the technical steps of using the requests library to send POST requests to the local llama 2 server, receiving embeddings in response, and storing these vectors in a numpy array. The embeddings are then added to the faiss index, which serves as the vector store. The process includes checking progress and handling the data to ensure it's in the correct format for the vector store.
🔍 Performing Similarity Searches for Recommendations
The script demonstrates how to use the vector store to perform similarity searches for movie recommendations. It involves loading the vector index from a file, crafting a textual representation of a movie (either from the dataset or a hypothetical one), and using the llama 2 model to embed this representation into the vector space. The index is then queried to find the top matches based on vector similarity, which are returned as indices. These indices are used to retrieve the corresponding movies from the dataset to provide recommendations.
🎉 Conclusion and Next Steps
The final paragraph wraps up the video by summarizing the process of building the movie recommender system and encouraging viewers to experiment with different textual representations to improve the recommendation quality. The script also invites viewers to like, comment, and subscribe for more content, and hints at the possibility of using different models or parameters for the llama 2 model to enhance the system's performance.
Mindmap
Keywords
💡Movie Recommender System
💡Large Language Model
💡Vector Space
💡Netflix Dataset
💡Textual Representation
💡Embedding
💡FAISS (Facebook AI Similarity Search)
💡Similarity Search
💡Kaggle
💡Python Packages
Highlights
Building a movie recommender system using a large language model and a vector store in Python.
Utilizing a local large language model to power the recommendation process.
Using a dataset from Kaggle containing Netflix information about TV shows and movies.
Features in the dataset include title, description, cast, release year, and genre.
Creating a textual representation of each movie or TV show from the dataset.
Embedding the textual representation into a high-dimensional vector space using LLaMA 2.
The expectation that similar movies will be closer in vector space.
Storing the movie vectors in a vector store called FAISS by Facebook.
Performing a similarity search to recommend movies based on a given movie's vector.
Installing LLaMA locally and using it to embed movie descriptions without the need for additional coding.
Downloading the Netflix dataset and installing necessary Python packages for the project.
Crafting a function to create a textual representation for each movie from the dataset.
Using the embeddings to populate a vector store for similarity searches.
Demonstrating the process of embedding a movie and searching for similar movies.
The ability to recommend movies based on a newly created imaginary movie's description.
The practical application of the recommender system in providing movie suggestions.
The potential for customization and experimentation with different textual representations.
The conclusion summarizing the process and the capabilities of the built movie recommender system.
Transcripts
what is going on guys welcome back in
this video today we're going to build a
movie recommender system using a large
language model and a vector store in
Python so let us get right into it not
[Music]
AED all right so we're going to build a
