11. Kombinasi Konsep Percabangan dan Perulangan
Summary
TLDRIn this class on computer programming, students learn about combining branching and looping concepts, including using `if`/`else` statements with loops like `while`, `for`, and `do-while`. Key assignments include writing programs to check if a number is even or odd, checking for prime numbers, and using nested loops to create patterns such as squares and diamonds. The class emphasizes understanding logic, error checking, and debugging, encouraging students to complete assignments via Google Classroom and prepare for upcoming lessons on loops and pattern generation. Practical examples and solutions guide students in mastering these foundational programming concepts.
Takeaways
- π Introduction to the concept of loops and conditionals in programming, covering types like for, while, and do-while loops.
- π Task to create a program that checks if a number is even or odd, looping until the user inputs -999 to stop.
- π Explanation of the difference between if-else and various looping structures like while and do-while.
- π Clear instructions on how to write a program that repeatedly accepts user input and checks if it's even or odd.
- π Introduction to checking prime numbers using a simple algorithm that checks divisibility from 2 to n.
- π Detailed explanation of how to implement loops and conditionals to check for prime numbers and handle edge cases (like 1 not being prime).
- π Nested loops concept introduced, with examples on how to generate patterns like diamonds and inverted triangles using loops.
- π Emphasis on how nested loops can be used for practical applications, such as generating different shapes or patterns in console-based programs.
- π Example of a task where students need to create a program that prints a square using the hash (#) symbol based on user input for the side length.
- π Instructions on writing an algorithm to check if a number is prime, including handling edge cases and the logic of checking divisibility.
- π Students are encouraged to think about how to break down problems logically, with the importance of understanding basic programming concepts like loops and conditionals before moving on to more complex algorithms.
Q & A
What is the topic discussed in the script?
-The script covers an introductory computer software course, specifically focusing on nested loops and branching concepts, as well as practical examples such as checking if a number is even or odd and determining prime numbers.
What are the types of loops mentioned in the script?
-The script mentions three types of loops: for loops, while loops, and do-while loops. It explains the syntax differences between them and discusses when to use each type.
What is the purpose of the sample program provided in the script?
-The sample program is designed to take an integer input and determine if the number is even or odd. It continues to run until the user enters -999, which stops the program.
What is the key difference between a while loop and a do-while loop?
-The key difference is that a while loop checks the condition before executing the code block, while a do-while loop executes the code block first and checks the condition afterward.
How does the program stop when the user inputs -999?
-The program uses a while loop with the condition 'n != -999'. This ensures that the loop continues running as long as the input is not -999. When -999 is entered, the loop ends, and the program stops.
What does the modulus operator (n % 2) do in the sample program?
-The modulus operator checks if the remainder of dividing 'n' by 2 is 0. If the result is 0, the number is even; otherwise, it is odd.
What is the algorithm to check if a number is prime?
-The algorithm involves checking if a number 'n' is divisible by any number from 2 up to n-1. If n is divisible by any such number, it is not a prime. The program includes an optimization to exclude 2 and 3 from the divisibility check.
What is the role of the 'isPrime' variable in the prime number program?
-'isPrime' is a boolean variable used to track whether the number being checked is prime. It is initially set to true, and if the number is divisible by any number other than 1 and itself, it is set to false, indicating the number is not prime.
What is nested looping and how is it explained in the script?
-Nested looping refers to having one loop inside another. In the script, it is explained using examples of geometric patterns like diamonds and L-shapes, where outer loops control the rows, and inner loops control the columns for each row.
How does the script explain the concept of nested loops using patterns?
-The script demonstrates nested loops by showing how they can be used to generate geometric shapes like diamonds or L-shapes. The outer loop handles the overall structure, while the inner loop manages the content for each row.
