Intro - GCD
Summary
TLDRThis video explains the Euclidean algorithm for calculating the Greatest Common Divisor (GCD) of two numbers. It starts by comparing a brute-force approach with the efficiency of the Euclidean method. The algorithm is described step-by-step, using examples like 8 and 6, and 102 and 21, demonstrating how division and remainders lead to the GCD. The video also covers the mathematical reasoning behind the algorithm and provides a simple Python code implementation. By following these steps, viewers will gain a clear understanding of the Euclidean algorithm and its practical applications.
Takeaways
- 😀 The GCD (Greatest Common Divisor) of two numbers can be calculated by finding the largest number that divides both, but a more efficient method is needed for larger numbers.
- 😀 A simple but slow method involves checking all numbers between 1 and the smaller of the two numbers to see if they divide both numbers.
- 😀 The Euclidean algorithm is a much faster method to calculate the GCD, relying on the modulo operation to reduce the size of the problem.
- 😀 The Euclidean algorithm repeatedly calculates the remainder (a % b) and swaps the values of the two numbers until the remainder becomes zero.
- 😀 The formula behind the Euclidean algorithm is: GCD(a, b) = GCD(b, a % b). This ensures that the GCD is found efficiently by reducing the numbers progressively.
- 😀 Using the example of 8 and 6, the Euclidean algorithm proceeds by checking the remainder until the GCD is found, demonstrating how much faster it is compared to the simple method.
- 😀 A mathematical understanding of the Euclidean algorithm reveals that it is based on the fact that the GCD of two numbers remains the same if we replace one number by the remainder of its division by the other.
- 😀 A key benefit of the Euclidean algorithm is its efficiency; it can find the GCD of very large numbers in much fewer steps than the basic method.
- 😀 The modulo operation (a % b) is a crucial component in the algorithm, representing the remainder when a is divided by b, and it plays a key role in simplifying the process.
- 😀 The Euclidean algorithm can be easily implemented in a programming environment, where the values of the numbers are updated iteratively through assignments and modulo operations until the remainder reaches zero.
Q & A
What is the purpose of the algorithm described in the transcript?
-The algorithm is designed to calculate the greatest common divisor (GCD) of two positive integers, m and n, using an efficient method based on the Euclidean algorithm.
How does the basic GCD algorithm work in the script?
-The basic algorithm starts by testing numbers from 1 to the smaller number (n), checking for divisibility. The largest number that divides both m and n is identified as the GCD.
Why is the basic GCD algorithm inefficient for large numbers?
-The basic method tests every number from 1 to n, which becomes computationally expensive as the numbers grow larger, especially when m and n are prime to each other.
What is the key improvement introduced by the Euclidean algorithm?
-The Euclidean algorithm improves efficiency by using the modulo operation to reduce the problem size at each step, with the formula GCD(a, b) = GCD(b, a % b), ultimately finding the GCD in fewer steps.
How does the Euclidean algorithm reduce the size of the problem?
-The algorithm repeatedly replaces the larger number with the remainder when the larger number is divided by the smaller one, reducing the problem size each time until the remainder is zero.
What is the significance of the modulo operation (%) in the Euclidean algorithm?
-The modulo operation calculates the remainder when one number is divided by another. This remainder is used in subsequent steps to reduce the size of the numbers until the GCD is found.
Can you explain the example of calculating the GCD of 102 and 21 in the script?
-In the example, 102 modulo 21 gives 18. Then, 21 modulo 18 gives 3. Finally, 18 modulo 3 gives 0, indicating that the GCD is 3.
What does the script mean by 'if b = 0, the algorithm ends'?
-The algorithm ends when the smaller number (b) becomes 0, at which point the larger number (a) is the GCD. This is because the remainder of the division in the Euclidean method reaches 0.
What is the role of variables a, b, and g in the Euclidean algorithm?
-In the algorithm, 'a' represents the larger number, 'b' represents the smaller number, and 'g' stores the result of the modulo operation. These variables are updated at each step to progressively find the GCD.
How does the script describe the concept of variable assignment in the algorithm?
-The script explains that variable assignment in the algorithm means updating the value of a variable. For example, the operation 'a = b' assigns the value of b to a, and 'b = g' assigns the value of g to b.
