Web Development 1.3 - Creating Elements Using the DOM
Summary
TLDRIn this lesson, you'll learn how to interact with HTML elements using the DOM. We explore accessing existing elements, modifying content with `innerHTML`, and creating new elements using `createElement`. You'll also learn how to add new elements to a page using `appendChild` and `insertBefore`, placing them exactly where you want. The lesson explains helpful DOM navigation methods like `firstElementChild`, `lastElementChild`, and `parentElement`, to easily traverse and manipulate the structure. By the end, you'll be able to dynamically modify and create HTML content, with examples to practice your new skills.
Takeaways
- π The Document Object Model (DOM) allows us to manipulate HTML elements using JavaScript.
- π The 'document' keyword provides direct access to elements like title and body in an HTML file.
- π '.innerHTML' is used to access and change the content of an existing HTML element.
- π The 'createElement' function allows you to create new HTML elements dynamically using JavaScript.
- π The 'createElement' function requires a string parameter that specifies the type of element to create (e.g., 'p' for paragraph).
- π Newly created elements, like a <p> tag, start off without any content or attributes.
- π To add content to a new element, you can modify its '.innerHTML' property.
- π The 'appendChild' function adds a newly created element as the last child of a specified parent element (e.g., the body).
- π 'insertBefore' allows you to add a new element before an existing one within a parent element.
- π Helper methods like '.firstElementChild', '.lastElementChild', '.children', and '.parentElement' assist in navigating and accessing parent and child elements in the DOM.
- π JavaScriptβs DOM manipulation enables creating and positioning new elements dynamically in a webpage, improving interactivity and structure.
Q & A
What is the purpose of the Document Object Model (DOM)?
-The DOM is a programming interface for HTML and XML documents. It represents the document as a tree structure where each element is a node. It allows us to interact with and manipulate the structure, style, and content of web pages dynamically.
How can you access HTML elements using the DOM?
-You can access HTML elements using the `document` object, followed by the element's identifier or type. For example, `document.title` accesses the title of the page, and `document.body` accesses the body of the HTML document.
What does the `.innerHTML` property do?
-.innerHTML allows you to access and modify the HTML content of an element. By setting `innerHTML`, you can update the content inside an element, like text or HTML tags.
What is the purpose of the `createElement` method?
-The `createElement` method creates a new HTML element as a JavaScript object. The new element is not yet part of the page, but it can be customized and added later.
How can you add newly created elements to a webpage?
-You can use methods like `appendChild` and `insertBefore` to add newly created elements to the DOM. `appendChild` adds the element to the end of the parent element, while `insertBefore` allows you to insert the new element before a specified child.
What is the difference between `appendChild` and `insertBefore`?
-`appendChild` adds a new element as the last child of the specified parent element. `insertBefore`, on the other hand, allows you to specify where the new element should be placed, before an existing child element.
Can you explain how to use `insertBefore` with an example?
-To use `insertBefore`, first identify the parent element and the reference element before which you want to insert the new element. For example, to insert a subtitle before a paragraph with the ID `essay`, you would use `document.body.insertBefore(subtitle, essay);`
What are some useful helper methods for navigating the DOM?
-Some useful DOM navigation methods include `.firstElementChild` (returns the first child element), `.lastElementChild` (returns the last child element), `.children` (returns a collection of all child elements), and `.parentElement` (returns the parent element of the current element).
How do you find the first and last child elements of an element?
-To find the first and last child elements of an element, you can use `firstElementChild` and `lastElementChild`. For example, `document.body.firstElementChild` gives the first child of the body, and `document.body.lastElementChild` gives the last.
How can you identify an element's parent using the DOM?
-You can identify an element's parent using the `.parentElement` property. For example, if you have a reference to a child element, you can access its parent element like this: `childElement.parentElement`.
