Laravel 11 Breeze Multi-Table Authentication tutorial
Summary
TLDRIn this tutorial, William walks through the process of implementing multi-guard authentication in Laravel, enabling login functionality for both regular users and customers using separate tables. The video covers setting up Laravel Breeze, configuring custom guards for users, and managing multiple authentication systems. It includes creating a customer model, adjusting the authentication logic, and implementing redirections based on user type. The tutorial provides practical insights into how to handle different user types and the challenges of multi-guard authentication, especially around password reset functionality.
Takeaways
- 😀 **Multi-Guard Authentication**: The tutorial demonstrates how to implement multi-guard authentication in Laravel using two separate user models – one for regular users (`users`) and one for customers (`customers`).
- 😀 **Installing Laravel Breeze**: Laravel Breeze is used to set up authentication. The necessary commands are: `composer require laravel/breeze` and `php artisan breeze:install`. Livewire is also included for dynamic interfaces.
- 😀 **User Type Selection in Login**: A select dropdown is added to the login page, allowing users to choose between 'user' and 'customer' as their user type. This value is bound to a model called `user_type`.
- 😀 **Validation for User Type**: A validation rule for the `user_type` field is added to ensure that only valid user types (either 'web' or 'customer') are accepted during login.
- 😀 **Setting Up Customer Guard**: A new `customer` guard is added in the `config/auth.php` file to distinguish between normal users and customers. This includes configuring a new provider that references the `customers` table.
- 😀 **Creating Customer Model and Migration**: The `Customer` model and migration are generated with the command `php artisan make:model Customer -m`. The migration file mirrors the `users` table but is designed specifically for customers.
- 😀 **Login Form Updates**: The login form logic is updated to authenticate based on the selected `user_type` (either `web` for users or `customer` for customers).
- 😀 **Redirecting Users Based on Guard**: After successful login, users are redirected to different dashboards based on their guard type: `user` redirects to the user dashboard, while `customer` redirects to the customer dashboard.
- 😀 **Creating Customer Dashboard View**: A new view `customers.blade.php` is created, which mirrors the user dashboard but is dedicated to customers and their specific dashboard features.
- 😀 **Testing Authentication**: After implementing the login form and guard logic, the tutorial walks through testing the system by logging in with both user and customer credentials to ensure correct behavior based on the selected guard.
- 😀 **Security & Customization Considerations**: While multi-guard authentication works, the tutorial mentions the need to handle certain issues such as password resets for different user tables (e.g., for the `customers` table). It’s also suggested that using a single user table with different roles might be a simpler solution in some cases.
Q & A
What is multi-guard authentication in Laravel?
-Multi-guard authentication in Laravel allows you to authenticate different types of users using separate guards, typically based on different models, such as 'User' and 'Customer'. This enables you to handle authentication for different user groups within the same application.
Why is multi-guard authentication useful?
-Multi-guard authentication is useful when you have multiple types of users with different needs and access levels. For example, in an e-commerce platform, you might have both normal users and customers who need to authenticate using different tables and models.
How do you set up multiple guards in Laravel?
-To set up multiple guards, you modify the 'guards' array in the `config/auth.php` file. You add custom guards for each user type, like 'web' for normal users and 'customer' for customers, specifying different providers and models for each guard.
What are the steps to implement multi-guard authentication?
-The main steps include: installing necessary dependencies (Laravel Breeze and Livewire), creating the necessary models (like 'Customer'), adding custom guards in the `auth.php` configuration, adjusting login forms to handle different user types, and implementing the logic to authenticate users based on the selected guard.
What are the issues with using multiple tables for authentication?
-Using multiple tables for authentication can complicate processes like password resets and role-based access control. Custom logic is required to handle these cases, which can increase complexity. Additionally, managing multiple user tables may lead to inconsistencies and maintenance challenges.
What is the significance of the `guard` in Laravel authentication?
-In Laravel, a guard defines how users are authenticated. A guard specifies which authentication driver to use, such as 'session' or 'token', and determines the provider (e.g., 'users' or 'customers') that holds the user data. Guards allow Laravel to support multiple types of users with different authentication logic.
