Shopping Cart project in .net core mvc (with authentication) | part 2
Summary
TLDRIn this tutorial, Ravindra walks through the process of adding role-based user management to an e-commerce project in .NET 7 MVC. He demonstrates how to add a new `StatusID` field to the order status table and update the database. The tutorial covers creating user roles (User, Admin) using enums and assigning roles to users. It also includes database seeding to ensure roles and an admin user are created automatically. Finally, Ravindra tests the login functionality for different roles, showing the admin display in the UI, and sets the stage for further UI enhancements in future lessons.
Takeaways
- π Add a new field 'StatusID' to the OrderStatus table and update the database using migrations.
- π Incorporate role management by defining 'User' and 'Admin' roles with an enum.
- π Update the registration process to assign roles (User/ Admin) to new users after successful registration.
- π Seed default roles (User, Admin) into the database, ensuring they exist before use.
- π Use the UserManager and RoleManager services to create and assign roles to users programmatically.
- π Create an admin user if it does not already exist, and assign them the Admin role.
- π Adjust the Program.cs file to call the seed method during application startup for role initialization.
- π Test role-based authentication by displaying user roles (Admin) in the UI after login.
- π Implement a check in the UI to display 'Admin' text when the logged-in user has an Admin role.
- π Bootstrap can be used to enhance the UI design, making the project visually responsive and user-friendly.
- π Ensure that migrations are applied correctly to avoid errors during role and user creation in the database.
Q & A
What is the purpose of adding the 'status ID' field in the order status table?
-The 'status ID' field is added to the order status table to enable tracking and categorization of the status of orders in the database. It is an integer field that will help in managing and querying order statuses more effectively.
Why is it important to run migration commands after modifying the database schema?
-Running migration commands updates the database schema to reflect changes in the application. This ensures that the database structure matches the applicationβs requirements, such as adding new fields or tables, which is necessary for the application to function correctly.
What is the role of the 'rules' enum created in the constants folder?
-The 'rules' enum defines the different roles in the application, such as 'user' and 'admin'. It allows the application to manage user roles in a structured way and assign these roles to users during registration.
What does the 'userManager.AddToRoleAsync' method do in the role management process?
-The 'userManager.AddToRoleAsync' method assigns a specified role to a user in the system. For example, after a user registers, they are assigned a role like 'user' or 'admin', which determines their level of access in the application.
What is the purpose of the 'seedDefaultData' method in the database seeding process?
-The 'seedDefaultData' method is used to populate the database with initial or default data. In this case, it seeds the roles (like 'admin' and 'user') and an 'admin' user into the database, ensuring that these essential roles are available for the application to function properly.
Why is the 'identityRole' used when creating roles in the database?
-The 'identityRole' is a predefined class in ASP.NET Identity that represents a role in the application's role-based access control system. It is used to define and manage roles such as 'user' and 'admin', which are assigned to users for authorization purposes.
What issue is resolved by adding a separate scope when seeding roles and users into the database?
-Adding a separate scope is necessary to avoid errors related to service resolution in the dependency injection system. By creating a new scope, the application ensures that the services required for seeding roles and users are available in the appropriate context, preventing potential issues with service lifetimes.
What does the 'await userManager.CreateAsync' method do when creating a new user?
-The 'await userManager.CreateAsync' method asynchronously creates a new user in the database, setting their properties such as username, email, and password. It also confirms the user's email address as part of the registration process.
Why does the tutorial use role-based checks in the layout page to display the 'admin' label?
-Role-based checks are used to determine the user's role after they log in. If the logged-in user is an admin, the 'admin' label is displayed in the UI, indicating their administrative privileges. This allows the application to dynamically adjust the content shown based on the user's role.
What steps does the tutorial suggest for improving the UI after implementing authentication and role management?
-After implementing authentication and role management, the tutorial suggests enhancing the UI with Bootstrap to improve design and layout. This includes making the UI more user-friendly and ensuring it is visually appealing, especially for managing user interactions like login, registration, and displaying user roles.