Outlines
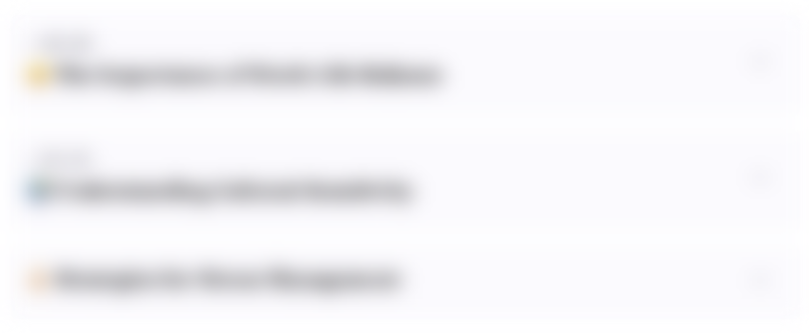
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
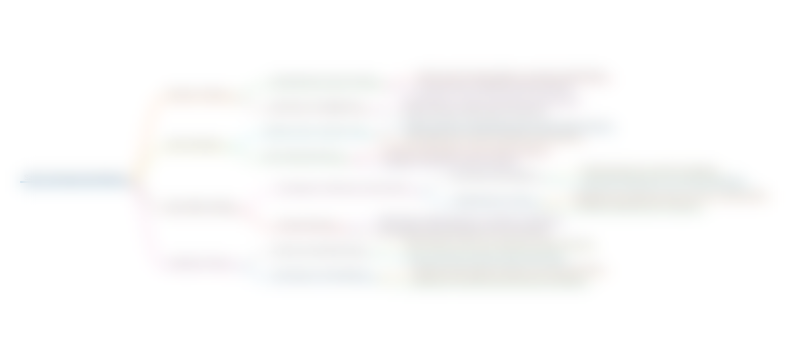
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
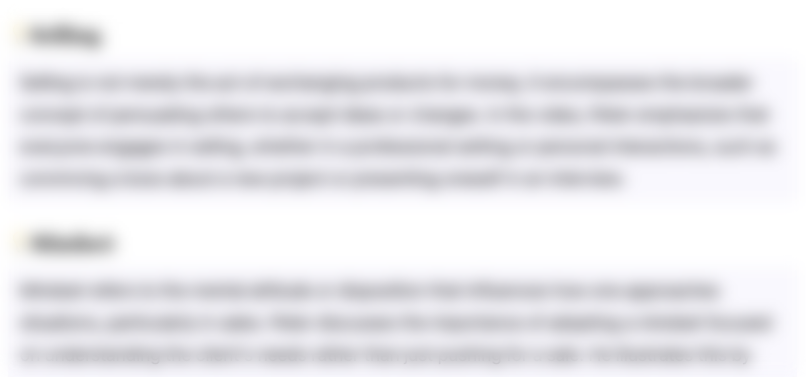
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
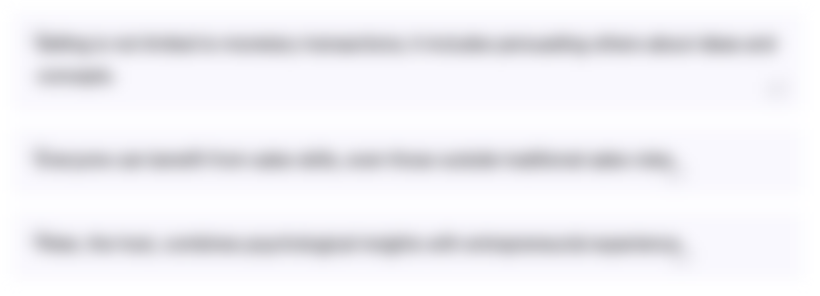
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
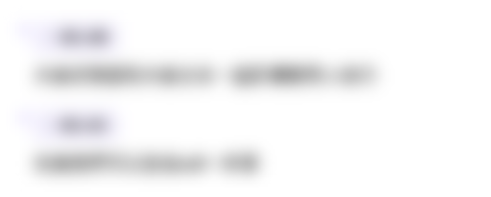
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)