#19 Understanding Directives | Angular Components & Directives | A Complete Angular Course
Summary
TLDRIn this lecture, the key concept of Angular directives is explained. Directives are instructions for manipulating the DOM, with three types: component directives (Angular components with templates), attribute directives (modifying the appearance or behavior of elements), and structural directives (adding or removing DOM elements). Examples include built-in directives like *ngIf, *ngFor, and custom attribute directives like changing background colors. The lecture emphasizes how directives are created using TypeScript classes and decorated with Angular's @Directive decorator. Future lessons will explore built-in directives in greater detail.
Takeaways
- 📦 Components are the basic building blocks of any Angular application.
- 📋 A directive is an instruction to the DOM, used to manipulate it in Angular.
- 🏗️ Directives can change the appearance, behavior, or layout of DOM elements.
- 📑 Angular directives are classified into three types: component directive, attribute directive, and structural directive.
- 🖼️ Component directives are Angular components, which come with a template and manipulate the DOM by rendering view templates.
- 🎨 Attribute directives change the appearance or behavior of a DOM element, like changing the background color, without adding or removing elements.
- 🛠️ Structural directives manipulate the DOM layout by adding or removing elements, using built-in directives like `ngIf`, `ngFor`, and `ngSwitch`.
- 💡 Structural directives are prefixed with an asterisk (*) to indicate DOM manipulation.
- 🏷️ Directives typically use attribute selectors but can also be configured as CSS class, HTML tag, or ID selectors.
- 🔧 A directive is essentially a TypeScript class decorated with a `@Directive` decorator to make it function as a directive in Angular.
Q & A
What is a directive in Angular?
-A directive in Angular is an instruction to the DOM, used to manipulate its behavior, appearance, or layout. Directives help Angular extend HTML and are a fundamental part of the framework.
What are the three types of directives in Angular?
-The three types of directives in Angular are component directives, attribute directives, and structural directives.
How is a component directive different from other directives?
-A component directive is a directive with a template, meaning it renders a view in the DOM. Other directives like attribute and structural directives do not have templates and manipulate DOM elements in different ways.
What is the purpose of an attribute directive?
-An attribute directive is used to change the appearance or behavior of a DOM element. It does not add or remove elements from the DOM but modifies the way an element behaves or looks.
Can you give an example of an attribute directive?
-An example of an attribute directive is a custom directive that changes the background color of an element, like `changeToGreen`. Angular also provides built-in attribute directives like `ngStyle` and `ngClass`.
What is a structural directive, and how does it differ from an attribute directive?
-A structural directive can change the layout of the DOM by adding or removing elements, unlike attribute directives which only modify existing elements. Examples of structural directives include `ngIf`, `ngFor`, and `ngSwitch`.
Why is an asterisk (*) used before structural directives in Angular?
-The asterisk before a structural directive, like `ngIf`, tells Angular that the directive will manipulate the DOM by adding or removing elements.
How is the selector of a directive different from that of a component?
-While a component's selector is typically used like an HTML tag, a directive’s selector is often used as an HTML attribute. However, both can also be configured to use CSS class selectors, tag selectors, or ID selectors.
What is the decorator used to define a directive in Angular?
-The `@Directive` decorator is used to define a directive in Angular. This decorator is applied to a TypeScript class, turning it into a directive.
How do structural directives manipulate the DOM?
-Structural directives manipulate the DOM by adding or removing elements based on specific conditions. For example, `ngIf` can add or remove elements based on a true or false condition.
