#25 How to get selected endpoint | Understanding Routing | ASP.NET Core MVC Course
Summary
TLDRIn this tutorial, we explore the workings of ASP.NET Core's routing middleware. The video explains how the `useRouting` middleware identifies the appropriate route based on the incoming request URL and HTTP method. It highlights how the endpoint object is created after `useRouting` executes, and how it can be accessed using `GetEndpoint()` for debugging or logging purposes. Through a practical example, the tutorial shows how to handle routing and endpoint details in real-time, providing insights on when the endpoint is `null` and when it becomes valid after routing is executed.
Takeaways
- 😀 The `UseRouting` middleware in ASP.NET Core identifies the appropriate route based on the incoming URL and HTTP method.
- 😀 The compiled code already contains information about all defined endpoints before the routing middleware executes.
- 😀 The routing middleware matches the incoming request with available endpoints based on the URL and HTTP method.
- 😀 The `Endpoint` object is created after the `UseRouting` middleware executes and stores information about the selected endpoint.
- 😀 Before the `UseRouting` middleware executes, the `Endpoint` object is not created and will return `null` if accessed.
- 😀 You can access the `Endpoint` object using `GetEndpoint` method after the routing middleware has executed.
- 😀 Middleware can be added before or after the `UseRouting` middleware to examine the request or access the endpoint object.
- 😀 If the `GetEndpoint` method is called before `UseRouting`, it will return `null` because the endpoint has not been created yet.
- 😀 The example demonstrates adding middleware to log or display the endpoint selected for an incoming request.
- 😀 The `Endpoint` object contains properties such as `DisplayName`, which shows the URL and HTTP method of the matched route.
- 😀 Understanding when and how the `Endpoint` object is created helps in logging and debugging route matching in ASP.NET Core.
Q & A
What is the role of the `UseRouting()` middleware in ASP.NET Core?
-The `UseRouting()` middleware is responsible for identifying the appropriate route based on the incoming request's URL and HTTP method. It matches the request with the defined routes and selects the correct endpoint to handle the request.
When does the endpoint object get created in the request pipeline?
-The endpoint object is created after the `UseRouting()` middleware executes, as it relies on the information gathered by the middleware to identify which endpoint should handle the incoming request.
Why does the endpoint object return `null` if accessed before `UseRouting()`?
-Before the `UseRouting()` middleware executes, ASP.NET Core is unaware of the defined routes and endpoints. Therefore, accessing the endpoint object at that time returns `null` since the middleware hasn't yet mapped the incoming request to any endpoint.
Can you access the endpoint object before `UseRouting()` in the middleware pipeline?
-No, you cannot access the endpoint object before `UseRouting()` because it is only created after the middleware has executed and matched the incoming request to a defined route.
How can you check the endpoint after `UseRouting()` is executed?
-You can check the endpoint after `UseRouting()` by using the `GetEndpoint()` method, which will return the endpoint object associated with the matched route, including details like the display name and HTTP method.
What happens if there is no route defined for a URL, such as the root URL?
-If there is no route defined for a URL, like the root URL, the `endpoint` will be `null`. A response indicating that the page was not found will be returned.
What is the significance of the `displayName` property in the endpoint object?
-The `displayName` property in the endpoint object provides information about the URL path and the HTTP method associated with the endpoint. This is useful for logging or debugging purposes to track which route is being matched.
What does the `requestDelegate` property in the endpoint object represent?
-The `requestDelegate` property points to the middleware function that should be executed for the given endpoint. It essentially defines what should happen when the endpoint is triggered for a specific route.
What happens if you try to access the endpoint object for a non-existent route, like the root URL, after `UseRouting()`?
-If you try to access the endpoint object for a non-existent route after `UseRouting()` executes, the `endpoint` will still be `null` because no endpoint is defined for that route. ASP.NET Core will not find a match and return a 'not found' response.
Why might you use the `GetEndpoint()` method in a real-world application?