How do you modify the login form to allow for multi-guard authentication?
-To modify the login form, you add a dropdown (`select`) field that allows users to choose between the available guards (e.g., 'User' or 'Customer'). The value selected in this field determines which guard will be used for authentication when the form is submitted.
What role does the `Auth::guard()` method play in multi-guard authentication?
-The `Auth::guard()` method is used to authenticate a user based on the selected guard. In multi-guard authentication, it allows Laravel to dynamically authenticate users with different models and databases, such as using the 'web' guard for users and the 'customer' guard for customers.
What happens if the selected user type is invalid in multi-guard authentication?
-If the selected user type is invalid, such as if an incorrect value is passed in the login form, the system should throw an exception to prevent unauthorized access. This ensures that only valid user types (like 'web' or 'customer') are processed.
What is the alternative to using multiple user tables for authentication?
-An alternative approach is to use a single `users` table with roles or user types (e.g., 'admin', 'customer', 'user'). This simplifies authentication management by using a single table and assigning different roles to users, which can be handled through policies and access control rather than managing separate tables.
Outlines
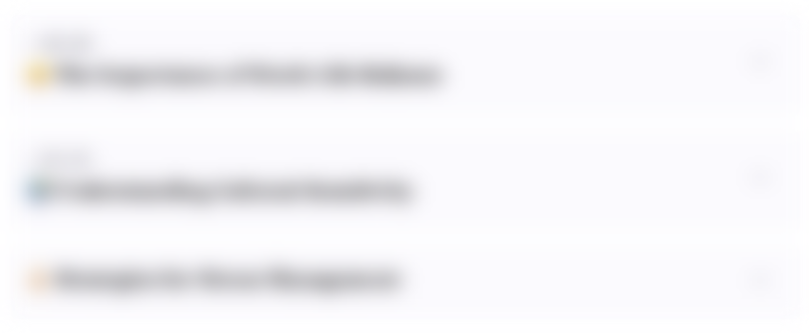