Outlines
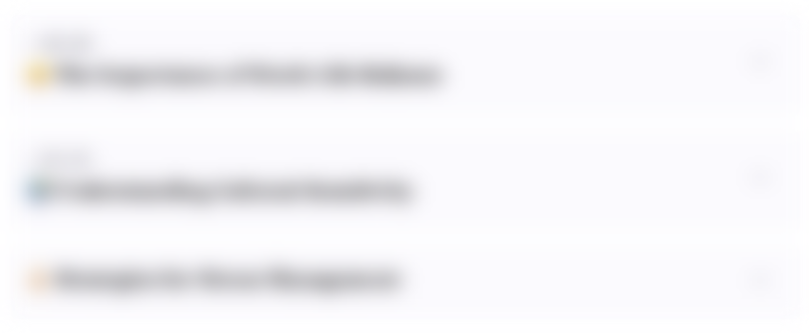
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
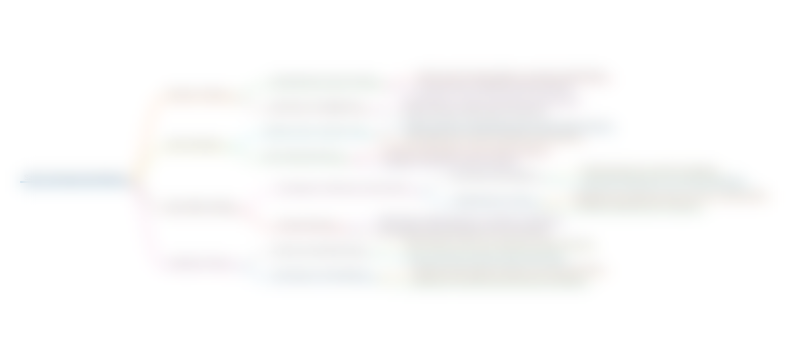
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
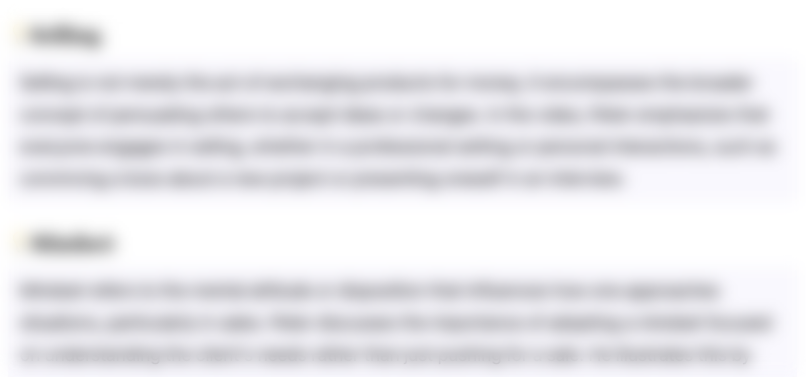
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
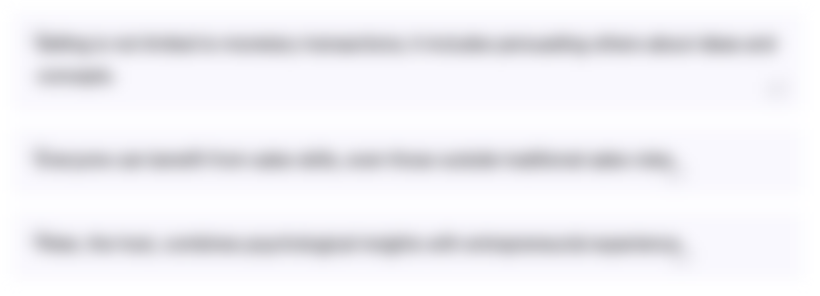
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
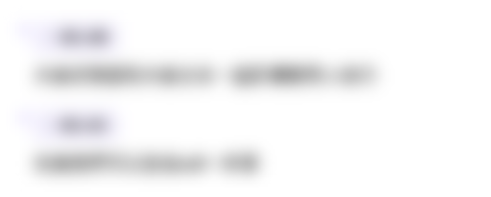
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)