The Unreal Engine Game Framework: From int main() to BeginPlay
Summary
TLDRThe video script delves into the intricacies of the Unreal Engine's game framework, illustrating the process from the game's initialization to the start of gameplay. It explains the concept of the game loop and how Unreal Engine abstracts it through classes like GameMode and PlayerController. The script outlines the engine's initialization stages, including module loading, engine object creation, and map loading, leading to the game's start with the BeginPlay event. It emphasizes the engine's flexibility for both single-player and multiplayer game development, highlighting the importance of understanding the framework for clean and efficient game design. The summary serves as a guide for developers to leverage Unreal Engine's powerful tools and systems for creating engaging game experiences.
Takeaways
- π **Game Loop Concept**: The fundamental concept in game programming is the game loop, which involves initialization, processing input, updating game state, and rendering to screen.
- π **Unreal Engine Workflow**: In Unreal Engine, you don't deal directly with the game loop. Instead, you work with subclasses like GameMode and functions such as InitGame, BeginPlay, or Tick.
- π οΈ **Extensibility and Flexibility**: Unreal Engine offers power and flexibility, being open-source and designed to be extended through various means, including subclassing and overriding functions.
- π§© **GameFramework Overview**: Understanding classes like GameMode, GameState, PlayerController, Pawn, and PlayerState is crucial for leveraging the engine's full capabilities.
- π **Source Code Exploration**: Gaining familiarity with the engine involves looking at its source code to understand how the game boots up and the sequence of operations.
- π§ **Engine Initialization Process**: The engine runs thousands of lines of code to set up global state and initialize systems before reaching higher-level abstractions.
- π **Module System**: The engine is divided into source modules, which are loaded in phases to manage dependencies and ensure only necessary parts are loaded for a given configuration.
- ποΈ **Game Instance and World Creation**: The engine creates a GameInstance, GameViewportClient, and LocalPlayer before loading the game map and initializing the world.
- πΎ **Actor Initialization and Registration**: Actors and their components are registered, initialized, and brought up for play during the LoadMap process.
- π₯ **Player Login and Pawn Possession**: Player login is handled by the GameMode, which spawns PlayerControllers and PlayerStates, leading to Pawn possession and player setup in the game world.
- π **Game Loop and Map Loading**: The game loop manages the entire process from initialization to shutting down, with map loading being a significant part of the game's startup sequence.
Q & A
What is the fundamental concept in game programming that the script begins with?
-The fundamental concept in game programming that the script begins with is the game loop, which involves initialization, processing input, updating the game world state, and rendering the results to the screen.
How does Unreal Engine abstract the game loop for developers?
-Unreal Engine abstracts the game loop by allowing developers to start with defining a GameMode subclass and overriding functions like InitGame, BeginPlay, or Tick, rather than dealing with the main function and the game loop directly.
What are some of the key classes in Unreal Engine's GameFramework?
-Some of the key classes in Unreal Engine's GameFramework include GameMode, GameState, PlayerController, Pawn, and PlayerState.
How does the engine handle the transition from the entry point to running game code?
-The engine handles the transition from the entry point to running game code by initializing various systems and setting up global state through thousands of lines of code, which, while complex, are necessary for the engine to function correctly.
What is the role of FEngineLoop in the Unreal Engine?
-FEngineLoop is a class that implements the main loop for the Unreal Engine. It manages the PreInit stage, full initialization of the engine, and then ticks every frame until the program is ready to exit.
How does the module system in Unreal Engine help with managing dependencies and configurations?
-The module system in Unreal Engine helps manage dependencies and configurations by splitting the engine into different source modules, allowing for essential systems to be initialized first and ensuring that only the necessary modules for a given configuration are loaded.
What is the purpose of a CDO (Class Default Object) in Unreal Engine?
-A CDO (Class Default Object) in Unreal Engine serves as a record of a class in its default state and acts as a prototype for further inheritance. It is used to allocate a default instance of a class and run its constructor, passing in the CDO of the parent class as a template.
What is the significance of the UEngine class in Unreal Engine's initialization process?
-The UEngine class is significant in Unreal Engine's initialization process as it is responsible for creating an instance of the GameEngine class, initializing it, and starting the game. It also contains functions for loading maps and browsing to URLs.
How does the LoadMap function contribute to the game's initialization?
-The LoadMap function contributes to the game's initialization by finding and loading the map package, initializing the World, registering components, initializing GameMode and GameSession, and finally calling BeginPlay on all actors, completing the game's startup sequence.
What is the difference between a PlayerController and a Pawn in Unreal Engine?
-A PlayerController in Unreal Engine represents the player within the game world and is responsible for controlling the player's interactions. A Pawn, on the other hand, is a specialized type of actor that can be possessed by a Controller and represents the in-world entity that the player or AI controls.
