Object-Oriented Programming Illustrated
Summary
TLDRIn this video, Alex explains the fundamentals of Object-Oriented Programming (OOP), breaking down key concepts like classes, inheritance, encapsulation, and polymorphism. He uses relatable examples, such as the 'Cat' class, to illustrate how classes define attributes and behaviors for objects. Alex also discusses the role of constructors in initializing object properties, and how inheritance enables new classes to share traits from existing ones. The video further explores access modifiers (private, protected, public) and concludes with a discussion of polymorphism, demonstrating how methods can be overridden in subclasses. A helpful guide for those looking to understand OOP in simple terms.
Takeaways
- ๐ A **class** in object-oriented programming (OOP) is a blueprint for creating objects with shared attributes and behaviors.
- ๐ **Instance variables** are unique attributes of each object created from a class, such as fur color or age in the Cat class.
- ๐ A class can define **behaviors** (methods), like running or sleeping, which all instances of the class share.
- ๐ **Initialization (constructors)** in OOP sets the initial values of instance variables when an object is created.
- ๐ **Inheritance** allows new classes (subclasses) to inherit traits and behaviors from an existing class (superclass), forming a hierarchy.
- ๐ **Encapsulation** in OOP hides certain details of a class through access modifiers like **private**, **protected**, and **public**.
- ๐ **Private** members of a class can only be accessed within the class itself, like private items inside your house.
- ๐ **Protected** members can be accessed by subclasses but not other classes, similar to how your children can access certain private items in the house.
- ๐ **Public** members are accessible by all classes, like things outside your house that anyone can see or use.
- ๐ **Polymorphism** allows the same interface (method) to be used for different underlying implementations, enabling more flexible and dynamic behavior.
- ๐ The **constructor** in OOP works like the time before you're born, where your traits (like hair or eye color) are set and can change later.
Q & A
What is a class in object-oriented programming?
-A class is a blueprint or template for creating objects. It defines attributes (properties) and behaviors (methods) that objects created from the class will have.
Can you explain the concept of instance variables in a class?
-Instance variables are attributes that define the properties of an object. Each instance of a class will have its own values for these instance variables. For example, in the 'Cat' class, attributes like fur color, eye color, and age are instance variables.
What does it mean to create an object from a class?
-Creating an object from a class means instantiating a new entity based on the template defined by the class. Each object will have specific values for its instance variables, but share the same behaviors and structure as the class.
How are behaviors defined in a class, and can you provide an example?
-Behaviors in a class are defined through methods or functions that objects of that class can perform. For example, in the 'Cat' class, behaviors like running, climbing, and sleeping can be defined as methods.
What is the purpose of a constructor in a class?
-A constructor (or init method) is automatically invoked when an object is created. It is used to initialize the instance variables with values, similar to how parents might pass traits to a child before they are born.
What is inheritance in object-oriented programming?
-Inheritance allows a new class to take on attributes and behaviors from an existing class. The new class is called a subclass, and the existing class is the superclass. This allows for code reuse and hierarchical class structures.
How does inheritance work with an example?
-For instance, a 'Mammal' class can inherit from an 'Animal' class. Both 'Mammal' and 'Bird' could inherit common properties like the number of legs and eyes, but 'Mammal' might also have additional properties like fur.
What is encapsulation, and how does it relate to access modifiers like private, protected, and public?
-Encapsulation is the concept of hiding internal details of a class from other classes. Access modifiers control this accessibility. Private variables are only accessible within the class, protected variables can be accessed by subclasses, and public variables are accessible by everyone.
What is polymorphism in object-oriented programming?
-Polymorphism is the ability to present the same interface for different underlying forms. This allows subclasses to override methods from a superclass, giving them different behaviors while using the same method name.
How does polymorphism work when a method is overridden in a subclass?
-If a method is overridden in a subclass, the subclassโs version of the method is called. If the subclass does not override the method, the superclassโs version is called by default.
