2. State and State Manager | 2D Snake Game - C++ & SFML #DevKage
Summary
TLDRIn this video tutorial, the focus is on implementing state management for a C++ snake game using SFML. The instructor walks through the creation of two essential classes: the `State` class and the `StateManager` class. The `State` class defines the core functionalities such as initialization, input processing, updating, and rendering, while the `StateManager` class manages state transitions using a stack data structure. The tutorial provides clear code examples and explains concepts like managing different game states (e.g., splash screen, gameplay, game over) to ensure smooth game flow and transitions.
Takeaways
- π The video is part of a C++ game development series, focused on creating a Snake game using C++ and SFML.
- π The game development involves creating a state management system with classes for handling different game states.
- π A game state is any stage that processes inputs, updates itself, and optionally loads a new state, like splash screens, menus, gameplay, or game over screens.
- π States in the game are managed like a stack data structure, where states can be pushed, popped, or replaced in a controlled manner.
- π The State class is an abstract base class that defines essential functions like init, process input, update, and draw, which all derived states must implement.
- π The init method handles the initial setup of the state, such as setting up sprites, text, and positions.
- π The process input method handles user inputs like key presses and mouse clicks.
- π The update method reacts to inputs and updates the state, adjusting things like sprite positions, ensuring consistent FPS across different machines.
- π The draw method is responsible for rendering sprites and text to the game window.
- π The StateManager class handles the stack of game states, with methods like add, pop, process state changes, and get current state to manage transitions between states.
- π The add method allows for adding new states, and the pop method removes the current state from the stack. State changes are processed in the process state change method, which modifies the stack based on flags like add, replace, and remove.
Q & A
What is the purpose of states in a game?
-States represent distinct stages or phases of a game where different processes occur, such as a splash screen, main menu, gameplay, or game over screen. Each state handles specific tasks like input processing, updates, and rendering.
What does the 'State' class define in the script?
-The 'State' class is an abstract base class that defines the core methods required for any game state, including `init()`, `processInput()`, `update()`, and `draw()`. Derived classes must implement these methods for specific states like the main menu or gameplay.
Why are the methods 'pause()' and 'start()' included in the 'State' class?
-'pause()' and 'start()' are optional methods used for pausing and starting states, such as when a game is paused and a transparent menu overlays the game world. These methods help control input and state transitions during special conditions.
What role does the 'StateManager' class play in the game?
-The 'StateManager' class manages the stack of game states. It allows states to be pushed, popped, or replaced in a controlled manner, ensuring proper state transitions. It also provides methods for adding, removing, and getting the current state.
How does the 'StateManager' class ensure memory management for states?
-The 'StateManager' class uses unique pointers (`std::unique_ptr`) to manage the memory of states. This ensures that the memory is automatically freed when a state is removed from the stack, preventing memory leaks.
What is the significance of using a stack to manage states in the game?
-A stack allows for easy management of game states by following a Last-In-First-Out (LIFO) approach. The current state is always on top of the stack, and the state manager can push, pop, or replace states efficiently while ensuring smooth transitions.
Why is the 'update()' method in the 'State' class passed a parameter of type 'SFTime'?
-The 'SFTime' parameter represents the elapsed time since the last update. It is essential for making the game frame rate-independent, ensuring that the game runs at the same speed across different machines.
What happens when a state is popped in the 'StateManager' class?
-When a state is popped, it is removed from the stack, and the 'start()' method of the new top state (if any) is called to begin processing inputs for the new state.
What is the purpose of the 'processStateChange()' method in the 'StateManager' class?
-The 'processStateChange()' method handles the actual modification of the state stack. It checks the flags for adding, replacing, or removing states and performs the appropriate actions, such as popping the current state, adding a new state, or replacing an existing one.
Why is it important to call 'pause()' on the current state before replacing it in the 'StateManager' class?
-Calling 'pause()' ensures that the current state is properly suspended before transitioning to a new state. This is especially important when the current state involves gameplay, as it prevents inputs from affecting the game world while the new state is being processed.
