Static and Dynamic binding in C++ with examples (Early and Late binding)
Summary
TLDRIn this informative video, the creator addresses a popular request from their audience by explaining the concepts of static and dynamic binding in C++. The video begins with an introduction to the topic, which was chosen through a community poll, and promises future engagement through similar voting. The script provides a clear definition of binding and distinguishes between static and dynamic binding, highlighting their respective advantages and disadvantages. Static binding, occurring at compile time, is noted for its efficiency, while dynamic binding, decided at runtime, offers flexibility. The creator demonstrates both concepts with practical examples, including function overloading for static binding and the use of virtual functions for dynamic binding. The video concludes with an invitation for viewers to participate in future topic selection and to provide feedback.
Takeaways
- 🎬 The video is about explaining static versus dynamic binding in C++ based on a poll result.
- 📊 The majority voted for the topic of static versus dynamic binding, which the video covers.
- 🔗 The presenter plans to conduct more polls for future video topics and encourages participation.
- 🔗 Links to previous videos and related topics are provided in the description for context.
- 📚 Static binding in C++ occurs at compile time, also known as early or compile-time binding.
- 📚 Dynamic binding happens at runtime and is also called late or runtime binding.
- ⚡ Static binding makes programs run faster because the function association is done at compile time.
- 🔄 Dynamic binding, while slower, offers flexibility by allowing function selection at runtime based on data.
- 📝 Static binding is achieved by default with normal function calls, function overloading, or operator overloading.
- 📝 Dynamic binding is achieved using virtual functions or function overriding in a derived class.
- 🤔 Function overloading (for static binding) should not be confused with function overriding (for dynamic binding).
- 💡 The video provides a code example demonstrating static binding with function overloading.
- 💻 A second code example illustrates dynamic binding using inheritance, virtual functions, and a list of user pointers.
- 📣 The presenter asks for a thumbs up and comments for future topic suggestions and mentions more polls for topic selection.
Q & A
What topic was voted for by the majority in the poll for the next video?
-The majority voted for a video on static versus dynamic binding in C++.
How can viewers participate in the decision-making for future video topics?
-Viewers can participate by voting in the polls, which are available both on the YouTube channel and the creator's Instagram account.
What does 'binding' refer to in the context of C++ functions?
-Binding in C++ refers to associating the call of a function with its definition.
What are the two types of binding in C++?
-The two types of binding in C++ are static binding and dynamic binding.
At what time is static binding resolved?
-Static binding is resolved at compile time.
What is another name for static binding in C++?
-Static binding is also known as compile time binding or early binding.
How does dynamic binding differ from static binding in terms of when it is resolved?
-Dynamic binding is resolved during runtime, as opposed to static binding which is resolved at compile time.
What is the main advantage of dynamic binding over static binding?
-The main advantage of dynamic binding is its flexibility, allowing decisions on which function definition to invoke to be made at runtime.
How is static binding achieved in C++?
-Static binding is achieved by default through normal function calls, function overloading, or operator overloading.
How is dynamic binding achieved in C++?
-Dynamic binding is achieved through the use of virtual functions or function overriding.
What is the difference between function overloading and function overriding?
-Function overloading is used with static binding and involves creating multiple functions with the same name but different parameters. Function overriding is used with dynamic binding and occurs when a function in a child class overrides a function in the parent class.
How does the video demonstrate static binding?
-The video demonstrates static binding by showing a function called 'sumNumbers' being called with different numbers of parameters, with the appropriate function being determined at compile time based on the parameters passed.
In the video, what is used to demonstrate dynamic binding?
-The video uses a base class called 'User' and a derived class called 'SuperUser', along with virtual functions, to demonstrate dynamic binding.
How does the video show that dynamic binding is decided at runtime?
-The video shows that dynamic binding is decided at runtime by creating a list of user pointers that includes both 'User' and 'SuperUser' objects, and then invoking a method called 'getPermissions', which is resolved differently for each object at runtime.
What does the video suggest for viewers who want to see their suggested topics covered in future videos?
-The video suggests that viewers should vote on both the YouTube and Instagram polls to increase the chances of their suggested topics being covered in future videos.
