OOPS Interview Questions and Answers | Object Oriented Programming Interview Questions C#
Summary
TLDRThis video script offers an in-depth exploration of Object-Oriented Programming (OOP) concepts, focusing on interview questions and answers using C#. It emphasizes the importance of understanding OOP for IT professionals, covering key topics like classes, objects, abstraction, encapsulation, inheritance, polymorphism, and interfaces. The script provides practical examples and advice for interviews, highlighting the need for preparedness, clear and concise answers, and the use of real-world scenarios over clichรฉd examples.
Takeaways
- ๐ฏ Object-oriented programming (OOP) concepts are crucial for IT interviews, regardless of the programming language you know.
- ๐ Importance of OOP lies in its pillars: Abstraction, Encapsulation, Inheritance, and Polymorphism, which are essential for developers to understand.
- ๐ก Abstraction in OOP means representing real-world objects in code, which helps in organizing and managing code more effectively.
- ๐ During interviews, it's advised to provide direct answers and support theoretical explanations with practical examples from your experience.
- ๐ซ Avoid using clichรฉ examples like cars or dogs; instead, use domain-specific examples relevant to the job or industry you're targeting.
- ๐ A class is a blueprint or template for creating objects, and an object is an instance of a class with specific values assigned to its properties.
- ๐ Abstraction is about showing only the necessary details to the outside world, while Encapsulation is about hiding the complexity and internal workings of a class.
- ๐งฌ Inheritance in OOP allows a class (child) to inherit properties and methods from another class (parent), promoting code reusability and establishing a hierarchy.
- ๐ Polymorphism enables an object to take on many forms or act differently under different conditions, which requires inheritance to be implemented.
- ๐ ๏ธ Method overloading and method overriding are distinct; overloading involves multiple methods with the same name but different signatures within the same class, while overriding involves a child class providing a new implementation for a virtual method of its parent class.
- ๐ Operator overloading allows customizing the behavior of operators for class instances, enhancing the flexibility and expressiveness of the code.
Q & A
Why is understanding Object Oriented Programming (OOP) concepts crucial for IT programming interviews?
-Understanding OOP concepts is crucial because OOP principles such as abstraction, encapsulation, inheritance, and polymorphism are widely used in software development. Interviewers expect candidates to demonstrate a clear grasp of these concepts, as they are foundational to designing and implementing efficient, maintainable, and scalable software systems.
What is the significance of thinking in terms of real-world objects in OOP?
-Thinking in terms of real-world objects allows developers to model software solutions based on tangible entities and their interactions, leading to more organized, manageable, and understandable code. This approach enhances the maintainability and scalability of software systems by mirroring real-world scenarios within the codebase.
What are the four pillars of OOP and how do they contribute to robust software design?
-The four pillars of OOP are Abstraction, Polymorphism, Inheritance, and Encapsulation. Abstraction allows hiding of unnecessary details and focusing on essential features. Polymorphism enables objects to behave differently under varying conditions. Inheritance promotes code reusability and establishes a hierarchy of classes. Encapsulation ensures that the internal state of an object is protected from external interference. Together, these principles lead to the creation of solid, flexible, and easily maintainable software.
How does the use of practical examples during an interview demonstrate a candidate's understanding and experience with OOP?
-Using practical examples in an interview shows that a candidate can apply theoretical OOP concepts to real-world scenarios. It indicates hands-on experience with enterprise applications and the ability to communicate complex ideas effectively, which are critical skills for software development roles.
What is the difference between Abstraction and Encapsulation in OOP?
-Abstraction is the process of simplifying complex reality by selecting only the essential features that are relevant to the problem at hand. Encapsulation is the mechanism of hiding the internal state and functionality of an object from the outside world. While both focus on managing complexity, abstraction is a design concept that decides what gets exposed, and encapsulation is an implementation concept that enforces this design by using access modifiers like private and public.
