Methods in Java
Summary
TLDRThis video provides a detailed exploration of Java methods, covering key concepts like void and value-returning methods. It explains how void methods perform actions without returning values, while value-returning methods output results using the `return` keyword. The video also discusses how to call methods, pass parameters, and handle errors related to incompatible return types. Practical examples are provided to demonstrate how methods are structured, invoked, and how the `return` keyword functions in various contexts. Ideal for beginners, this video offers a solid foundation in understanding Java methods and their application.
Takeaways
- ๐ Void methods do not return a value and their return type is 'void'.
- ๐ The 'public' keyword makes a method accessible from outside its class, while the 'static' keyword allows it to be called from the 'main' method.
- ๐ A method header includes modifiers (e.g., 'public', 'static'), the return type, method name, and parameters.
- ๐ The method body contains the code executed when the method is called.
- ๐ Void methods can be called from other methods, like the 'main' method, where their execution temporarily halts the caller method until finished.
- ๐ Value-returning methods must specify a return type that matches the type of the value they return.
- ๐ The 'return' keyword is used to return a value from a method, ending the method's execution at that point.
- ๐ When a value-returning method is called, the return value can be assigned to a variable or used directly in expressions.
- ๐ Unreachable code occurs if statements after a 'return' keyword in the same method are never executed.
- ๐ Returning a value incompatible with a method's return type will result in an error (e.g., returning an integer from a void method).
- ๐ Method parameters are local variables within the method and must be provided with the correct type and order when calling the method.
Q & A
What is a void method in Java?
-A void method is a method that does not return any value. Its return type is declared as 'void'. The method can execute tasks like printing messages or performing actions, but it does not send any data back to the caller.
How do you define a void method in Java?
-To define a void method in Java, use the 'public' and 'static' modifiers followed by the return type 'void', the method name, and parentheses. Example: `public static void sayHi()`.
Can a void method take parameters?
-Yes, a void method can take parameters. Parameters are specified within the parentheses in the method definition. However, even though the method may accept parameters, it does not return a value.
What happens when a void method is called in Java?
-When a void method is called, the program temporarily exits the calling method (e.g., the main method) and executes the code inside the void method's body. Once the void method finishes executing, the program resumes execution from the point where the void method was called.
What is the role of the 'return' keyword in a void method?
-In a void method, the 'return' keyword is used to exit the method early. It does not return any value but causes the method to stop executing further code and return control to the calling method.
What is the difference between a void method and a value-returning method in Java?
-A void method does not return any value (its return type is 'void'), while a value-returning method returns a value of a specified type. A value-returning method uses the 'return' keyword to send a value back to the caller.
How do you define a value-returning method in Java?
-To define a value-returning method, use the desired return type (such as 'int', 'double', etc.), followed by the 'public static' modifiers, the method name, and parameters. The method should return a value using the 'return' keyword.
What happens if a value-returning method is called but the return value is not used?
-If the return value of a value-returning method is not used, the method will still perform its calculations, but the returned value is discarded. For example, if the return value is not assigned to a variable or printed, it will be ignored.
What happens if you place code after a 'return' statement in a method?
-Code placed after a 'return' statement in a method is considered unreachable. This will result in a compilation error, as the program will never execute that part of the code after the method has returned a value or exited.
Why is it important to match the return type with the data being returned in a method?
-It is important to match the return type with the data being returned to avoid compilation errors. If a method is defined to return a certain type (e.g., 'int'), returning a different type (e.g., 'String') will cause a type mismatch and lead to an error.