Outlines
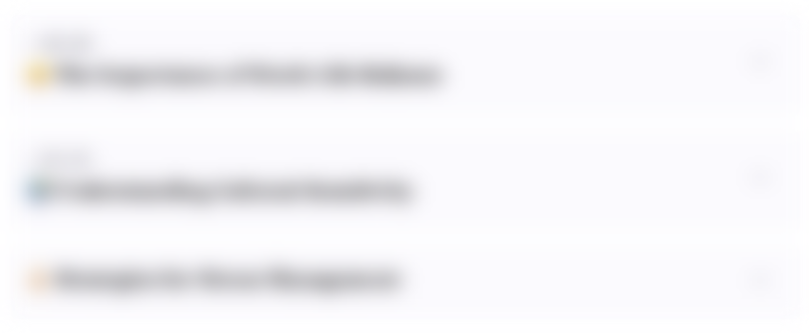
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
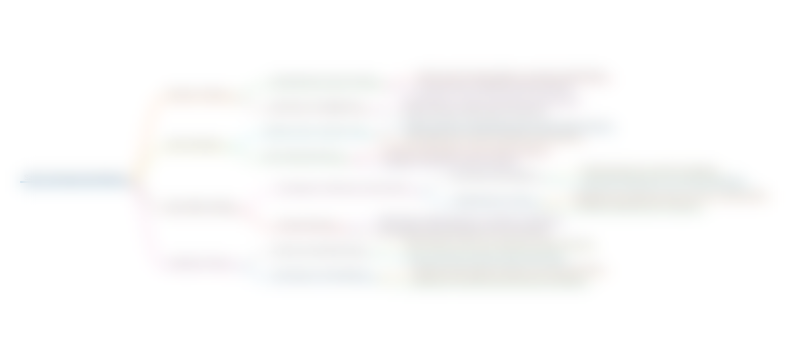
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
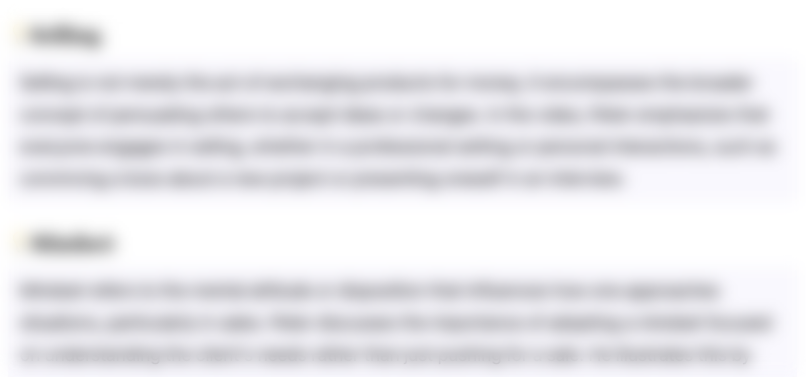
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