Explain the concept of Inheritance in OOP and its benefits.
-Inheritance in OOP is a mechanism that allows a class (child) to inherit properties and methods from another class (parent), promoting code reusability and establishing a hierarchical relationship between classes. This leads to reduced redundancy, easier maintenance, and a more organized codebase as common functionality can be defined once in the parent class and extended or modified by child classes as needed.
What is the purpose of the 'virtual' keyword in C# and how does it relate to method overriding?
-The 'virtual' keyword in C# is used to indicate that a method or property in a base class can be overridden by a derived class. This provides a mechanism for runtime polymorphism, allowing derived classes to provide their own implementation of a member that is already defined in the base class, thus enabling flexible and dynamic behavior changes based on the object's actual type at runtime.
How does method overloading differ from method overriding in OOP?
-Method overloading refers to the ability of a class to have multiple methods with the same name but different signatures. It is a form of compile-time polymorphism where the appropriate method to call is determined based on the number and types of arguments passed. Method overriding, on the other hand, is a form of runtime polymorphism where a derived class provides its own implementation for a method that is already defined in its base class, allowing for different behavior depending on the actual object type.
What is polymorphism in OOP and why is it necessary to have inheritance for implementing polymorphism?
-Polymorphism in OOP is the ability of an object to take on many forms, or in this context, to behave differently under different conditions. It is necessary to have inheritance for implementing polymorphism because it establishes a parent-child relationship that allows a child class object to be treated as an object of its parent class. This enables the runtime determination of which method implementation to invoke based on the actual object type, leading to flexible and reusable code.
What are the two types of polymorphism in C# and how are they implemented?
-The two types of polymorphism in C# are static (or compile-time) polymorphism and dynamic (or runtime) polymorphism. Static polymorphism is implemented through method overloading, where multiple methods with the same name but different signatures exist within the same class. Dynamic polymorphism is implemented through method overriding, where a derived class provides a new implementation for a virtual method inherited from its base class.
Explain the concept of operator overloading in OOP and how it is used in C#.
-Operator overloading in OOP is the ability to redefine the behavior of existing operators such as addition, subtraction, or concatenation, for custom classes. In C#, this is achieved using the 'operator' keyword followed by the operator symbol and a method definition that specifies the custom logic for the operator. This allows developers to define how objects of their class interact with operators, enabling custom behaviors without changing the fundamental syntax of the language.
What is an abstract class in OOP and why can't instances of it be created?
-An abstract class in OOP is a partially completed class that serves as a base for other classes. It often contains both implemented and abstract methods. Instances of an abstract class cannot be created because it is meant to be inherited and extended by other classes. By preventing the instantiation of abstract classes, it ensures that the class is only used as a base and that its abstract methods are implemented by derived classes, providing a clear structure and preventing misuse.