movie recommender system in Python today
which is going to be powered by a large
language model which runs locally on our
machine now I'm going to give you a
brief sketch of the process so that you
understand how the reom Commendation
process works and you don't have to just
copy paste the code without
understanding what it does so it's not
going to be the most beautiful sketch
here but I'm going to try to explain it
as simply as possible what we're going
to do is we're going to work with a data
set from kaggle and this is going to be
a Netflix data set containing
information about TV shows and movies
and it has features like title of uh the
movie or show and description and cast
and release year and genre and so on so
each row represents a movie or a TV show
and has features like the type is it a
movie or TV show uh stuff like the title
and the description and the release here
and the director and the cast and so on
so we have a couple of features here per
row and what we want to do now is we
want to take these and turn them into a
textural representation now this is
quite simple we don't have to do
anything else but just create a string
that says something like type is
whatever for example movie then title is
whatever the title of the movie is and
so on and all of this is going to be one
uh one large string containing all the
information about that movie now what
our large language model will do with
that string is it will take this string
and it will embed it into Vector space
to keep it simple this basically means
that the large language model does
something with this content with this
string and turns it into a vector into a
high dimensional vector to be precise
we're going to use llama 2 in this
video uh locally here and llama 2 will
produce a vector for each row with the
size
4,096 so we're going to have 4,096
different values here numbers that will
position this Vector in the vector space
in the 4,096 dimensional Vector space
this is what we're going to do here so
every movie will end up being some point
in this Vector space some Vector in this
vector space now the idea is that
similar movies or movies that are
somehow uh yeah similar to one another
will end up closer in the vector space
than movies that are very different now
how does it happen that's the
intelligent of that's the intelligence
of Lama 2 so we don't have to do any
fancy coding here we don't have to
implement some intelligent algorithm we
don't have to train a machine learning
model we expect llama 2 to have the
intelligence this is already inside of
the model the intelligence needed to do
that to take movies that are somewhat
similar and to put them together closer
together in the vector space than movies
that are completely different that's the
basic idea and then what we do is we
store all of these vectors into a vector
store so a vector database we're going
to use face which is a uh Facebook I
don't know what the acronym is but it's
a vector store by Facebook and this
Vector store will contain all the
different vectors and what we can do
then with this Vector store is we get
some new movie with the string title uh
and type and director and so on as a
string we feed this into the index into
our Vector store and as a result we get
for example the top five most similar
movies that could be interesting to you
if you like this one so you get a list
of five movies that could be interesting
to you that is what we're going to build
in this video today using llama 2