-The `GetEndpoint()` method can be useful for scenarios such as logging or tracking which endpoints are being matched for specific requests. It can help developers understand the flow of requests through the application and debug routing issues.
Outlines
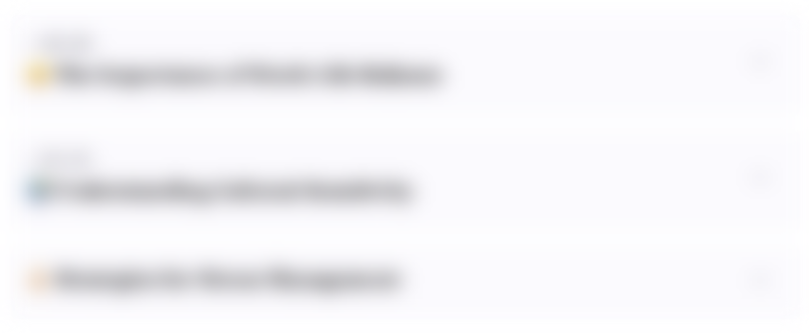
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
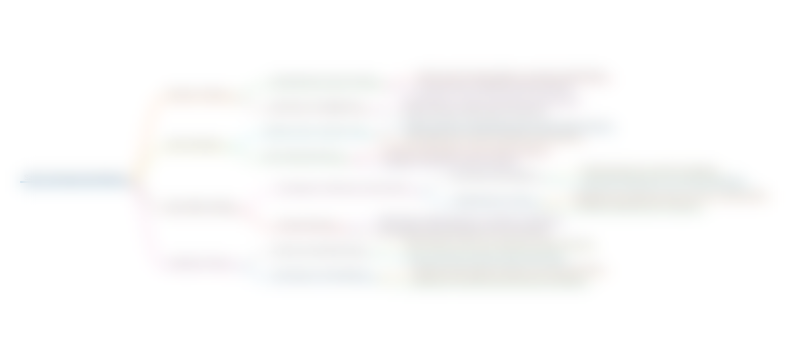
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
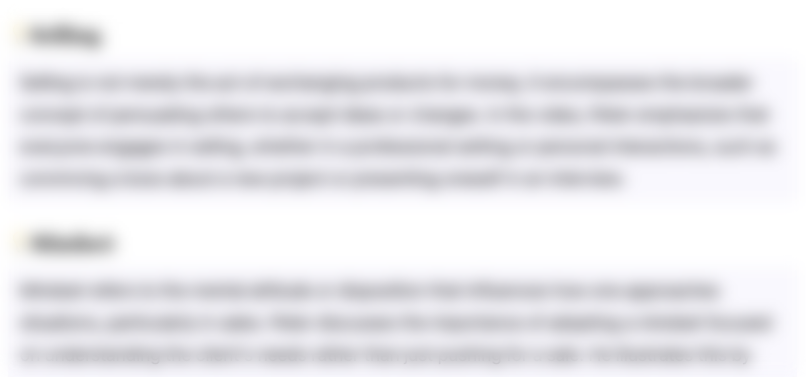
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