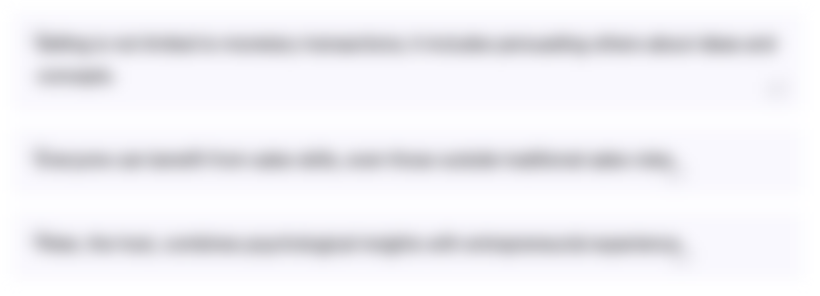
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
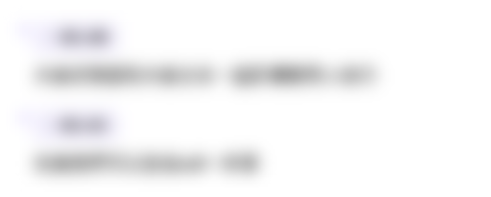
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
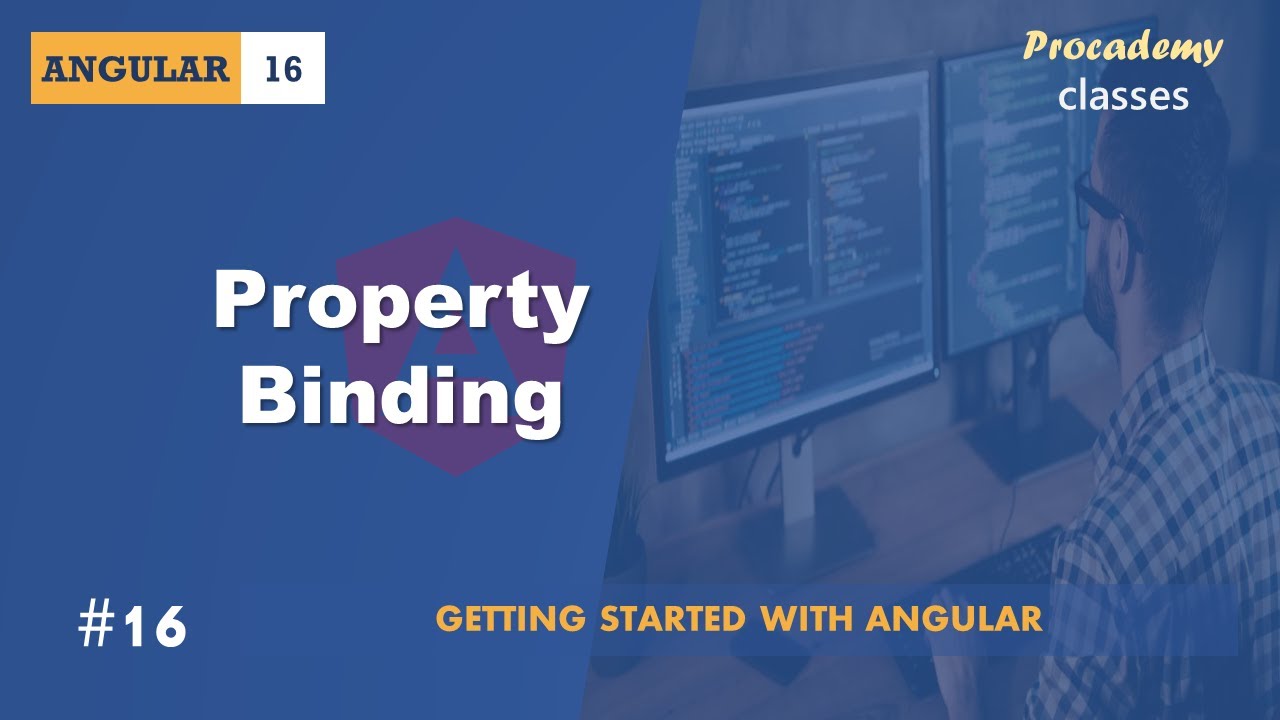
#16 Property Binding | Angular Components & Directives | A Complete Angular Course
