6.1 Functions in C++ | Guaranteed Placement Course
Summary
TLDRThis tutorial introduces the concept of functions in programming, focusing on their syntax, implementation, and execution. The instructor explains the importance of functions for code modularity and reusability, demonstrating with examples like calculating factorials and adding two numbers. Key topics include function structure, memory management with the call stack, and understanding how function calls affect the program’s execution. The video also uses analogies to clarify complex concepts, making it accessible for beginners to grasp how functions work and why they are essential in programming.
Takeaways
- 😀 Functions are essential in programming as they allow for code reuse, organization, and simplicity.
- 😀 A function's return type specifies the type of output it produces, such as `int`, `float`, etc.
- 😀 The function name is used to call the function in your code, making it accessible for execution.
- 😀 Parameters are inputs passed into the function to be processed by its code.
- 😀 The function body contains the logic or operations that define what the function does.
- 😀 A return statement in a function specifies the value the function will output after execution.
- 😀 When a function is called, a new memory block (stack frame) is created to hold local variables and parameters.
- 😀 Once a function completes its task, its memory stack is removed, returning control to the calling function.
- 😀 The main function is typically the entry point of the program, but other functions can be called from within it.
- 😀 Functions can call other functions, including themselves, which is useful for structuring complex operations.
- 😀 The stack memory model works like a stack of books—functions are added (pushed) and removed (popped) as they execute, ensuring efficient memory use.
Q & A
What is the role of functions in programming?
-Functions in programming help to break down complex problems into smaller, manageable tasks. They allow code to be modular, reusable, and more organized, which improves readability and ease of debugging.
What are the key components of a function?
-A function consists of the following key components: return type (the type of value the function will return), function name (the function's identifier), parameters (the inputs the function takes), and the function body (the block of code that performs the task).
What is meant by the 'return type' of a function?
-The 'return type' of a function indicates the type of value the function will return, such as an integer (`int`), floating-point number (`float`), or void (if no value is returned).
How does a function's body work in programming?
-The function's body contains the code that defines the actions or operations the function will perform. It executes when the function is called and returns the result (if any) to the caller.
What does the syntax of a function look like in programming?
-The syntax of a function typically follows this structure: `return_type function_name(parameter1, parameter2, ...) { /* function body */ }`. For example: `int factorial(int n) { /* code */ return result; }`.
How are functions called in a program?
-Functions are called in a program by referencing their name and passing the required parameters (if any). The function executes and returns a value to the calling code. For instance: `int result = factorial(5);`.
What happens to memory when a function is called?
-When a function is called, memory is allocated on the call stack. This includes space for the function's parameters, local variables, and the execution code. Once the function finishes executing and returns a result, this memory is freed and removed from the stack.
What is the concept of the call stack in function execution?
-The call stack is a memory structure that keeps track of function calls. Each time a function is called, a new 'stack frame' is created and pushed onto the stack. Once the function completes, its stack frame is popped off the stack, freeing the memory.
Can you call a function from another function? If so, how?
-Yes, you can call one function from another. This is done by simply invoking the function inside the body of another function. For example, a main function can call a `calculateFactorial` function to perform a task.
What is the significance of understanding the call stack in programming?
-Understanding the call stack is crucial because it helps manage memory efficiently and prevents issues such as stack overflow or memory leaks. It also provides insight into how functions interact with memory during program execution.
Outlines
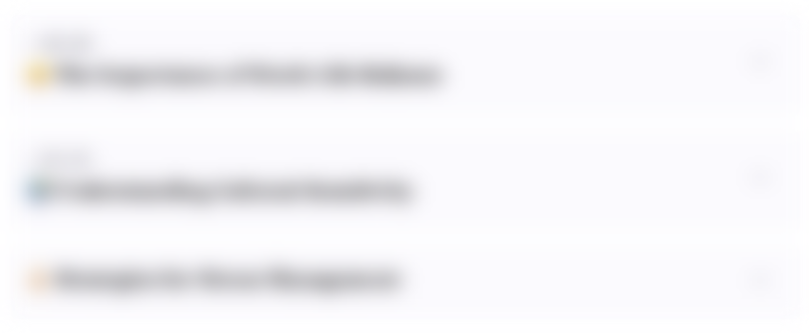
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
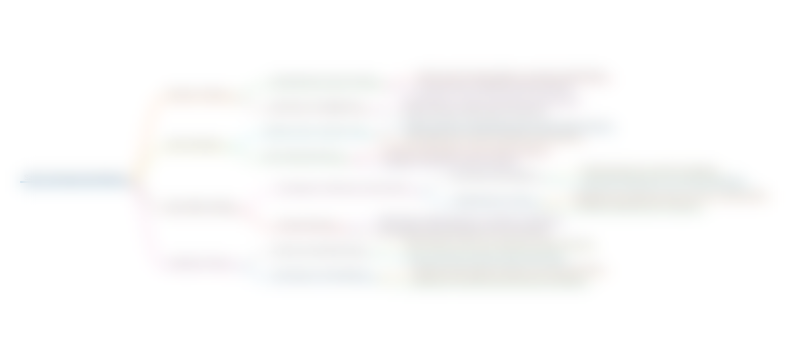
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
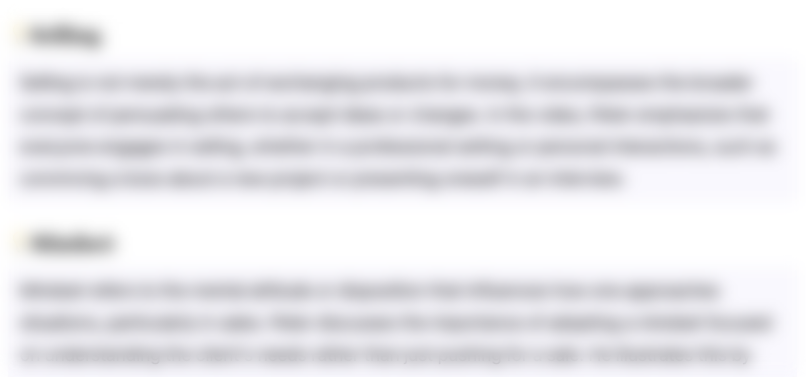
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
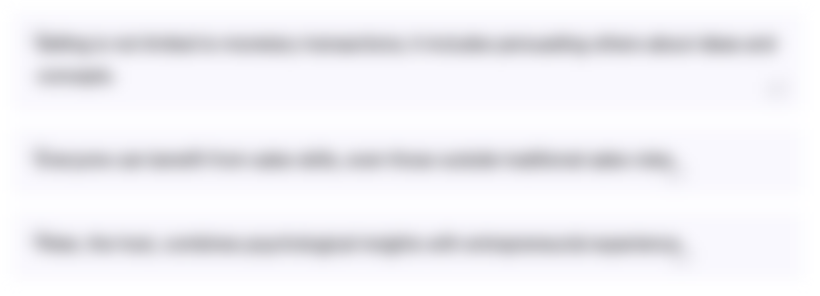
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
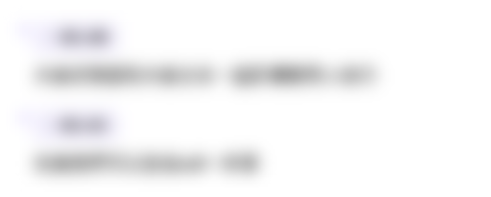
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)