Outlines
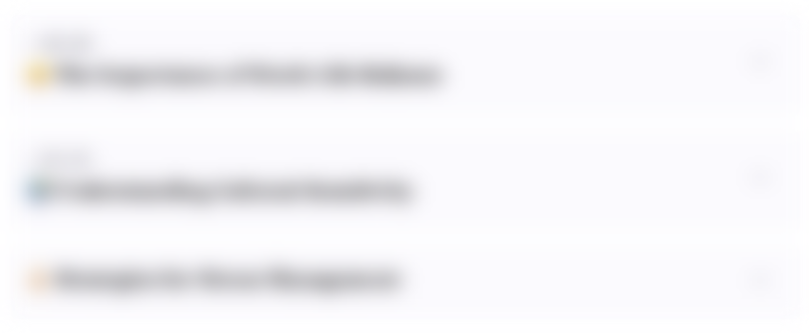
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
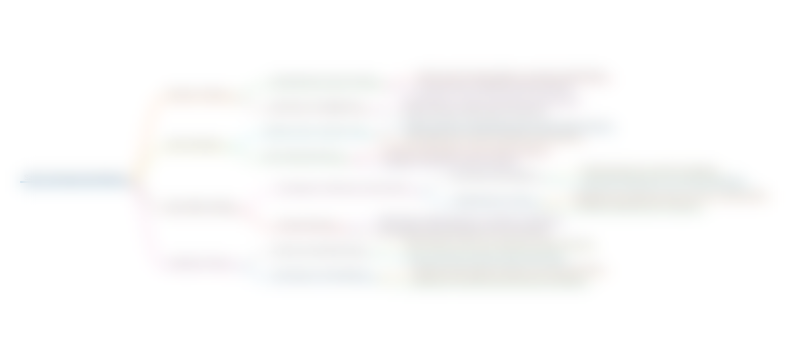
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
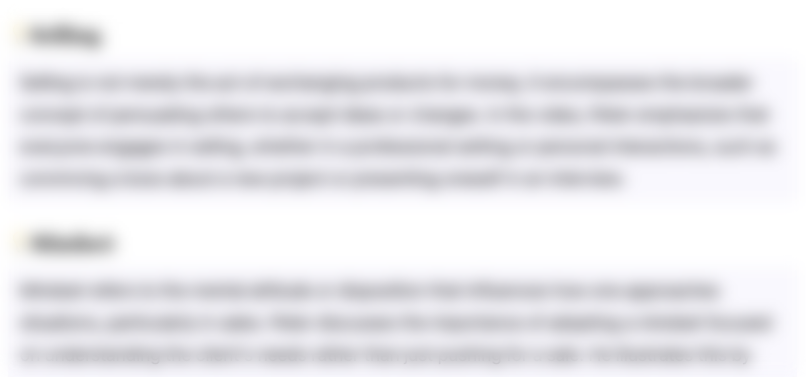