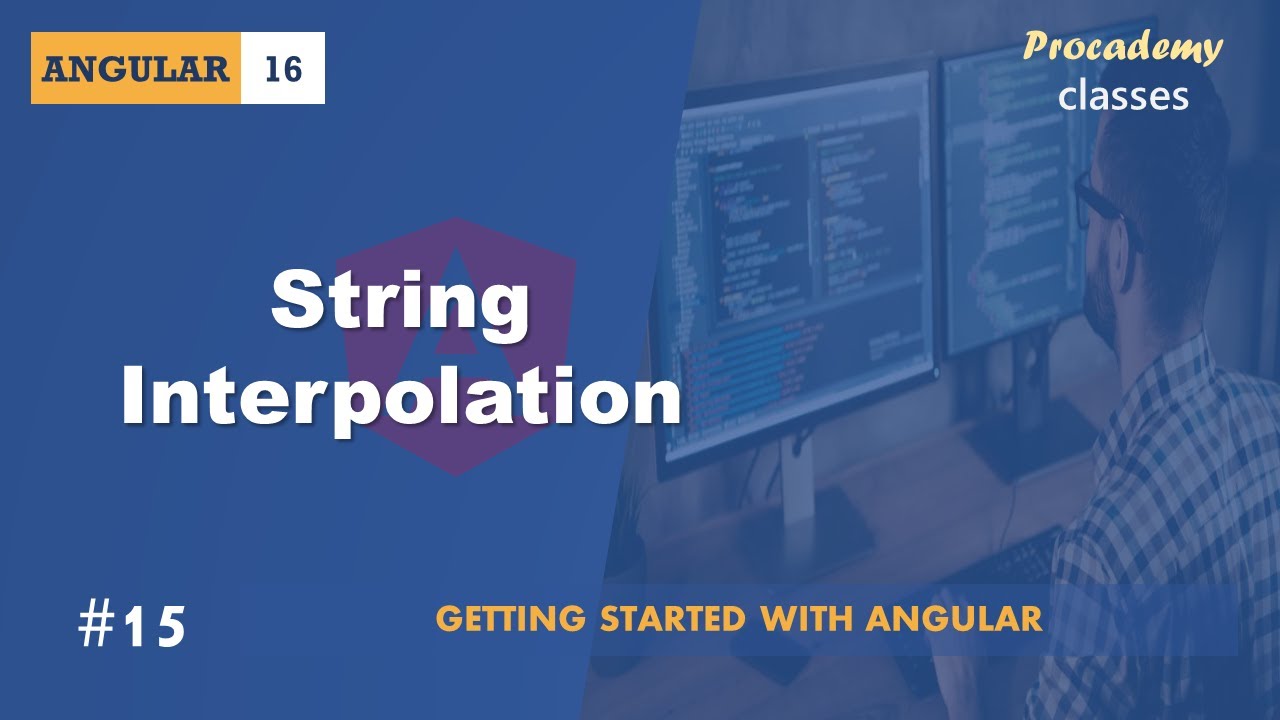
#15 String Interpolation | Angular Components & Directives | A Complete Angular Course

#18 Two way Data Binding | Angular Components & Directives | A Complete Angular Course
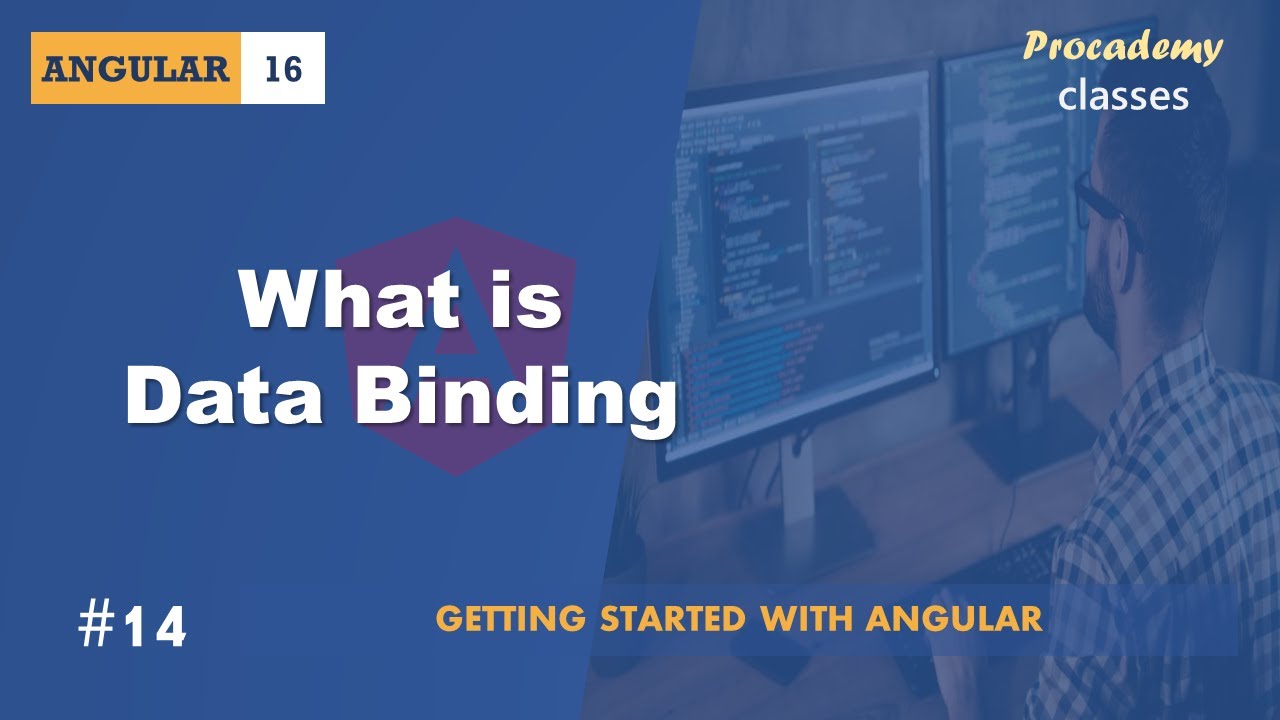
