How to Program A Calculator in C++! (Great C++ Microsoft Visual Studio Beginner Project)
Summary
TLDRIn this video, the presenter guides viewers through creating a basic four-function calculator using C++ in Microsoft Visual Studio. The tutorial covers essential programming concepts such as user input and output, console display, and basic mathematical operations. The script includes detailed steps for setting up the project, defining variables, and implementing an if-else statement to handle different arithmetic operations. The video is designed for beginners to practice handling real-time user data and processing it with simple calculations.
Takeaways
- π» The video is a tutorial on creating a simple four-function calculator using C++ in Microsoft Visual Studio.
- π The tutorial covers the basics of user input and output, displaying console messages, and performing arithmetic operations.
- π It introduces the use of `iostream` for console interaction and `cmath` for mathematical functions.
- π’ The calculator is designed to handle basic arithmetic operations: addition, subtraction, multiplication, and division.
- π― The script guides viewers on defining variables for operations and numbers, using strings and doubles for flexibility.
- π¨βπ» It demonstrates how to prompt users for input and store it in variables, using `std::cout` for output and `std::cin` for input.
- π The tutorial introduces conditional statements (`if`, `else if`, `else`) to handle different arithmetic operations based on user input.
- π οΈ The script emphasizes the importance of proper formatting and indentation in C++ code for readability.
- π The video serves as a beginner project to help viewers understand how to process real-time user data in C++ programs.
- π The presenter encourages viewers to engage with the content by liking, subscribing, and providing feedback for future tutorial topics.
Q & A
What is the main focus of the video?
-The video focuses on teaching viewers how to create a simple four-function calculator using C++ in Microsoft Visual Studio.
What are the basic functionalities of the calculator being built in the video?
-The calculator is designed to perform basic arithmetic operations: addition, subtraction, multiplication, and division.
Which programming language is used in the video to create the calculator?
-The programming language used is C++.
What are the user inputs required for the calculator?
-The user inputs required are two numbers and an operation (either addition, subtraction, multiplication, or division).
What does the 'cmath' library provide in the context of the calculator project?
-The 'cmath' library is used to perform basic mathematical functions if needed.
Why is the 'iostream' library included in the project?
-The 'iostream' library is included to handle user inputs and outputs, allowing the program to display information on the console and receive user input.
How does the video guide viewers to handle user inputs for the calculator?
-The video demonstrates how to use 'cin' for taking user inputs and 'cout' for displaying prompts and results to the user.
What data types are used for the variables storing the operation and numbers in the calculator?
-A string data type is used for the operation variable, and double is used for the two number variables to allow for decimal values.
How does the video handle different arithmetic operations in the calculator?
-The video uses a series of 'if-else if' statements to check the entered operation and perform the corresponding arithmetic operation.
What does the video suggest for handling unrecognized operations?
-For unrecognized operations, the video suggests displaying an error message stating 'not a recognized operation'.
What is the purpose of the video in the context of learning C++?
-The video serves as a tutorial for beginners to get familiar with user inputs, outputs, console interactions, and basic arithmetic operations in C++.
Outlines
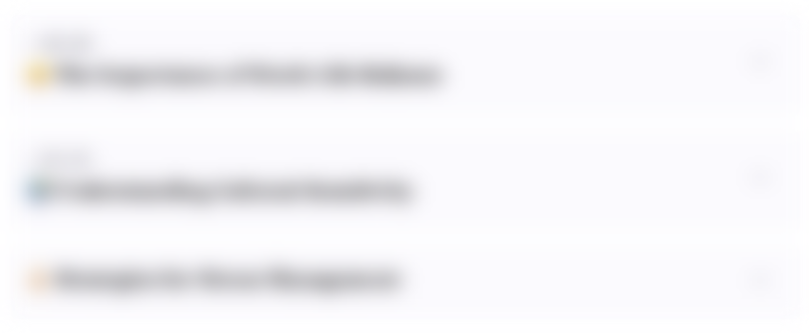
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
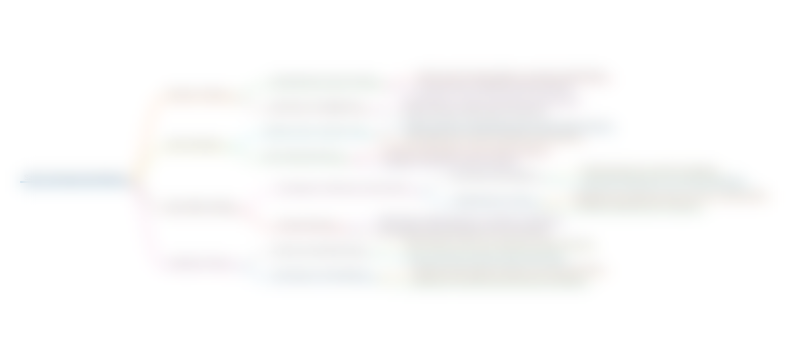
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
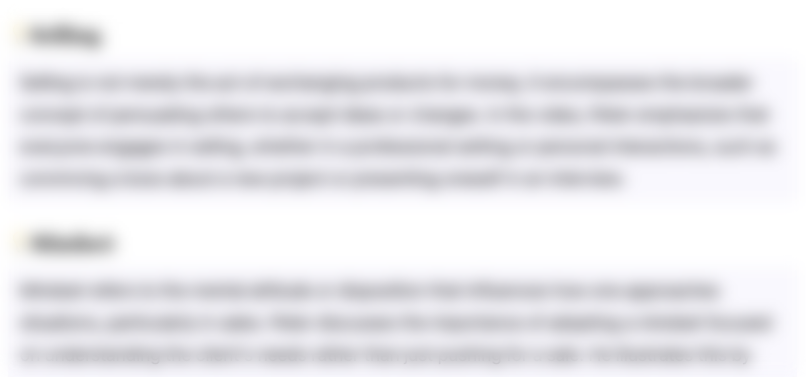
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
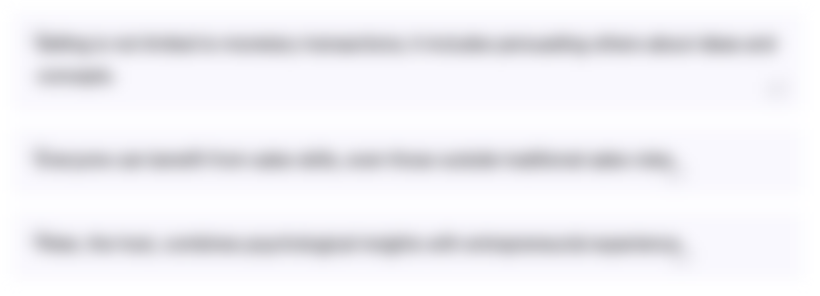
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
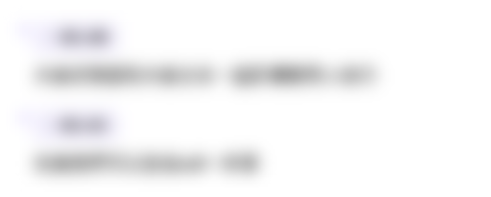
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)