running on our system locally if you can
do that otherwise you will probably have
to um to host it somewhere or you have
to use the API of something else you can
also use chat GPT but you have to pay
for it or actually just GPT not chat GPT
but if you have uh the resources you can
just run this locally using olama so
this is what we're going to build in
this video today now how are we going to
do that we're going to start by first of
all installing olama now olama is quite
easy to install you just go to the
website olama com you download it for
your op operating system on Linux you
just run this command and then you can
use it on your system if you have
troubles with that I have a video on
this channel about olama where you can
look uh where you can see how I install
it and and how it works basically but
it's really not complicated what we're
going to do then is once you have ol
Lama installed you're going to type into
your terminal ol Lama pull and then the
model that you want to use I as I said
I'm going to use llama 2 you can also
provide another uh model name I think on
uh their website you should be able to
see the models that they offer you have
llama 3 you have mistol you have llama 2
with the different parameters also as
well if you want to have a a larger
model but you can do that uh and install
the model that you want to use all right
so once you have that done we're going
to also download a data set from kaggle
the Netflix movies and TV shows data set
you will find a link in the description
down below just download the Netflix
title CSV v file and then we can get
started with the coding now actually
before we get started with the coding we
also need to install the python packages
that we're going to use in this video
and for this we're going to open up a
command line and install numpy pandas
face and requests these are the four
packages that we're going to need in
this video today numpy and pandas
obviously because we're going to work
with data face because that is our
Vector store and requests because we
need to send requests to olama uh now
now actually we cannot say face I think
we need to say face CPU or face GPU so
depending on whether you have a GPU that
you can use here run face GPU or face
CPU if you want to use your processor
for this just install the packages and
once you have them we can get
started all right so the first thing is
we're going to obviously import pandas
aspd and we're going to then say the
data frame is going to be PD read
CSV Netflix title
CSV and then we can look at it and you
can see we have let me just close this
here uh we have a couple of features the
IDE of the show we have the type of the
show movie or uh TV show we have the
title we have the director the cast the
country that it was produced in I guess
uh the date it was added to Netflix the
release year also some rating uh the
duration and also the genres here so
what kind of movie is it and the most
important thing I assume is going to be
the description because it tells us
briefly what the movie is about and I
think that's the most important thing uh
maybe together with the documentaries
and with a cast that is going to be
relevant for uh a similarity search all
right so we're going to keep it simple
here all we're going to do is we're
going to craft for each line a string
that represents this or the individual
movies uh textually so we're going to
create a function down here we're going
