Handling Permission in Flutter Apps | Permission Handlers | The right way to check for permissions
Summary
TLDRThis FL tutorial explains how to handle permissions in a Flutter app using the 'permission_handler' package. The video demonstrates how to request and manage permissions for accessing device-specific APIs, such as the camera or gallery. It covers how to track permission status, display alerts, and guide users to system settings to modify denied permissions. The tutorial walks through implementing this feature, handling exceptions, and ensuring a smooth user experience by rendering the appropriate UI components based on permission status.
Takeaways
- ๐ฑ The tutorial focuses on handling permissions in a Flutter app, specifically for accessing device-specific APIs like the camera, microphone, and gallery.
- ๐ The 'permission_handler' package is introduced as a tool to manage permission requests and track their status across Android and iOS platforms.
- ๐ธ An example is provided where the app uses the 'permission_handler' to access the device's gallery, with an elevated button to trigger the permission request.
- ๐ซ If the user denies the permission request, the app uses the 'permission_handler' to check the status and displays an alert dialog to educate the user about the denied permission.
- ๐ The tutorial demonstrates how to handle exceptions that occur when a permission is denied, using a try-catch block to manage the flow of the app.
- ๐ ๏ธ The 'image_picker' package is used in conjunction with 'permission_handler' to open the device gallery and select a photo.
- ๐ The tutorial guides through adding dependencies in the 'pubspec.yaml' file, which are essential for image picking and permission handling.
- ๐ It emphasizes the importance of informing users when a permission is denied and providing options to change the permission settings manually.
- ๐ The 'open_app_settings' method from the 'permission_handler' package is highlighted as a way to direct users to the app's settings page to modify permissions.
- ๐ป The tutorial concludes with a coding segment that implements the discussed features, enhancing the app's user interface based on permission status.
- ๐ฅ The video script is designed to help developers create more intuitive apps by effectively managing permissions and providing a better user experience.
Q & A
What is the purpose of the 'permission_handler' package in Flutter?
-The 'permission_handler' package is used to manage device-specific permissions, such as access to the camera, microphone, or gallery. It raises permission requests and tracks their status, allowing developers to control app behavior based on the user's response.
How does the 'permission_handler' package help in handling denied permissions?
-When a permission is denied, the 'permission_handler' package keeps track of the permission status and can notify the user. It allows the app to show a dialog informing the user that the permission was denied and gives an option to open system settings to manually change the permission.
What happens when a user denies a permission in a Flutter app using 'permission_handler'?
-If a user denies a permission, the app won't be able to access the requested device feature (like gallery or camera). The permission status is tracked, and the app can inform the user, providing an option to navigate to the system settings to change the permission manually.
What is the difference in app behavior when a permission is granted versus when it is denied?
-When permission is granted, the app follows a sequential flow, allowing access to the requested feature, such as opening the gallery. If permission is denied, the app will handle the exception by showing a dialog with options to open settings and change the permission.
How can developers prevent platform exceptions when a permission is denied?
-Developers can prevent platform exceptions by wrapping the permission request logic inside a try-catch block. This ensures that any permission denial is caught and handled gracefully without crashing the app.
What is the purpose of the 'Image Picker' package in the example?
-The 'Image Picker' package is used to open the device gallery and allow users to choose an image. It works in conjunction with the 'permission_handler' package to request access to the gallery and handle permissions.
How does the app notify the user when a permission is denied?
-When a permission is denied, the app uses an alert dialog to notify the user. The dialog includes information about the denied permission and offers options such as 'Cancel' or 'Settings' to allow the user to navigate to system settings and change the permission.
What role does the 'openAppSettings' method play in the permission flow?
-The 'openAppSettings' method allows the app to directly open the system settings, enabling the user to manually change the permission that was previously denied. This is helpful when the app needs access to a specific device feature.
What should developers add to the 'pubspec.yaml' file to implement the features discussed in the video?
-Developers should add the 'permission_handler' and 'image_picker' packages as dependencies in the 'pubspec.yaml' file to implement permission handling and image selection from the gallery.
How does the app behave after a denied permission is manually granted through system settings?
-Once the denied permission is manually granted through system settings, the app behaves as if the permission was granted initially. For instance, if the gallery permission was granted, the app will directly open the gallery the next time the user clicks the corresponding button.
