Hibernate & JPA Tutorial - Crash Course
Summary
TLDRThe video script introduces the concepts of Hibernate and JPA, explaining their role in mapping Java classes to database tables and simplifying database operations. It emphasizes the importance of understanding these technologies through practice and provides a step-by-step guide on setting up Hibernate, using annotations for mapping, and executing basic CRUD operations. The script also touches on the use of HQL and JPQL for queries, the dynamic Criteria API, and the benefits of using IDE features and plugins to aid development. Finally, it briefly discusses how frameworks like Spring Boot and Spring Data simplify the use of JPA by handling infrastructure and providing repositories.
Takeaways
- 📚 Understanding Hibernate and JPA requires time and practice, with a focus on mastering core concepts rather than memorizing every feature.
- 🔍 Hibernate's primary function is to simplify the mapping between Java objects and relational databases, bridging the gap between object-oriented programming and SQL.
- 📈 The setup for Hibernate involves adding a single dependency (hibernate-core) and configuring it through a hibernate.cfg.xml or equivalent configuration file.
- 🏷️ Annotations are used in Java classes to map database tables and columns to Java objects, with @Entity, @Table, @Id, and @GeneratedValue being some of the key Hibernate annotations.
- 💡 JPA (Java Persistence API) provides a standardized set of tools and interfaces for object-relational mapping that multiple providers, including Hibernate, implement.
- 🌐 HQL (Hibernate Query Language) and JPQL (Java Persistence Query Language) allow for the creation and execution of type-safe queries against the database using the object model, rather than raw SQL.
- 🔧 The Criteria API in Hibernate offers a way to build dynamic SQL queries in a type-safe manner, without the need for string concatenation.
- 🛠️ IDEs like IntelliJ IDEA Ultimate offer features that can greatly assist in working with Hibernate and JPA, such as autocompletion, validation, and running queries directly from the IDE.
- 📚 Books like 'Java Persistence with Hibernate' provide in-depth knowledge and examples for mastering Hibernate and JPA, and are recommended for further learning.
- 🏗️ Using frameworks like Spring Boot and Spring Data simplifies the use of JPA and Hibernate by handling much of the configuration and boilerplate code, allowing developers to focus on application logic.
- 📈 The transition from Hibernate to JPA-compliant code is straightforward, as many annotations and concepts are shared between the two, enabling developers to switch between providers if needed.
Q & A
What problem does Hibernate solve?
-Hibernate solves the problem of mapping and persisting data between a relational database and Java objects. It simplifies the process of saving and retrieving data from a database by eliminating the need for manual SQL queries and string concatenation.
What is the core benefit of using Hibernate over other ORM tools?
-The core benefit of using Hibernate is its ability to provide a simpler, more intuitive way to interact with databases through Java objects, reducing the complexity and effort required to manage database operations manually.
What is the significance of the @Entity annotation in Hibernate?
-The @Entity annotation in Hibernate marks a Java class as a mapped entity that corresponds to a database table. It tells Hibernate that this class is special and should be mapped to a database table.
How does the @Table annotation work in Hibernate?
-The @Table annotation is used to specify the table name in the database to which the Hibernate-annotated class should be mapped. By default, Hibernate assumes the table name is the same as the class name, but with the @Table annotation, you can explicitly define a different table name.
What is the role of the @Id and @GeneratedValue annotations in Hibernate?
-The @Id annotation designates a field as the primary key of the entity, while the @GeneratedValue annotation indicates that the value of the primary key field should be generated by the database, such as through an auto-increment mechanism.
Why is it important to have an empty constructor in a Hibernate-annotated class?
-An empty constructor is important in a Hibernate-annotated class because it allows Hibernate to instantiate the class and manage its state, which is necessary when fetching and populating objects from the database.
What is the purpose of the Hibernate configuration file (hibernate.cfg.xml)?
-The Hibernate configuration file (hibernate.cfg.xml) is used to provide Hibernate with the necessary configuration settings, such as the database connection details, SQL dialect, and other properties required to establish a connection and interact with the database.
How does the SessionFactory in Hibernate work?
-The SessionFactory in Hibernate is responsible for creating Session objects, which represent a single database session. It is the entry point for all database operations and is typically configured through the hibernate.cfg.xml file.
What is the difference between HQL and JPQL?