to call it def uh we're going to call it
create textual representation given a
row from the data frame and what we're
going to do is we're going to say
textual representation is going to be
equal to a multi-line
string like this um and the important
thing is to not have any tabs here and
we're going to say something like type
and type is going to be just
so uh actually we need to make this a
formatted multi-line string like
this the type is going to be the type
then we're going to say that the title
is going to
be the title and actually we can then
just go and copy paste this I think and
we can say we want to have the director
we want to have the cast and we want to
have uh the release year and genre
and the most important thing also in the
end the
description so this is going to be
description this is going to
be actually we need to turn this into
row
title and into row
director and into
row cast
and into
row release I think release year
right and
into row and I think it
was what was it called listed in is what
we're looking for listed in and then
finally of course row
description so that should work and this
function now applied to the rows and
we're going to do it like this here this
function applied of course we need to
return the textual
[Music]
representation this function now applied
to the individual roles will give us
textual
representation uh representations for
the individual R so I can say DF
apply and then I can just apply the
create textual representation function
axis equals 1 and I will get for every
single um row here I will get
the string so I can say actually that
this is my textual
representation
column then you can see I have it and
then I can just get the
[Music]
column and show the different values so
if I print the first one we're going to
see that this is what what this looks
like in the end so this is just our
basic representation now which we're
going to use to ask llama 2 to turn this
into a vector into Vector space or in
the vector space so what we're going to
do next is we're going to say
import Face our Vector store import
requests so that we can send requests to
llama 2 or to O
Lama and import numpy SNP we're going to
need this here to create uh to create
the array for our uh Vector store we're
going to define the dimension of our
output as I set to be 496 because that
is what is going to be returned by llama
2 uh for the embedding
Dimensions we're going to say index is
equal to face
index flat
L2 with a dimension here as a parameter
this is basically our database you could
say our Vector store that we're creating
and then we're going to initialize an X
full of
zeros and the dimensions here are going
to be the length of
the textural representations so how many
instances do we have how many movies or
shows do we have and how large is the
dimension for each of them so we're
going to have n vectors here this amount
of vectors uh off size
dimension and the data type is going to
be float
32 all right so that is now an array
full of zeros as you can see and this is
going to be filled up with the
embeddings from llama 2 so we're going
to say now 4 I uh actually 4 I Row in or
actually for I
representation in
enumerate data frame textual
[Music]
representation what we want to do is
want to say if I is
200s or not 200 if I is divisible by 200
so every 200 uh row I want to print the
progress so I know how long it takes I'm
not going to run all of this on camera
because it's going to take quite some
