The Dining Philosophers Problem
Summary
TLDRThis lecture explores the Dining Philosophers Problem, a classic synchronization issue in computer science. It introduces the scenario of five philosophers seated around a table with limited forks, each needing two forks to eat. The problem is analogous to processes in an operating system competing for shared resources. The lecture discusses using semaphores to prevent two adjacent philosophers from eating simultaneously, thus avoiding deadlocks. Solutions include limiting the number of philosophers at the table, ensuring both required forks are available before eating, and adopting an asymmetric approach to picking up forks. The lecture concludes by emphasizing the importance of these concepts in resource allocation and synchronization in operating systems.
Takeaways
- 🍽️ The Dining Philosophers Problem is a classic synchronization problem in computer science, illustrating the challenge of resource allocation among processes.
- 🤔 Philosophers can be in two states: thinking or eating, and they need two forks to eat, representing the need for multiple resources to perform a task.
- 🥢 Each fork is a limited resource, and a philosopher must use two forks (one from each side) to eat, symbolizing the contention for resources in a system.
- 🔒 Semaphores are introduced as a method to solve synchronization issues by ensuring that a philosopher can only eat if both required forks are available.
- 👨🍳 The problem can lead to a deadlock where each philosopher holds one fork and waits indefinitely for the second, which is held by another philosopher.
- 🔑 A semaphore is a synchronization tool that controls access to a common resource by multiple processes, preventing conflicts.
- ❌ A naive solution using semaphores can still result in deadlocks if all philosophers grab the left fork simultaneously and wait for the right fork.
- 🚫 To prevent deadlocks, solutions include limiting the number of philosophers that can eat at once, ensuring philosophers pick up both forks before eating, or using an asymmetric strategy for picking up forks.
- 🔄 An asymmetric solution involves odd-numbered philosophers picking up forks in one order and even-numbered philosophers in the opposite order, preventing circular wait conditions.
- 💡 The Dining Philosophers Problem is not just an academic exercise; it reflects real-world challenges in operating systems regarding process synchronization and resource management.
Q & A
What is the Dining Philosophers Problem?
-The Dining Philosophers Problem is a classic problem in computer science that illustrates synchronization issues among concurrent processes. It involves five philosophers seated around a round table with a single fork between each pair. Each philosopher alternates between thinking and trying to eat, but to eat, they must pick up the forks to their left and right. The challenge is to design a system where philosophers can eat without causing deadlocks or starvation.
How do philosophers represent processes in the Dining Philosophers Problem?
-In the Dining Philosophers Problem, each philosopher represents a process in an operating system. They can be in two states: thinking (not using resources) or eating (requiring resources). The forks or chopsticks represent shared resources that must be managed to prevent conflicts and ensure proper synchronization.
What is the significance of forks in the Dining Philosophers Problem?
-Forks in the Dining Philosophers Problem represent limited resources that must be shared between processes (philosophers). Each philosopher needs two forks to eat, which introduces the potential for resource contention and the need for synchronization mechanisms to avoid deadlocks and ensure fair resource allocation.
How can semaphores be used to solve the Dining Philosophers Problem?
-Semaphores can be used to control access to the forks in the Dining Philosophers Problem. Each fork is represented by a semaphore, and philosophers use wait (P) operations on the semaphores for the left and right forks before they can eat. After eating, they release (V) the forks by performing signal operations on the semaphores, allowing other philosophers to pick up the forks.
What is a deadlock in the context of the Dining Philosophers Problem?
-A deadlock occurs when each philosopher picks up the fork to their left and waits indefinitely for the fork to their right, which is also being held by another philosopher. This situation leads to all philosophers being unable to eat because they are each waiting for a resource held by another, creating a circular wait condition.
How can the possibility of a deadlock be prevented in the Dining Philosophers Problem?
-Deadlocks can be prevented by ensuring that not all philosophers can pick up their left forks simultaneously. This can be achieved by allowing only four philosophers to pick up their left forks at any time, ensuring one fork is always available. Alternatively, philosophers can be required to pick up both forks before eating, or an asymmetric strategy can be used where odd-numbered philosophers pick up the left fork first and even-numbered philosophers pick up the right fork first.
What is a binary semaphore and how does it relate to the forks in the Dining Philosophers Problem?
-A binary semaphore is a synchronization mechanism that can be in one of two states: 1 (available) or 0 (unavailable). In the Dining Philosophers Problem, each fork is represented by a binary semaphore. When a philosopher wants to eat, they perform a wait operation on the semaphore for the fork, which changes its state from 1 to 0, indicating it's in use. When the philosopher finishes eating, they perform a signal operation, changing the semaphore back to 1, making the fork available again.
What is the role of the modulo operator in the Dining Philosophers Problem?
-The modulo operator is used to handle the circular arrangement of philosophers and forks. When a philosopher wants to pick up the fork to their right, they calculate the index of the right fork using the formula (i + 1) % 5, where i is the philosopher's index. This ensures that the fifth philosopher, for example, correctly picks up the fork at index 1 instead of going out of bounds.