Outlines
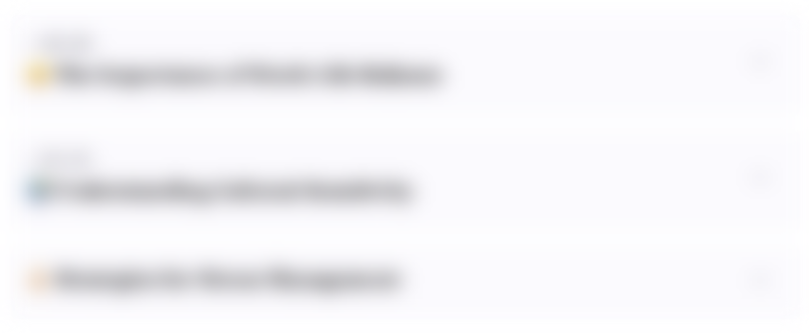
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
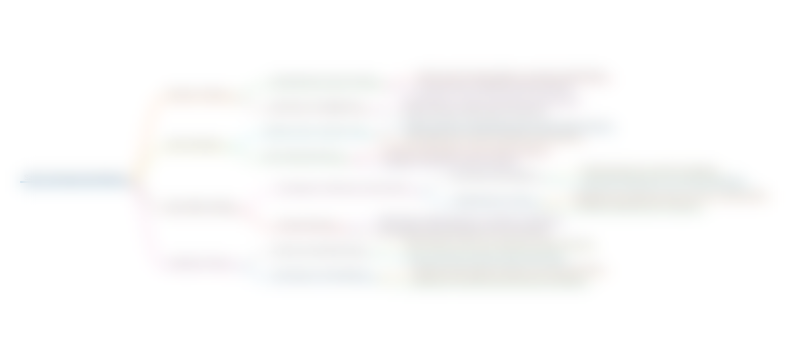
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
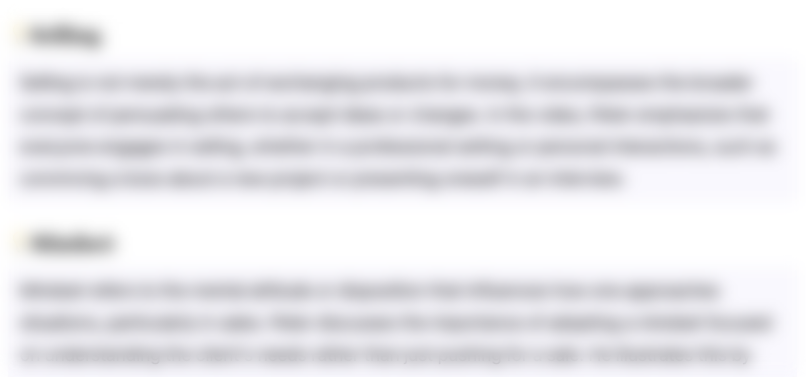
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
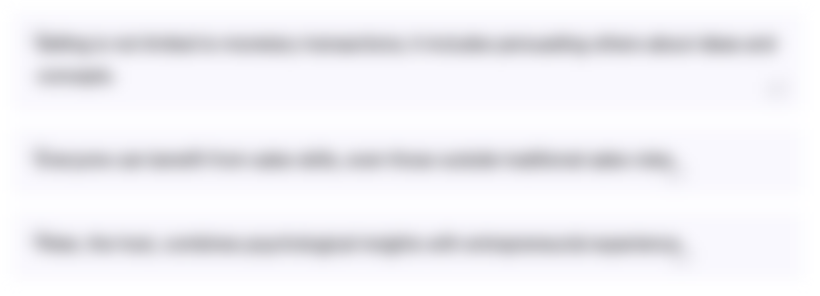