Outlines
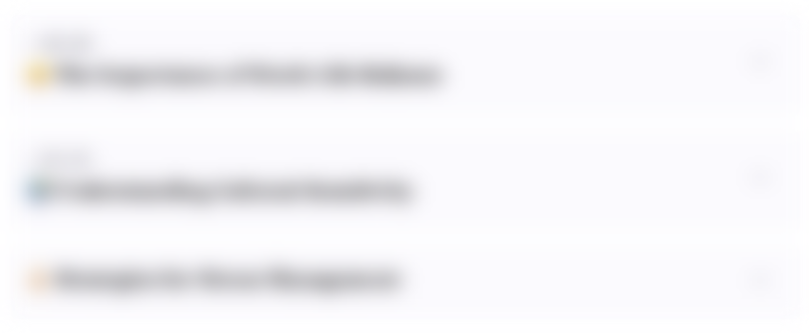
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
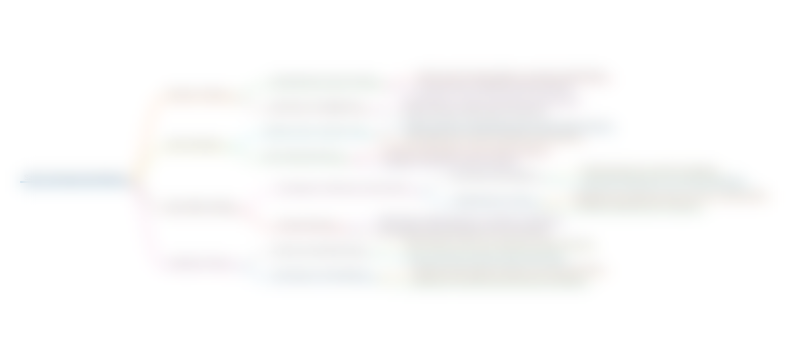
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
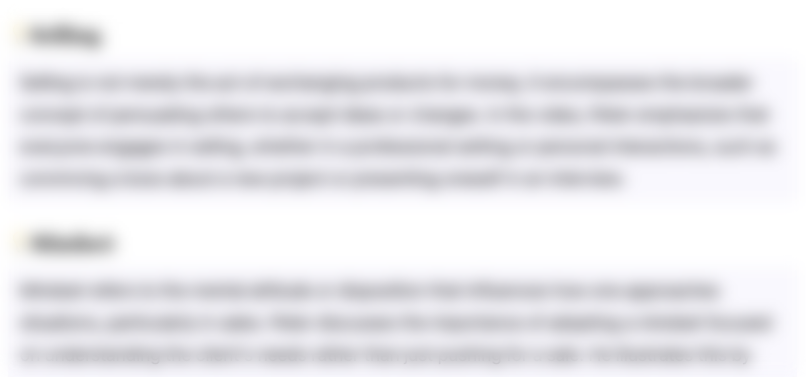
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