Outlines
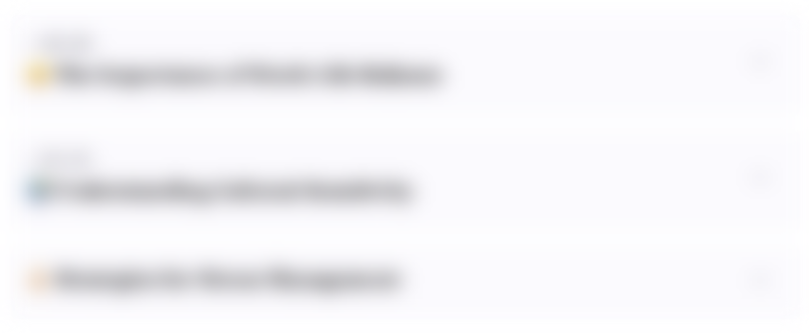
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
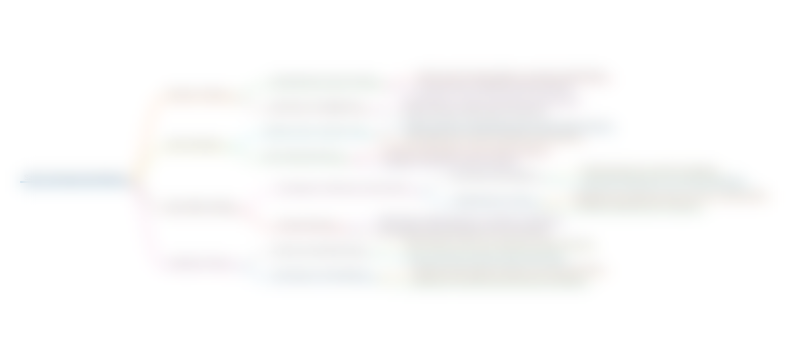
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
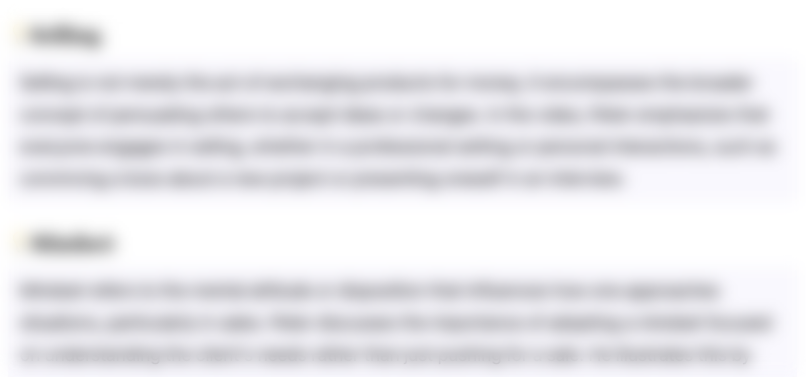
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
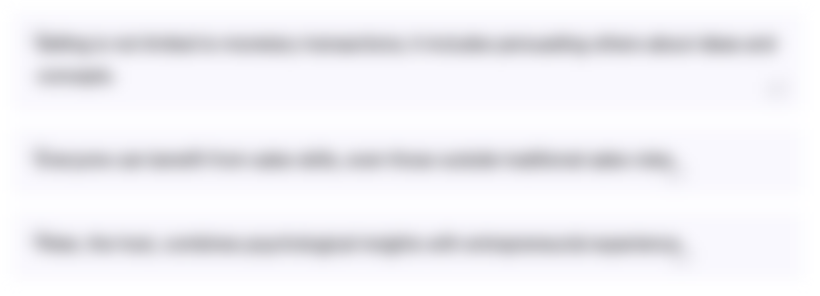