Why is it important to understand the lifetime of different game framework actors in Unreal Engine?
-Understanding the lifetime of different game framework actors is important because it determines when and how these actors are created, used, and destroyed in the game. This knowledge helps developers manage resources effectively and ensures that game state is maintained correctly across different game phases.
How can developers extend functionality in Unreal Engine beyond class inheritance?
-Developers can extend functionality in Unreal Engine beyond class inheritance by binding callback functions to delegates that represent specific engine events, or by using the subsystem feature which allows for the automatic creation of instances of custom classes tied to the lifetime of corresponding objects.
Outlines
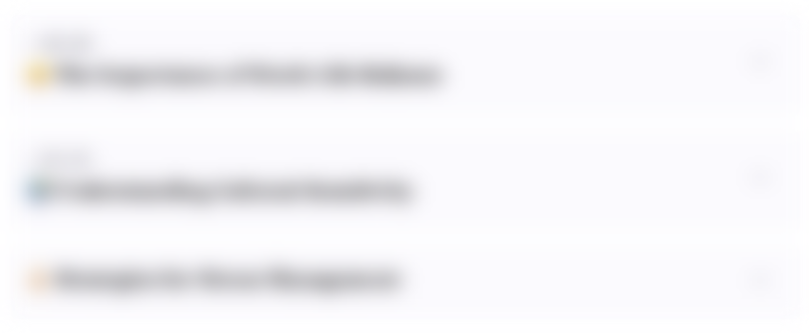
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
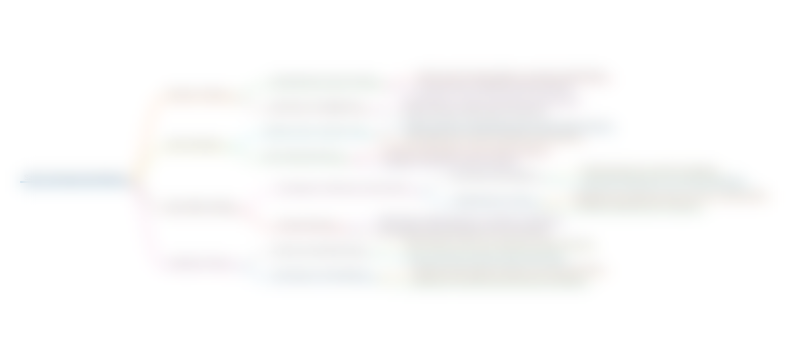
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
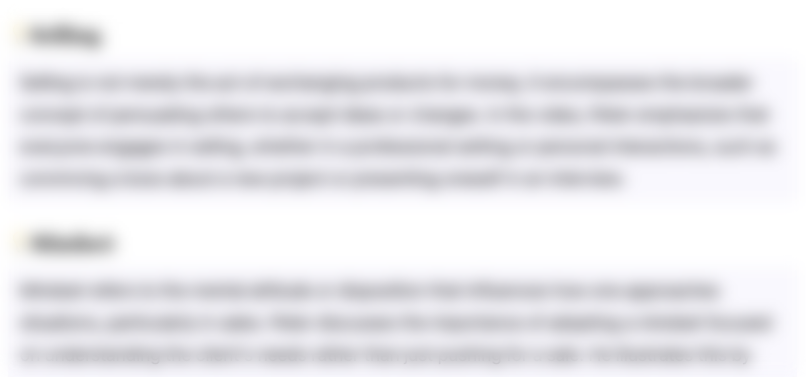
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
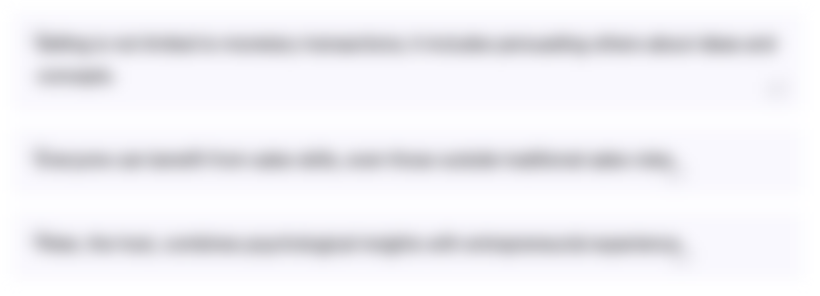
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
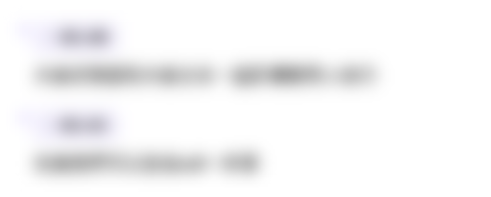
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)