Word Ladder - LeetCode Hard - Google Phone Screen Interview Question
Summary
TLDRThis video focuses on solving the 'Word Ladder' coding problem, where the objective is to find the shortest transformation sequence from a given start word to an end word by changing one letter at a time, using a provided word list. The instructor breaks down the problem, discussing the intuition and approach using Breadth-First Search (BFS) and a queue. They provide a step-by-step explanation of the algorithm, including code implementation in Java. The video aims to help viewers prepare for coding interviews by understanding advanced tree data structure problems and their solutions.
Takeaways
- 📌 The video is part of a D algorithms boot camp series focusing on advanced questions for the tree data structure.
- 📚 Viewers are encouraged to follow the playlist in the provided order for a structured learning experience.
- 🔥 The specific problem discussed was asked in a Google phone screen and is popular on LeetCode with close to 12,000 thumbs up.
- 🚧 Despite being labeled as a hard question on LeetCode, the presenter suggests it is not as difficult as it seems.
- 💬 The challenge involves transforming one word to another using a dictionary list, changing one letter at a time, under specific conditions.
- 📈 The solution employs a Breadth-First Search (BFS) approach utilizing a queue and a set for efficient lookups.
- 📝 A step-by-step solution process is explained, emphasizing the importance of incrementing the length for each word change.
- 📊 The time complexity is detailed as O(N^2 * M), where N is the number of words and M is the length of the word, highlighting the algorithm's efficiency.
- 🛠 The presenter walks through coding the solution in LeetCode, providing insights into handling base cases and iterating through character changes.
- 📦 Additional resources, including code and assignments, are available in the video description for further exploration and practice.
Q & A
What is the problem being discussed in the video?
-The video discusses the 'Word Ladder' problem, where the task is to find the shortest transformation sequence from a given 'beginWord' to an 'endWord' by changing one letter at a time, and all intermediate words must belong to a given 'wordList'.
Can you provide an example to better understand the problem?
-Yes, the example given in the video is: beginWord = 'hit', endWord = 'cog', wordList = ['hot', 'dot', 'dog', 'lot', 'log', 'cog']. The shortest transformation sequence is 'hit' -> 'hot' -> 'dot' -> 'dog' -> 'cog', which requires 5 steps.
What approach is suggested to solve the Word Ladder problem?
-The video suggests using a Breadth-First Search (BFS) approach, where we start with the 'beginWord', add adjacent words (differing by one letter) to a queue, and continue the process until we find the 'endWord' or exhaust all possibilities.
How is the adjacency between words determined?
-To determine if two words are adjacent (differing by one letter), the video proposes replacing each character of the current word with all possible 26 English alphabet characters and checking if the resulting word exists in the 'wordList'.
What data structures are used in the proposed solution?
-The solution uses a queue (implemented using a LinkedList) to perform the BFS traversal, and a set (HashSet) to store the 'wordList' for efficient lookup.
What is the time complexity of the proposed solution?
-The time complexity of the solution is O(N^2 * M), where N is the number of words in the 'wordList', and M is the length of each word. This is because for each word, we need to check all other words (O(N^2)) and replace each character (O(M)).
What is the space complexity of the proposed solution?
-The space complexity of the solution is O(N), as we need to store the 'wordList' in a set for efficient lookup.
What are the base cases or terminating conditions for the BFS traversal?
-The BFS traversal terminates if (1) the 'endWord' is found, in which case the length of the transformation sequence is returned, or (2) the queue becomes empty, indicating that no transformation sequence is possible, and zero is returned.
How does the solution handle visited words to avoid cycles or redundant computations?
-The solution uses a set called 'visited' to keep track of words that have already been processed. When a new word is generated, it is added to the queue and the 'visited' set only if it exists in the 'wordList' and has not been visited before.
Are there any limitations or considerations mentioned for the proposed solution?
-The video does not explicitly mention any limitations or considerations for the proposed solution. However, it is worth noting that the solution assumes the 'wordList' is finite and contains only valid words, and that the length of all words in the list is the same.
