Python Requests | Get and Post Requests
Summary
TLDRThis video tutorial introduces viewers to the fundamentals of HTTP GET and POST requests using Python's requests library. It explains how to effectively retrieve data from APIs with GET requests, emphasizing the importance of query strings and handling response content. The tutorial then transitions to POST requests, showcasing how to submit data for form processing and file uploads. By demonstrating practical examples with httpbin, the video equips viewers with the necessary skills to implement these HTTP methods in their applications, highlighting best practices and common use cases.
Takeaways
- ๐ GET requests are used to retrieve data from a specified resource.
- ๐ GET requests should not be used for transmitting sensitive information.
- ๐ To make a GET request in Python, import the requests library and use requests.get() with the URL.
- ๐ Httpbin.org is a useful tool for testing HTTP requests without requiring authentication.
- ๐ URL parameters can be passed in a GET request using a query string format.
- ๐ Query strings start with a '?' after the domain and can include multiple parameters separated by '&'.
- ๐ A response object contains information received from the request, including the status code.
- ๐ A status code of 200 indicates a successful request.
- ๐ The response body can be accessed as bytes using r.content or as a decoded string using r.text.
- ๐ POST requests are used for submitting data, creating or updating resources, and can handle larger amounts of content.
Q & A
What is the primary purpose of an HTTP GET request?