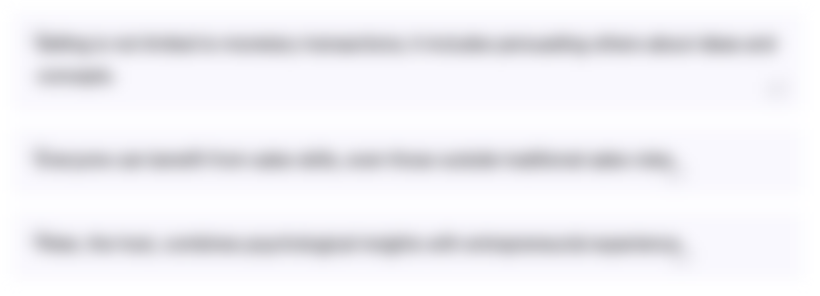
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
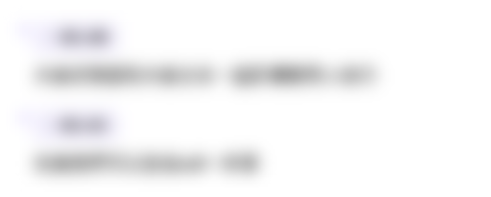
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

EKMA4216 Manajemen Pemasaran - Ruang Lingkup Manajemen Pemasaran
