Chapter 5 - Repetition Structures
Summary
TLDRThis video provides an introduction to repetition structures in programming, covering key loop types such as conditional loops (while, do-while, do-until), count-controlled loops, and nested loops. The discussion also includes concepts like running totals, sentinel values that mark loop termination, and best practices to avoid infinite loops. The tutorial emphasizes how loops help reduce code duplication and improve efficiency, allowing for dynamic control over repetitive tasks in a program. Key takeaways include the importance of proper loop structure and how to effectively use loops in different scenarios.
Takeaways
- π Repetition structures, also known as loops, allow for repeated execution of blocks of code without duplicating them in a program.
- π Conditional control loops include 'while', 'do while', and 'do until' loops, each of which executes code based on a condition's truth value.
- π A 'while' loop is a pre-test loop, meaning the condition is tested before any code is executed. If the condition is false initially, the loop does not execute.
- π A 'do while' loop is a post-test loop, meaning it executes the code first and then checks the condition after the loop completes one iteration.
- π A 'do until' loop runs until a condition becomes true, and it's essential to manage the stopping condition to avoid infinite loops.
- π Infinite loops can occur if the exit condition is never met, so it's crucial to include logic within loops to ensure they terminate.
- π Count control loops run a specific number of times, typically based on a variable initialized to a starting value and incremented until it reaches a maximum value.
- π Count control loops can also decrement (count backwards), and the loop's control variable must be initialized, tested, and incremented/decremented correctly.
- π Running totals accumulate a sum during loop execution, and an accumulator variable keeps track of the total as the loop iterates over a series of numbers.
- π Sentinels are special values used to mark the end of a sequence or list, helping to control when a loop should terminate by signaling that the end has been reached.
- π Nested loops occur when one loop is placed inside another, allowing for more complex iteration over multi-dimensional data or performing tasks in a sequential manner.
Q & A
What is the primary purpose of using loops in programming?
-The primary purpose of using loops in programming is to repeat a block of code multiple times without having to duplicate the code, which makes programs more efficient, easier to maintain, and less error-prone.
What are conditional control loops and what types are discussed in the lecture?
-Conditional control loops are loops that run based on a condition being true or false. The lecture discusses three types: the 'while' loop (pre-test loop), the 'do while' loop (post-test loop), and the 'do until' loop, which iterates until a condition becomes true.
How does a 'while' loop differ from a 'do while' loop?
-'While' loops are pre-test loops, meaning the condition is checked before the loop executes. If the condition is false initially, the loop will not run. In contrast, 'do while' loops are post-test loops, meaning the loop will execute at least once, and the condition is checked after each iteration.
What is a key caution when using loops in programming?
-A key caution is to avoid creating infinite loops. It's important to include a stopping condition within the loop to ensure the loop terminates at the right time and does not run indefinitely.
What is the concept of a running total in loops, and how is it typically used?
-A running total is the accumulation of a series of numbers as the loop iterates. It involves summing numbers with each iteration and using an accumulator variable to hold the total, which is updated after each loop execution.
What is a sentinel in programming, and how is it used in loops?
-A sentinel is a special value that marks the end of a sequence of data. It is used as a stop value in loops, allowing the program to terminate the loop when the sentinel is encountered, instead of relying on a predetermined number of iterations.
What is a nested loop, and when might it be useful?
-A nested loop is a loop inside another loop. It is useful when you need to repeat a set of operations multiple times within another operation, such as iterating through rows and columns in a table or grid.
What is the significance of the 'do until' loop, and which programming languages typically support it?
-'Do until' loops iterate until a condition becomes true, and they are particularly useful when the number of iterations is unknown at the start. Not all programming languages support 'do until' loops, so it's important to verify language compatibility.
In a count control loop, what are the main steps involved in its execution?
-The main steps in a count control loop are: initializing the loop control variable, testing the condition (comparing the variable to its maximum value), executing the loop, and incrementing (or decrementing) the loop control variable. The loop terminates when the variable reaches the maximum value.
Why is it important to initialize and modify the loop control variable in a count control loop?
-Initializing and modifying the loop control variable is crucial to ensure the loop executes the correct number of times. Failure to do so may result in incorrect loop behavior, such as skipping iterations or running indefinitely.