time but you can run this here to see
the progress just
say uh something like
processed and then I and
then processed I Str I uh instances for
example and uh then we're going to say
that I want to send a request which is
going to return a response so rest is
equal to request I want to send a post
request to ol Lama and ol Lama by
default unless you change that is
running at
HTTP and then Local Host
Port um
11434 so 11434 is the port that ama is
running at and we want to use the
embeddings API so/ API SL
embeddings and what we want to send to
this API is a Json object and this Json
object will will contain the model that
we want to use so the model is going to
be llama 2 and also the prompt that we
want to use uh or the prompt that should
be embedded so the prompt is going to
be equal to the
representation that we're currently
at there you go and this needs to be
closed like this actually this should be
indented uh yeah let's let's just leave
it like this and this is going to return
a response and this response will have a
field embedding so we want to say
embedding is equal to the response get
me the Json object from the response and
what I'm interested in is the field
embedding this
embedding is the vector so all we have
to do now is we have to go to the I
position this is why we enumerate this
uh we going want to go to the I position
in our zero vector and we want to
replace the zeros with this new
embedding that we got from the large
language model so NP
array
embedding and then finally in the end we
can add all of x to our Vector store now
I will turn this number down to let's
say 30 so that you can see how fast this
works we have remember uh 8, 87 rows to
be processed and if I run this you can
see that it isn't
that fast uh actually now I need to
check that this is equal to
zero and then I can run this again
process zero
instances then it takes some time
process 30 instances and so on I think
my video is lagging I'm pretty sure my
video was lagging while this was running
because it was running on the GPU so I'm
not going to run this here on camera
what I have actually done is I have
trained this already and I will show you
how you can save this when you've
trained it already so you run this you
wait for it to finish and once it's
finished what you do is you say face
right
index and then you just take the index
so this object here that you created and
you write it to a file for example let's
call it index as well once you do that
you're going to create a file index that
contains the index so you only have to
run this once then it's done and then
you can use it because you now have a
vector database full of embeddings for
the different movies um
and in order to load it then which is
what I'm going to do now in order to
load it again you just need to go and
say um you just need to go and say index
is equal to pH read index and then the
file for for example index and this is
what I'm going to do here now so now I
just copy pasted the exported Vector
store the index file from my prepared
code and all I have to do now is I have
to open up a new cell and say that the
index is equal to phase re Del index and
then I just have to provide index and
then I can go ahead and use this index
to do a similarity search so how exactly
can I now recommend a movie based on
another movie now first of all I can
craft my own textural representation of
whatever movie I like I don't have to
use a movie that's already part of the
