C_104 Recursion in C | Introduction to Recursion
Summary
TLDRThis video serves as an introduction to recursion in C programming, explaining its definition and significance in exams and interviews. The instructor clarifies the concept of recursion, differentiating between direct and indirect recursion, and highlights the crucial role of base conditions to prevent infinite loops. Using a factorial calculation as an example, the video demonstrates how recursive functions operate through stack frames, illustrating the process of calling a function within itself. Viewers can expect to gain a foundational understanding of recursion, setting the stage for more complex topics in future videos.
Takeaways
- 😀 Recursion in C is when a function calls itself directly or indirectly, making it a fundamental concept in programming.
- 😀 Understanding recursion is essential for competitive exams and interviews, where questions often focus on this topic.
- 😀 The base condition in a recursive function is crucial; it determines when the recursion stops to prevent infinite loops.
- 😀 Types of recursion include direct, indirect, tail, and non-tail recursion, each with its specific characteristics.
- 😀 An example of recursion is calculating factorial, where n! can be expressed as n × (n-1)!.
- 😀 Without proper base conditions, recursive functions can lead to stack overflow and undefined behavior.
- 😀 When a recursive function executes, memory is allocated for each call, creating a stack frame that stores local variables.
- 😀 The process of recursion involves breaking down problems into smaller subproblems, eventually reaching the base case.
- 😀 In recursion, once the base condition is met, the function returns values back through the chain of calls, resolving the initial problem.
- 😀 Mastering the concept of recursion can simplify complex problems, making it easier to write efficient algorithms.
Q & A
What is recursion in C programming?
-Recursion in C programming occurs when a function calls itself, either directly or indirectly, to solve a problem by breaking it down into smaller subproblems.
Why is recursion considered a tough topic for beginners?
-Beginners often find recursion challenging because it requires understanding both the recursive process and the importance of base conditions to prevent infinite loops.
What are the two main types of recursion?
-The two main types of recursion are direct recursion, where a function calls itself, and indirect recursion, where a function calls another function that eventually calls the first function.
What is a base condition in recursion?
-A base condition is a stopping criterion for the recursive function, ensuring that the recursion terminates at a certain point, preventing infinite loops.
Can you provide an example of a base condition in a factorial calculation?
-In a factorial calculation, the base condition is typically when n is equal to 0 or 1, as both 0! and 1! equal 1.
What could happen if the base condition is not implemented correctly?
-If the base condition is not correctly implemented, it can lead to infinite recursion, resulting in a stack overflow error due to excessive memory usage.
What is the difference between tail recursion and non-tail recursion?
-In tail recursion, the recursive call is the last operation in the function, allowing for optimization, while in non-tail recursion, additional operations follow the recursive call.
How can recursion be advantageous in programming?
-Recursion can simplify code for problems that can be divided into smaller, similar problems, making the implementation cleaner and more intuitive.
What example was provided in the transcript to illustrate recursion?
-The example provided was the calculation of factorial, showing how n! can be expressed in terms of (n-1)! with a base case to terminate the recursion.
What should programmers be cautious of when using recursion?
-Programmers should be cautious about memory usage and performance issues that can arise from excessive function calls and ensure proper base conditions to avoid stack overflow.
Outlines
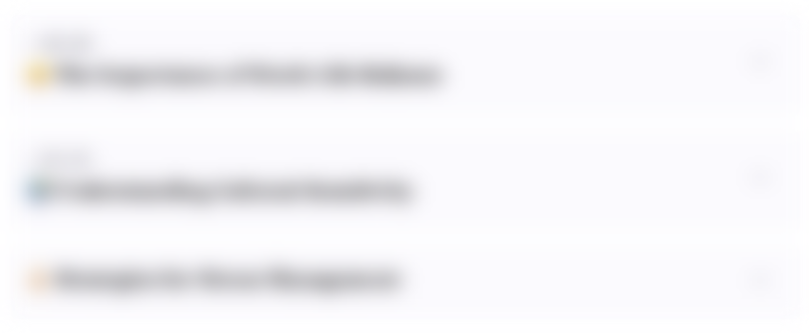
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
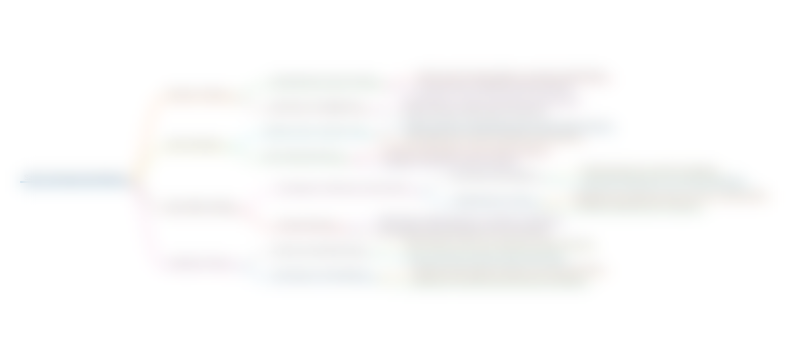
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
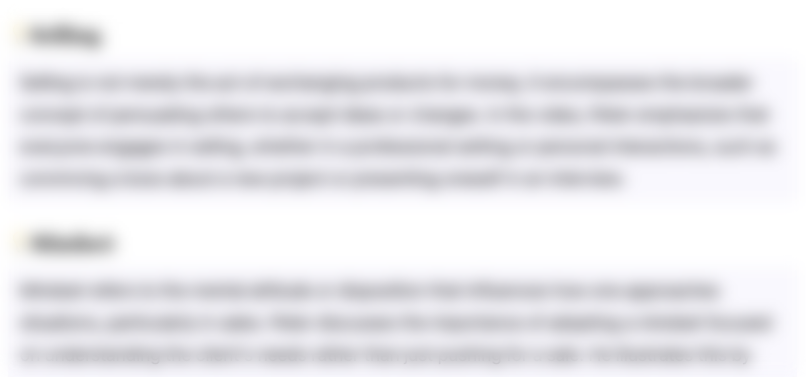
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
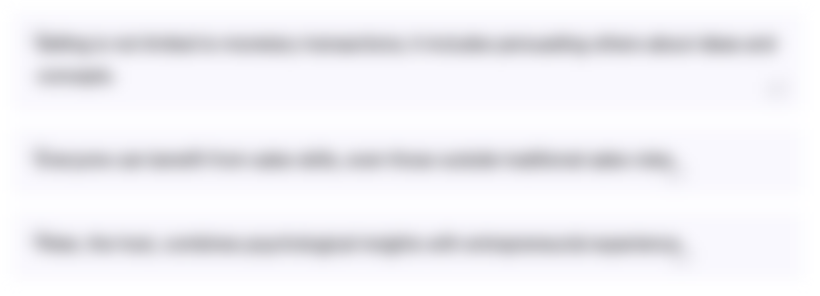
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
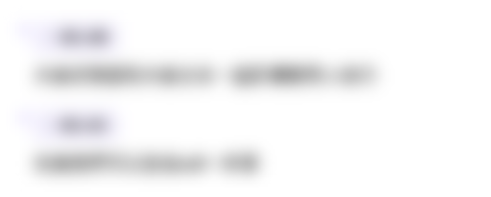
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)