UE5 - Inventory System: Actor Component (5)
Summary
TLDRThis tutorial guides you through building an inventory system in Unreal Engine using Blueprints. It covers creating an `AC_Inventory` component to manage items, storing them in a map indexed by name, and updating inventory with a `Give` function. The function checks if the item exists, stacks quantities, or adds the item if itโs new. It also demonstrates how to integrate the inventory system into the character Blueprint and test it by displaying item details. By the end, users will be able to manage and display an inventory in their game with ease.
Takeaways
- ๐ Create an inventory system by adding an inventory component as an actor component in Unreal Engine Blueprints.
- ๐ Use a `Map` to store inventory items, indexed by their name, with the item details stored in a custom `St_InventoryItem` structure.
- ๐ Implement a `Give` function to allow the player to pick up items, which takes inputs like item ID, quantity, and whether the item was dropped.
- ๐ Set up a default quantity value of `-1` to make it optional when calling the `Give` function for item pickup.
- ๐ Ensure the item exists in the inventory data table before adding it to the player's inventory. If not, the function should exit early.
- ๐ Use a sequence node to manage multiple steps like checking the itemโs validity, updating its quantity, and adding it to the playerโs inventory.
- ๐ Implement logic to stack items in the inventory by checking if the player already has the item, then adjusting the quantity.
- ๐ Add items to the player's inventory by using the `Add` function on the `Items` map, either updating an existing item or adding a new one.
- ๐ Attach the `AC_Inventory` component to the player character so the character can interact with the inventory system.
- ๐ Test the inventory system by adding a simple UI feature to list the items the player has collected by pressing a designated key (e.g., `P`).
- ๐ Use a `For Each` loop to display the collected items' names and quantities, using formatted text to print the information to the screen.
Q & A
What is the main purpose of the `AC_Inventory` component in this tutorial?
-The main purpose of the `AC_Inventory` component is to store and manage inventory items in Unreal Engine. It allows the player to receive items and update their inventory, which is stored in a map indexed by item names.
Why is the `items` variable created as a map, and what does it store?
-The `items` variable is created as a map because it stores inventory items in a way that each item is indexed by its name. The map allows for easy lookups, additions, and updates of items based on their names, mimicking a structure similar to data tables.
What is the significance of the `give` function in this inventory system?
-The `give` function allows items to be added to the player's inventory. It takes the item ID (name), quantity, and whether the item was dropped by the player as inputs, then updates or adds the item to the inventory accordingly.
How does the system ensure that an item is only added if it doesn't already exist in the player's inventory?
-The system checks if the item exists in the inventory by using the `find` function to search for the item ID. If the item is found, it updates the quantity; if not, it adds the item to the inventory.
What role does the `quantity` variable play in the `give` function?
-The `quantity` variable specifies how many of an item the player will receive. If the quantity is not provided (i.e., it's set to -1), the system assumes the default quantity from the inventory table, but if it's specified, it updates the quantity of the item accordingly.
How does the system handle the case where the player drops an item?
-If an item is dropped by the player, the `drop item` input is used to provide the item data. If the drop item is valid, the system will use the dropped item's data; otherwise, it will use the default data from the inventory table.
Why is the `branch` node used when checking the `quantity` in the `give` function?
-The `branch` node is used to check if the `quantity` is different from -1. If it is, the system will update the item's quantity. Otherwise, it leaves the quantity as is, based on the default value from the inventory table.
What happens when the player already has an item in their inventory and attempts to pick up the same item again?
-If the player already has the item, the system will add to the existing quantity instead of adding a new item. This ensures that items are stacked in the inventory instead of being duplicated.
How does the `get items` function help in displaying the player's inventory?
-The `get items` function retrieves the list of items in the player's inventory. It is used in conjunction with the `for each` loop to iterate over all the items and display their names and quantities on the screen.
How can you modify the system to allow the player to specify a different quantity when picking up an item, instead of using the default quantity from the inventory table?
-To allow the player to specify a different quantity, you can modify the `give` function to accept a quantity input from the player. This quantity would then be passed when the item is received, allowing for customized item pickup amounts.