Outlines
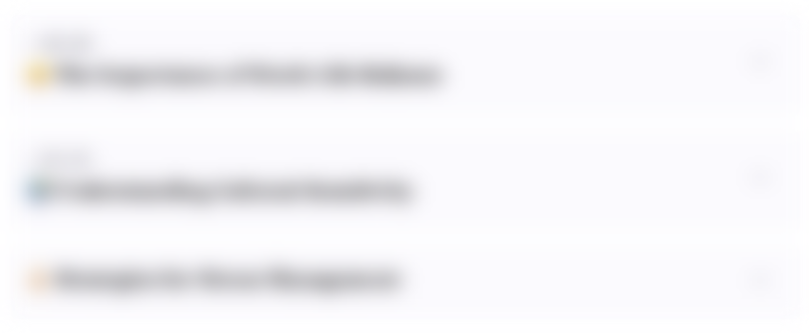
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
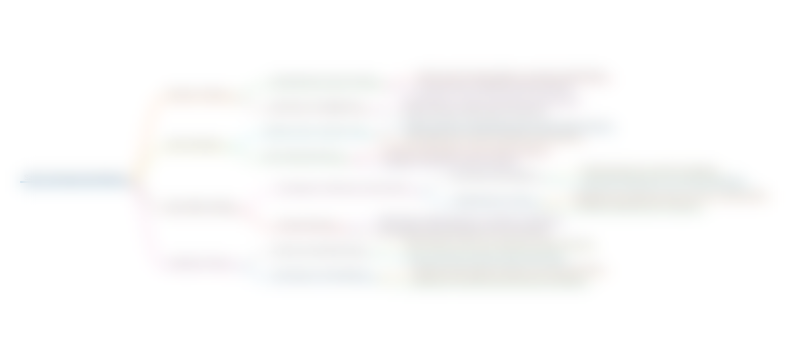
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
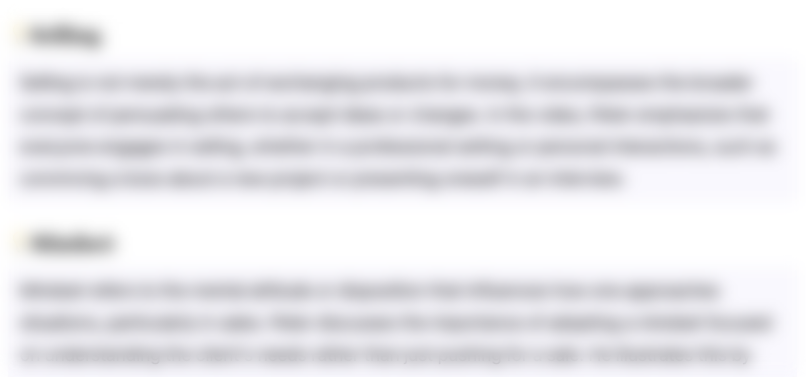
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
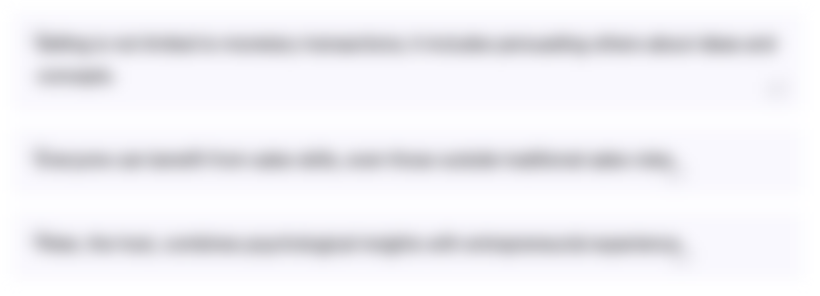