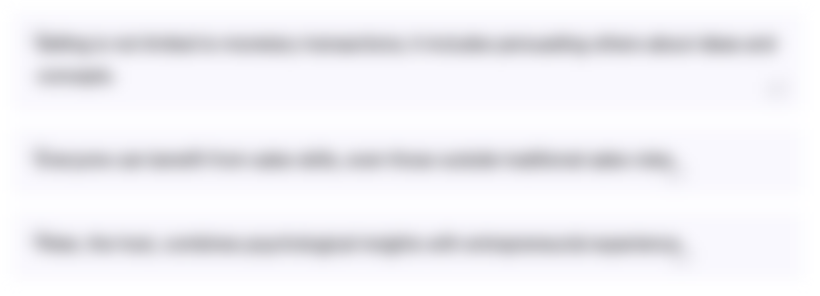
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
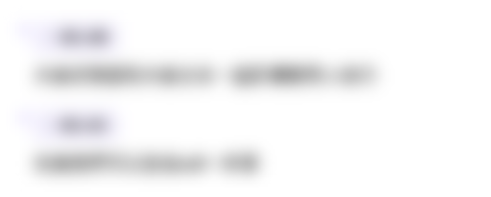
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
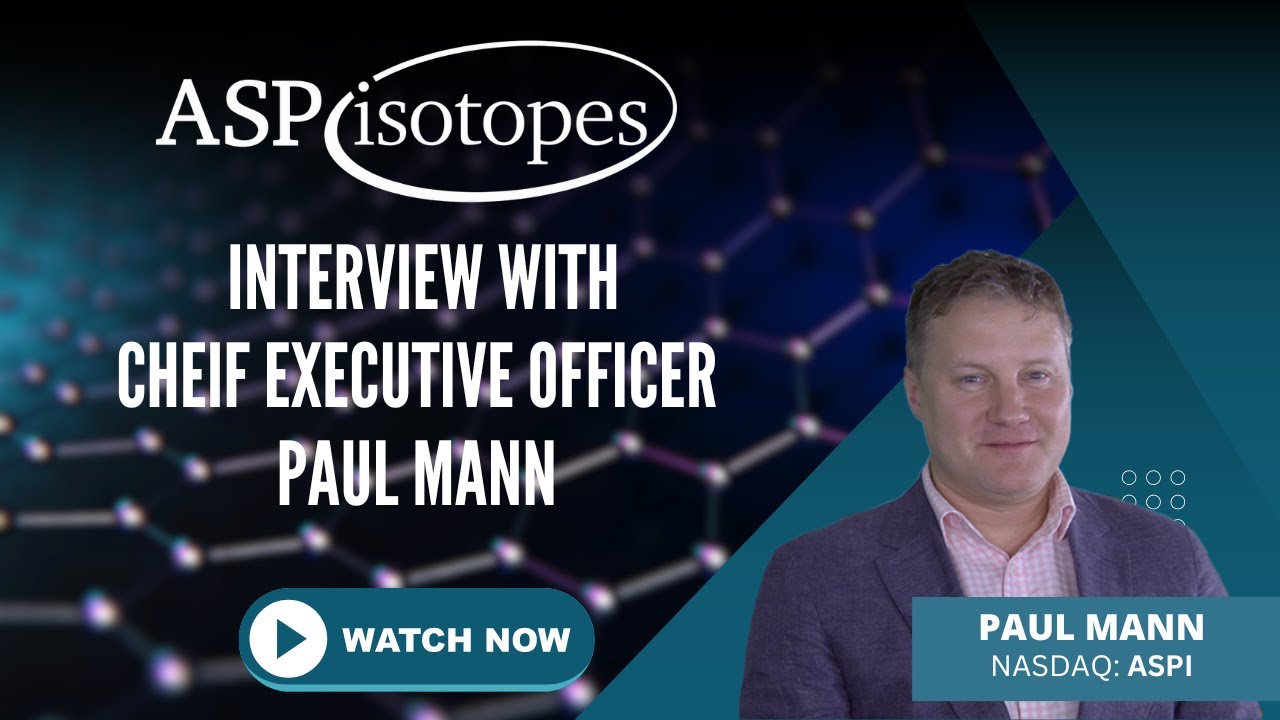
How ASP Isotopes is Revolutionizing the Global Isotope Supply Chain