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
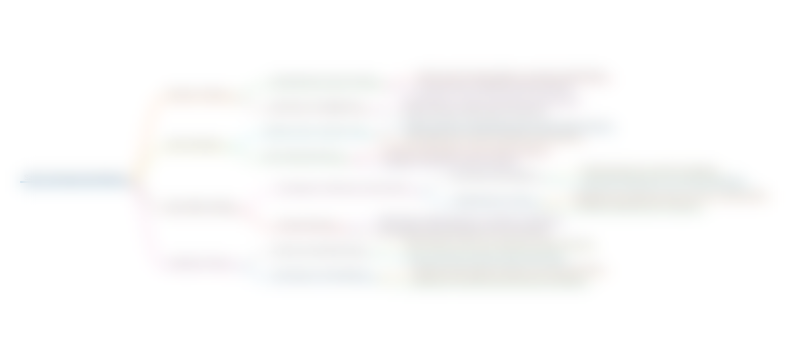
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
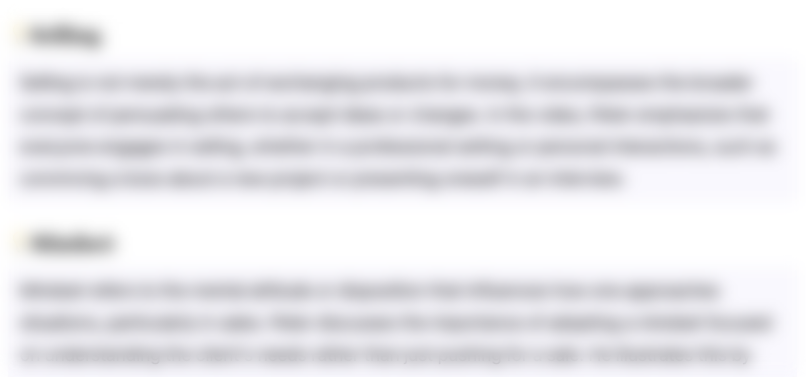
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
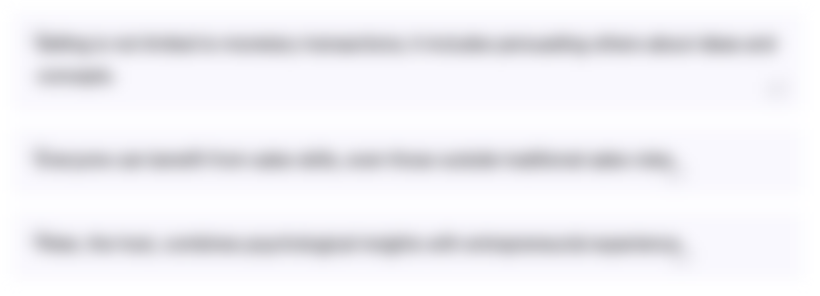
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
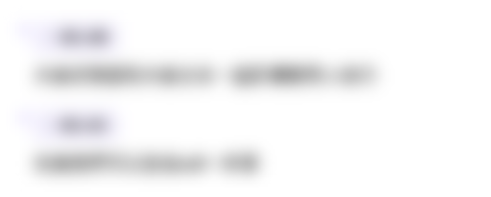
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
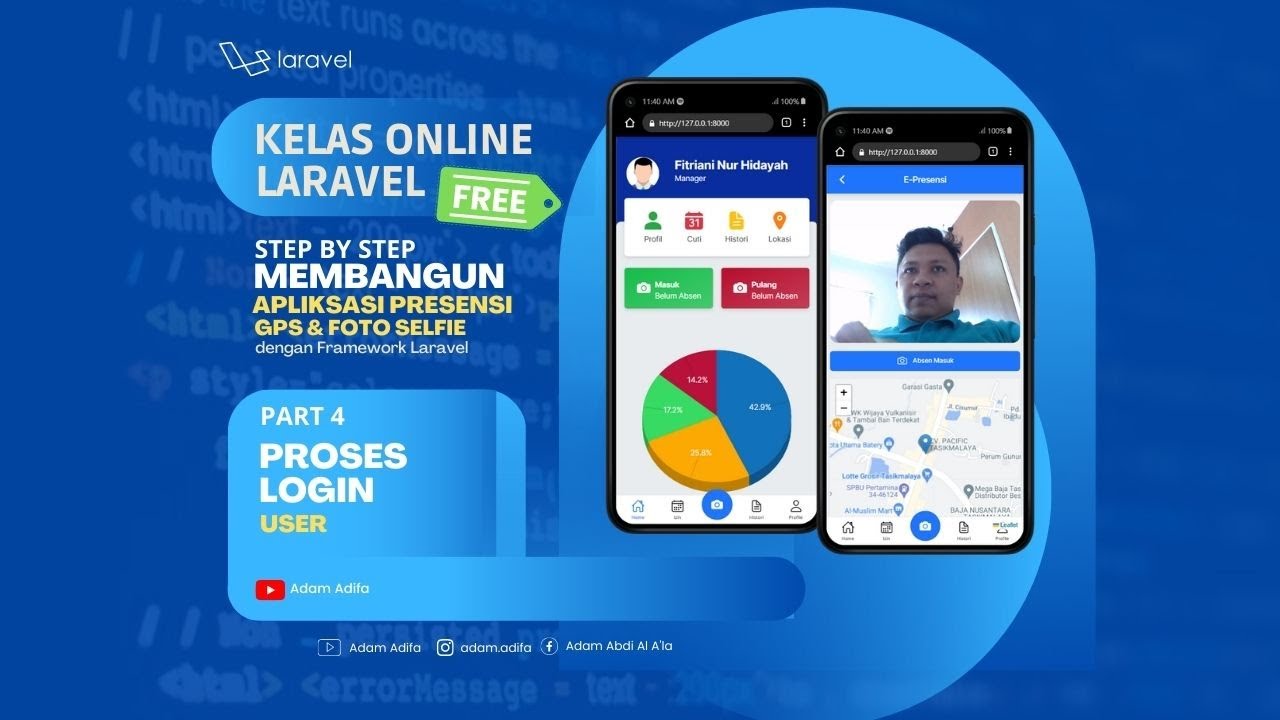
04 - Membuat Proses Login Untuk User