Outlines
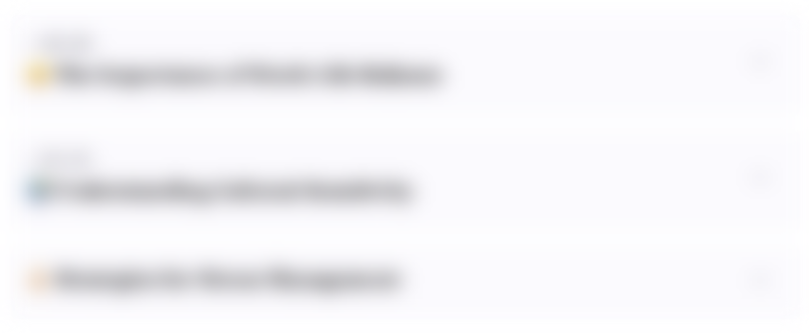
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
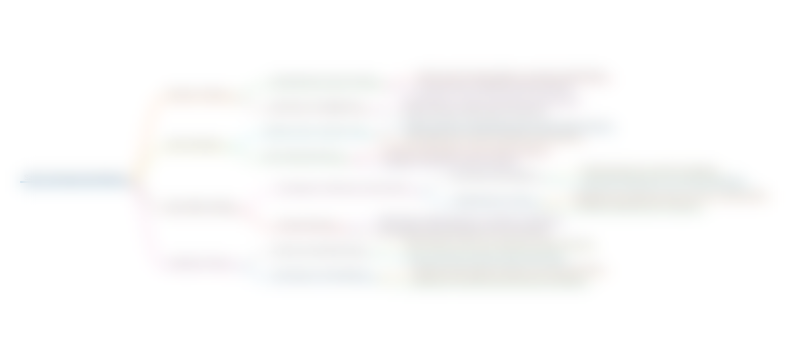
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
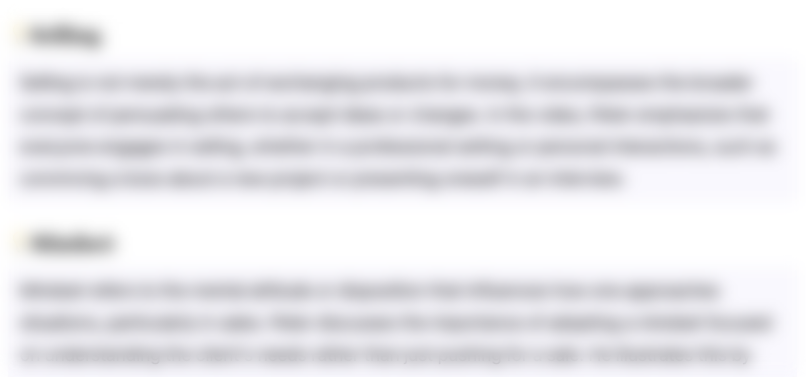
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
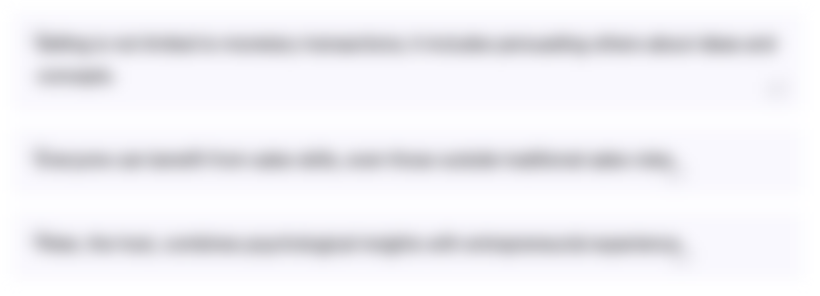