Outlines
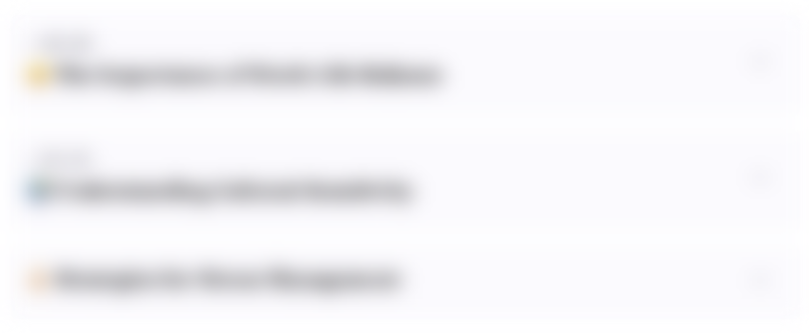
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
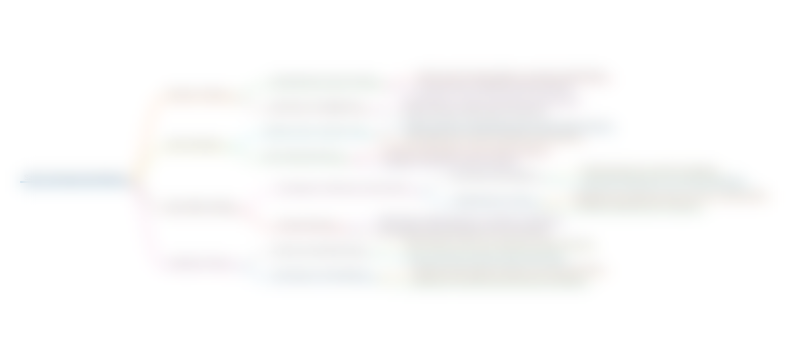
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
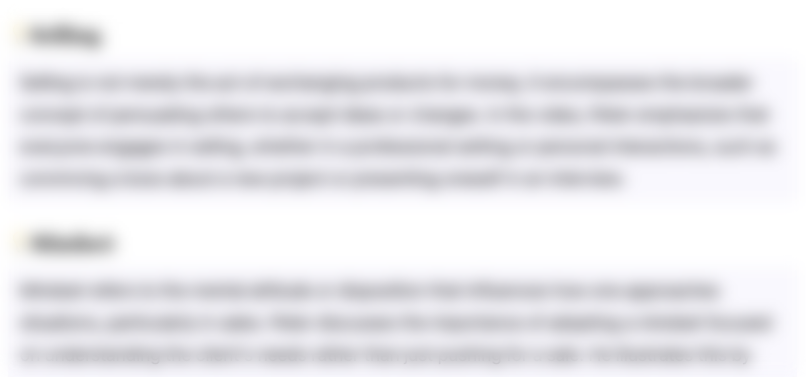
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
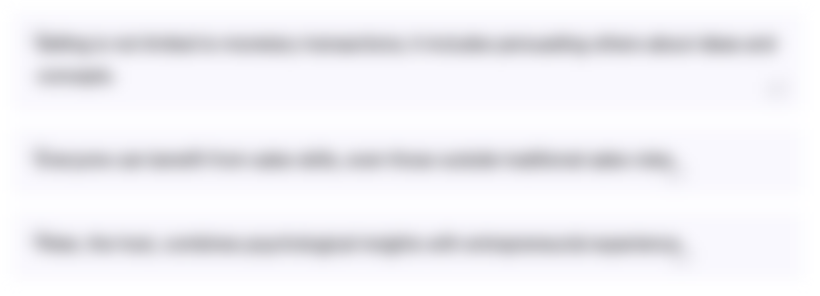
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
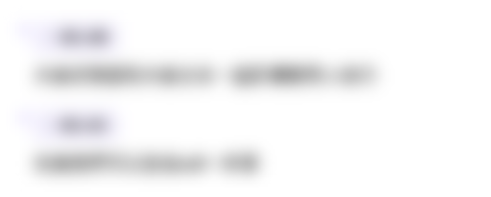
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)