-HQL (Hibernate Query Language) is specific to Hibernate and allows you to write type-safe queries against the Hibernate entity model. JPQL (Java Persistence Query Language), on the other hand, is a standardized query language for the JPA specification and is more portable across different JPA providers.
What does the Criteria API in Hibernate offer?
-The Criteria API in Hibernate provides a way to construct type-safe, dynamic queries using a fluent interface. It allows developers to build complex queries without the need for string concatenation or manual SQL, making the code more maintainable and less prone to errors.
How can IDEs like IntelliJ IDEA Ultimate enhance the development experience with Hibernate and JPA?
-IDEs like IntelliJ IDEA Ultimate offer features that can greatly enhance the development experience with Hibernate and JPA. These features include automatic code completion for entity fields, error highlighting for missing annotations, and the ability to run queries directly from the IDE. Additionally, plugins like JPA Buddy can further streamline the process of creating entities and managing mappings.
How does Spring Boot simplify the use of JPA and Hibernate?
-Spring Boot simplifies the use of JPA and Hibernate by automatically creating an EntityManager and EntityManagerFactory based on the properties defined in the application.properties file. It also handles transaction management through the @Transactional annotation and provides a repository-based approach for data access, allowing developers to write simple interfaces that Spring Data will implement with the appropriate Hibernate/JPA code.
Outlines
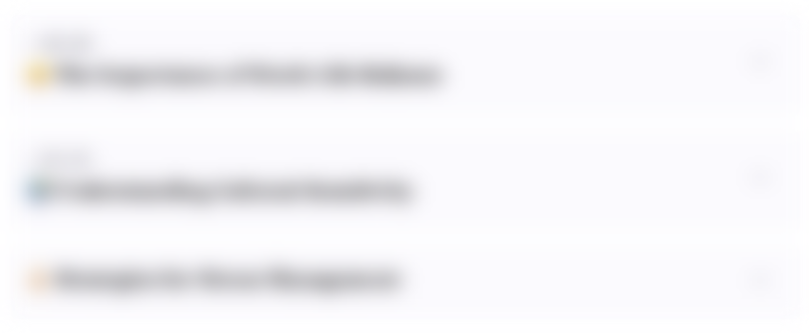