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
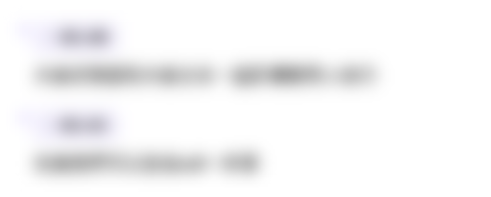
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Langage C #10 - préprocesseur
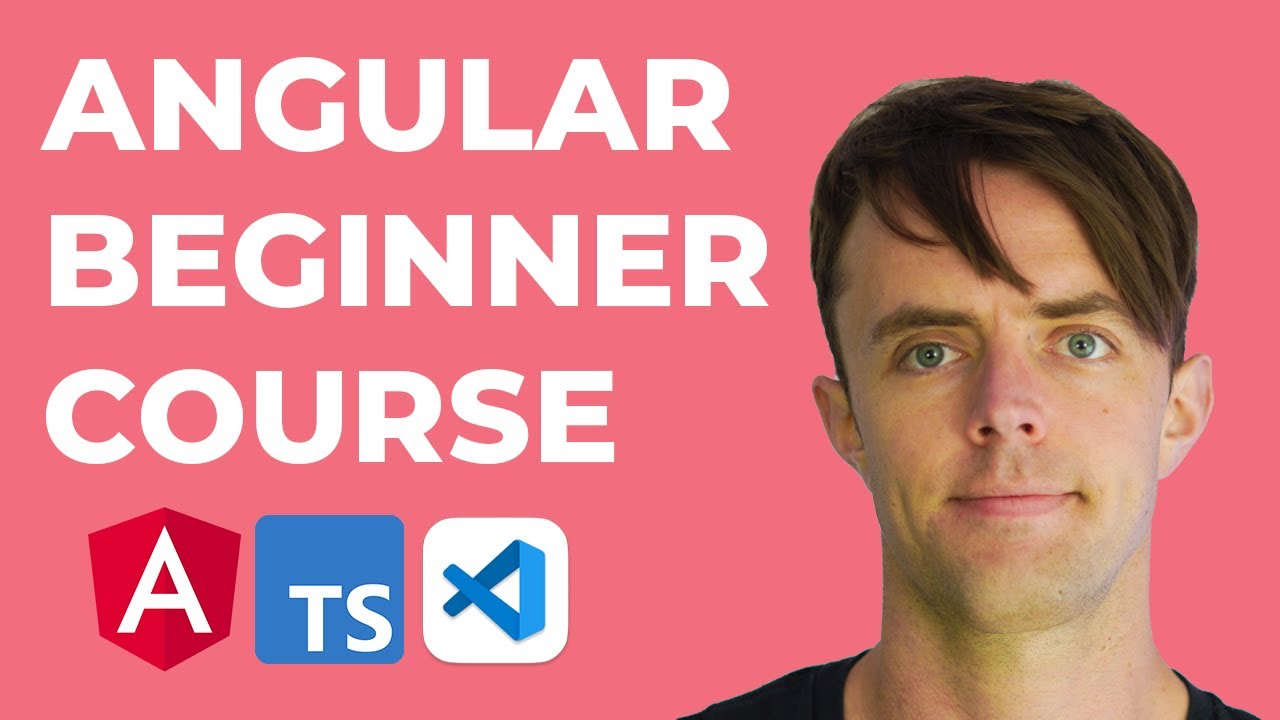
Angular Tutorial For Beginners 2022 - 1. Install & Folder Structure
