TypesNTypeConversion
Summary
TLDRThis screencast introduces fundamental concepts in Python programming, focusing on values and objects. It explains that everything in Python is an object, and these objects are categorized by classes or data types. The video demonstrates how to use the 'type' function to identify an object's class, such as 'str' for strings and 'int' for integers. It differentiates between integers and floating-point numbers, and shows how to create strings using single, double, or triple quotes. The lesson also covers type conversion functions like 'int()', 'float()', and 'str()' to change an object's data type, emphasizing the importance of using the correct syntax and avoiding errors.
Takeaways
- ๐ The fundamental concept introduced is 'value', which can be any data type like numbers or words, often referred to as objects in Python.
- ๐ Everything in Python is considered an object, emphasizing the object-oriented nature of the language.
- ๐ The 'type' function in Python is used to determine the data type of a variable or value.
- ๐ข Integers are whole numbers without decimal points, classified under the 'int' class in Python.
- ๐ Strings are sequences of characters and are created by enclosing them in single, double, or triple quotes.
- ๐ฏ The distinction between integers and floating-point numbers is that the latter includes decimal points.
- ๐ Strings can span multiple lines using triple quotes, which is useful for longer text or code that needs to be displayed as text.
- โ Commas are not allowed within strings as they are not legal in integer representations.
- ๐ Type conversion functions like `int()`, `float()`, and `str()` are used to change the data type of a value.
- โ ๏ธ When converting to an integer, the value must be numeric and cannot include any alphabetic characters or special characters.
- ๐ The script highlights the importance of understanding data types and type conversion for effective Python programming.
Q & A
What is a fundamental concept in Python programming discussed in the script?
-A fundamental concept in Python programming discussed in the script is the concept of 'value', which can be anything like a number or a word, often referred to as 'objects'.
What is the significance of the term 'object' in Python?
-In Python, the term 'object' is significant because everything in Python is considered an object, emphasizing the object-oriented nature of the language.
What are the interchangeable terms used to classify objects in Python?
-The interchangeable terms used to classify objects in Python are 'classes' and 'data types'.
How can you determine the type of a Python object?
-You can determine the type of a Python object by using the 'type' function, which directly tells you the class or data type of the object.
What is the difference between integers and floating-point numbers in Python?
-In Python, integers are whole numbers without decimal points, while floating-point numbers include decimal points, representing real numbers.
How do you create a string in Python?
-In Python, you create a string by surrounding text with quotation marks. You can use single quotes, double quotes, or triple quotes for this purpose.
Why might you use triple quotes when creating a string in Python?
-You might use triple quotes to create a string in Python when you want the string to span multiple lines or include both single and double quotes within the string.
What is the purpose of the 'int', 'float', and 'str' functions in Python?
-The 'int', 'float', and 'str' functions in Python are used for type conversion, allowing you to convert values to integers, floating-point numbers, and strings, respectively.
What happens when you try to convert a non-numeric string to an integer in Python?
-Attempting to convert a non-numeric string to an integer in Python results in an error because the conversion requires the string to contain only numeric characters.
How does Python handle the conversion of floating-point numbers to integers?
-When converting floating-point numbers to integers in Python, the number is rounded down to the nearest whole number using the 'int' function.
What is the importance of not using commas in strings in Python?
-Using commas in strings in Python is not allowed as it can lead to syntax errors or misinterpretation of the string, as commas are not valid characters within string literals.
Outlines
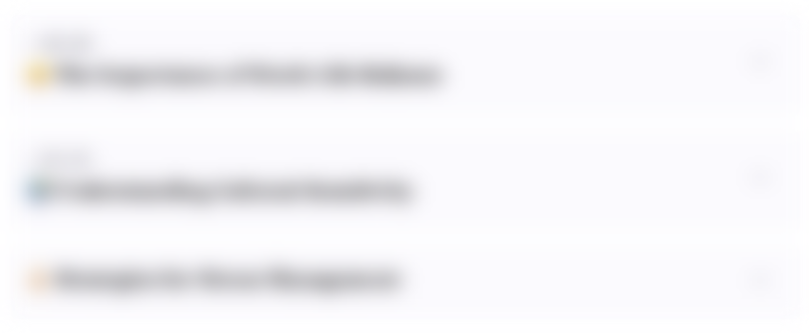
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
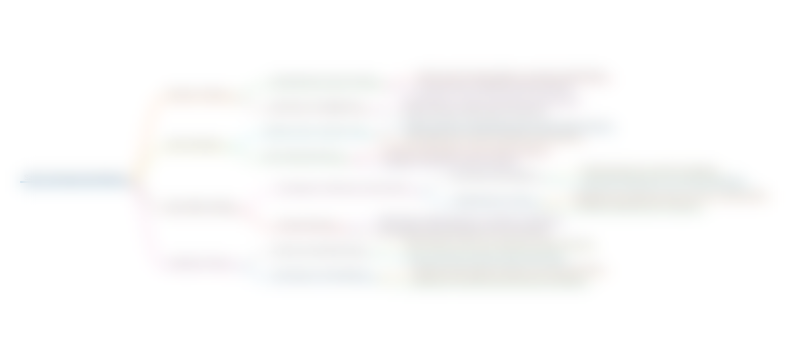
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
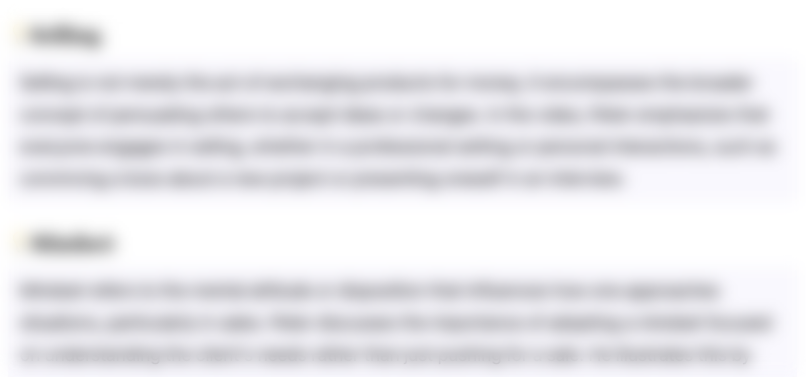
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
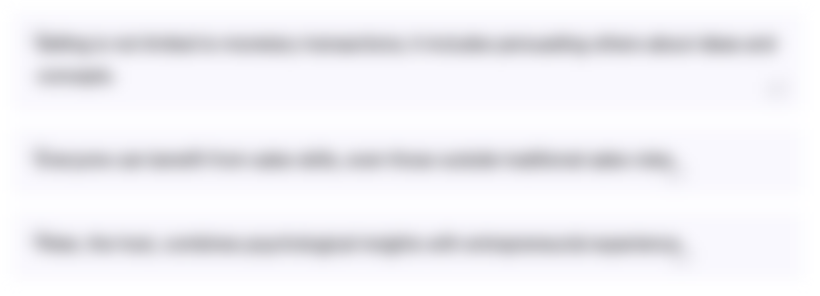
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
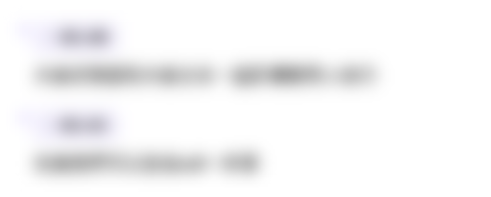
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)