#14 What is Data Binding | Angular Components & Directives | A Complete Angular Course
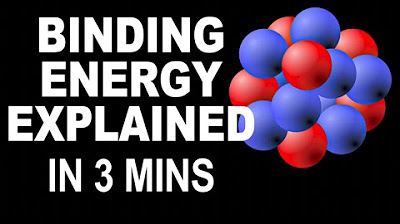
A 'cheatsheet' on Binding Energy in nuclear physics
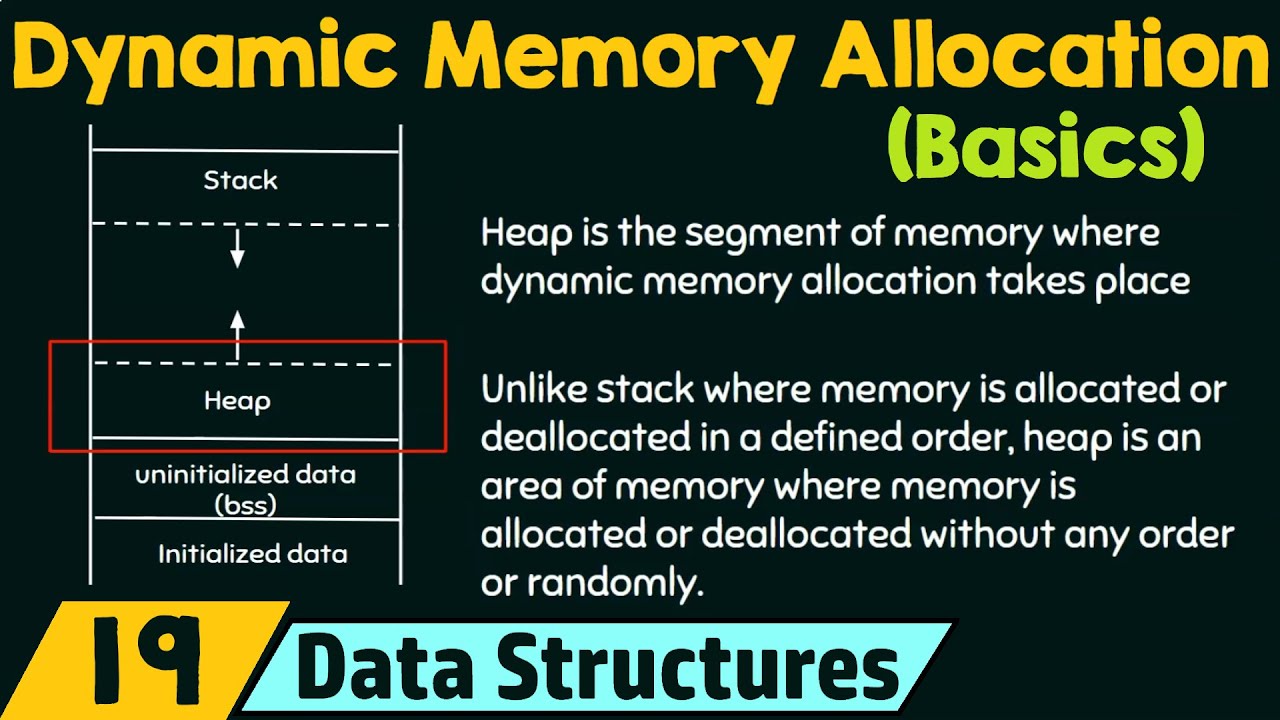
Basics of Dynamic Memory Allocation
5.0 / 5 (0 votes)