Outlines
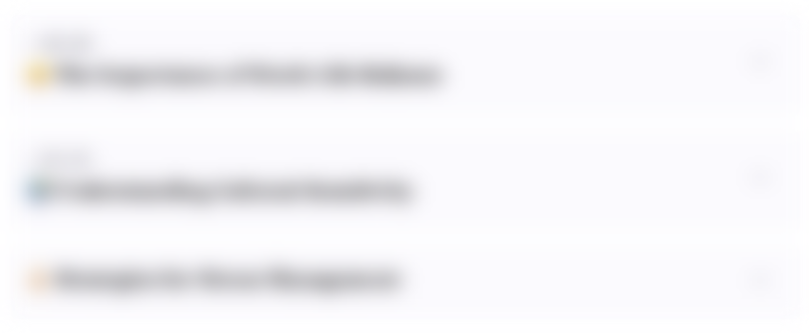
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
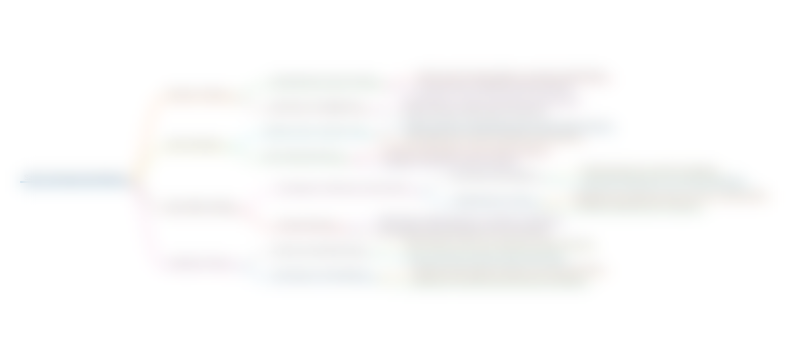
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
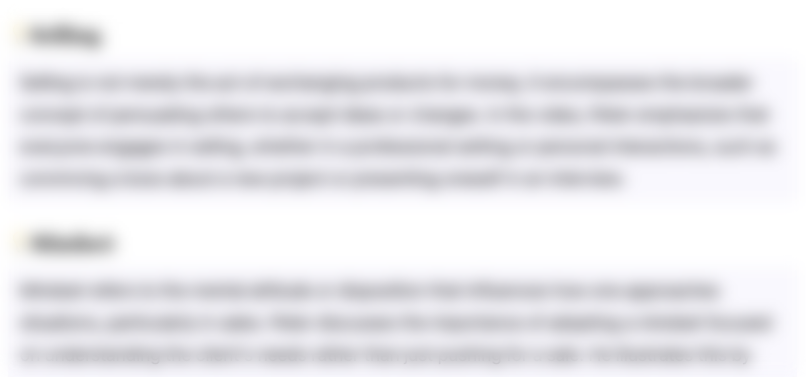