-The primary purpose of an HTTP GET request is to retrieve data from a specified resource without modifying it.
Why should sensitive information not be sent via GET requests?
-Sensitive information should not be sent via GET requests because the data is included in the URL, making it visible and potentially accessible to unauthorized parties.
How do you initiate a GET request using the Python requests library?
-To initiate a GET request in Python using the requests library, you first import the library and then call 'requests.get()' with the URL as an argument.
What is the purpose of the 'params' keyword in a GET request?
-The 'params' keyword allows you to pass URL parameters as a dictionary, which are then converted into a query string and appended to the URL.
What function is used to check the status of an HTTP response?
-You can check the status of an HTTP response by accessing the 'status_code' attribute of the response object.
What differentiates a POST request from a GET request?
-A POST request is used to submit data to a server for creating or updating resources, while a GET request is used to retrieve data. POST requests can send larger amounts of data and do not include parameters in the URL.
What should be modified in the request to change from a GET to a POST request?
-To change from a GET to a POST request, you modify the method from 'get' to 'post', change the URL to the appropriate POST endpoint, and use 'data' instead of 'params' to send the payload.
How are URL parameters structured in a GET request?
-URL parameters are structured as a query string, starting with a question mark after the URL, followed by parameter names and values in pairs, separated by '&' for multiple parameters.