Why is it important for a philosopher to pick up both forks before eating?
-Requiring a philosopher to pick up both forks before eating prevents deadlocks by ensuring that a philosopher will only start eating when both necessary resources are available. This avoids scenarios where each philosopher holds one fork and waits indefinitely for the second, which is held by another philosopher, thus preventing any progress.
How does the asymmetric solution work in the Dining Philosophers Problem?
-The asymmetric solution involves odd-numbered philosophers picking up their left fork first and then their right fork, while even-numbered philosophers pick up their right fork first and then their left fork. This strategy prevents all philosophers from simultaneously picking up the fork to their left, which could lead to a deadlock, by ensuring that at least one fork is available for a philosopher to pick up.
Outlines
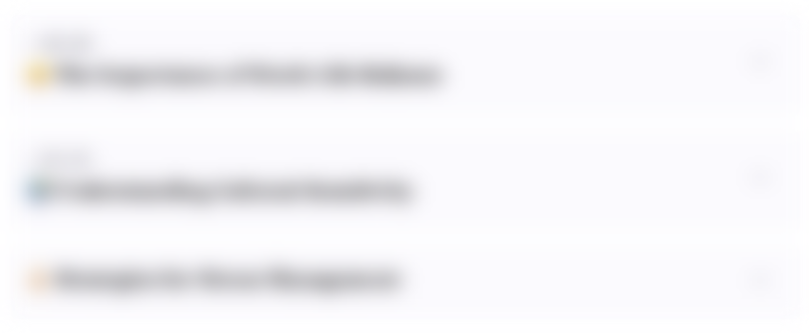
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
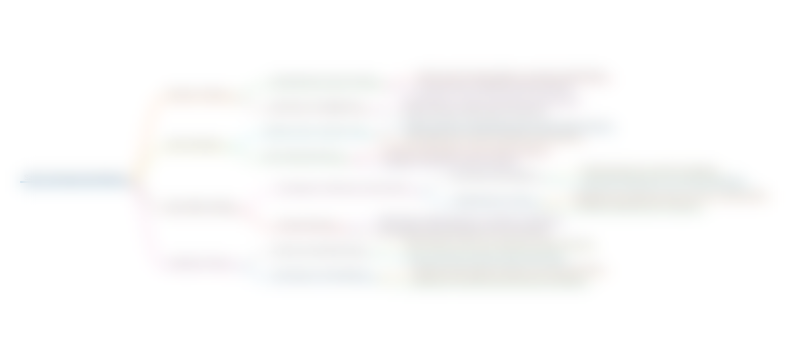
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
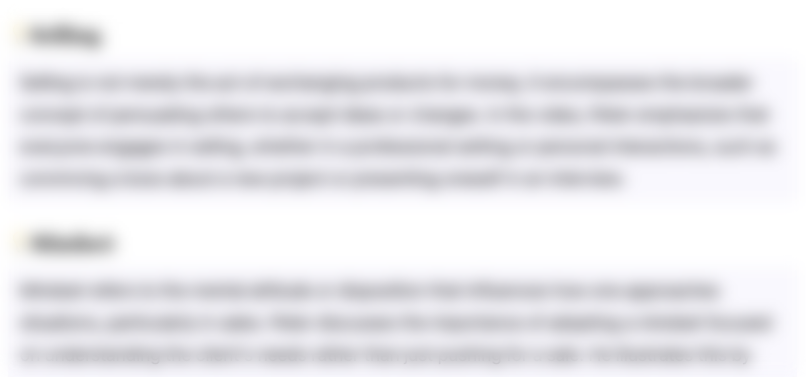
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
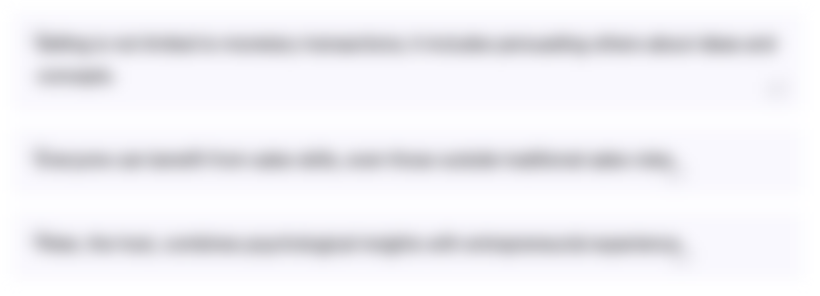
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
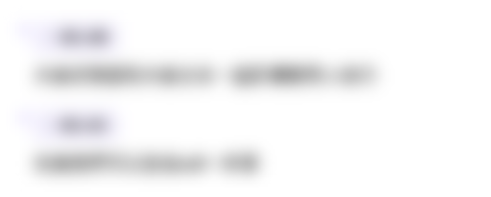
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)