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
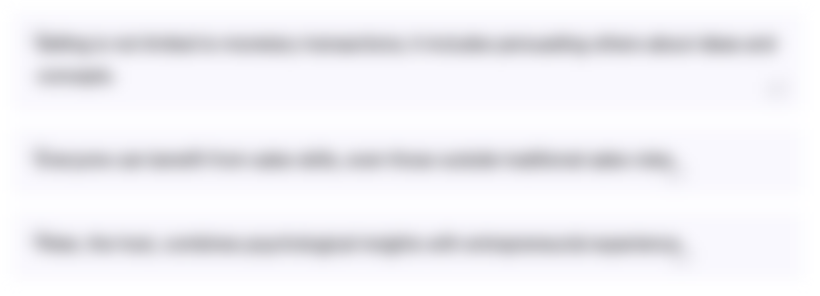
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
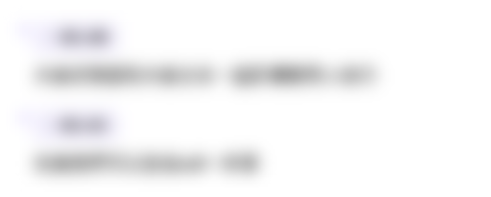
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
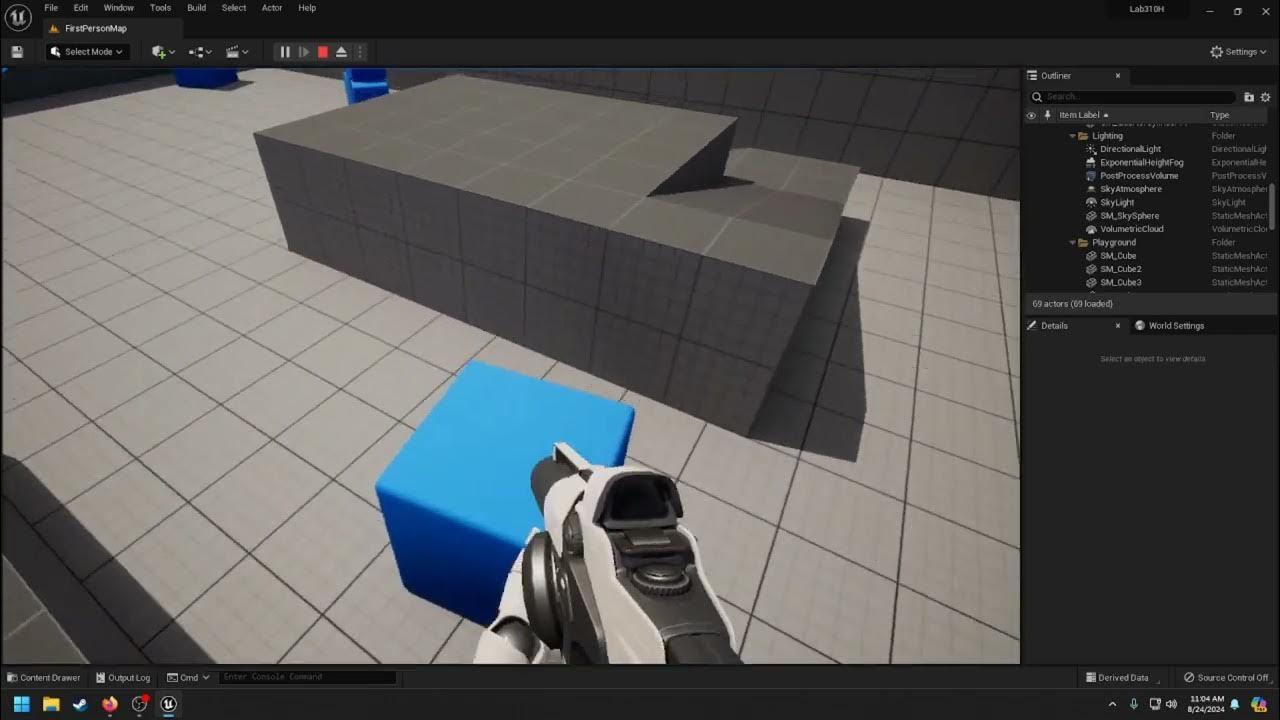
IDEA/CS 310H - Video 1
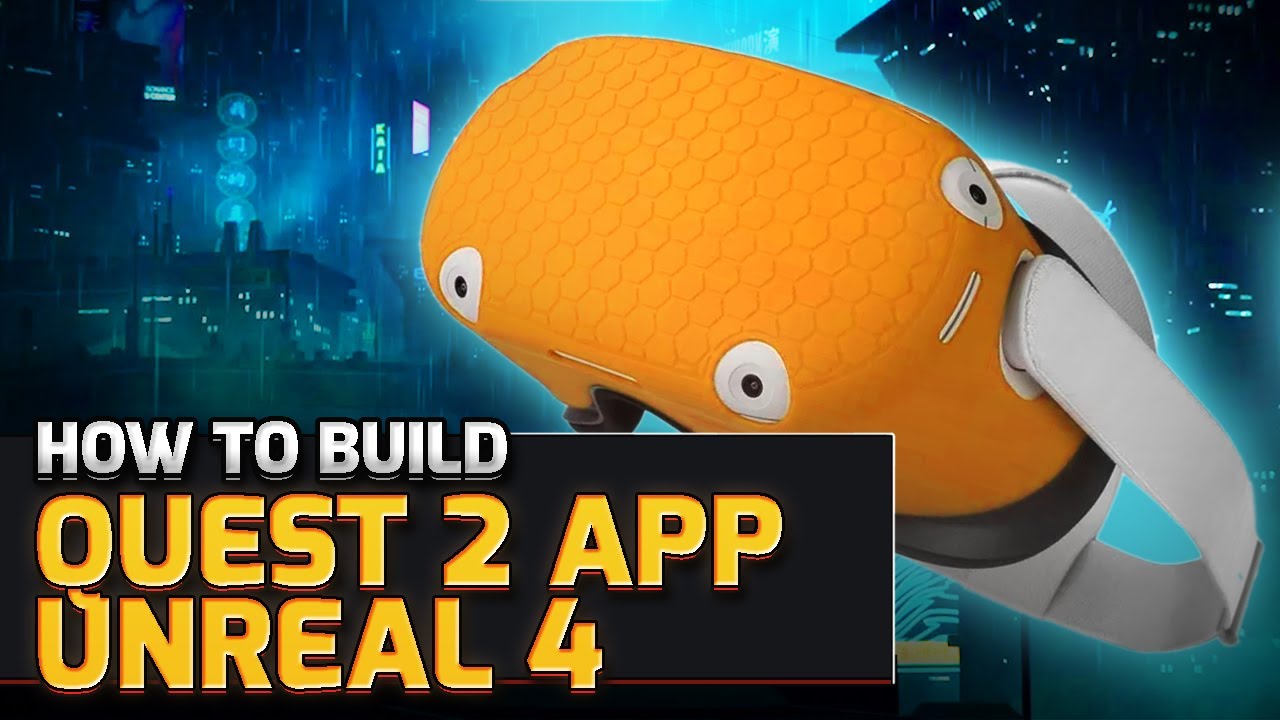
How to Build Quest 2 VR Apps with Unreal Engine 4.27 | VR Development