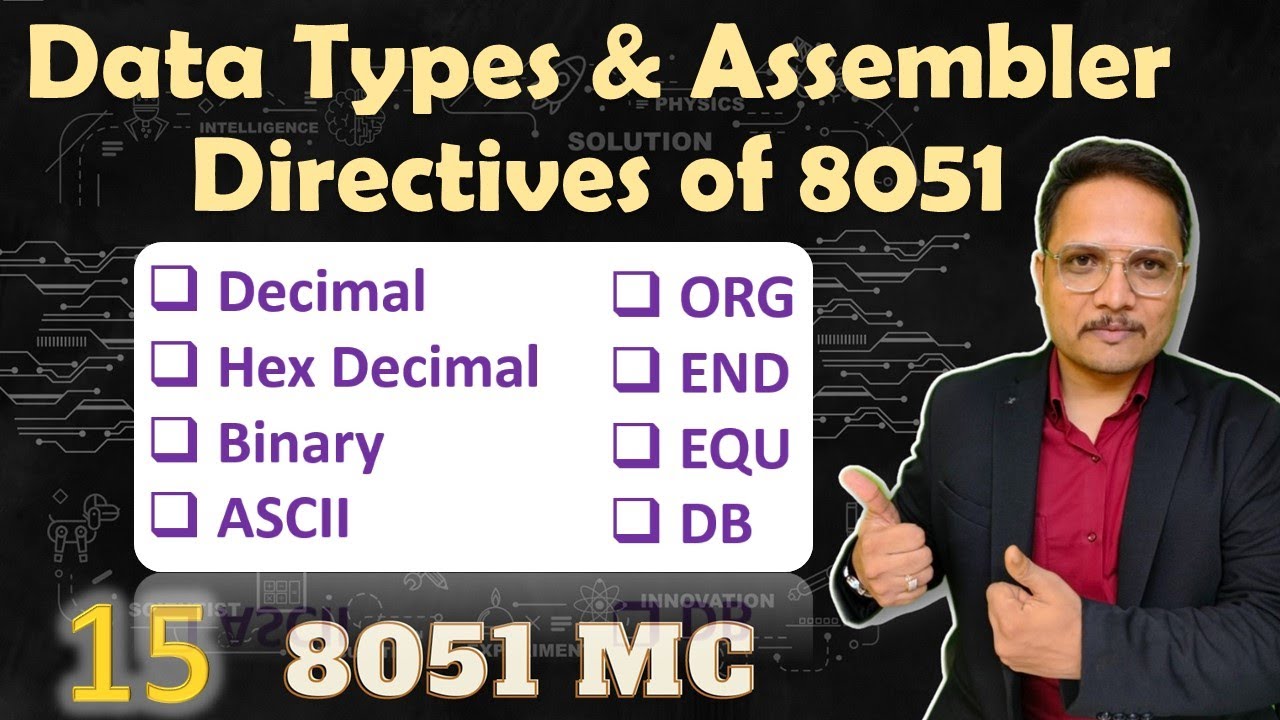
Data Types and Assembler Directives of 8051 Microcontroller | Data Types of 8051 | ORG | END | EQU

Writing Memos (COM1110 English Communication Skills)

Getting Started with Alpine.js
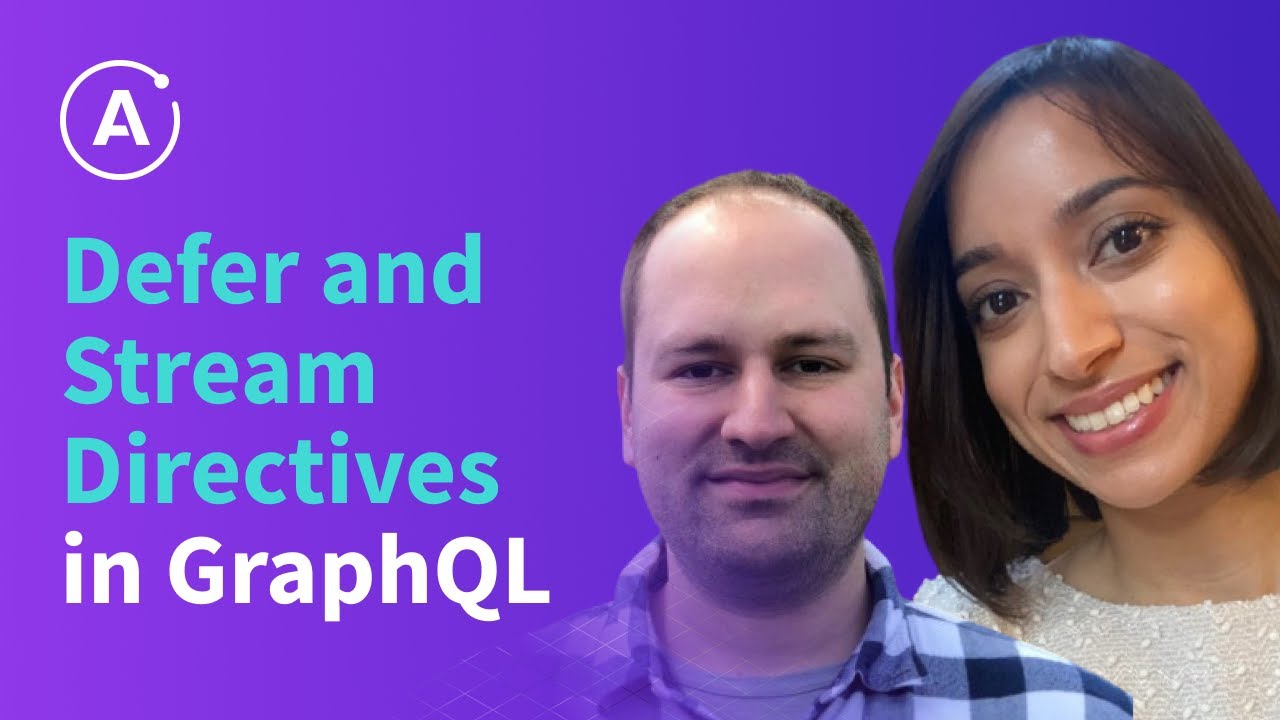
Defer and Stream Directives in GraphQL
5.0 / 5 (0 votes)