What function would you use to retrieve the content of a response in bytes?
-To retrieve the content of a response in bytes, you would use the 'content' function on the response object.
What is the significance of using 'httpbin.org' for testing HTTP requests?
-Httpbin.org is significant for testing HTTP requests because it provides a simple way to send and receive well-formatted responses without needing authentication, making it ideal for quick testing.
Outlines
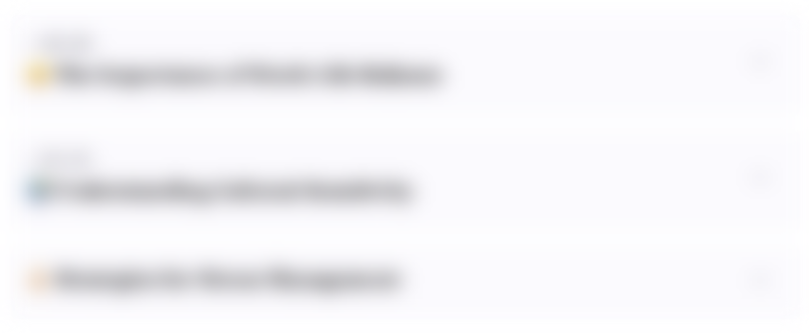
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
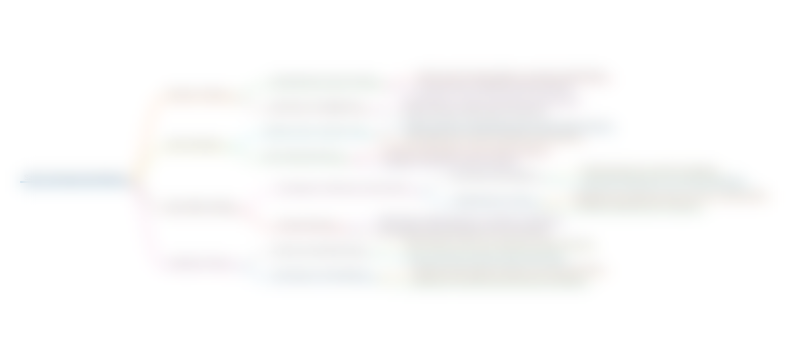
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
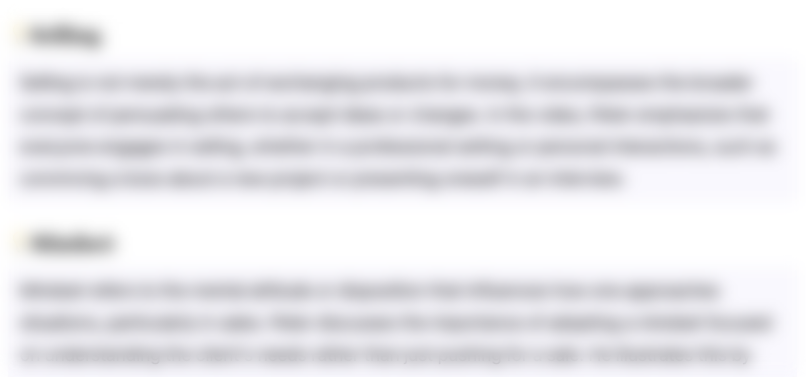
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
