C_120 File Handling in C - part 2 | File Pointer and fopen() function
Summary
TLDRIn this video, the presenter introduces core concepts of file handling in C programming. The discussion focuses on the importance of file pointers, which allow interaction with files, and the use of the `fopen` function to open files in different modes such as read, write, and append. The video also emphasizes the significance of closing files using `fclose` to free up resources. Key file-handling functions like `fscanf`, `fprintf`, `fgetc`, and `fputc` are briefly introduced. The session is geared towards providing a foundational understanding of how to work with files in C, setting the stage for practical demonstrations in future videos.
Takeaways
- 😀 File handling in C allows data to be permanently stored on the hard disk, unlike RAM which is volatile.
- 😀 A file pointer, of type `FILE`, is required to interact with files in C. It points to a specific file in memory.
- 😀 The `FILE` type is defined in the `stdio.h` header file and should be used when declaring file pointers in C programs.
- 😀 A file pointer in C is created using the `fopen()` function, which opens the file and returns a pointer to it.
- 😀 The `fopen()` function takes two parameters: the file name and the mode (e.g., read, write, append).
- 😀 There are various modes available for opening files, including `r`, `w`, `a`, `r+`, `w+`, and `a+` for different file operations.
- 😀 After performing operations on a file, it is essential to close it using the `fclose()` function to release system resources.
- 😀 A file pointer allows you to read from or write to the file. Operations like `fscanf()`, `fputc()`, `fgets()` are used for this purpose.
- 😀 It is important to manage file operations properly: opening with `fopen()`, processing the file, and closing with `fclose()`.
- 😀 Proper understanding of file modes is crucial as they determine the type of operation you can perform on the file (e.g., reading or writing).
Q & A
What is the purpose of file handling in C programming?
-File handling in C programming is used to store data permanently in a file, which resides on the hard disk. It allows programs to read from, write to, or modify files even after the program execution ends.
Why do we need a file pointer in C file handling?
-A file pointer is needed to reference a file in memory. It allows programs to perform operations like reading, writing, and appending to a file by pointing to a file-type variable that stores the file's information.
What is the role of the `fopen()` function?
-The `fopen()` function is used to open a file in a specific mode (read, write, append, etc.). It returns a pointer to the file, which can then be used to perform operations on the file.
What are the common modes used in `fopen()`? Provide examples.
-Common modes in `fopen()` include 'r' for read, 'w' for write, 'a' for append, 'r+' for reading and writing, 'w+' for writing and reading, and 'a+' for appending and reading. For example, 'fopen('file.txt', 'w')' opens a file for writing.
What happens if `fopen()` fails to open a file?
-If `fopen()` fails, it returns `NULL`, indicating that the file could not be opened. This could be due to issues such as an incorrect path, insufficient permissions, or a non-existent file.
What is the significance of the file pointer `fp` in file handling?
-The file pointer `fp` stores the address of the file object created by `fopen()`. It points to the file in memory and is used to perform file operations like reading or writing data.
Why is it necessary to close a file after operations in C?
-Closing a file using `fclose()` ensures that any changes made to the file are saved, and it frees up system resources. It also prevents file corruption and memory leaks.
What would happen if a file is not closed after being opened in a program?
-If a file is not closed, the program may not save the data properly, and system resources may not be released. This can lead to memory leaks and may prevent other programs from accessing the file.
Can you explain the relationship between the file pointer and the buffer in memory?
-The file pointer refers to a file object in memory, and the buffer is a temporary storage area where file content is loaded during program execution. The pointer helps access the data in this buffer, reflecting changes in the file on disk.
What is the syntax for the `fopen()` function and its parameters?
-The syntax for `fopen()` is `FILE *fopen(const char *filename, const char *mode);`. The `filename` is the name (and optionally path) of the file, and `mode` specifies the file access type (e.g., 'r', 'w').