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
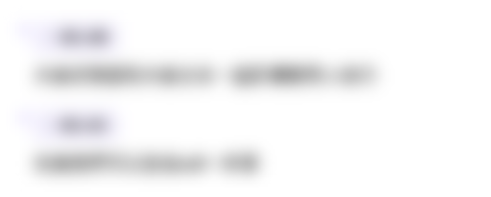
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
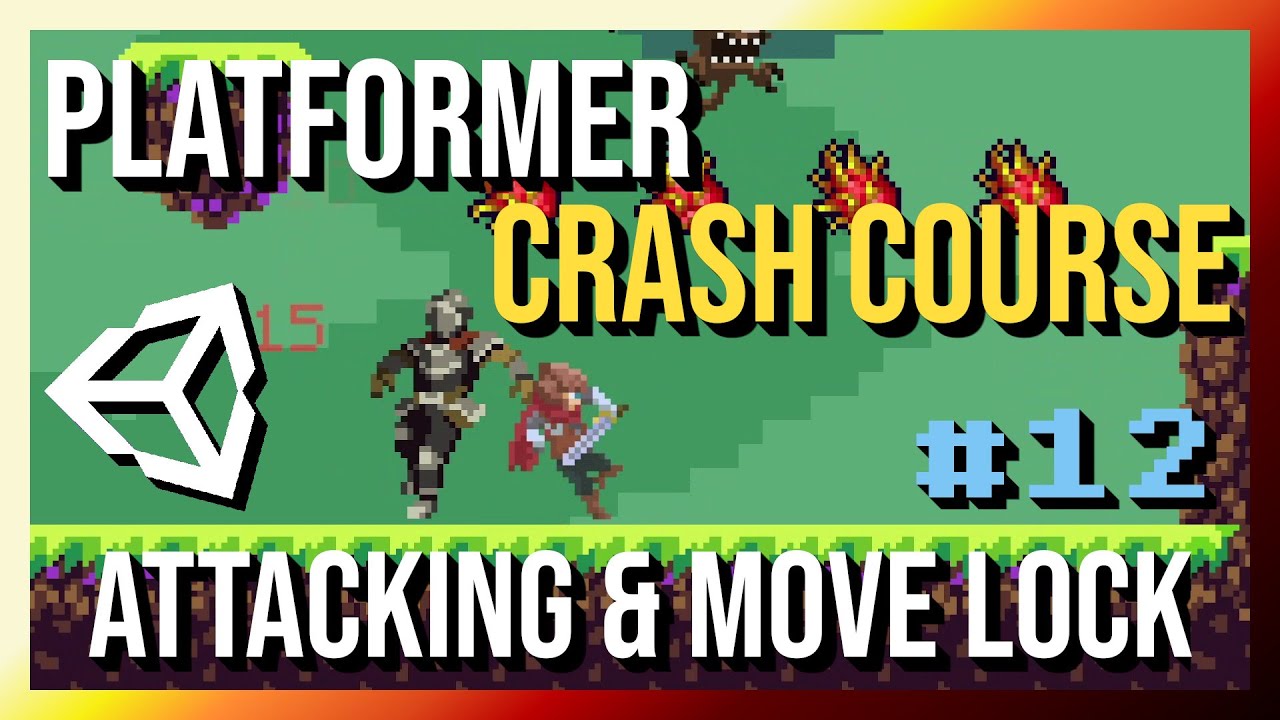
Attack Animation and Movement Lock - 2D Platformer Crash Course in Unity 2022 (Part 12)
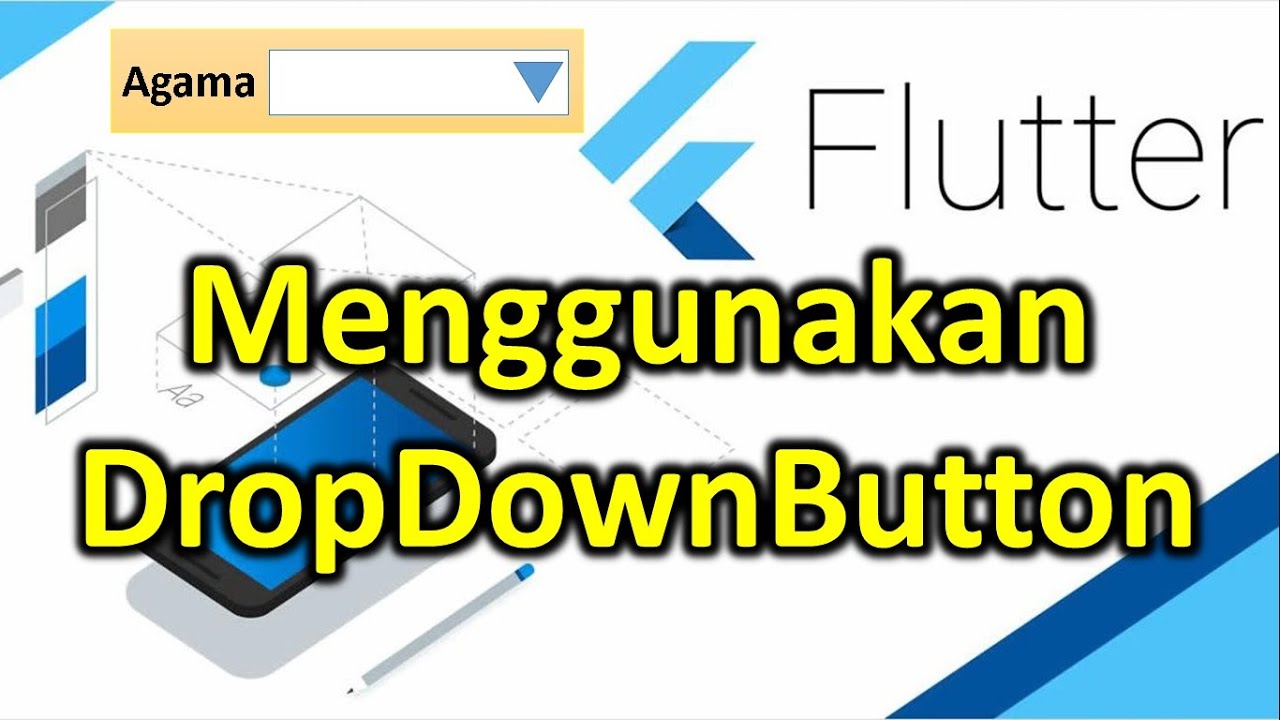