data frame but let's start with a movie
that's part of the data frame so what I
can do for example is I can say one of
my favorite movies is Shutter Island I
want to see if that's part of the data
frame so let's go and say DF where DF
title string contains and let's see if
we have Shutter Island in
here and in this case we have the movie
Shutter and we have the movie Shutter
Island so let's copy this ID and let's
say that what I want to use now here as
a base uh for my recommendation is my
favorite movie is actually DF iock and
then this so we can see that my favor
movie is shudder Island here and now I
can just go ahead and I can get the or
actually let's go and uh write the code
directly what we need to do now is we
need to send this movie to O Lama to be
embedded now we're going to pretend that
this movie is not already embedded so
we're not going to use it directly we're
going to actually embed it again because
this is now our new movie that we passed
to the uh to the recommender system here
and we want it to be embedded into the
vector space and then it should find the
most similar movie so the closest
embeddings to the embeddings uh or to
the embedding of this particular movie
so what we do is we say response equals
requests. poost and we post to the same
URL so Local
Host uh Port
11434 SL API
embeddings and then we want to say that
the
Json is going to be equal to a
dictionary and the content here is going
to be model
again llama 2 and the prompt in this
case is going to
[Music]
be our favorite
movie and from it the textual
representation like
this that is going to be our uh
requested we sent here and this is going
to result in a embedding so in an
embedding so we're going to get the
embedding from this response again get
the Json object get the
embedding and uh actually we need to do
some additional processing so we're
going to say here uh we're going to turn
this into a numpy array that's just some
formatting stuff here so we're going to
say it's a numpy rate off a list of the
embedding with a data
type float
32 and this is now what we're going to
use as a basis for the similarity search
so we're going to search our index for
similar embeddings to this one so we're
going to say d and I is equal to index
search given the embedding give me the
top five matches so I can say five here
and this will result in the top five
matches now what I'm going to get here
as a response is of course not the
actual title not the actual data not the
textual representation I will get the
indices I will get the actual um we can
look at it um I will get the numbers I
will not get any
specific uh movies so what I have to do
is I have to take the data frame and get
these particular movies so what I can do
is I can say the best matches are going
to be equal to NP
array and then data frame textual
[Music]
representation um and from this we're
going to go and get I flatten get all
these indices flatten them and use them
as an index here and then I can just say
for match in best
matches uh I want to print next
movie then I want to print the match so
the movie representation and then just
an empty line and this is going to give
me the top five recommendations now
obviously the most similar
um match here to my prompt to my
embedding is the embedding itself
because Shutter Island was already part
of the vector store so of course the
closest match is shter Island itself the
second closest one is this one Devil's