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
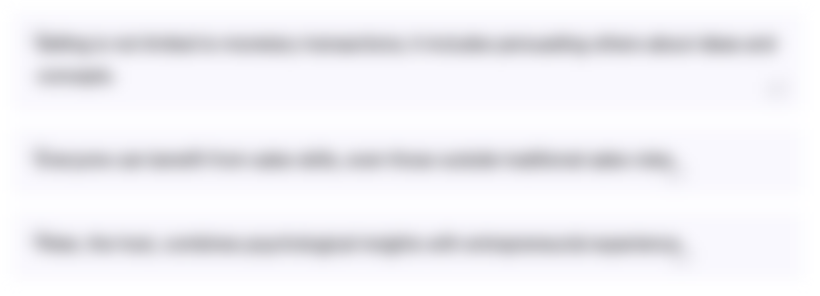
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
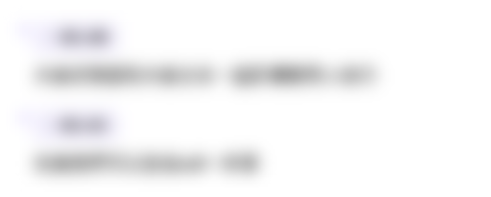
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
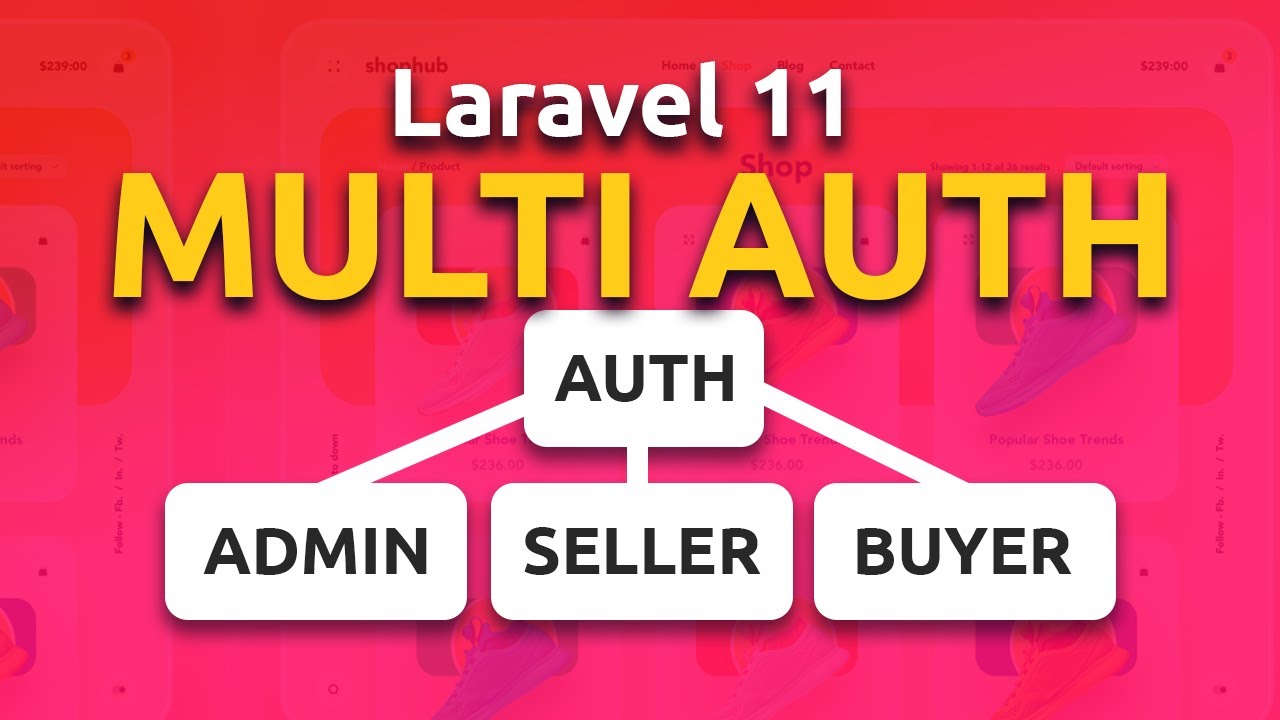
Laravel 11 Multi Auth For Ecommerce Website | Step By Step
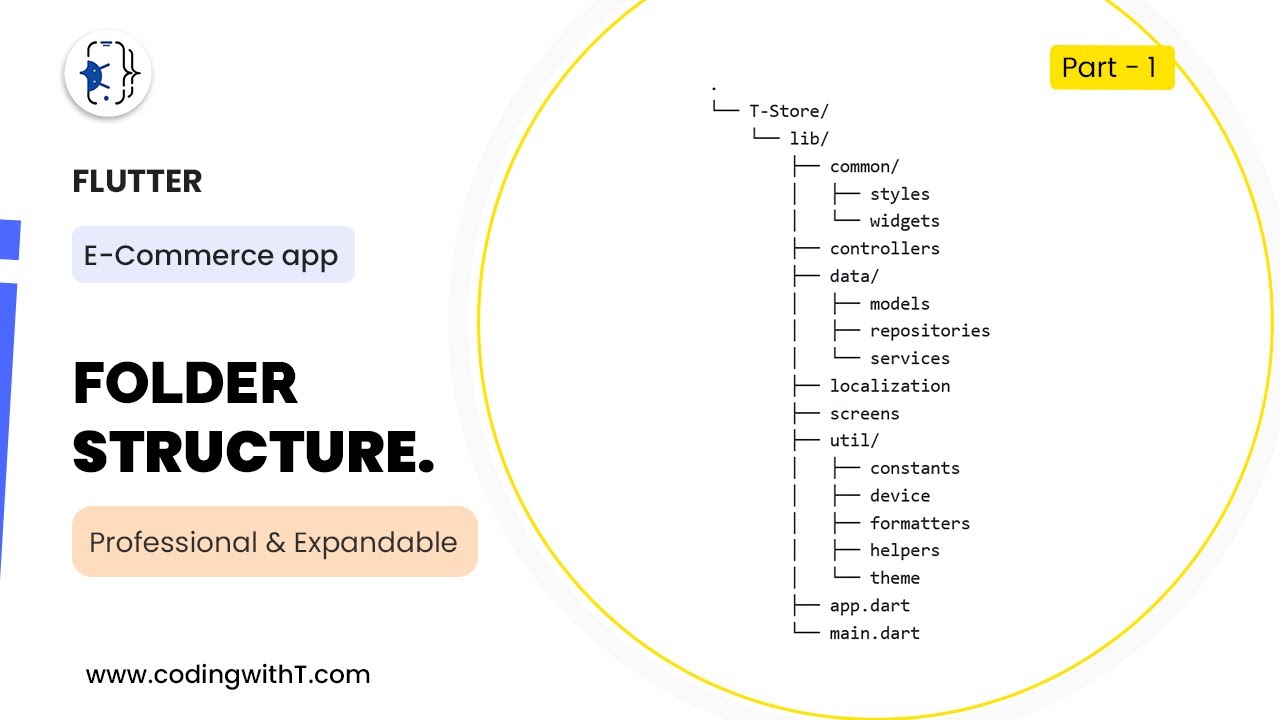
Efficient Folder Structures for Large