Use Penggunaan Drop Down Button in Flutter | DropDownButton in Flutter

Flutter | Clean Architecture | Dashboard Feature
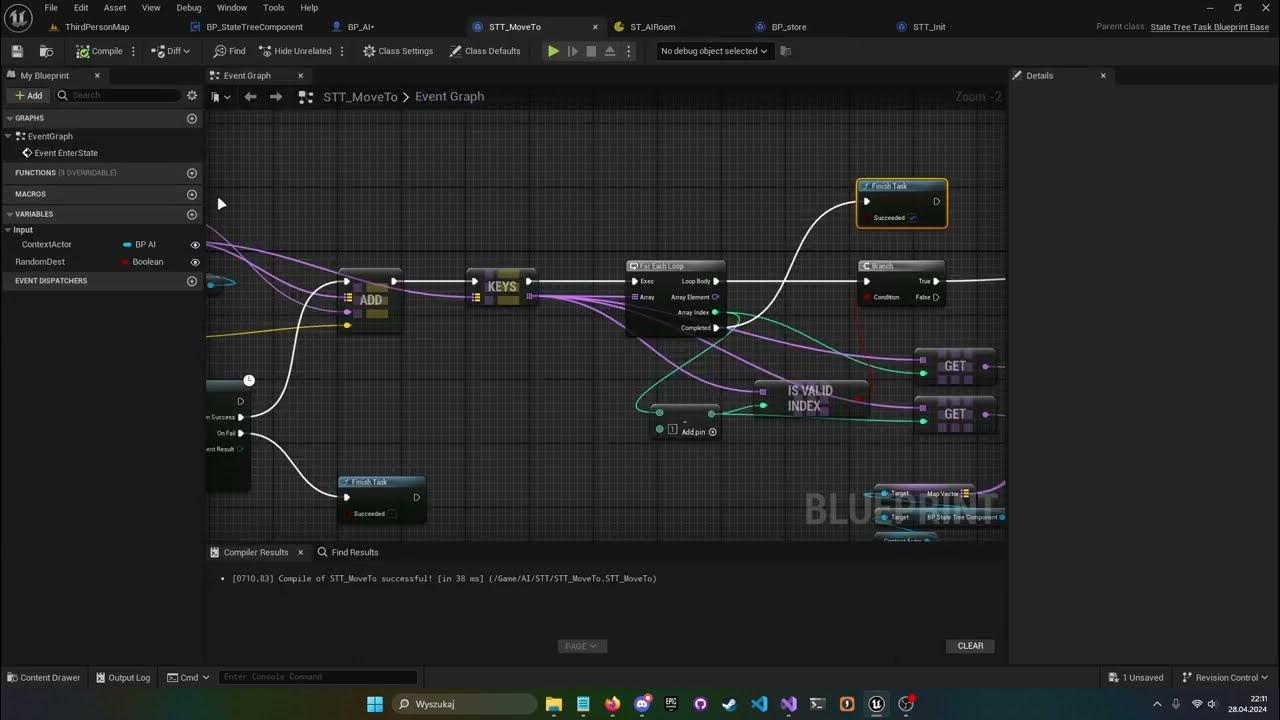
UE5.4 State Tree Data Management

Conditional Rendering | Mastering React: An In-Depth Zero to Hero Video Series
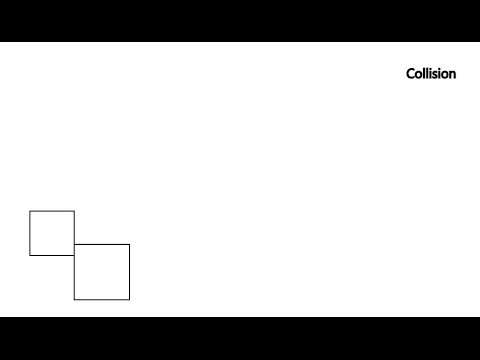
Game Dev: Collision Detection - Bounding Box
5.0 / 5 (0 votes)