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
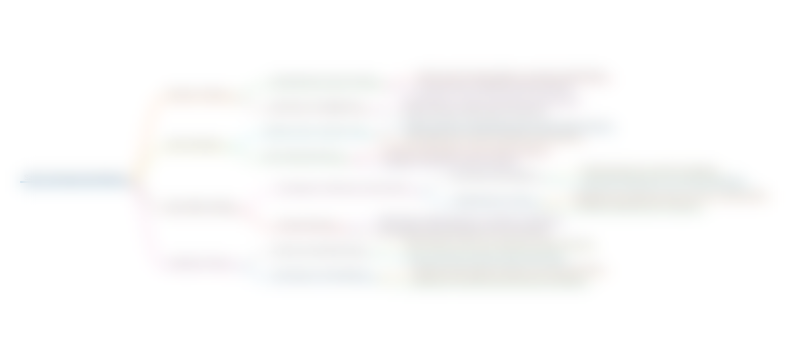
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
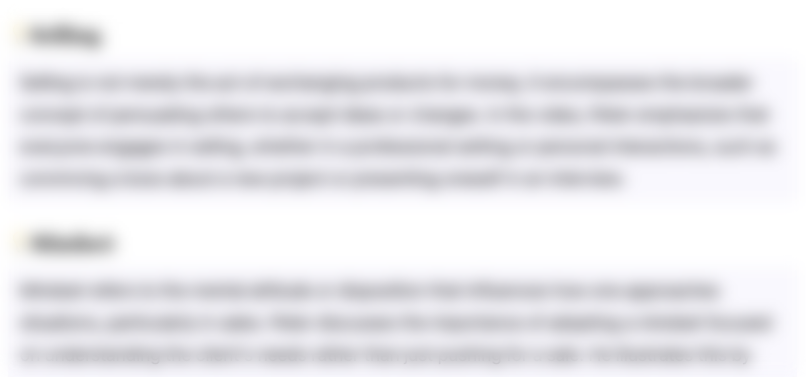
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
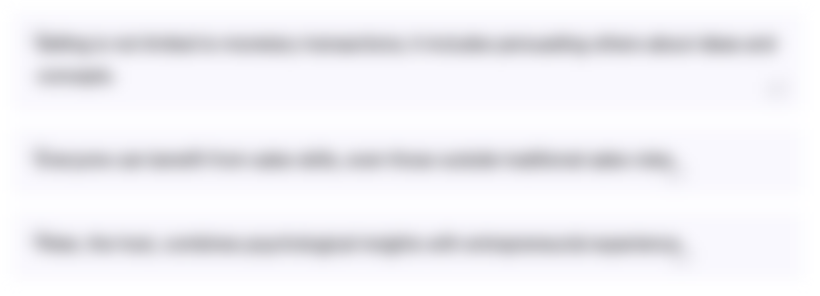
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
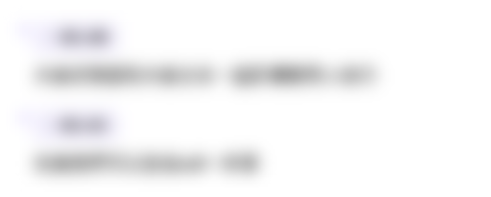
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
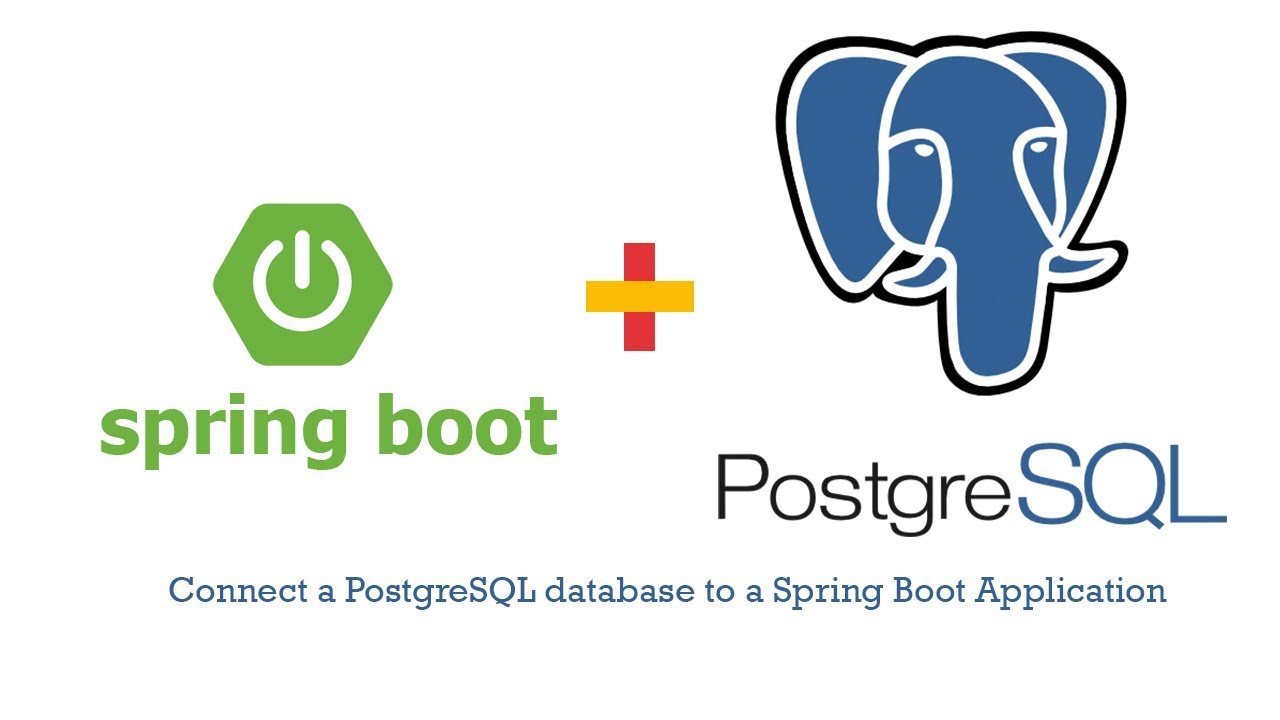
Connect a PostgreSQL database to a Spring Boot Application Tutorial