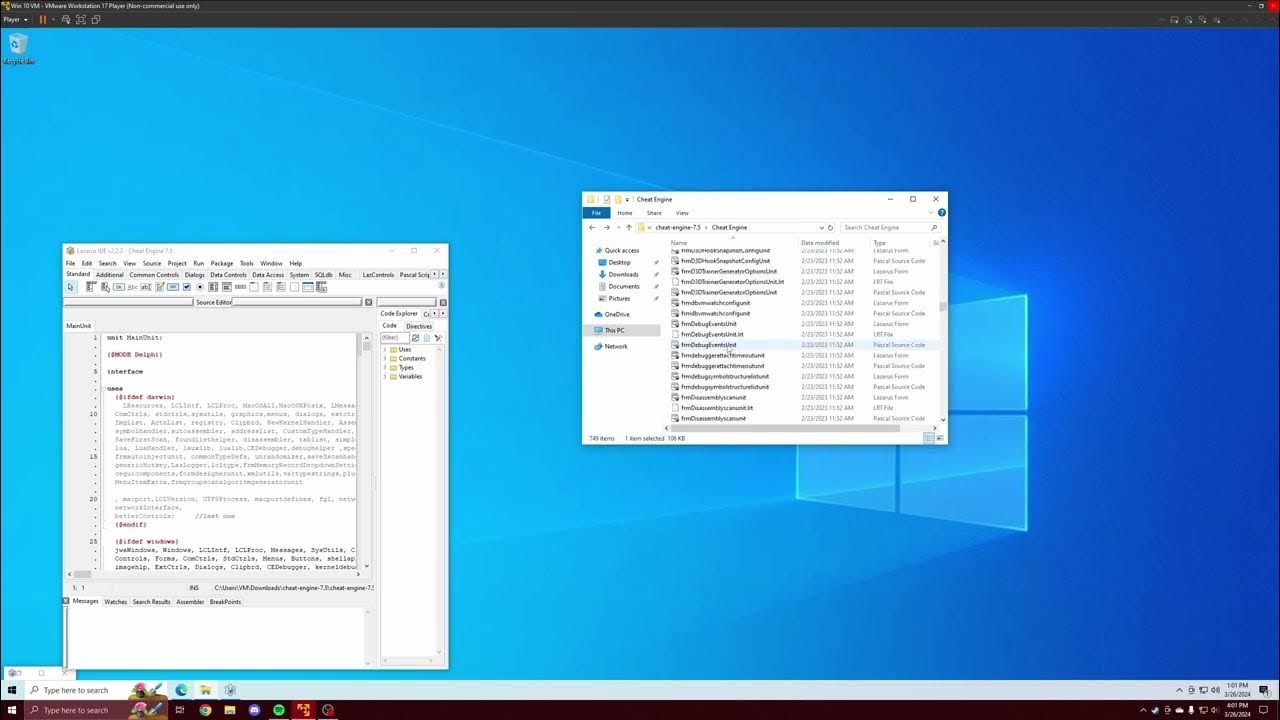
Cheat Engine Built From Source Tutorial | Driver | DBVM | VEH Debugger | Renaming | Error Correction
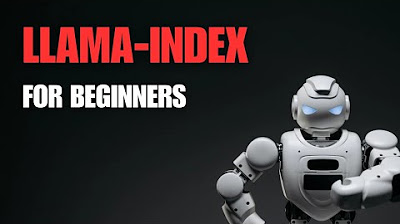
Llama-index for beginners tutorial