Outlines
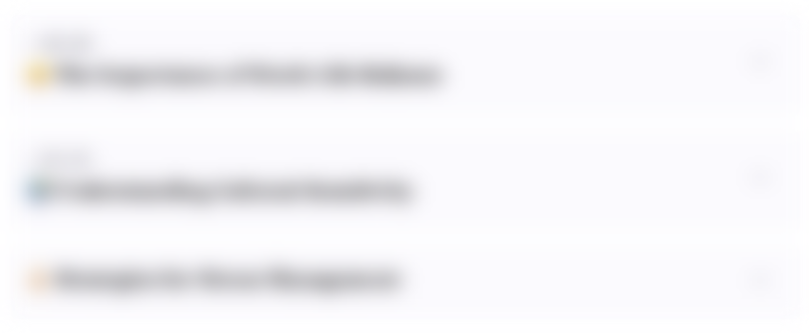
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
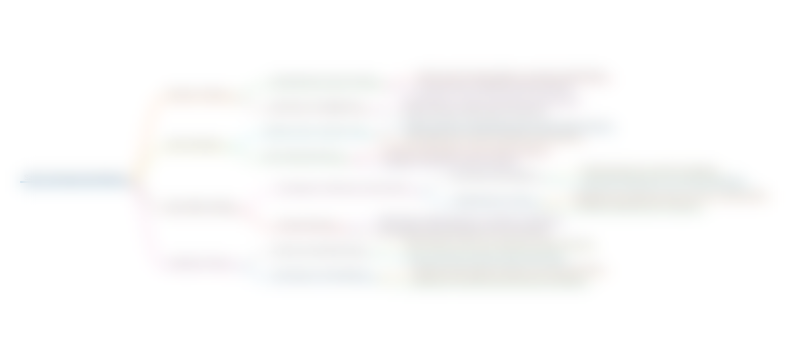
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
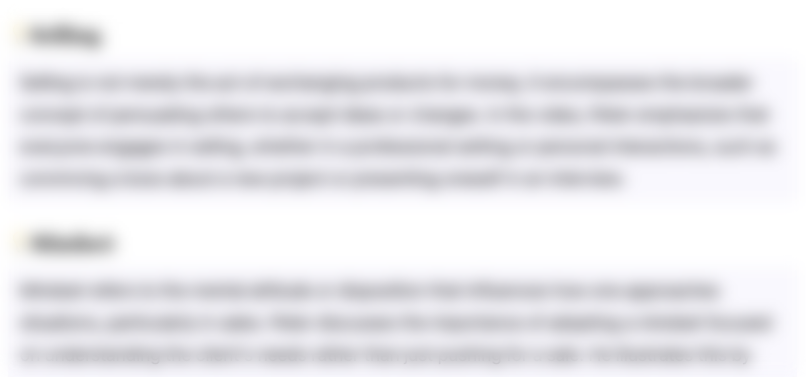