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
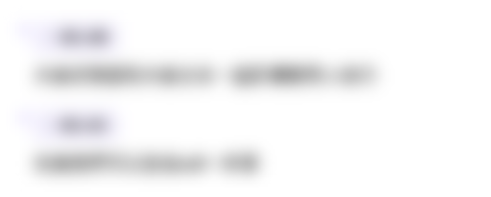
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Overview of Object-oriented programming in C# | C# object-oriented programming | C# oops
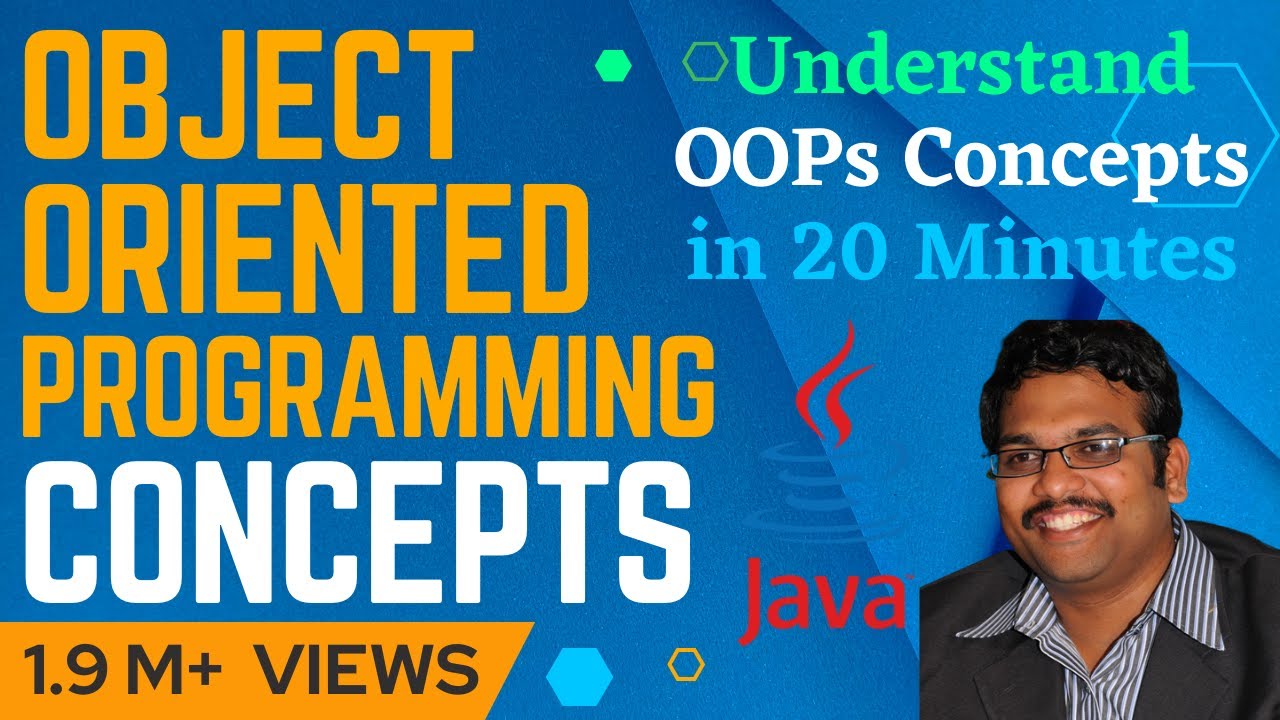
OOPS CONCEPTS - JAVA PROGRAMMING