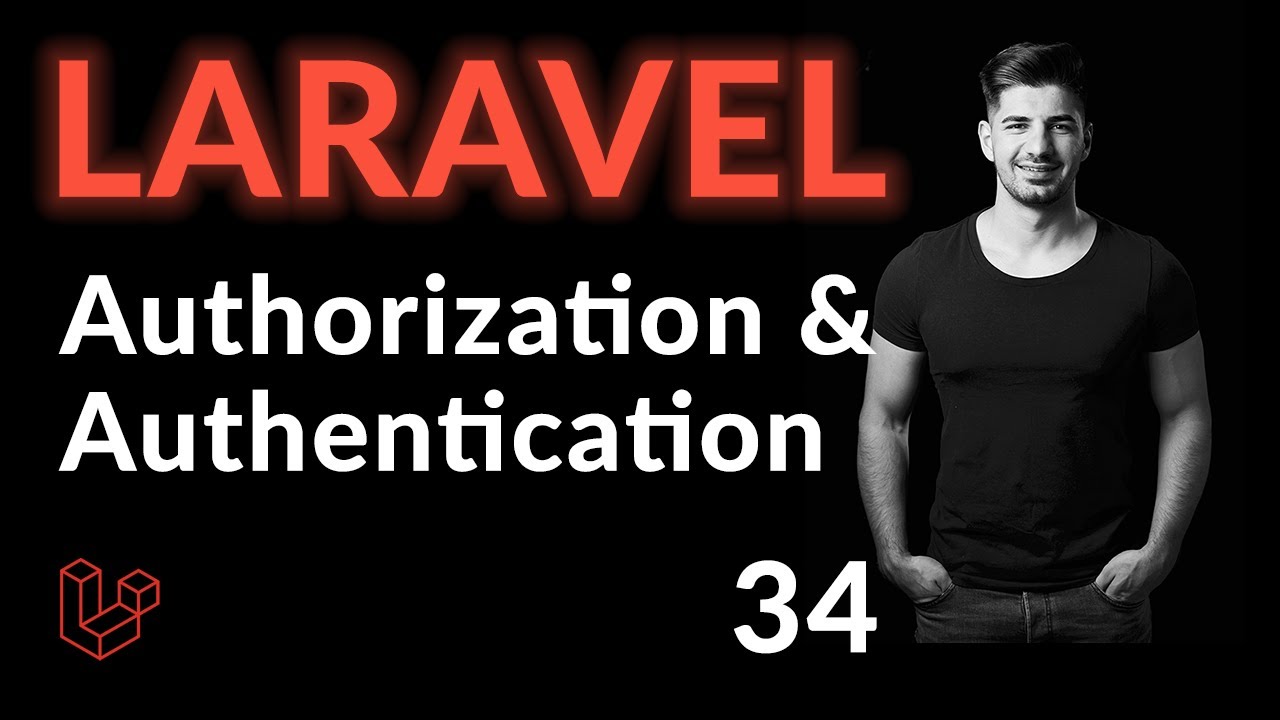
Authorization & Authentication | Login & Register System In Laravel | Laravel For Beginners
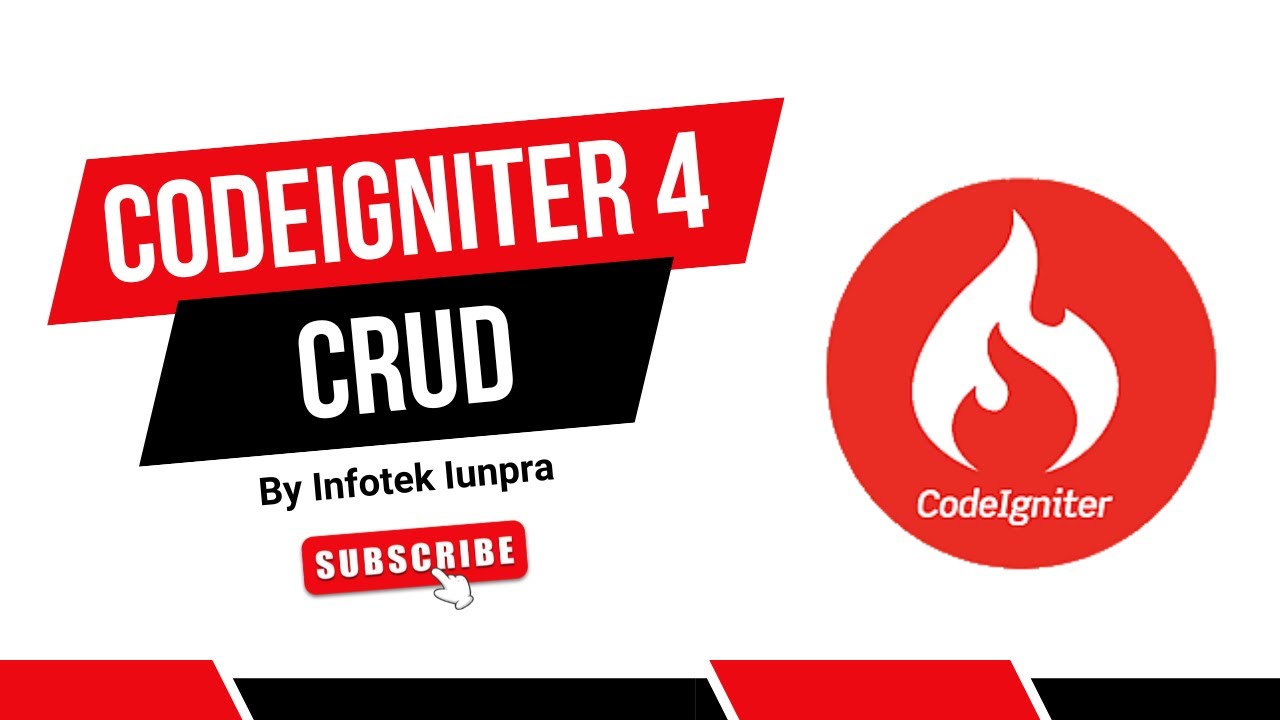
Seri 11 PWEB Login, Logout dan Auth
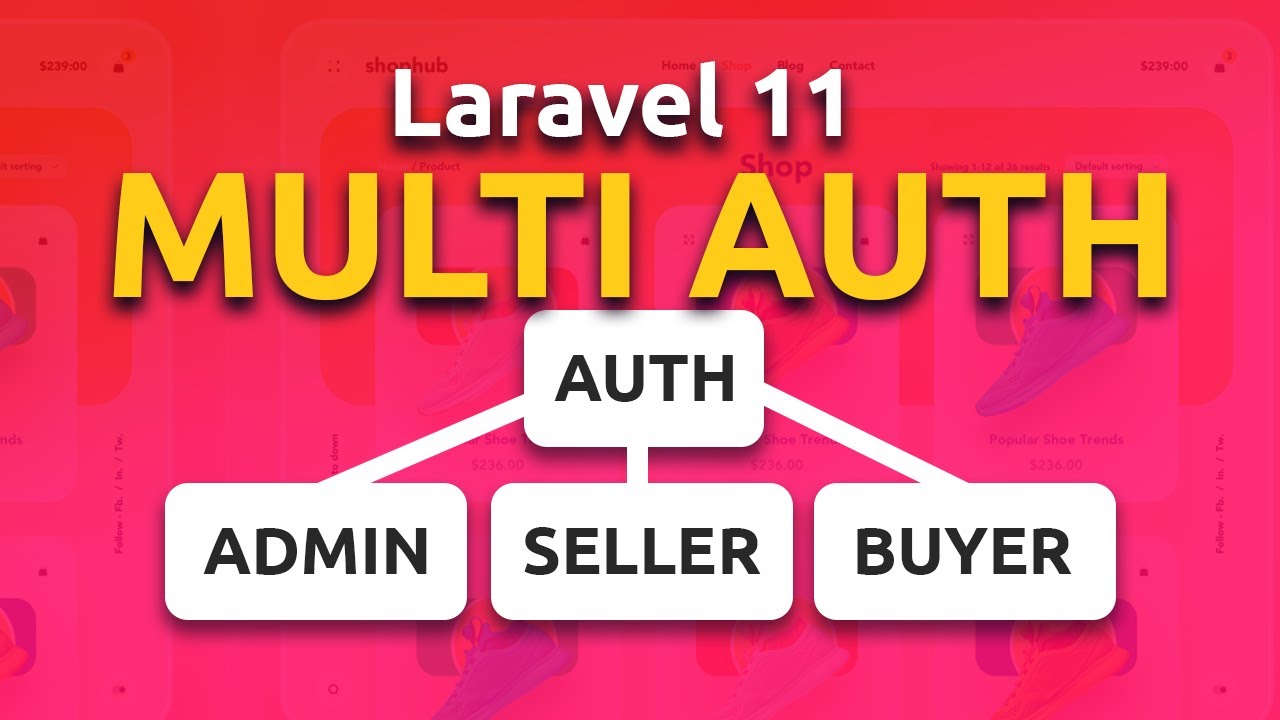
Laravel 11 Multi Auth For Ecommerce Website | Step By Step

03 - Membuat Database Presensi
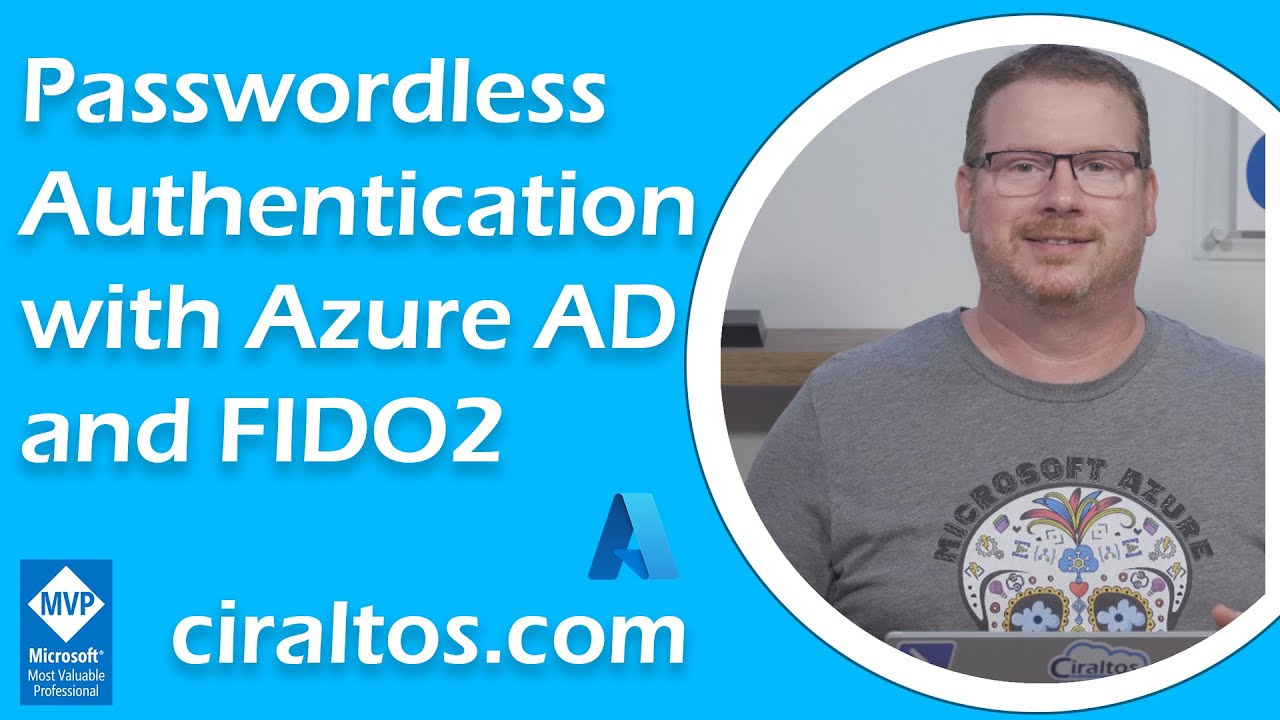
Passwordless Authentication with Azure AD and FIDO2 Security Keys and Yubikey Bio
5.0 / 5 (0 votes)