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
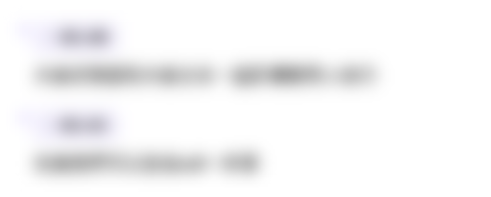
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
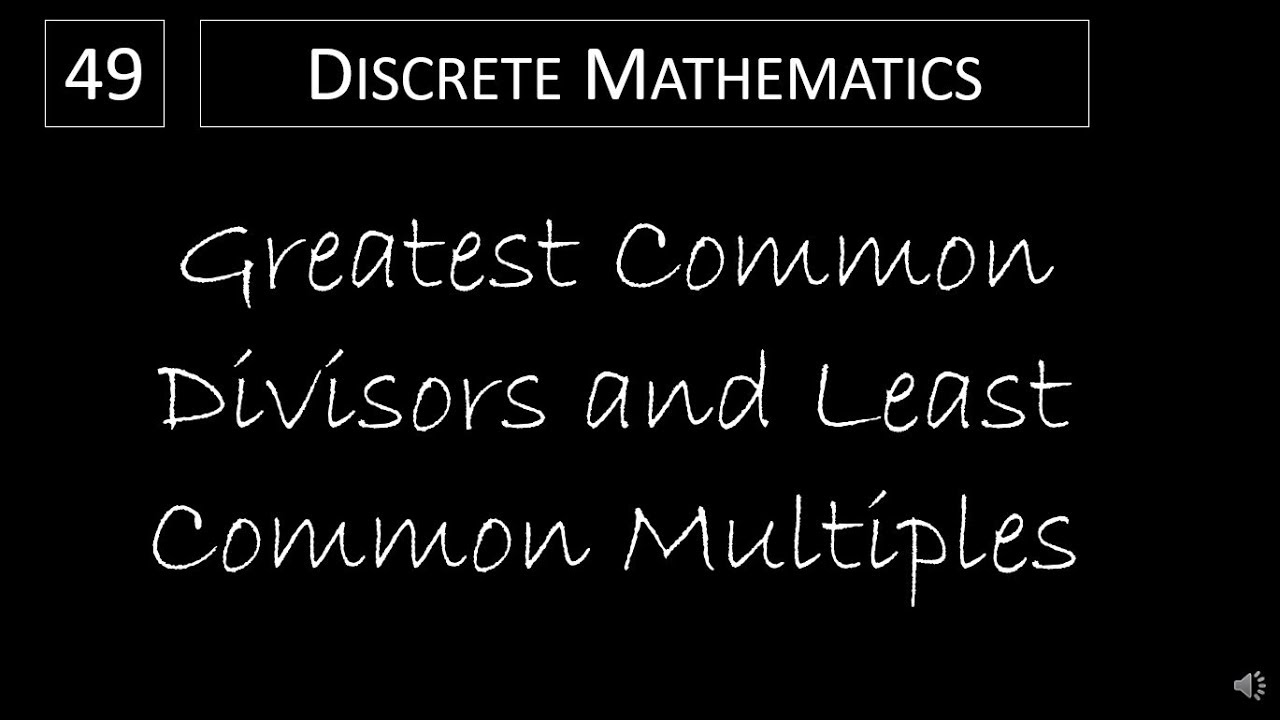
Discrete Math - 4.3.2 Greatest Common Divisors and Least Common Multiples
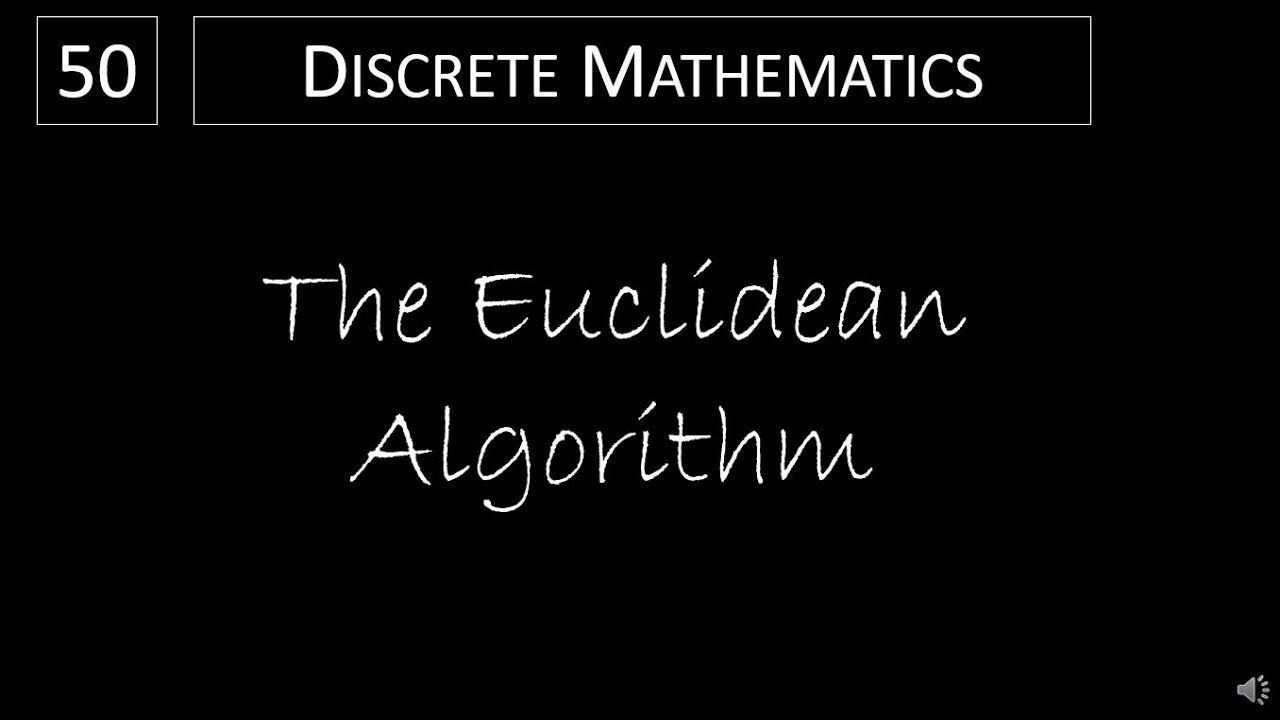
Discrete Math - 4.3.3 The Euclidean Algorithm