Flutter Apps | Feature-First vs. Module-First Approach

Google Data Studio Tutorial π - How to build a Dashboard with GDS
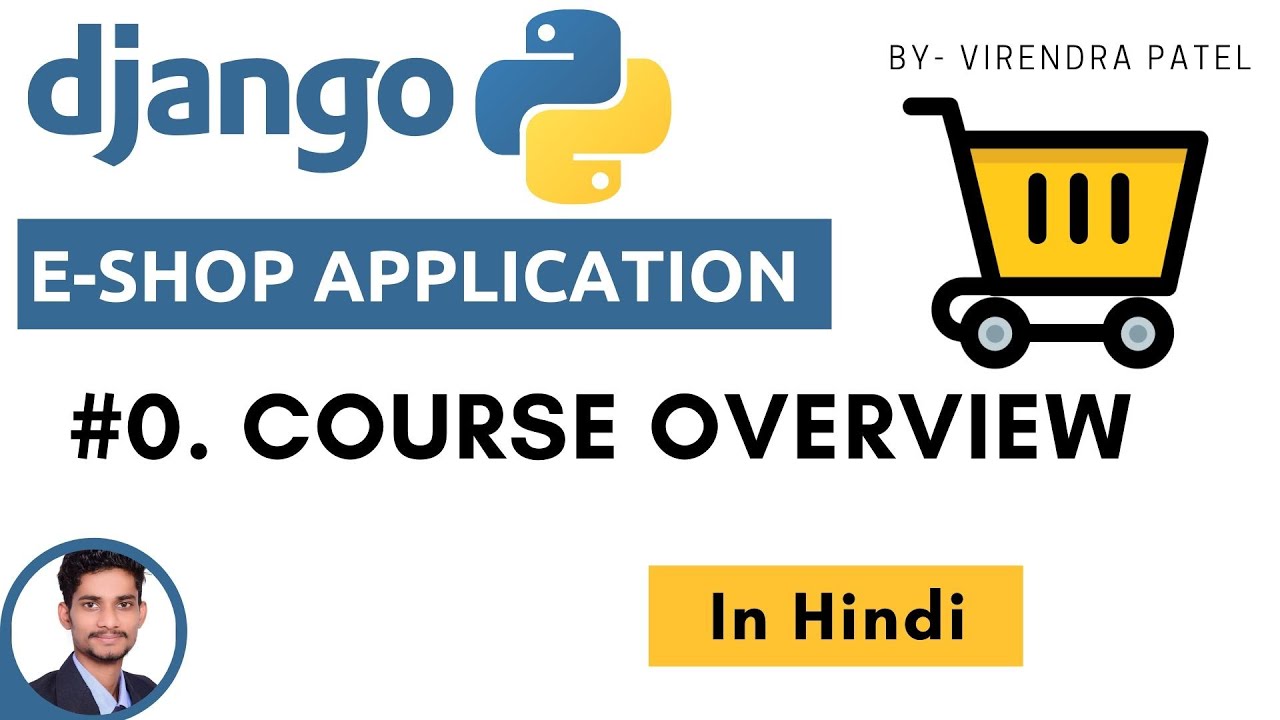
#0. Overview | Imaginary E-Commerce Application | Django | In Hindi π₯π³