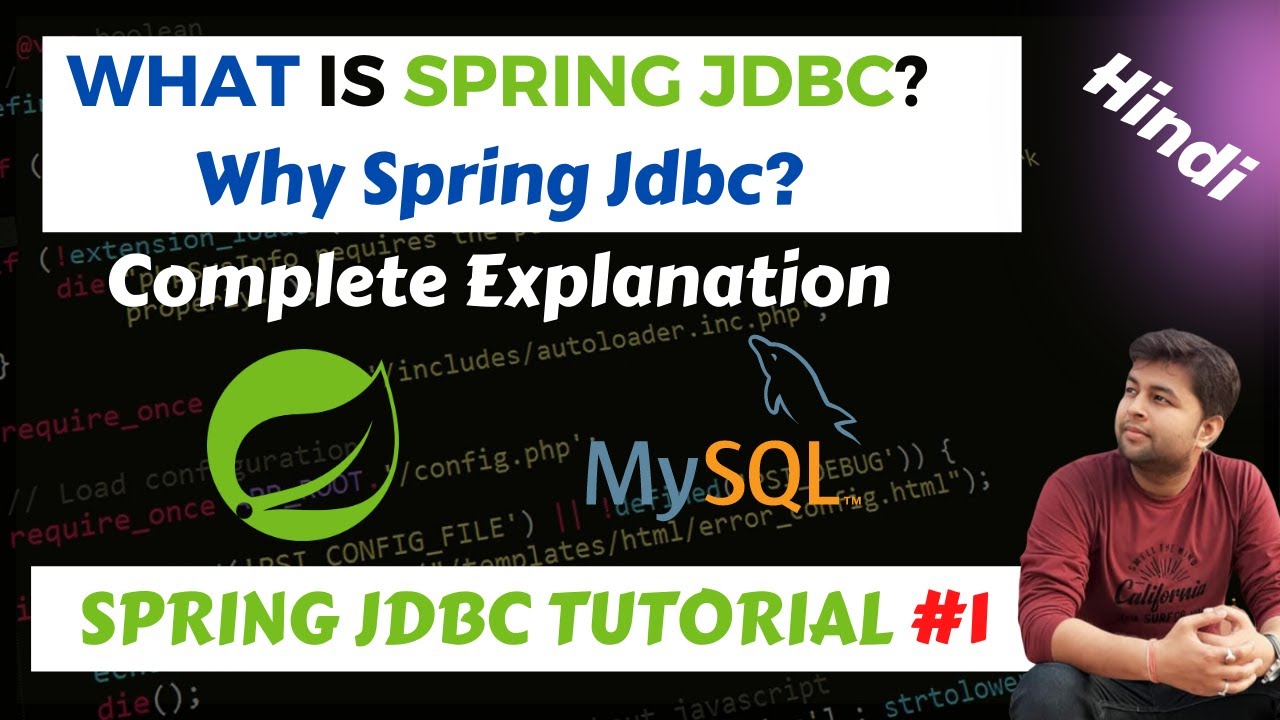
What is Spring JDBC | Introduct to Spring JDBC | Why to use Spring JDBC | Spring JDBC Tutorial
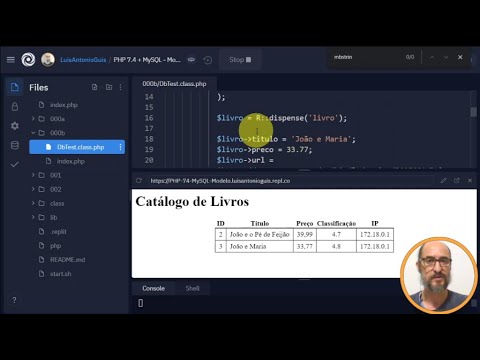
Mód VII - Sem III - PHP+RedBeanPHP & MySQL (Parte 1) - WebDev [ANP2020/Integrado]
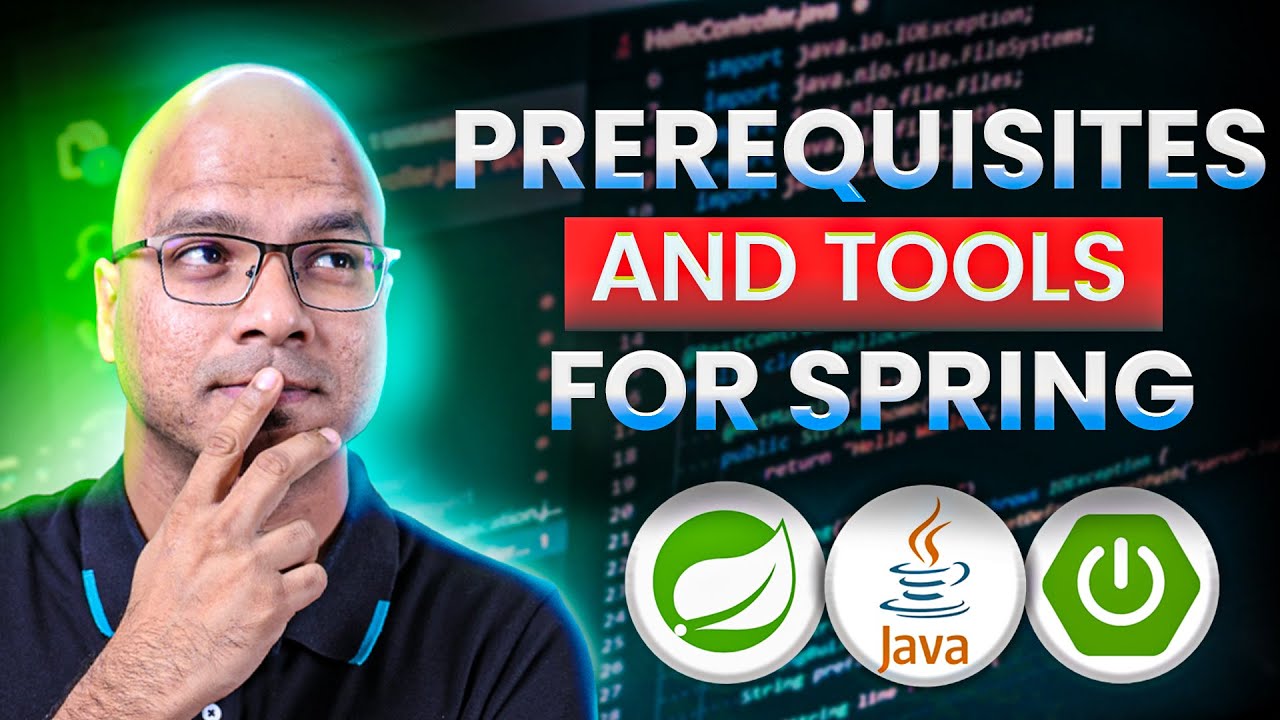
#3 Prerequisite and Tools required for Spring
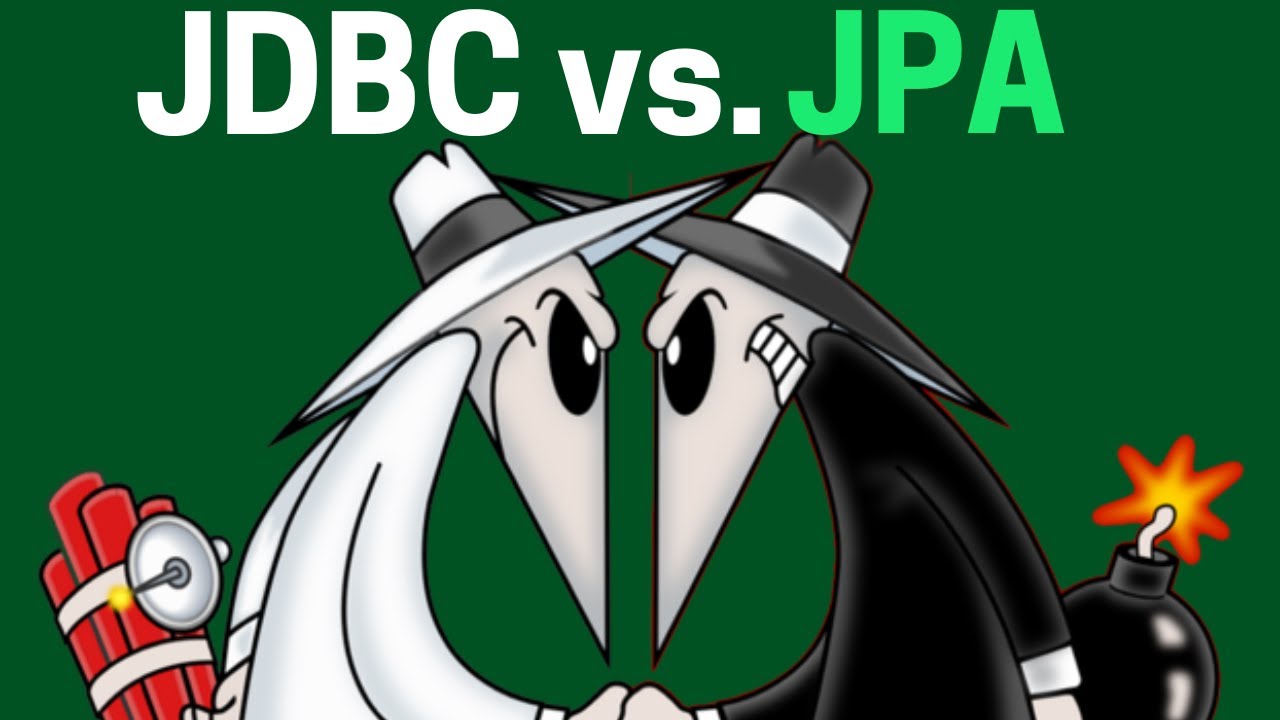
JDBC vs JPA: Pros and Cons
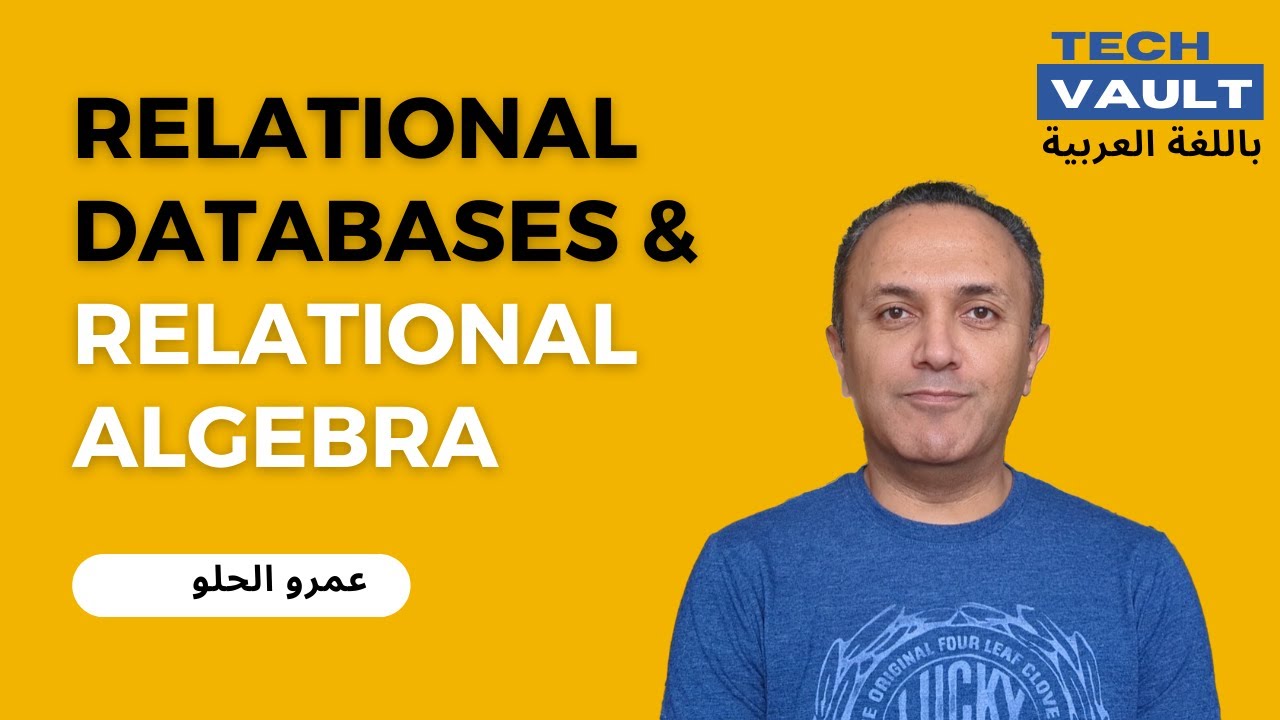
Relational Databases & Relational Algebra (Arabic - عربي) with Amr Elhelw - Tech Vault
5.0 / 5 (0 votes)