Outlines
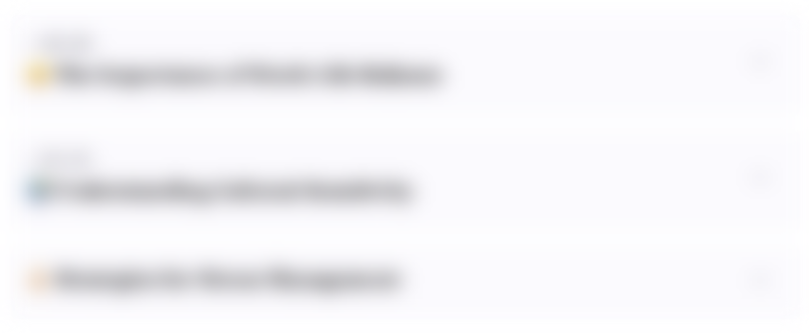
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
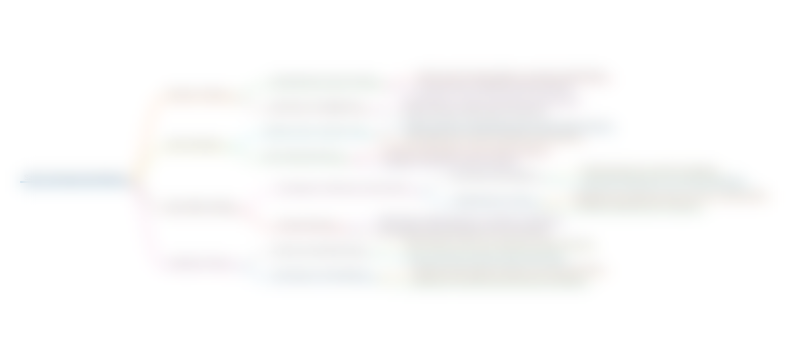
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
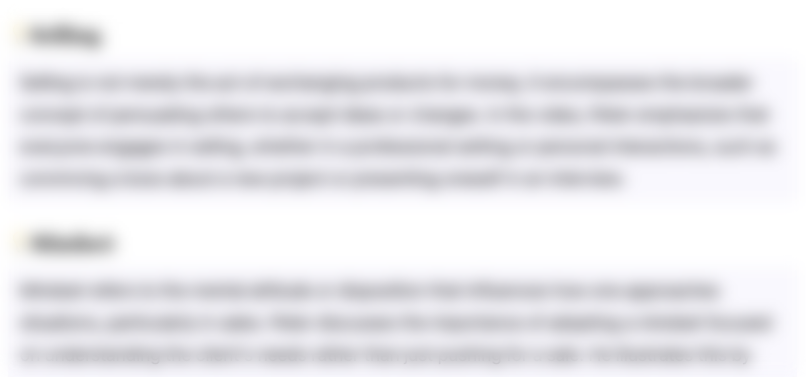
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
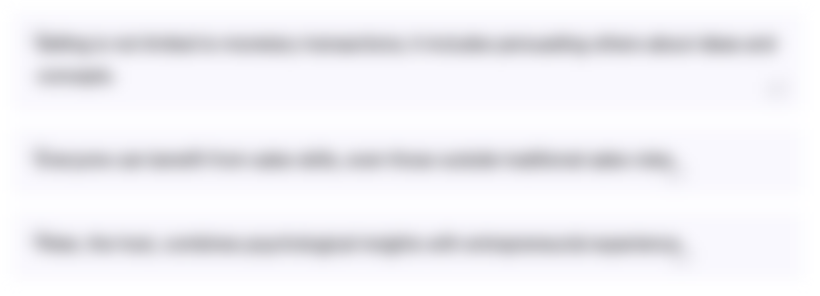
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
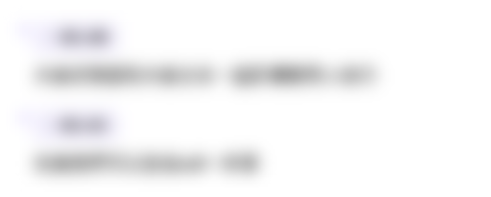
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)