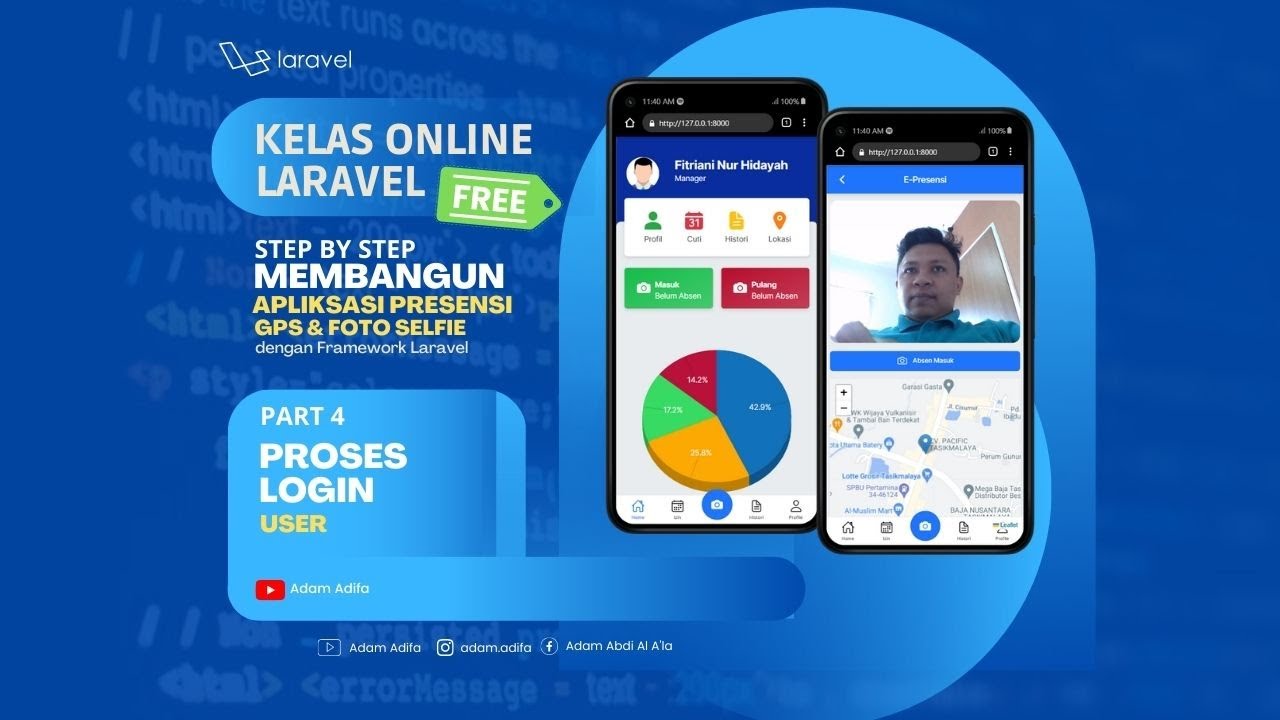
04 - Membuat Proses Login Untuk User
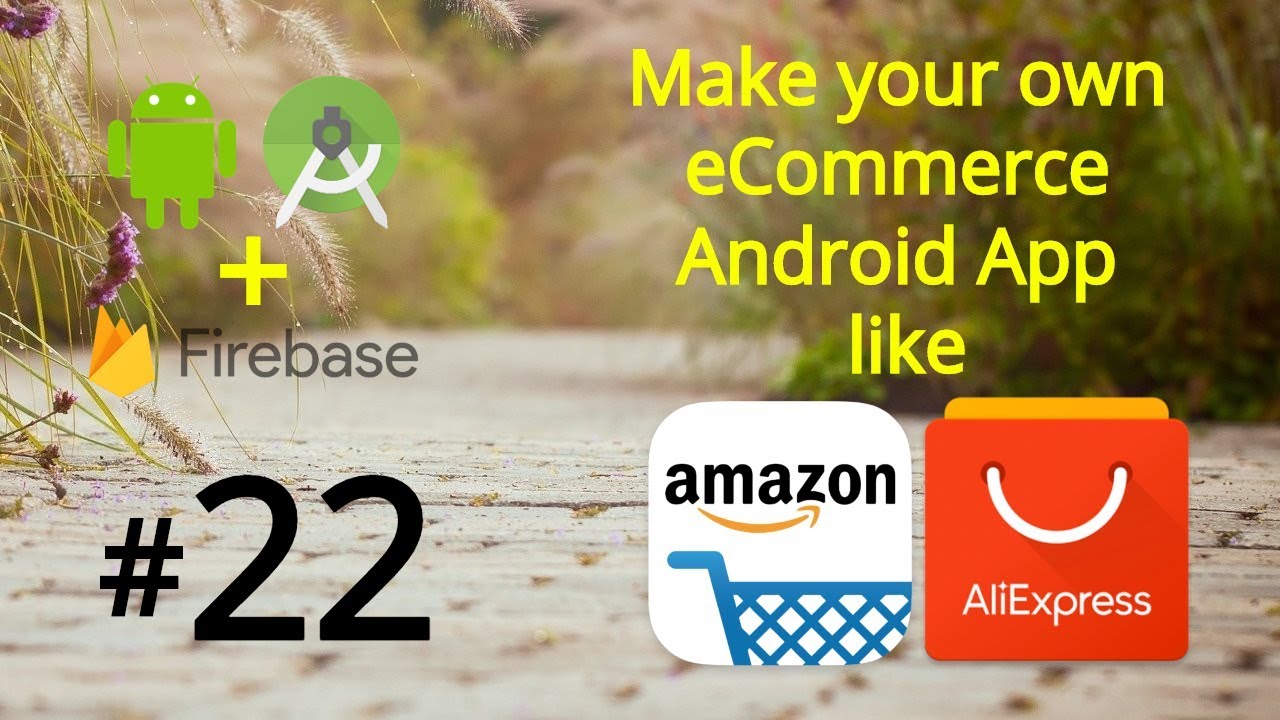
Android Shopping Cart Tutorial - Android Firebase eCommerce App like Amazon
5.0 / 5 (0 votes)