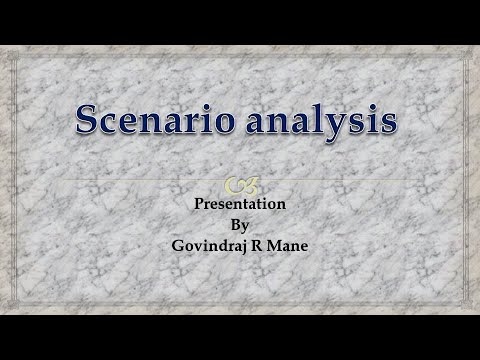
How to analyze the risk using Scenario Analysis Techniques
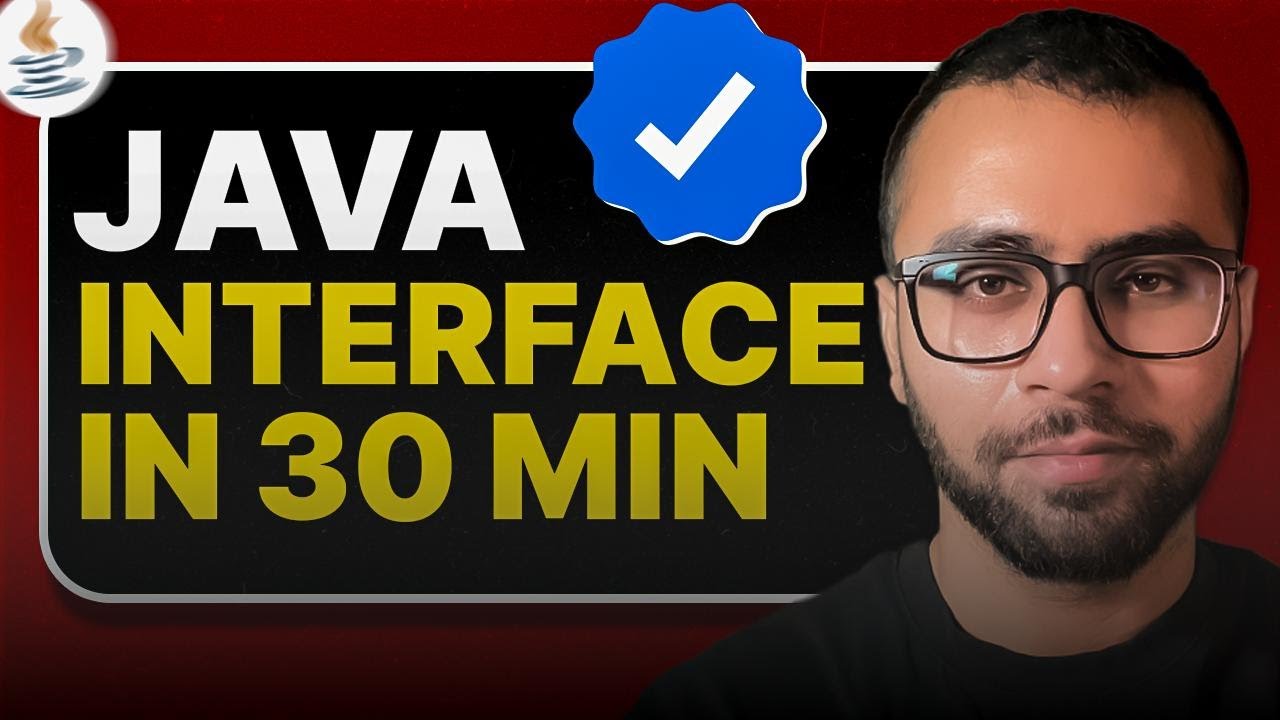
Mastering Java Interfaces: Static & Default Methods, Multiple Inheritance Explained
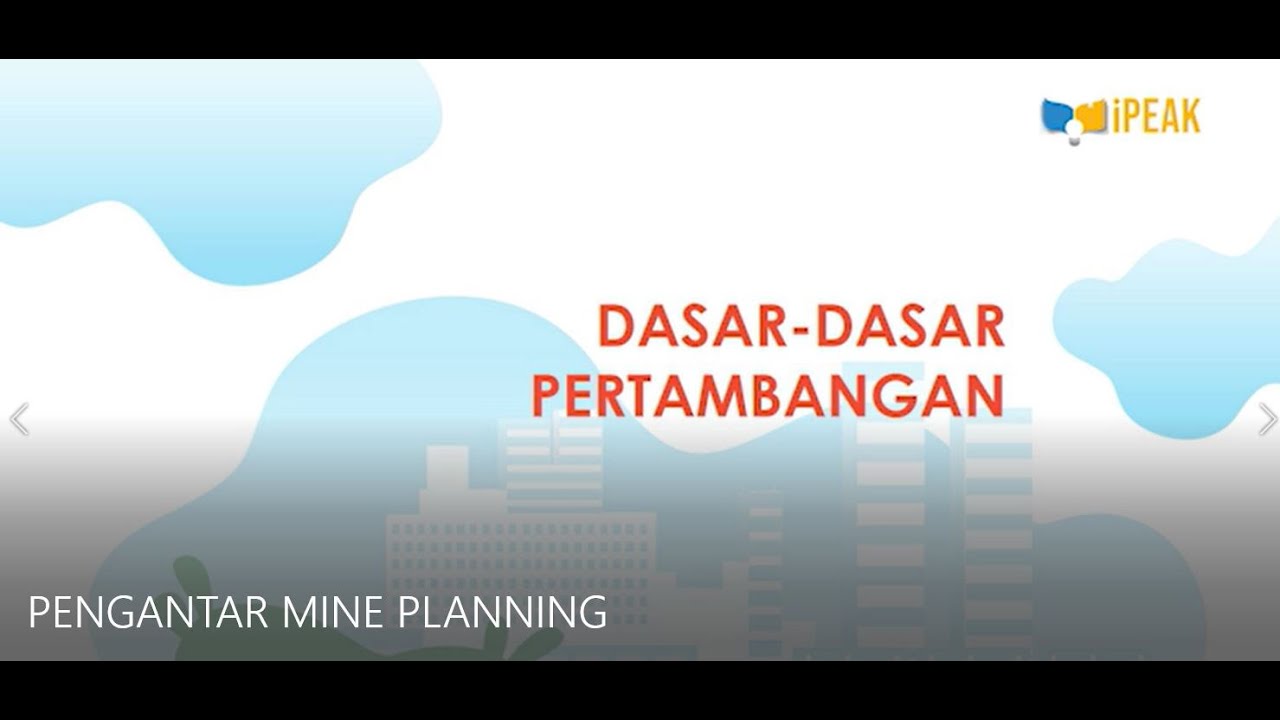