Outlines
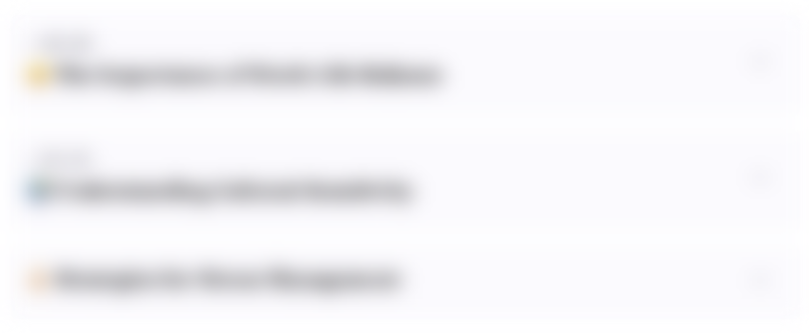
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
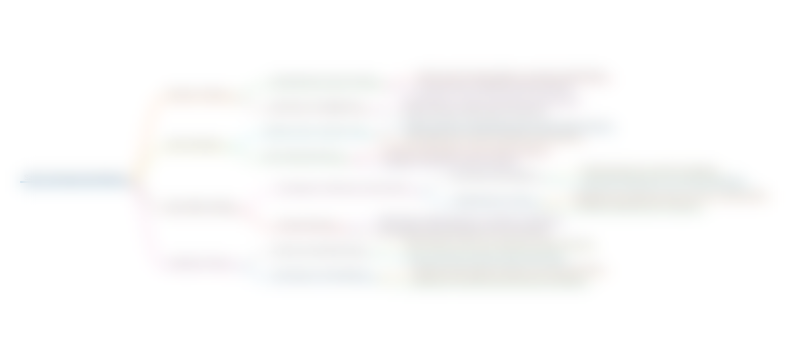
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
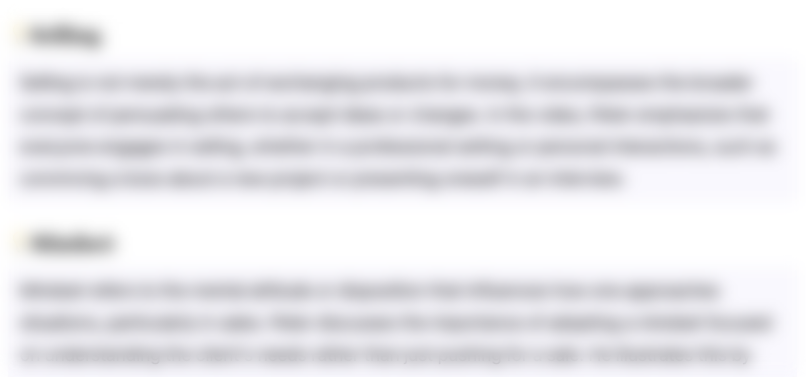