Fundamental Concepts of Object Oriented Programming

Introduction to OOPs in Python | Python Tutorial - Day #56
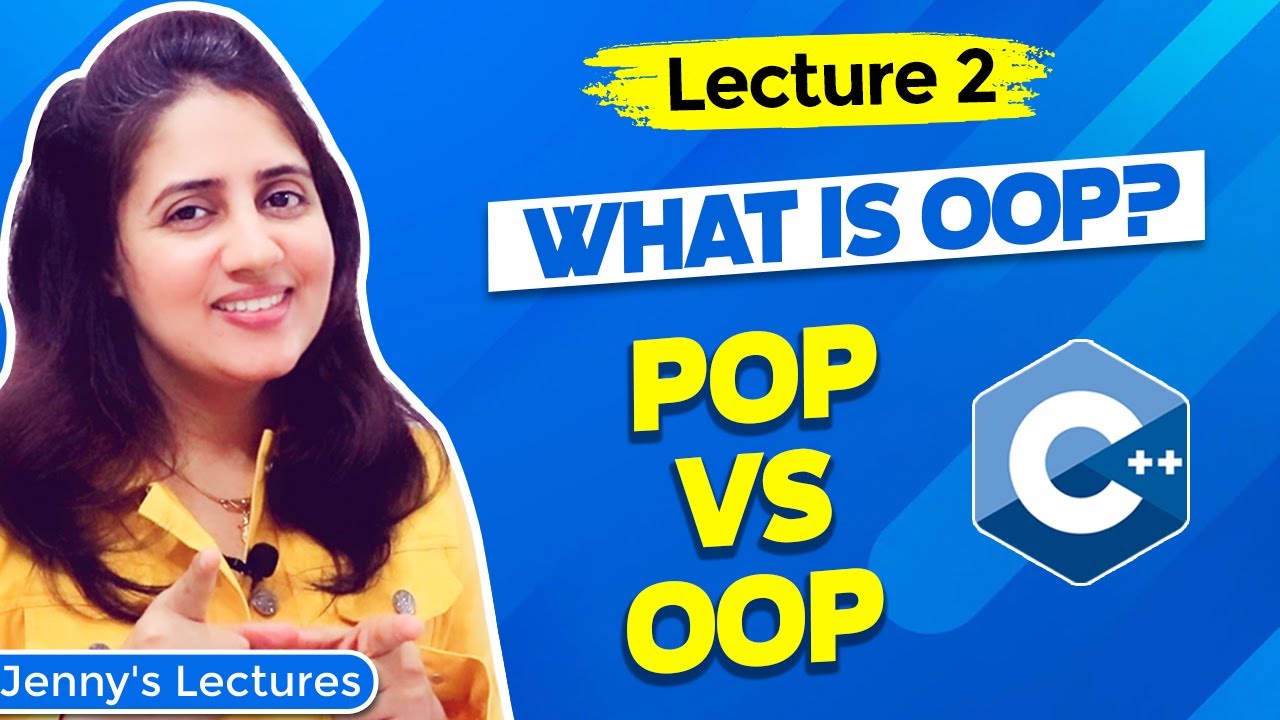
Lec 2: What is Object Oriented Programming (OOP) | POP vs OOP | C++ Tutorials for Beginners
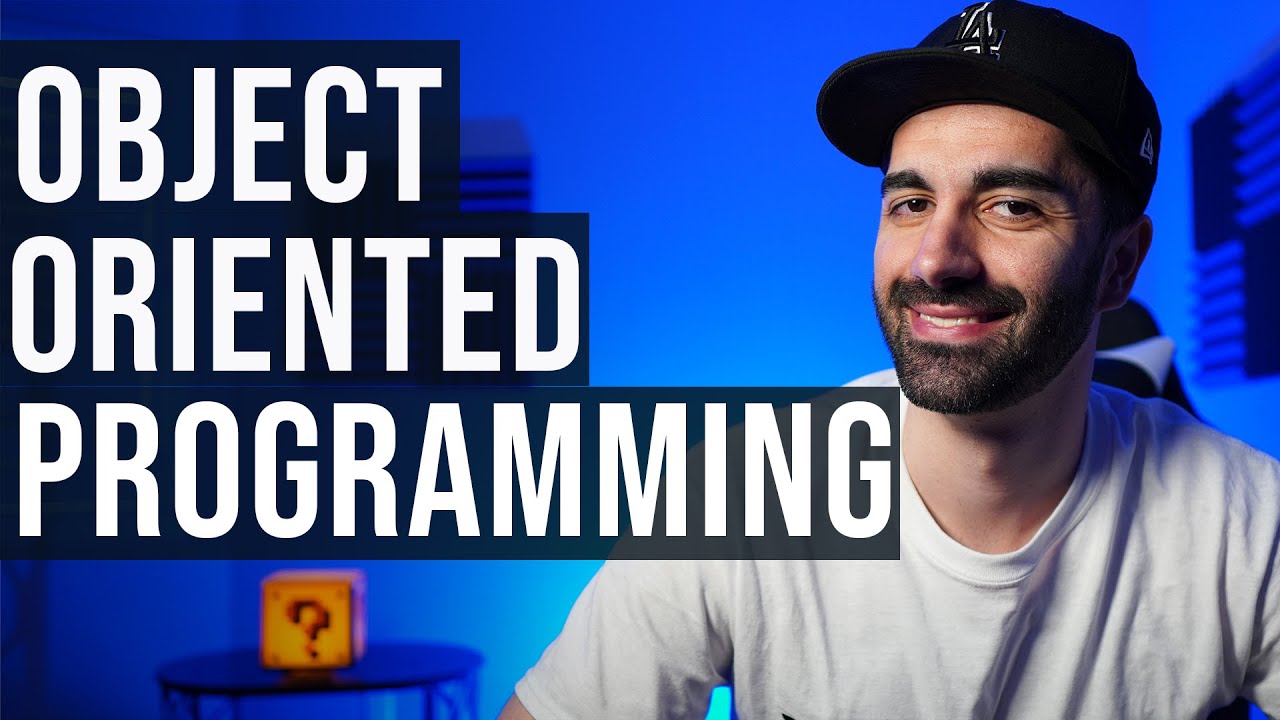
Object Oriented Programming - The Four Pillars of OOP
5.0 / 5 (0 votes)