PENGANTAR MINE PLANNING
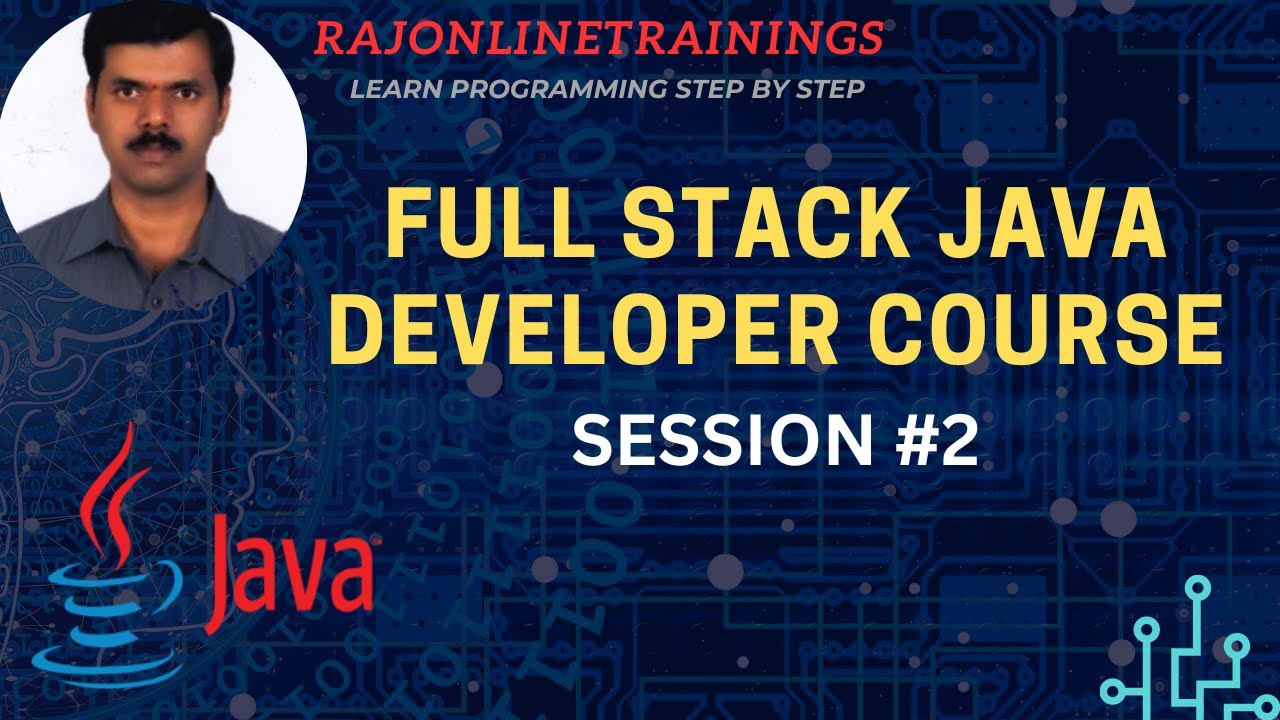
Full Stack Java Developer Course | Session - 2 | Programming Concepts | rajonlinetrainings
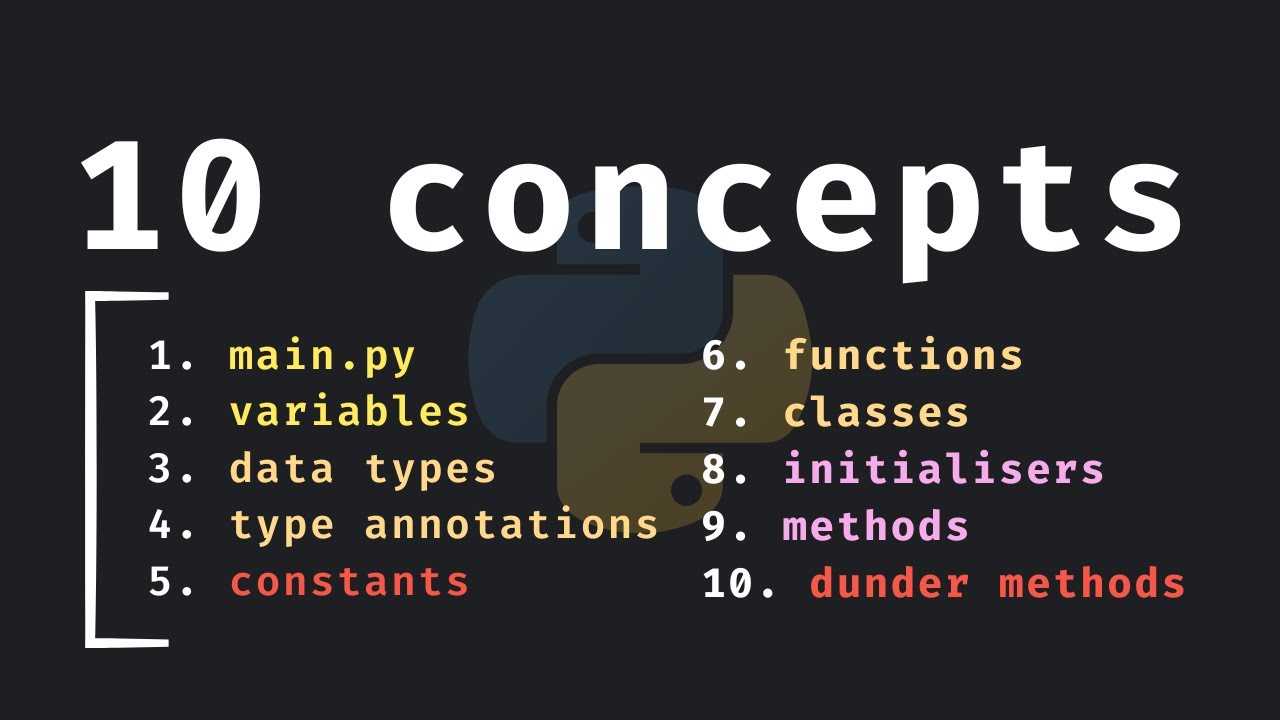
10 Important Python Concepts In 20 Minutes
5.0 / 5 (0 votes)