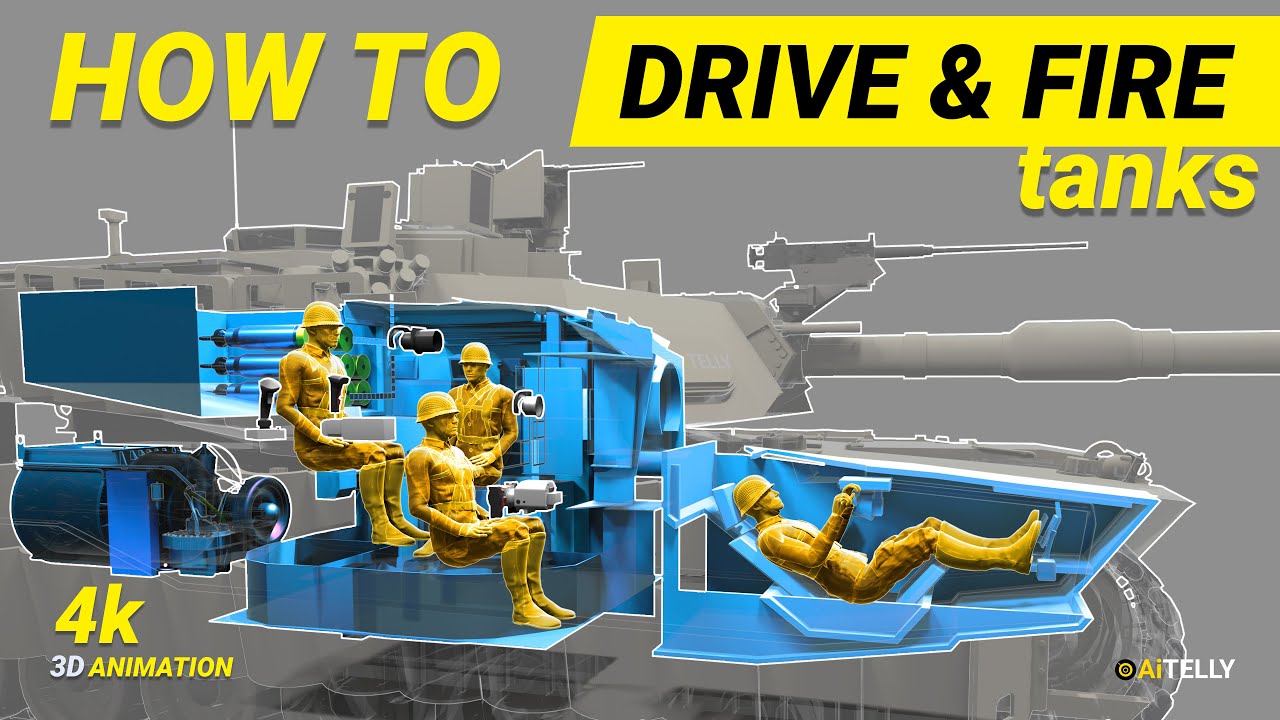
How to Drive and Fire Tanks | How it Works Abrams M1A2 M1A2C Tanks
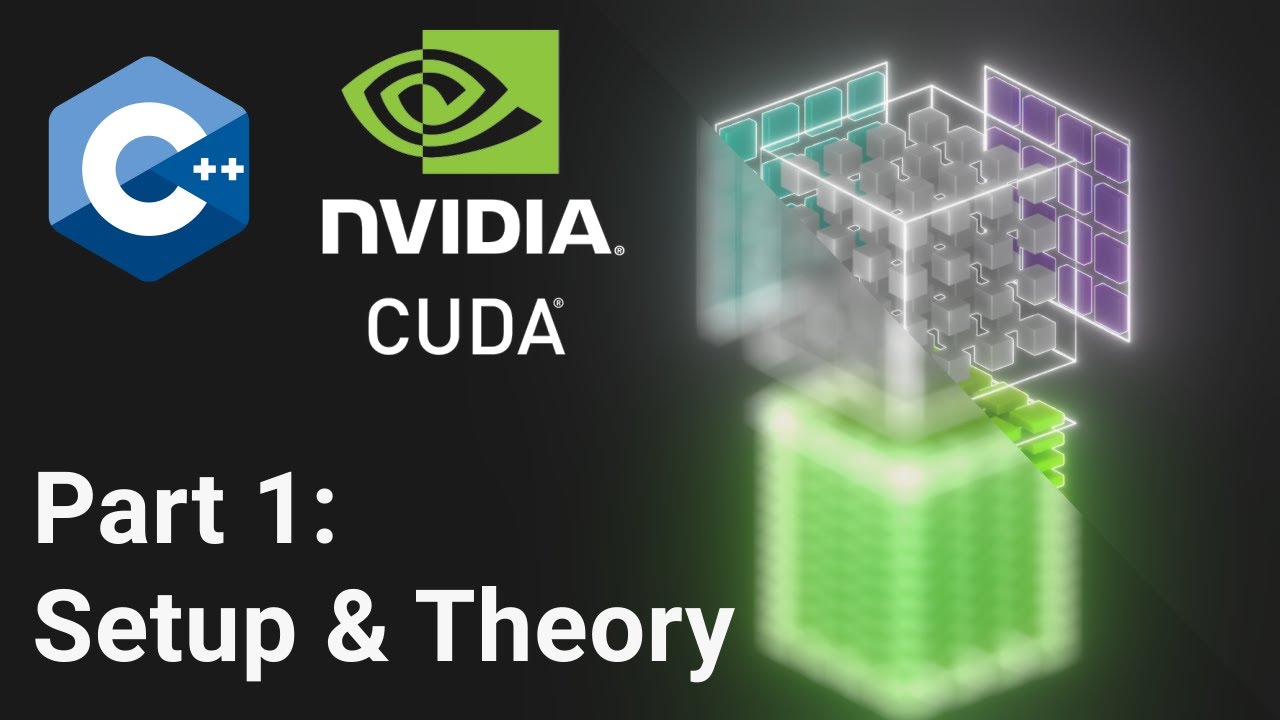
C++ CUDA Tutorial: Theory & Setup

EDB1 IMD UFRN (2020.6): Ordenação por Seleção e Inserção (2/2)