gate uh seeking a missing woman in North
Dakota an FBI agent and a sheriff focus
on her religious zot husband but
discovers something far more Sinister
sounds like something mysterious and
something slightly scary which is also a
little bit like shutter Island I've not
watched this movie but I think that
could be a good match at least from the
description it sounds like that um and
then you can also see the other matches
here now the interesting thing is I
don't have to provide actually an
existing uh movie I can also make up a
movie I can maybe say I can imagine a
movie so let's go and copy
this and let's turn this into a
string and let's just make up something
so let's say my title my movie title is
the
mysterious python the director
is who did chter Island let's use the
same
director and then let's say the cast is
Leonardo
decapo and then maybe I don't know
uh I don't know let let's go with
someone else like uh
Sylvester is it written like this alone
I hope so I hope this is not too
embarrassing I'm not a movie guy so I
don't know uh let's just say these are
the two people and then it's released
2020 and it's
mystery drama thriller or something like
this and then we can say that the
description
is uh a group of
adventurers
discover a mysterious programming snake
in the jungle
and uh I don't
know and find something extremely
shocking yeah let's say this is my movie
description now I can take this and I
can actually save this now as
representation and then I can use
that as an input here
representation then I get the embeddings
and the best matches are the following a
patch of ful this is when a guard
catches a rider television host
shoplifting instead of turning him in he
only asks to be a friend then begins to
rule his life okay I don't know why that
is super similar to what I
said uh but yeah I mean you can play
around with that and see how good the
recommendations are maybe it's also
reasonable to only include the
description destion or to only include
genres in description something like
this maybe the other things are too um
too confusing but that's the basic idea
this is how you can build a recommender
system based on any data set you just
pick a textual representation you uh or
you create a textual representation you
embed these representations and then you
just perform a similarity search and you
hope that the embeddings are somewhat
intelligent based on the large language
model that you use to create them so
that's it for today's video I hope you
enjoyed it and I hope you learned
something if so let me know by hitting a
like button and leaving a comment in the
comment section down below and of course
don't forget to subscribe to this
Channel and hit the notification Bell to
not miss a single future video for free
other than that thank you much for
watching see you in the next video and
bye
Browse More Related Video
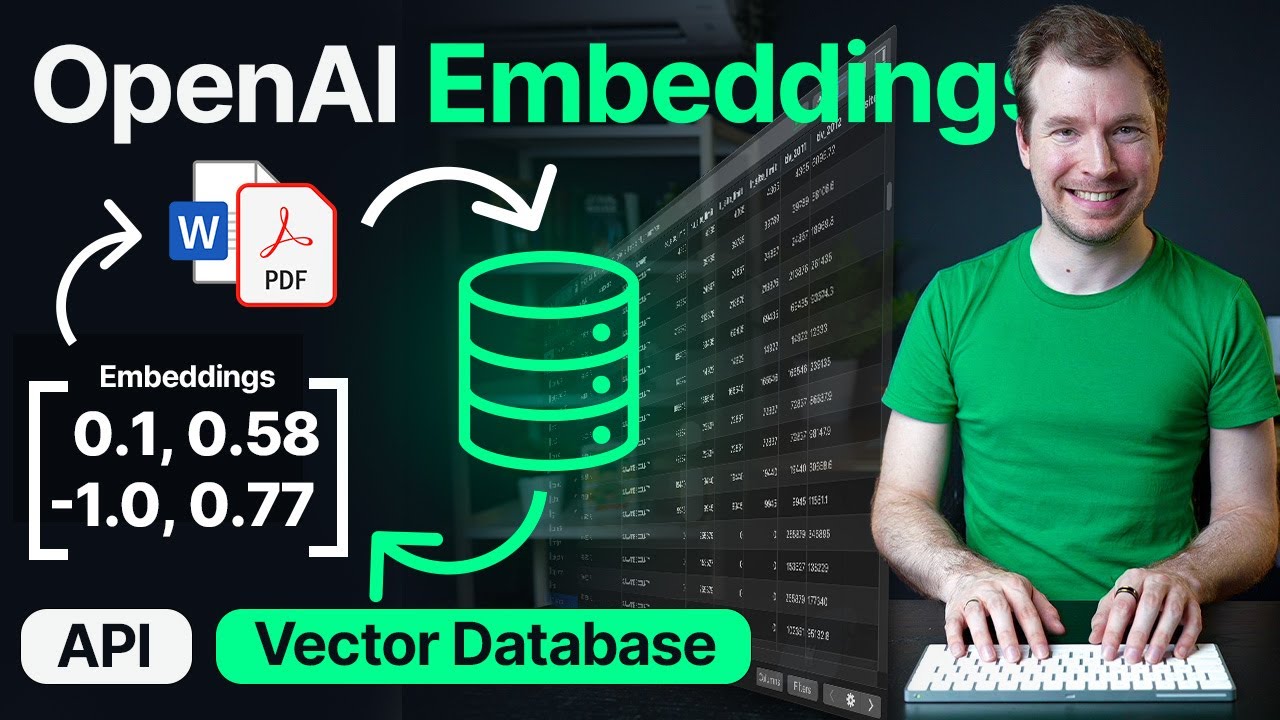
OpenAI Embeddings and Vector Databases Crash Course
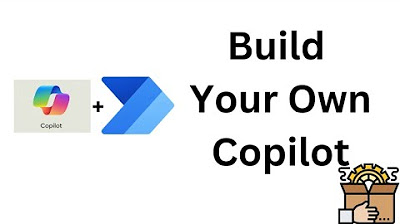
Microsoft Copilot Studio: How to Build your Copilot | Microsoft Copilot | Build your first Copilot

RAG from scratch: Part 10 (Routing)

Machine Learning Tutorial Python - 15: Naive Bayes Classifier Algorithm Part 2
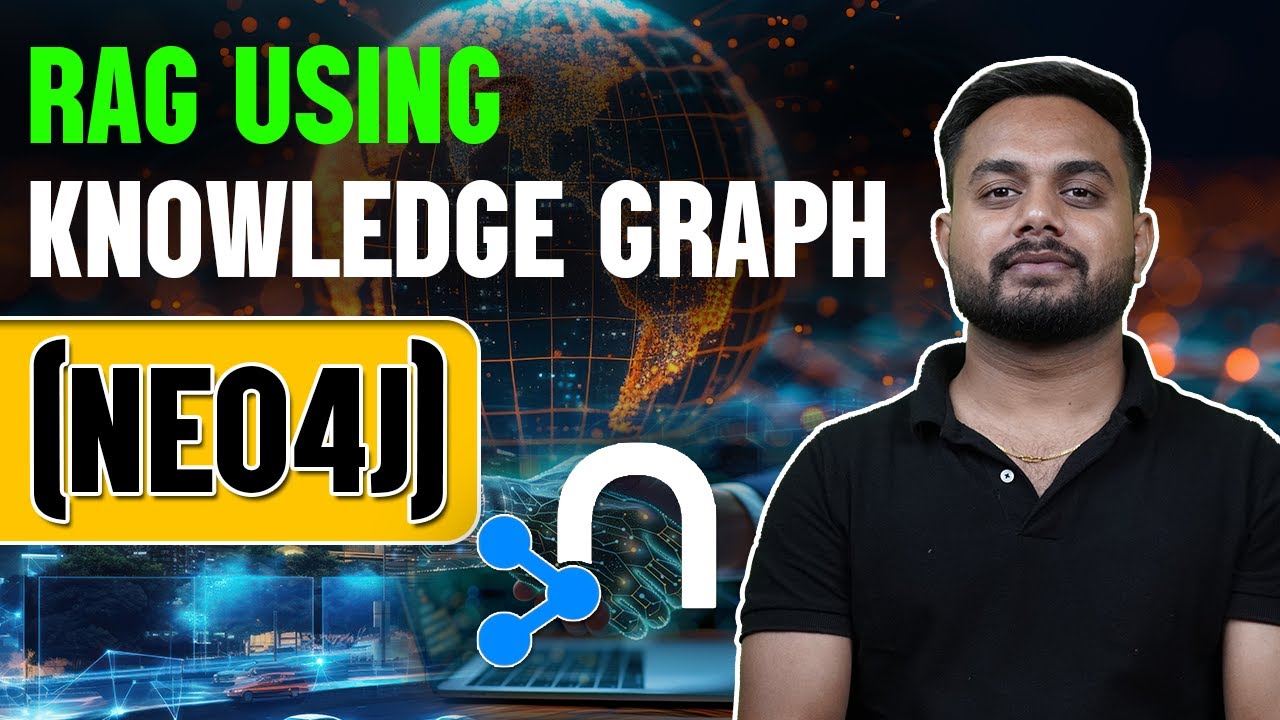
Realtime Powerful RAG Pipeline using Neo4j(Knowledge Graph Db) and Langchain #rag
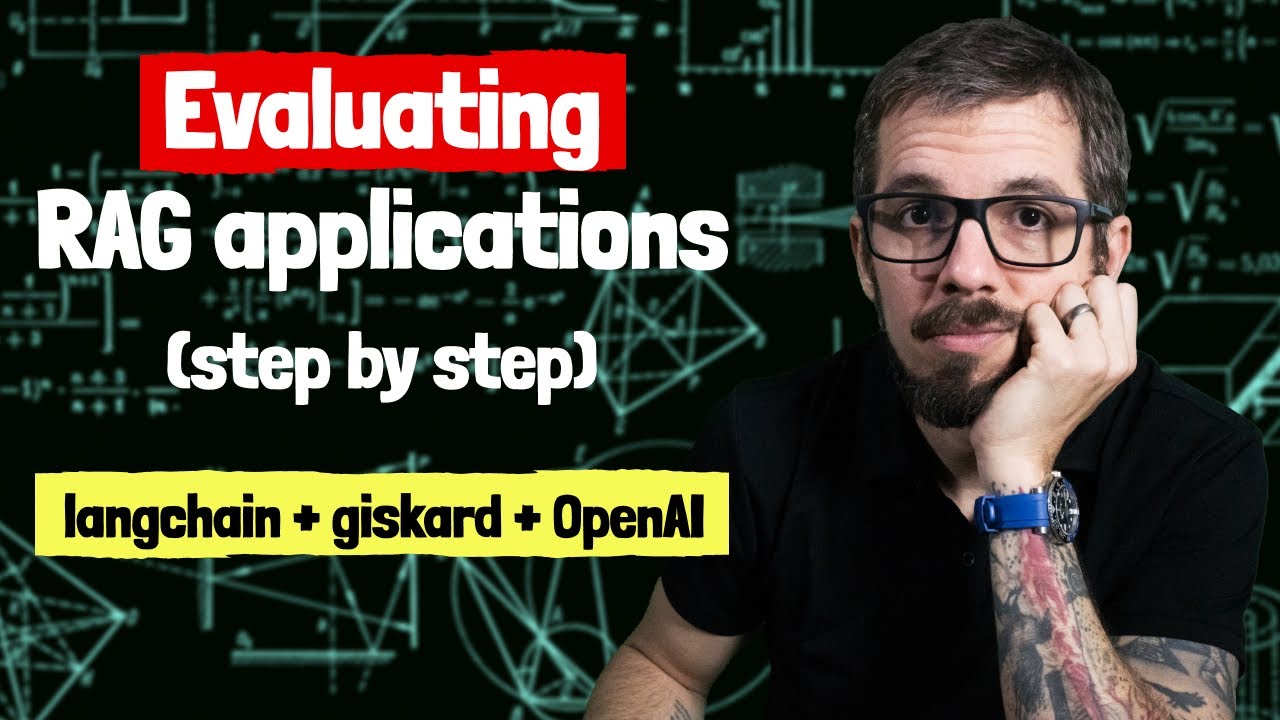
How to evaluate an LLM-powered RAG application automatically.
5.0 / 5 (0 votes)