Outlines
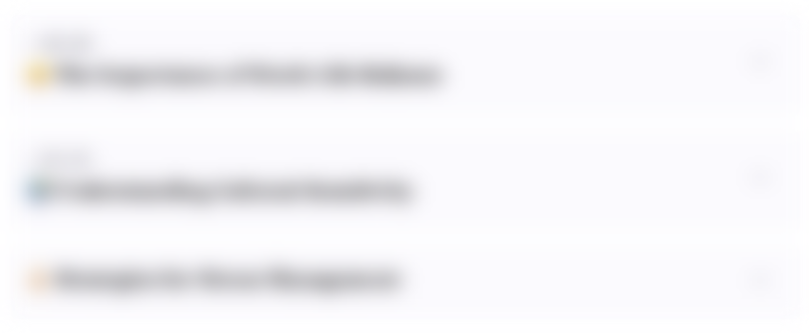
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
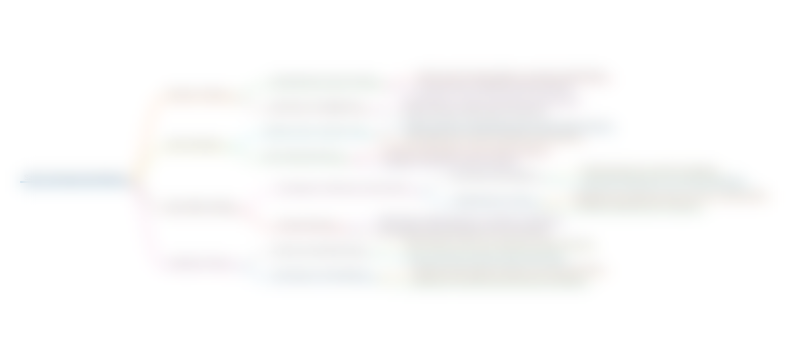
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
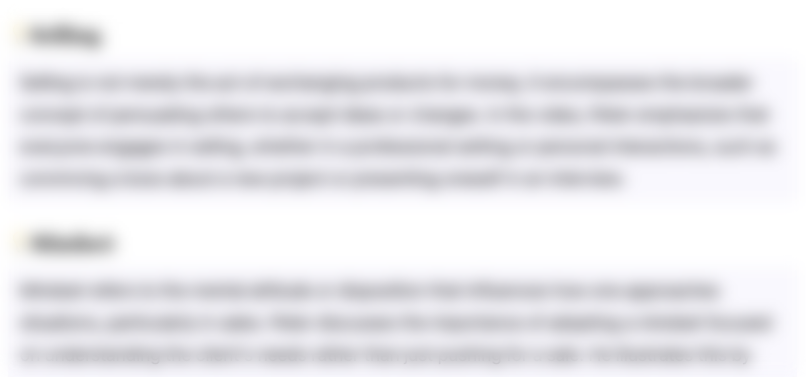
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
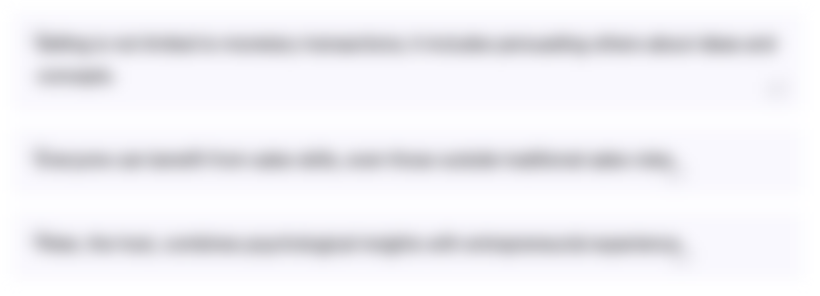