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
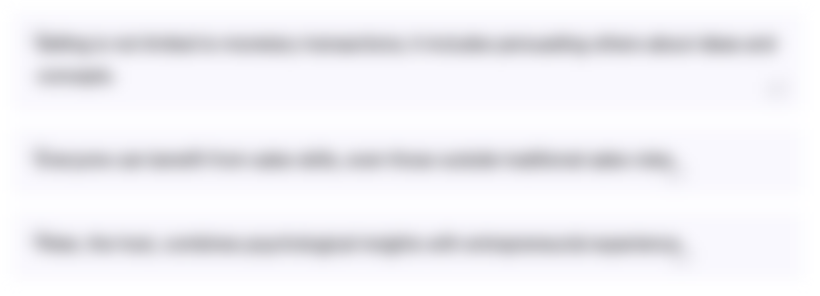
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
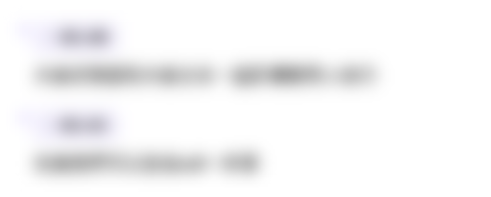
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
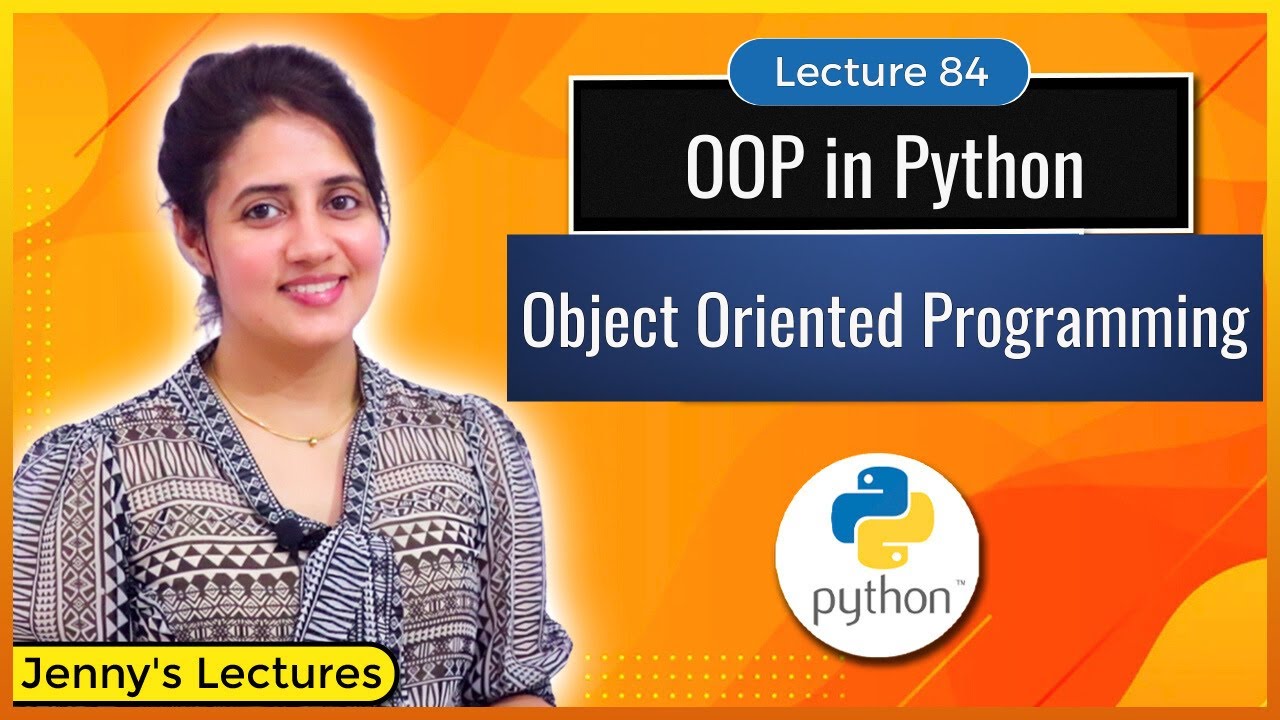
OOP in Python | Object Oriented Programming | Python for Beginners #lec84
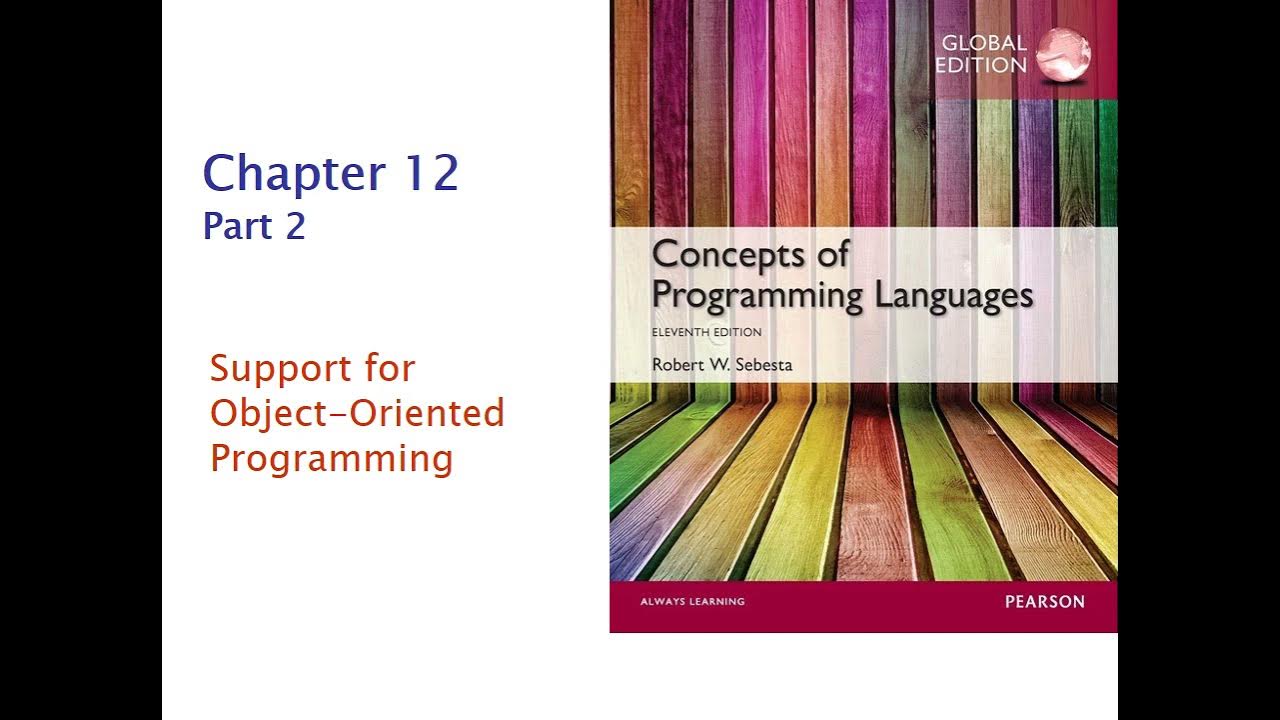
COS 333: Chapter 12, Part 2
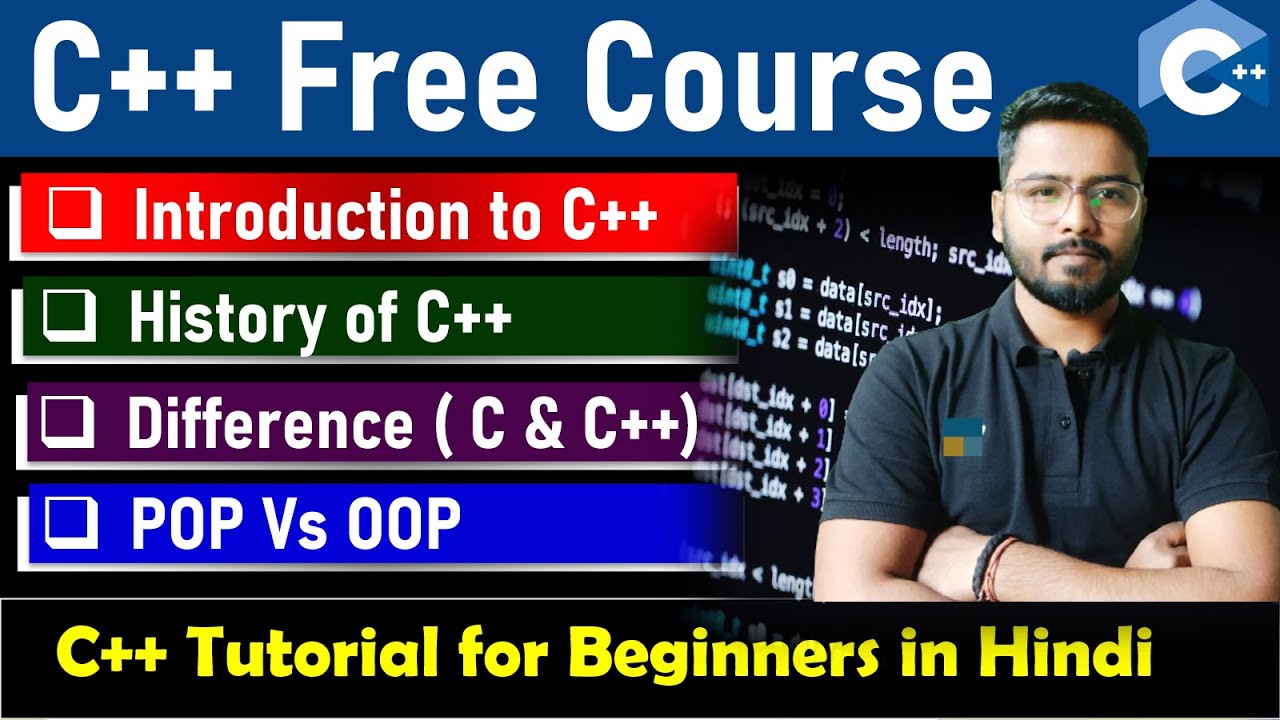
C++ Tutorial - Introduction to C++ | C++ Tutorial For Beginners #c++