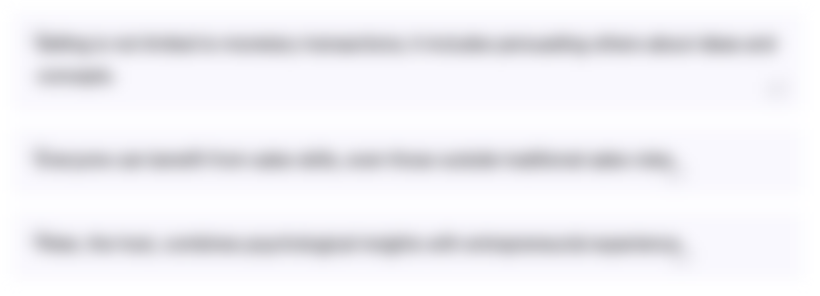
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
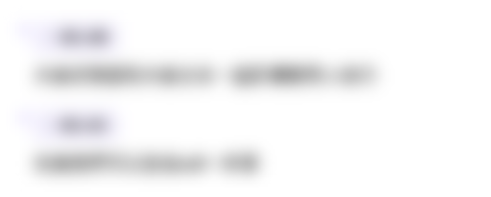
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
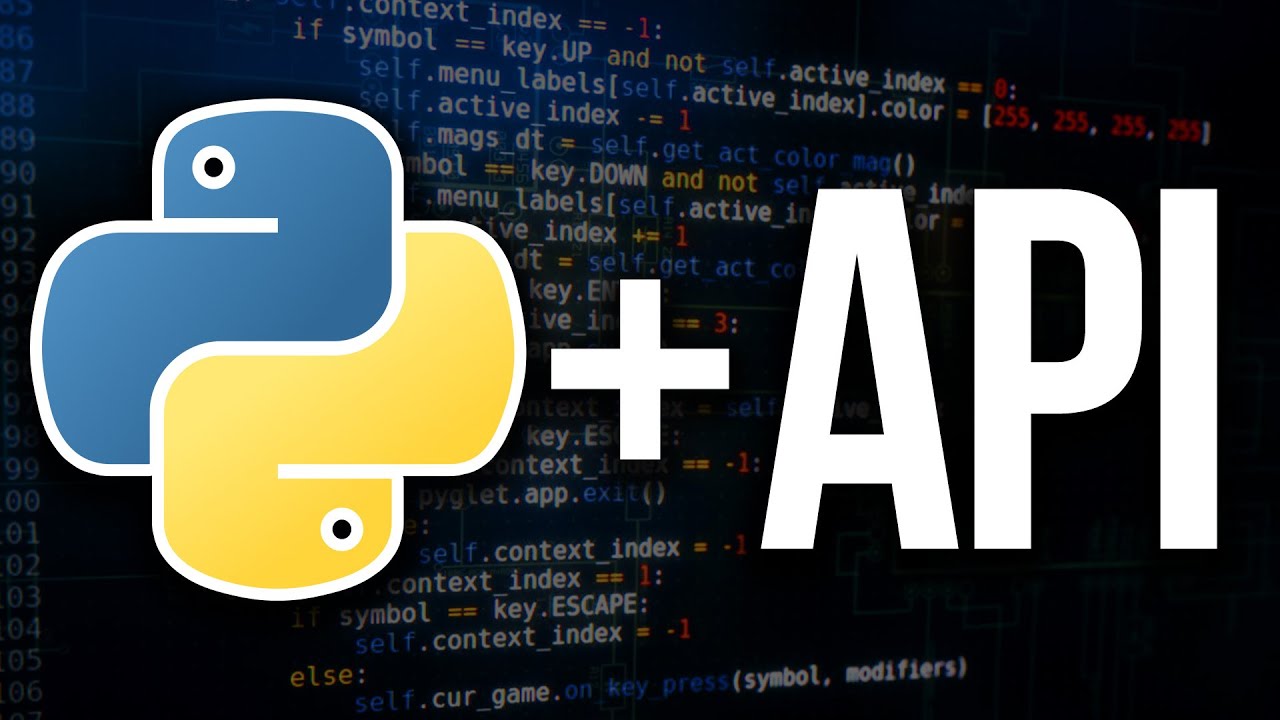
Create A Python API in 12 Minutes
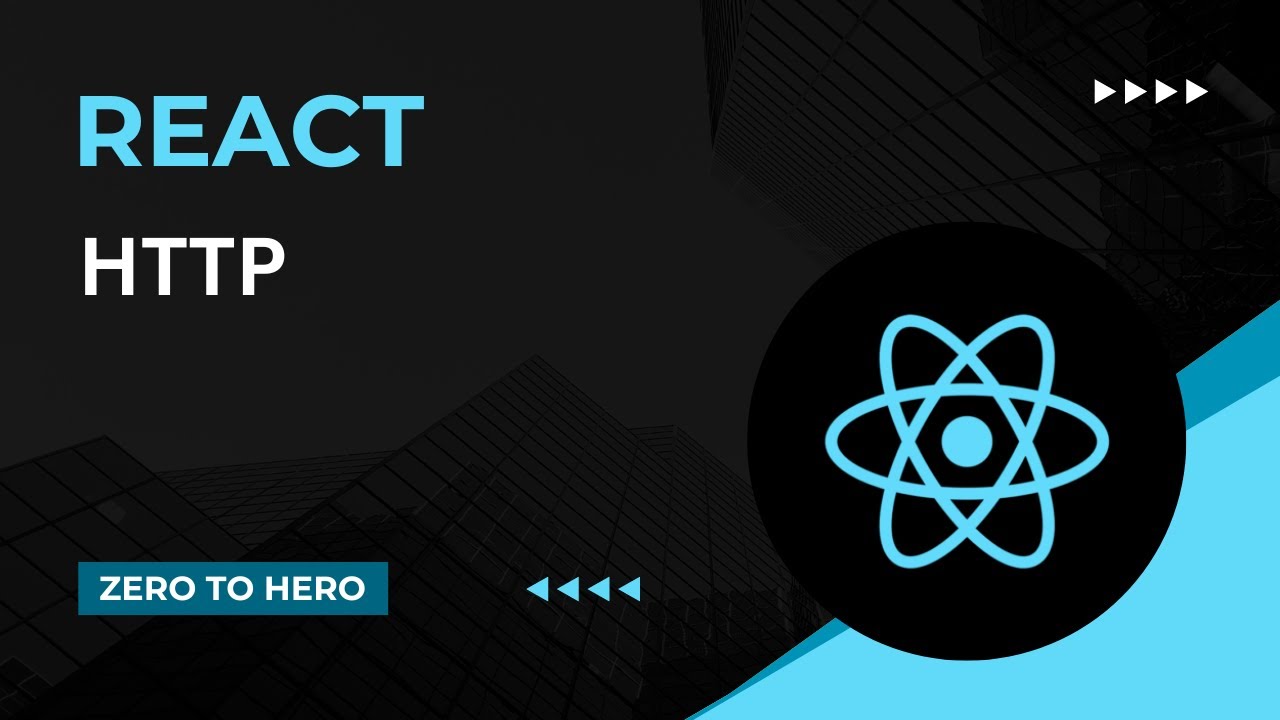
HTTP | Mastering React: An In-Depth Zero to Hero Video Series
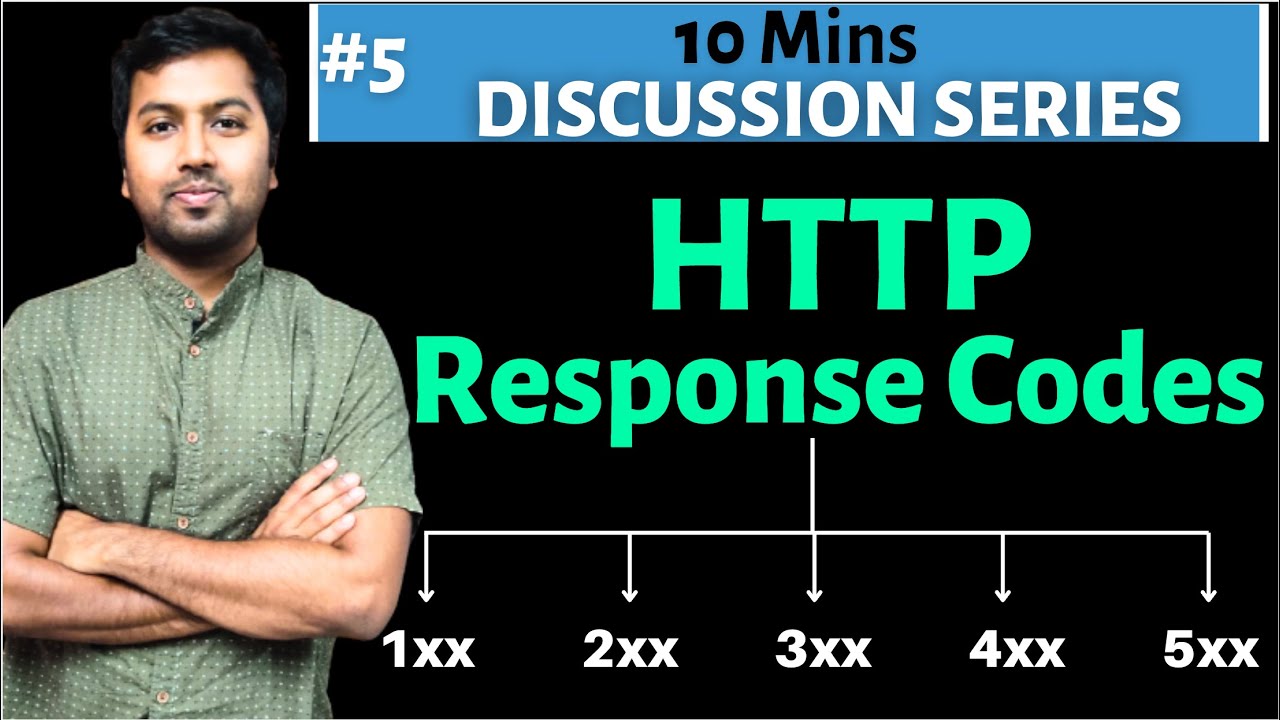
HTTP response status codes | REST API response codes | @ConceptandCoding
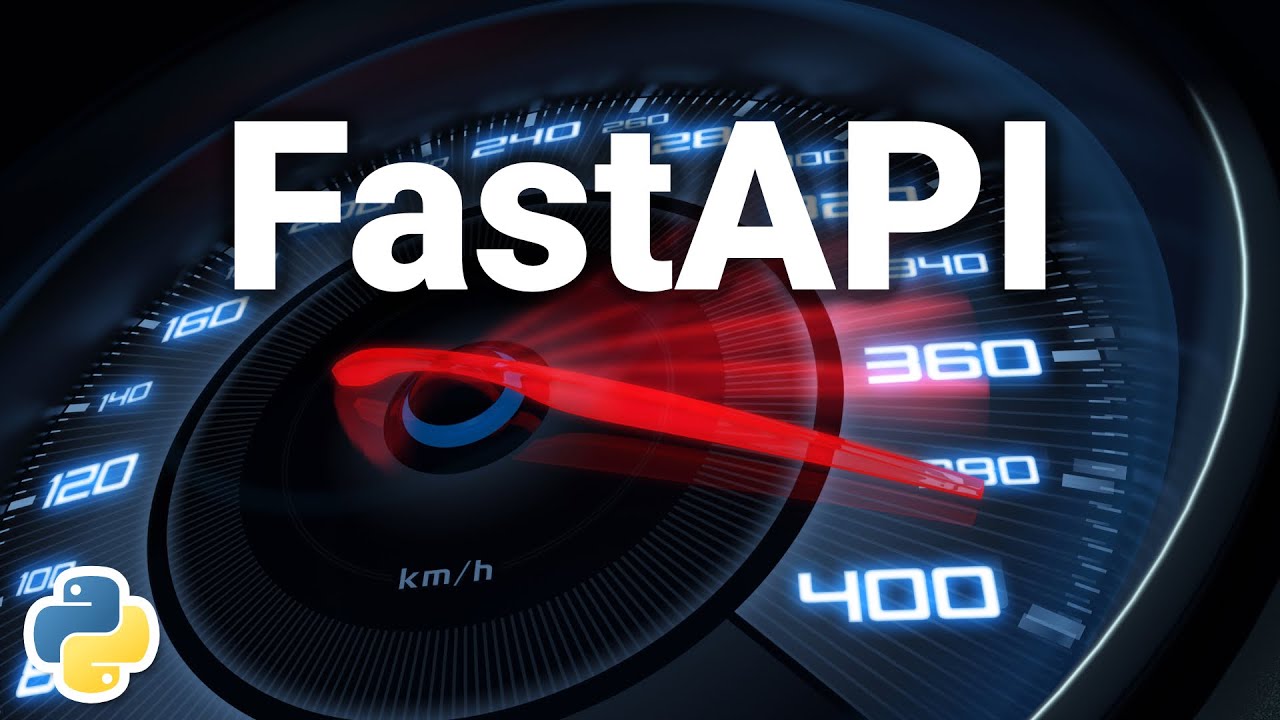
How to Use FastAPI: A Detailed Python Tutorial
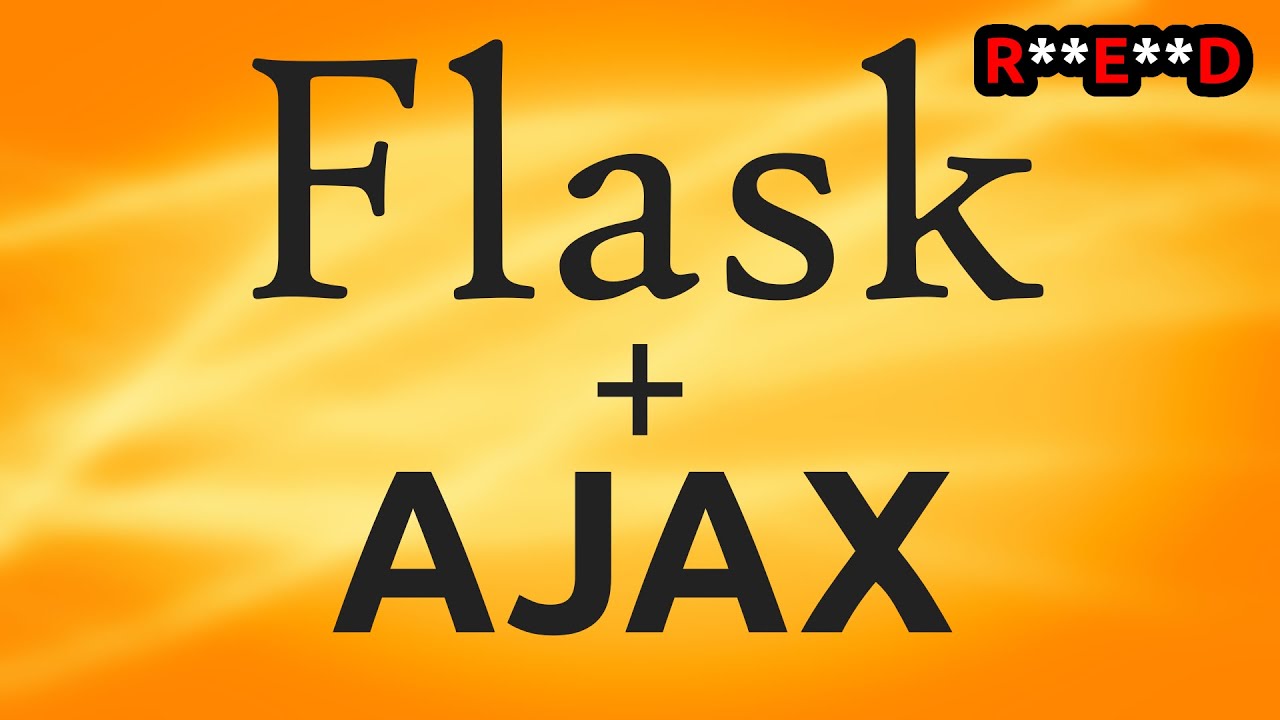
Flask AJAX Tutorial: Basic AJAX in Flask app | Flask casts
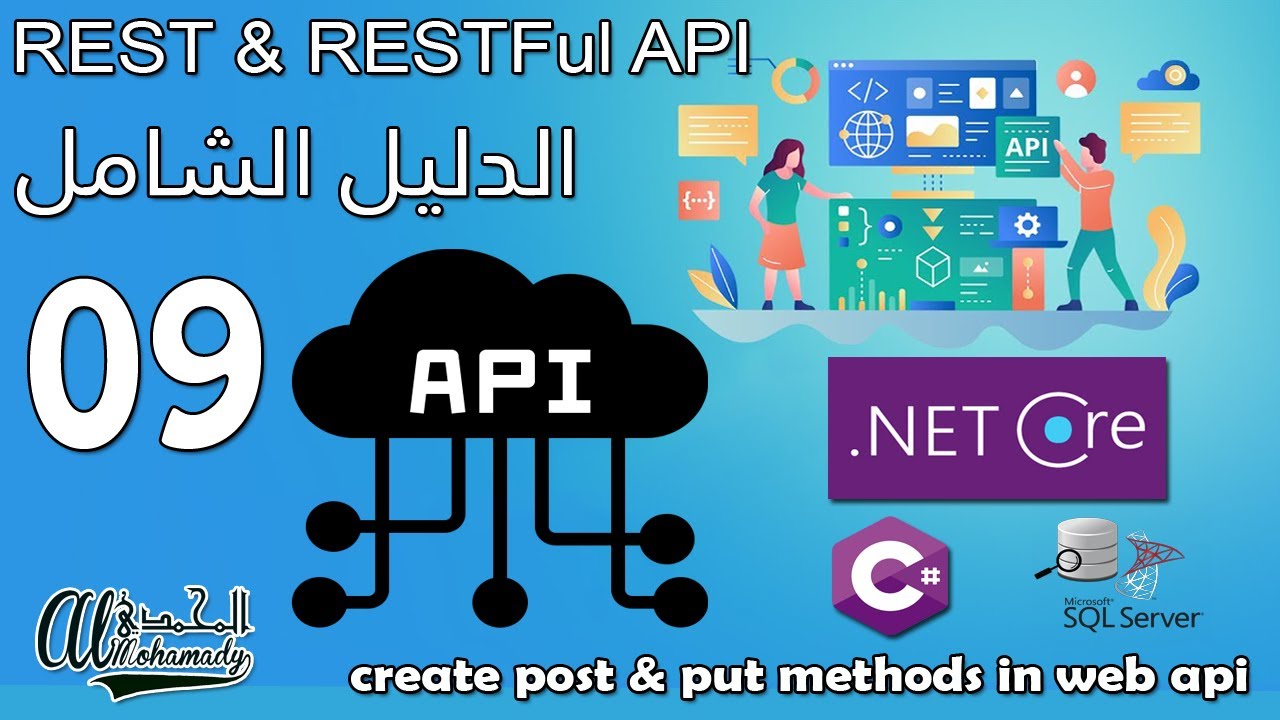
09 ุฅูุดุงุก ุฏูุงู ุงูุญูุธ ู ุงูุชุนุฏูู create post & put methods in web api
5.0 / 5 (0 votes)