1.2 Array Operations - Traversal, Insertion | Explanation with C Program | DSA Course
Summary
TLDRThis video explains how to insert data into an array at specific positions, including handling invalid positions and inserting at the beginning or end of the array. It covers the process of shifting elements and adjusting the array size accordingly. The time complexity of the insertion operation is discussed, highlighting how it varies depending on the position of insertion, with the best case scenario for unsorted arrays being constant time (Theta(1)). The speaker also touches on the importance of maintaining order in sorted arrays and introduces the upcoming topic of data deletion from arrays.
Takeaways
- 😀 Validating array insertion positions is crucial, ensuring the position is within the correct range (greater than 0 and less than or equal to size).
- 😀 Insertion at specific positions in an array requires shifting elements to the right to make space for the new value.
- 😀 Inserting at the beginning of an array involves shifting all elements, which increases the time complexity to O(n).
- 😀 Inserting at the end of the array does not require shifting any elements and is an O(1) operation.
- 😀 Invalid insertion positions should be handled by printing an error message if the position is out of bounds.
- 😀 When inserting at the beginning, the array elements are shifted from the last index to the first to make room for the new value.
- 😀 For an unsorted array, inserting a value at the end can be done efficiently in O(1) time, without needing to rearrange existing elements.
- 😀 The time complexity for inserting at any position in an array depends on the position chosen, with the worst case being O(n).
- 😀 Inserting in a sorted array requires additional considerations to maintain the order, leading to a higher time complexity due to element shifting.
- 😀 The best insertion strategy in an unsorted array is to insert the new element at the end of the array for optimal performance (O(1)).
Q & A
What is the first step to insert data at a specific position in an array?
-The first step is to validate the position by checking if it is greater than 0 and less than or equal to the size of the array. If the position is invalid, an error message is printed.
What happens when a user provides an invalid position for insertion?
-If the position is invalid (less than or equal to 0, or greater than the size of the array), the program prints 'Invalid Position' and does not proceed with the insertion.
How are elements shifted in the array during insertion?
-During insertion at a specific position, elements from the last index to the given position are shifted one position to the right to make room for the new element.
What is the time complexity of inserting an element in an array?
-The time complexity of inserting an element at a specific position is O(n) in the worst case because elements might need to be shifted. In the best case, when inserting at the end, it can be O(1).
How do you insert an element at the beginning of an array?
-To insert an element at the beginning of an array, all elements need to be shifted one position to the right, starting from the last index. The new element is then inserted at index 0.
What modification is made in the code to insert an element at the beginning?
-The for loop is modified to start from the last index (`size - 1`) and go down to index 0, shifting all elements to the right. The new element is inserted at index 0, and the size of the array is increased.
How is insertion at the end of the array handled differently?
-Inserting at the end of the array does not require shifting any elements. The new element is simply inserted at the index equal to the current size of the array, and the size is then incremented.
What is the time complexity when inserting at the end of the array?
-Inserting at the end of the array takes constant time, O(1), as no shifting of elements is required.
What is the best method to insert data in an unsorted array?
-In an unsorted array, the best method is to insert the new element at the end of the array and update the size. This avoids shifting elements and takes constant time, O(1).
How does the insertion method differ in a sorted array compared to an unsorted array?
-In a sorted array, you cannot insert an element at the end directly because you need to preserve the order. You must shift elements and perform comparisons to insert the new element at the correct position, which increases the time complexity.
Outlines
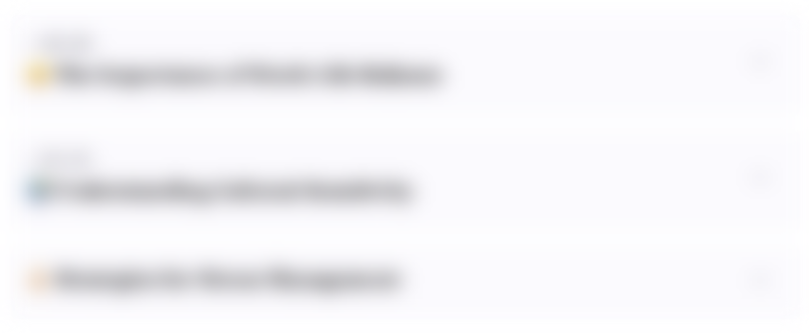
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
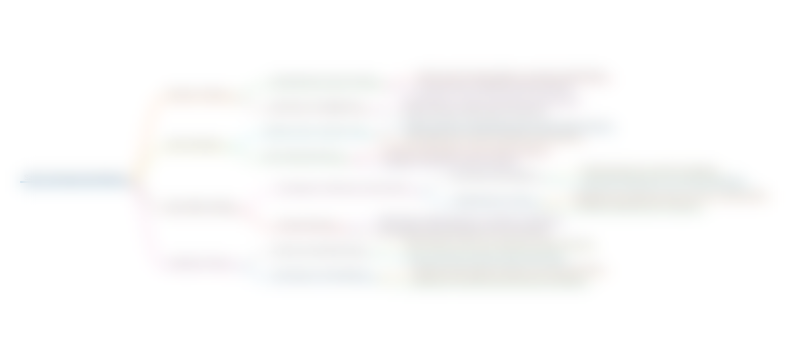
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
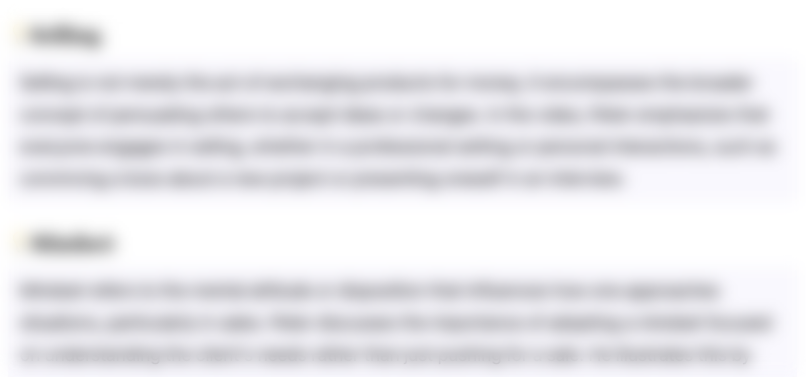
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
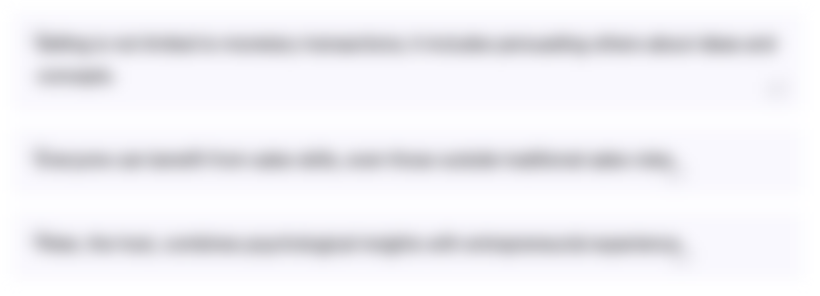
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
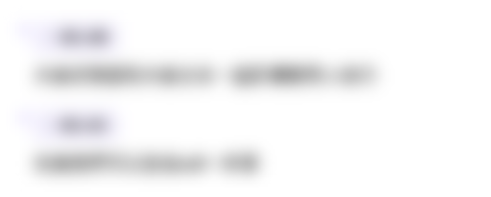
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)