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
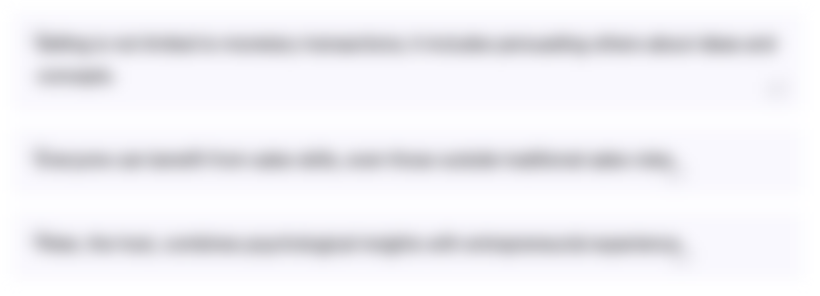
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
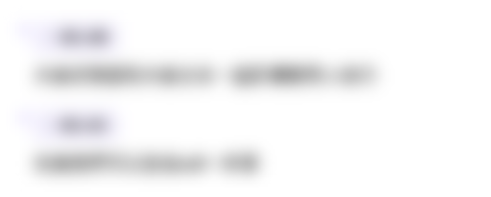
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
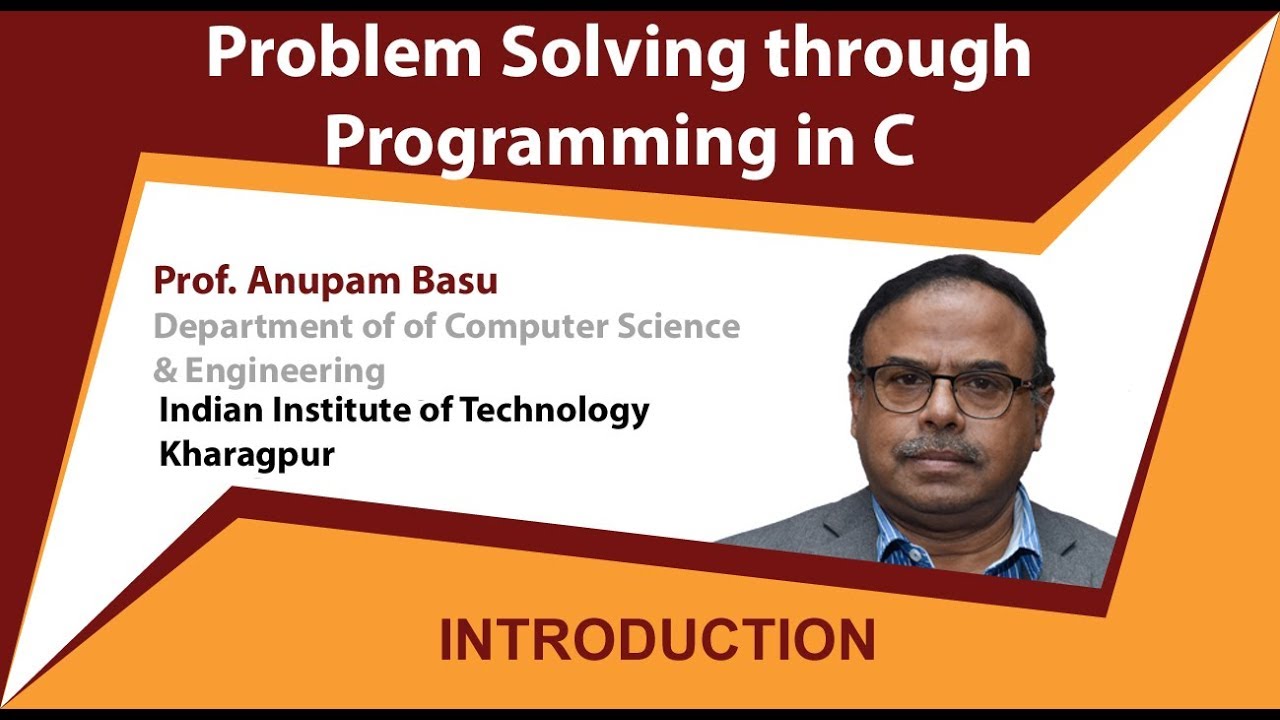
Prof A Basu

C_119 File Handling in C - part 1 | Introduction to Files
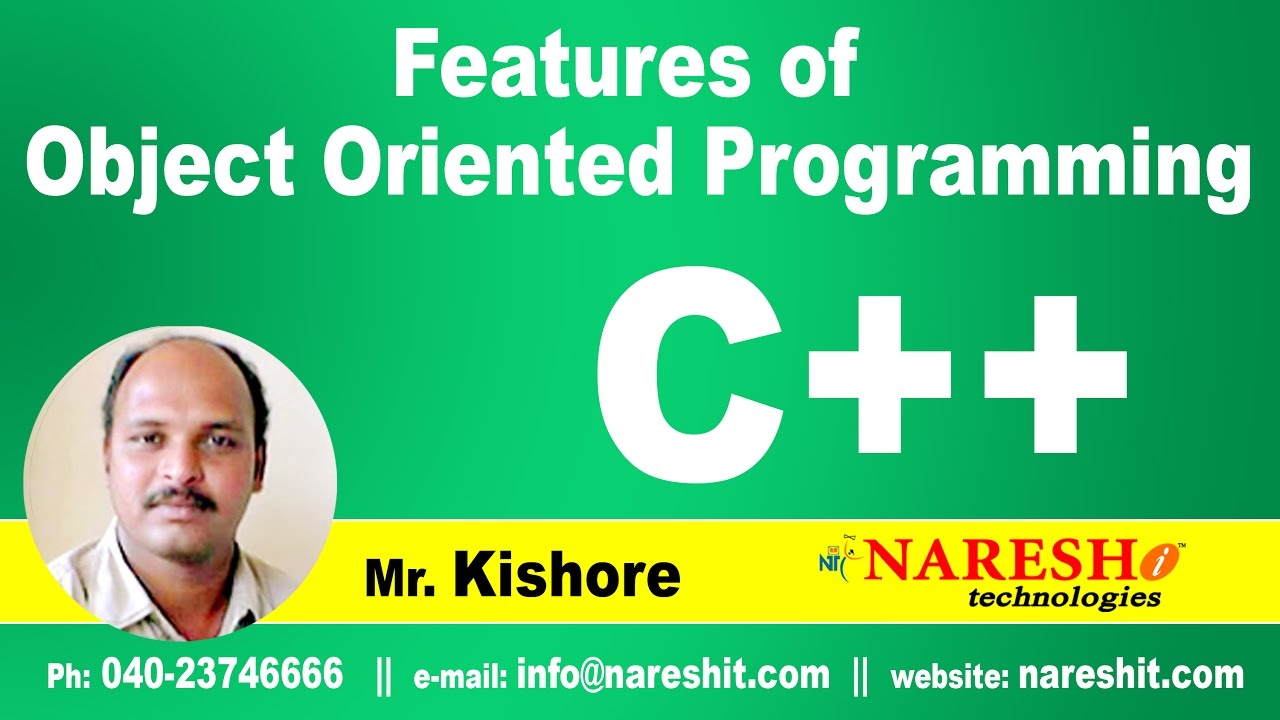
Features of Object Oriented Programming Part 2 | C ++ Tutorial | Mr. Kishore