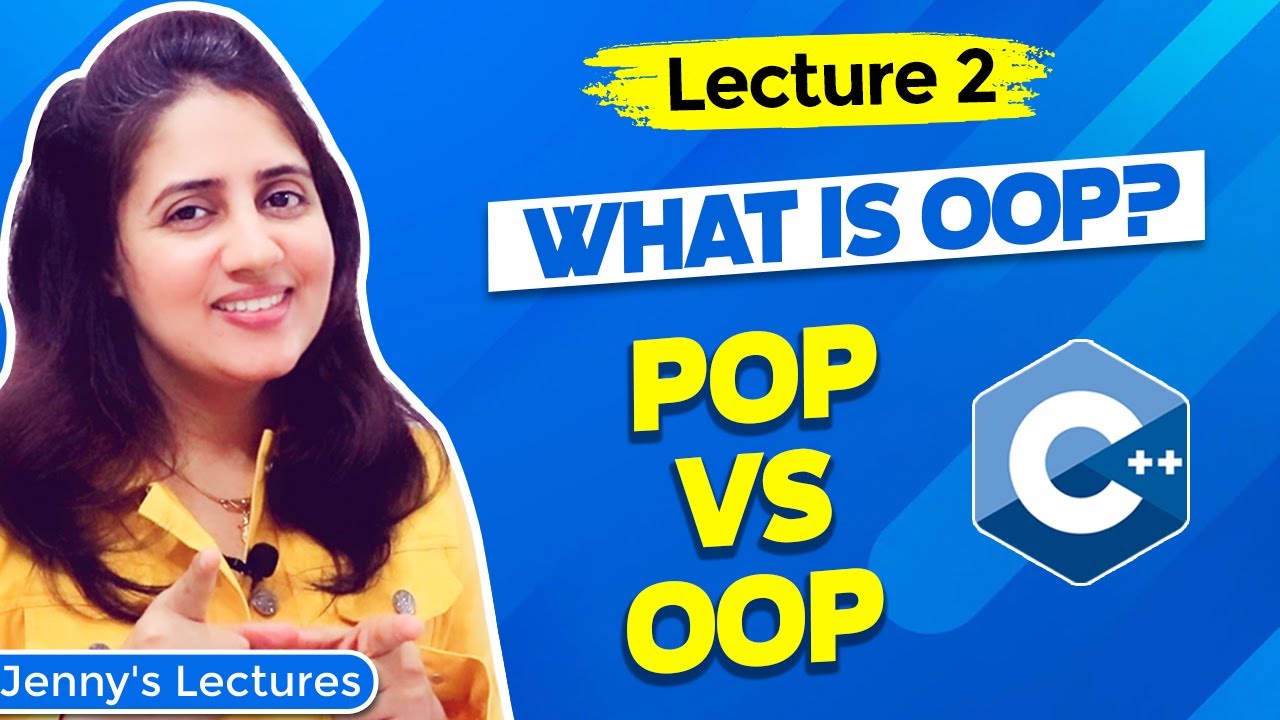
Lec 2: What is Object Oriented Programming (OOP) | POP vs OOP | C++ Tutorials for Beginners

KONSEP DASAR PEMROGRAMAN BERORIENTASI OBJEK

1.2 Pengantar Java
5.0 / 5 (0 votes)