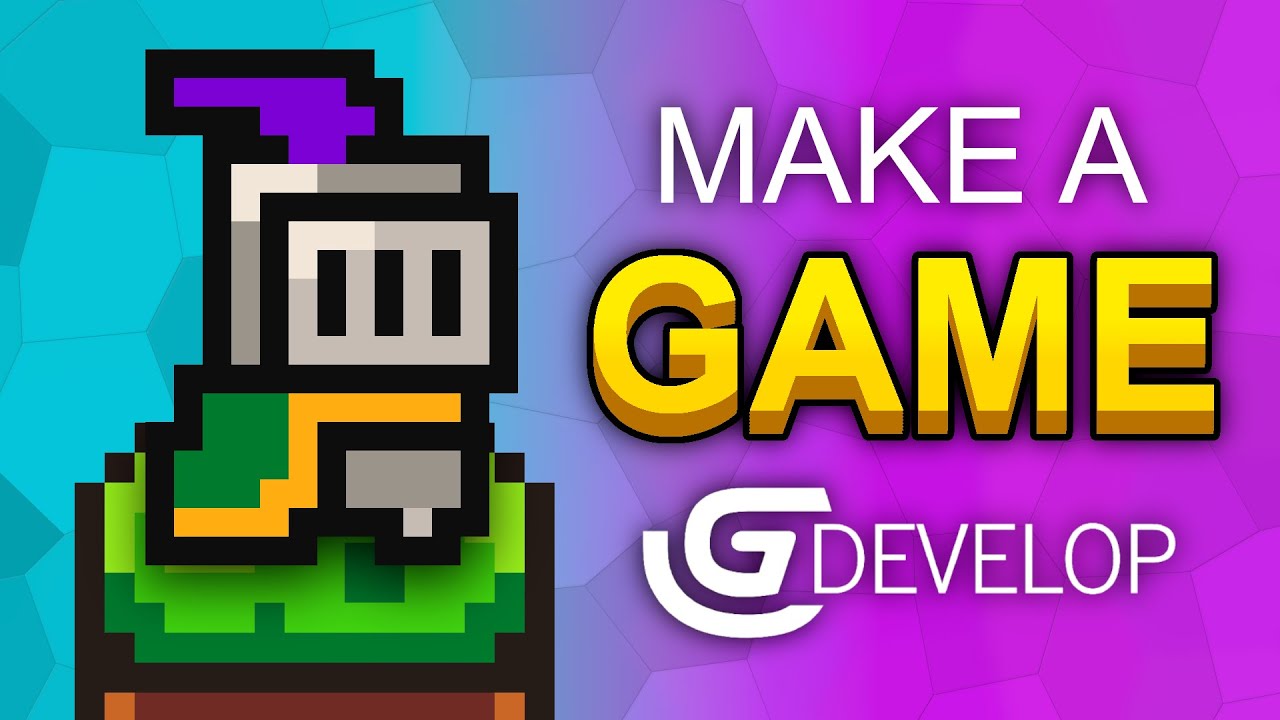
How To Make A Video Game - GDevelop Beginner Tutorial
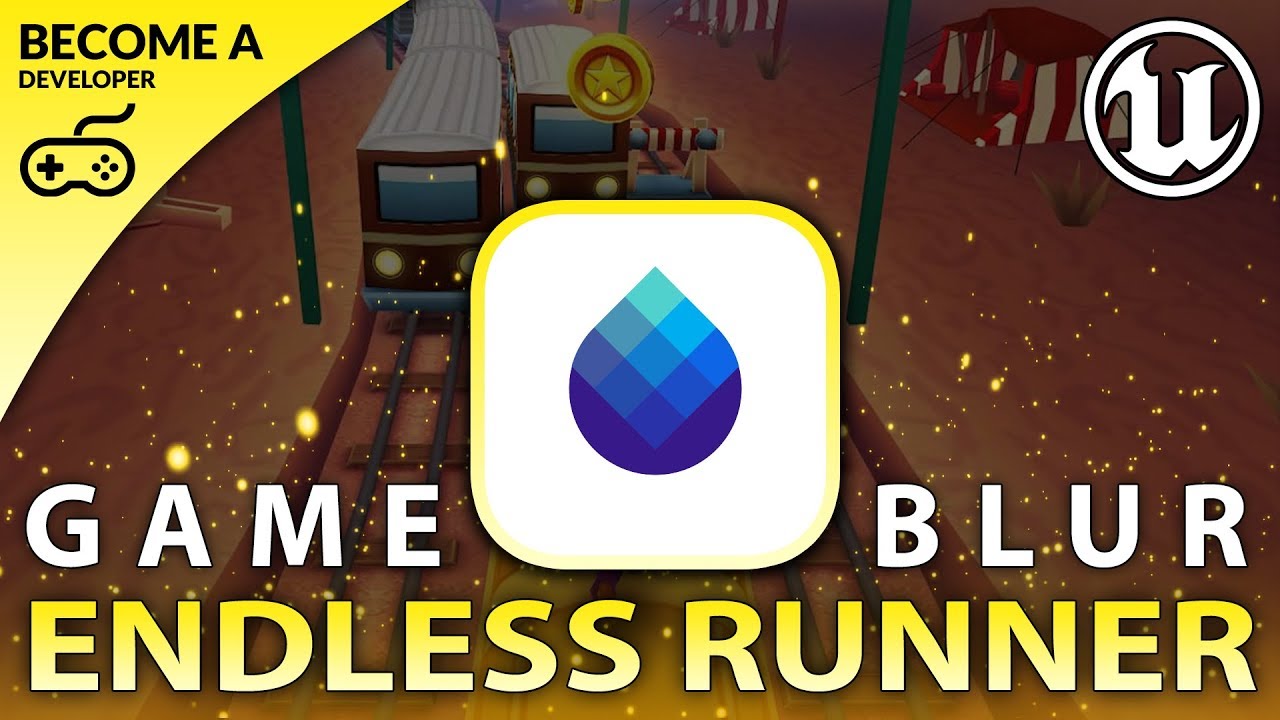
Pause & Endgame Blur - #15 Creating A MOBILE Endless Runner Unreal Engine 4
5.0 / 5 (0 votes)