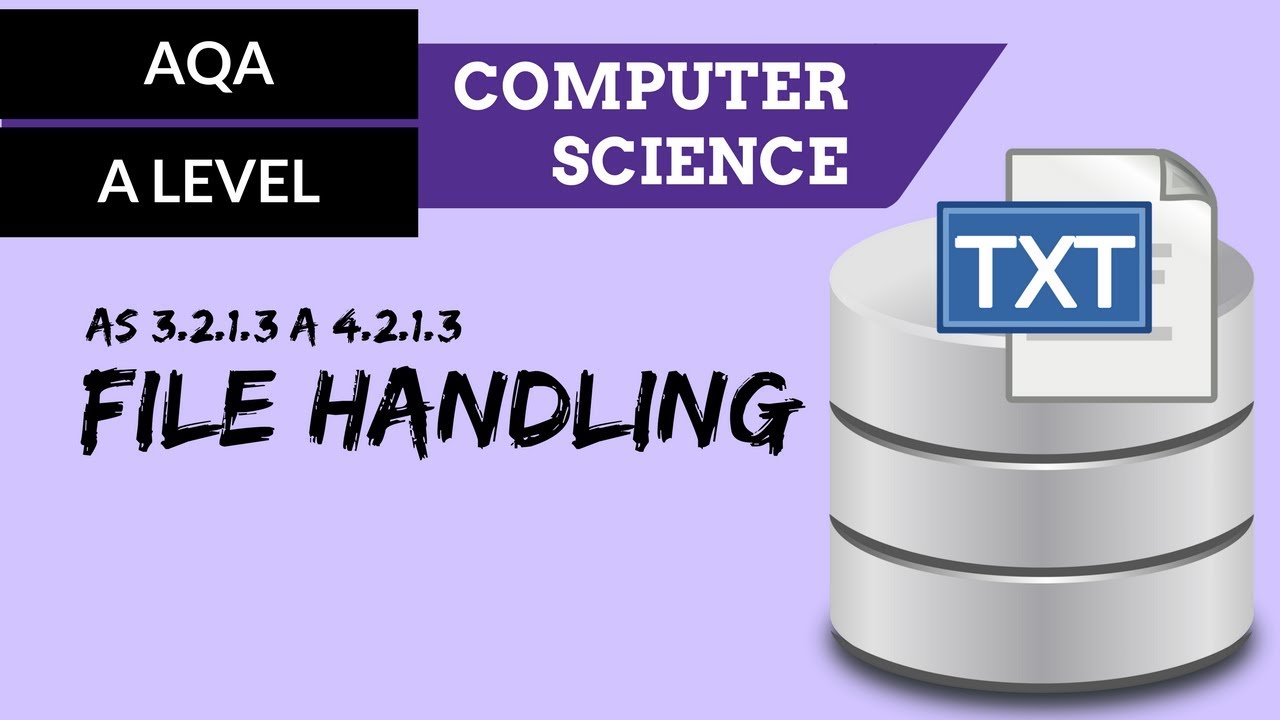
AQA A’Level File handling

Part 1 Introduction || C#.Net Tutorials For Beginners & Experienced || @NehanthWorld
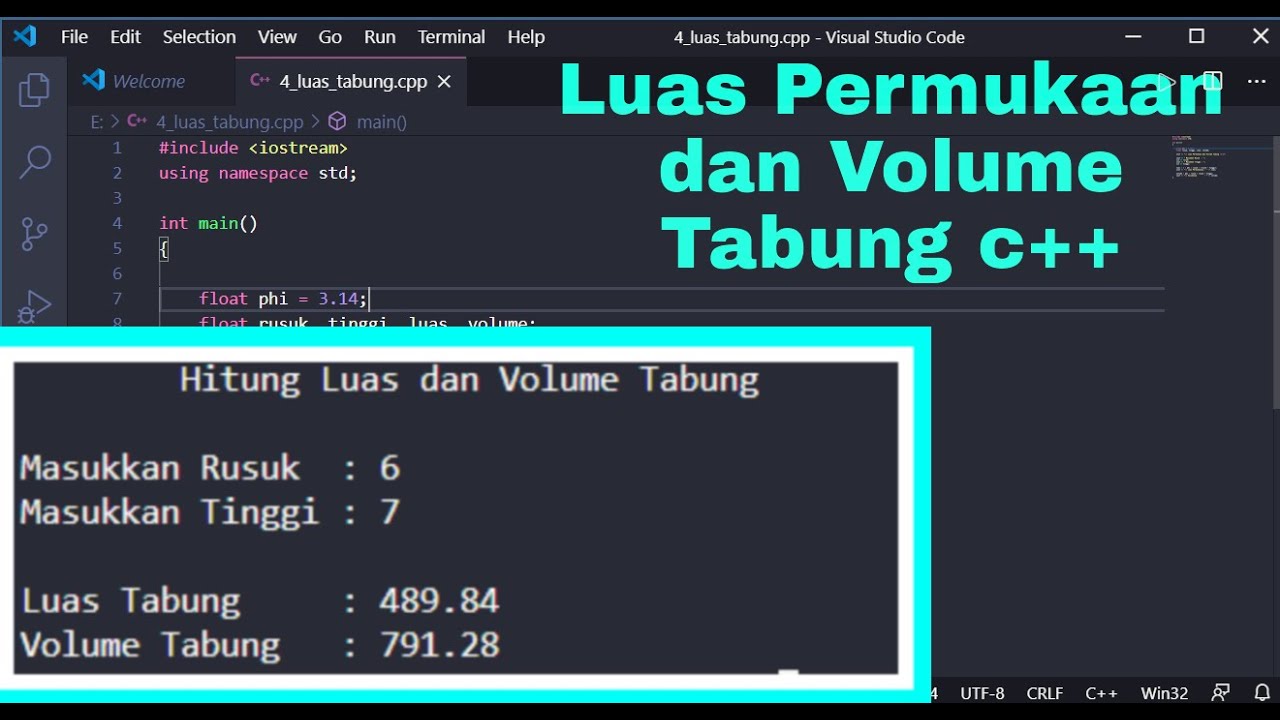
Menghitung Luas Permukaan dan Volume Tabung c++
5.0 / 5 (0 votes)