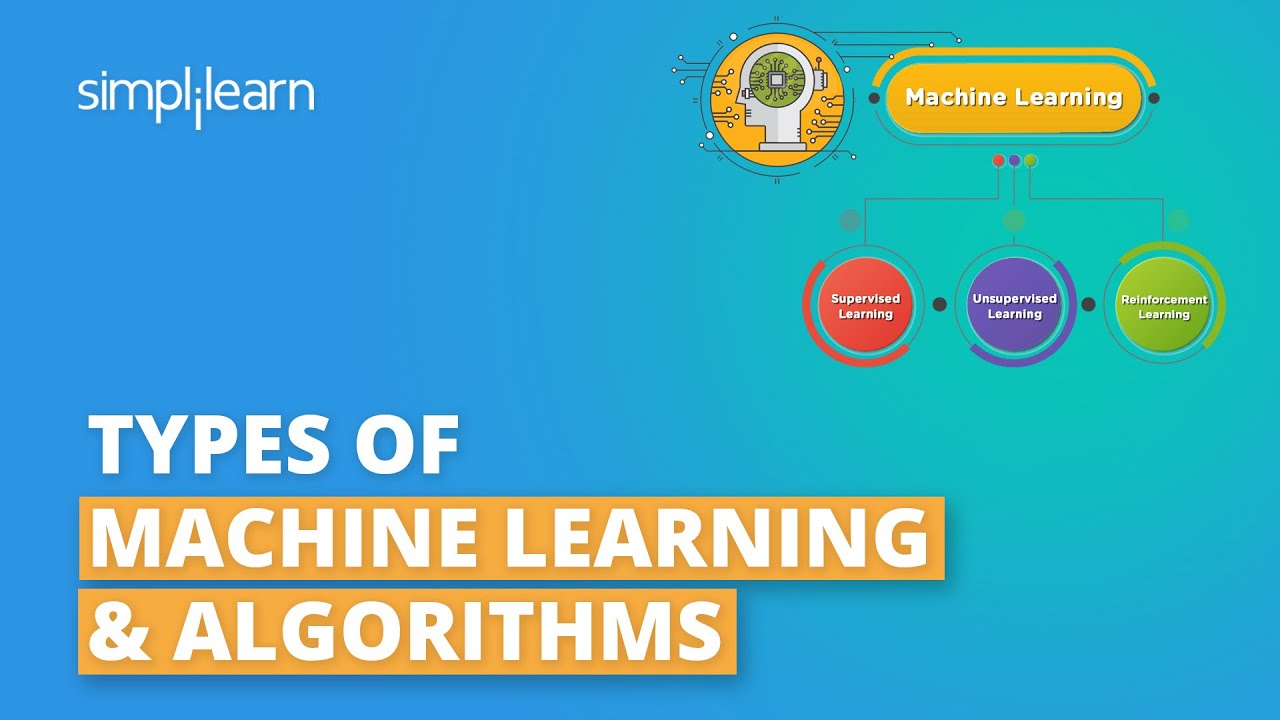
Types Of Machine Learning | Machine Learning Algorithms | Machine Learning Tutorial | Simplilearn
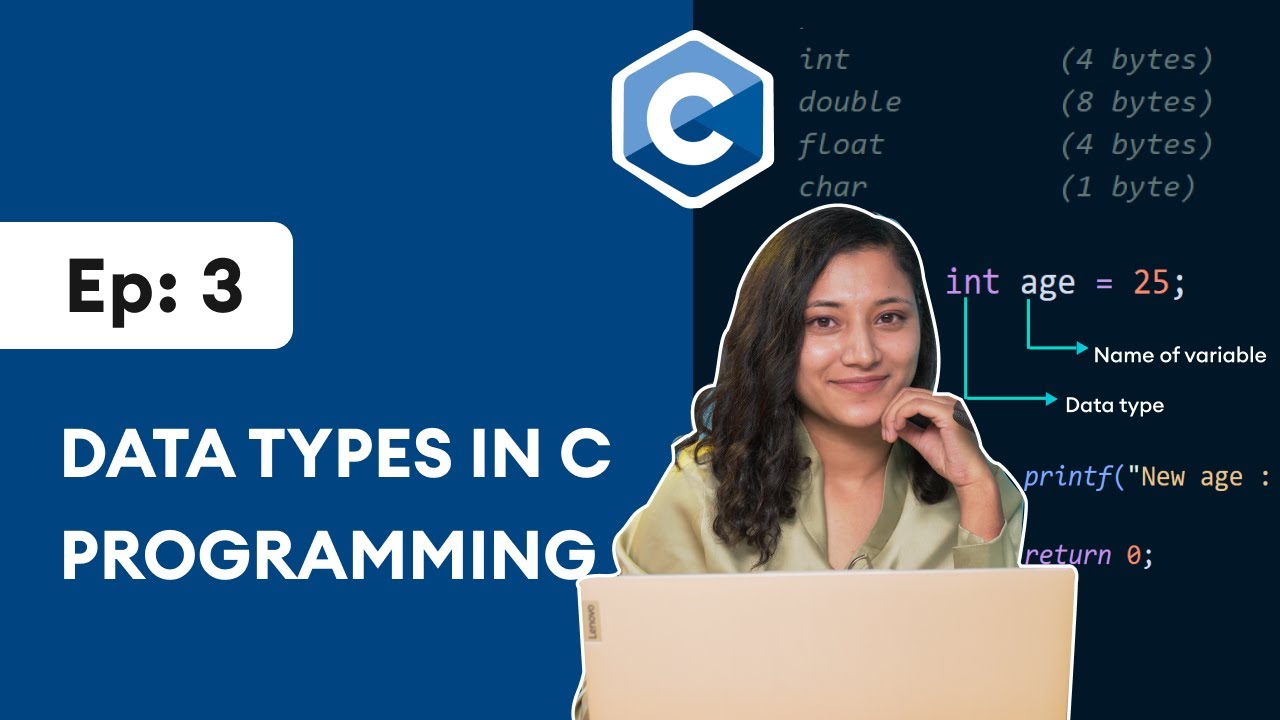
#3: Data Types in C Programming | C Programming for Beginners
5.0 / 5 (0 votes)