TEORI BILANGAN BULAT-PART.1 (MATEMATIKA DISKRIT-PART.5)
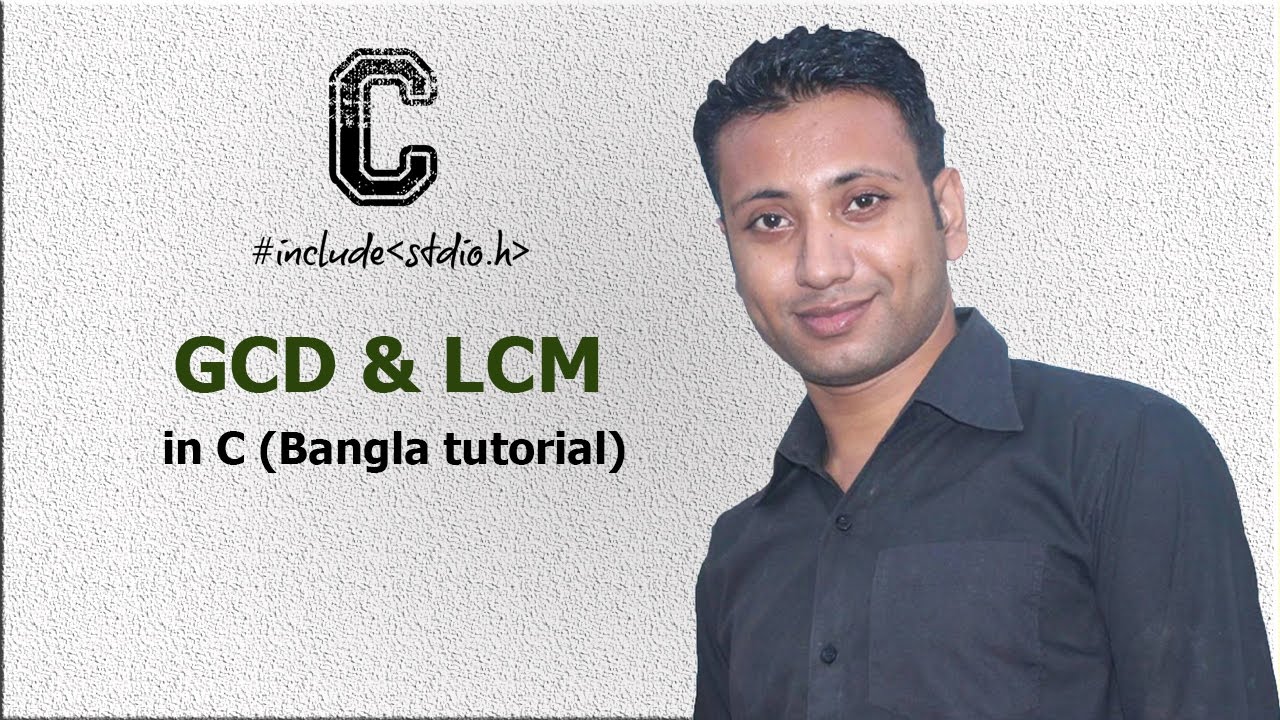
C programming Bangla Tutorial 5.106 : GCD(গসাগু) ও LCM(লসাগু) নির্ণয়ের জন্য Algorithm, Flowchart, C

Extended Euclidean Algorithm and Inverse Modulo Tutorial

Contracting the Extended Euclidean Algorithm (Proof)
5.0 / 5 (0 votes)