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
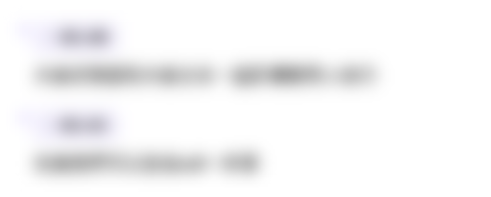
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
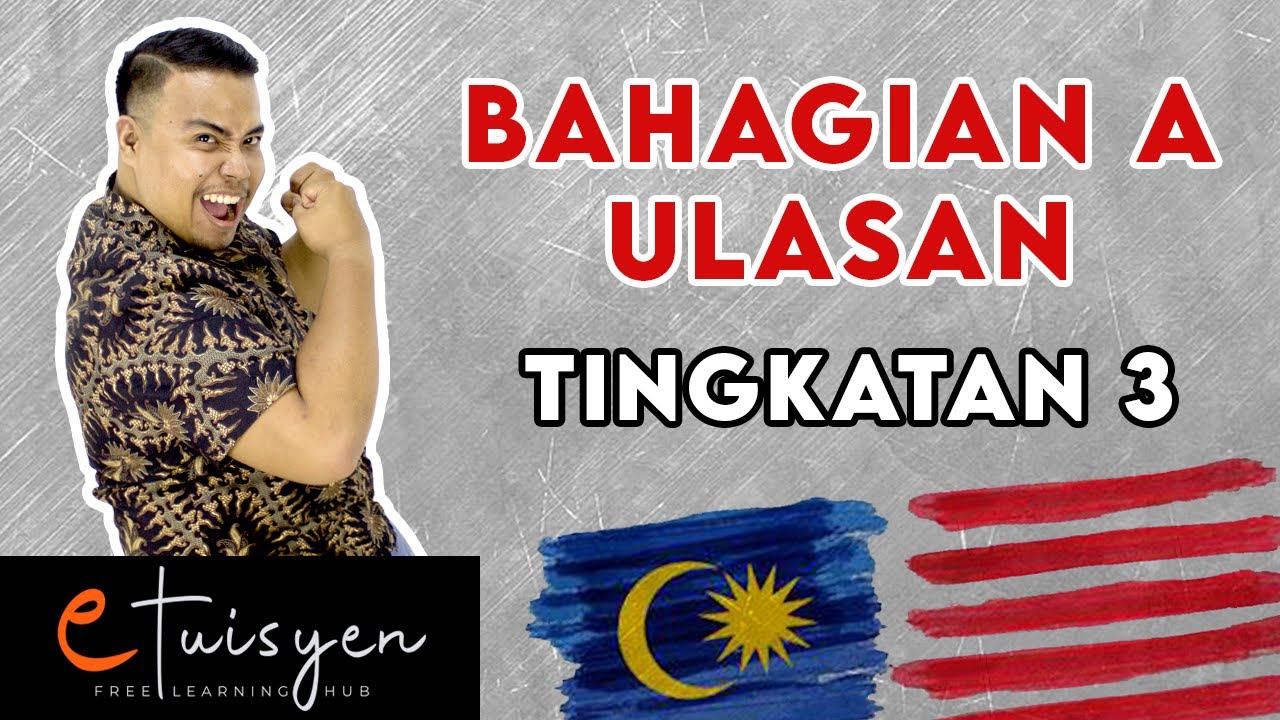
[eTuisyen] TINGKATAN 3 BAHASA MELAYU: Bab - Ulasan (Bahagian A)
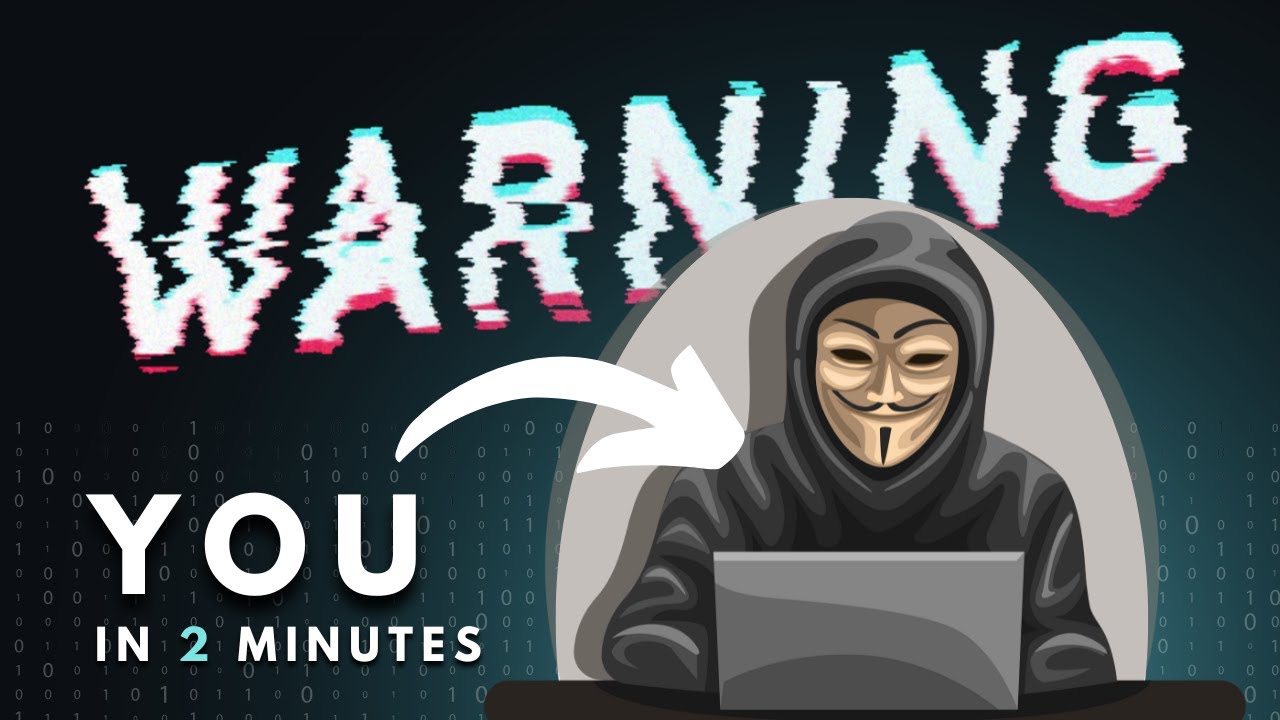
The Ultimate Hacker Effect That Anyone Can Do

Permutations - Jumbled Words 01
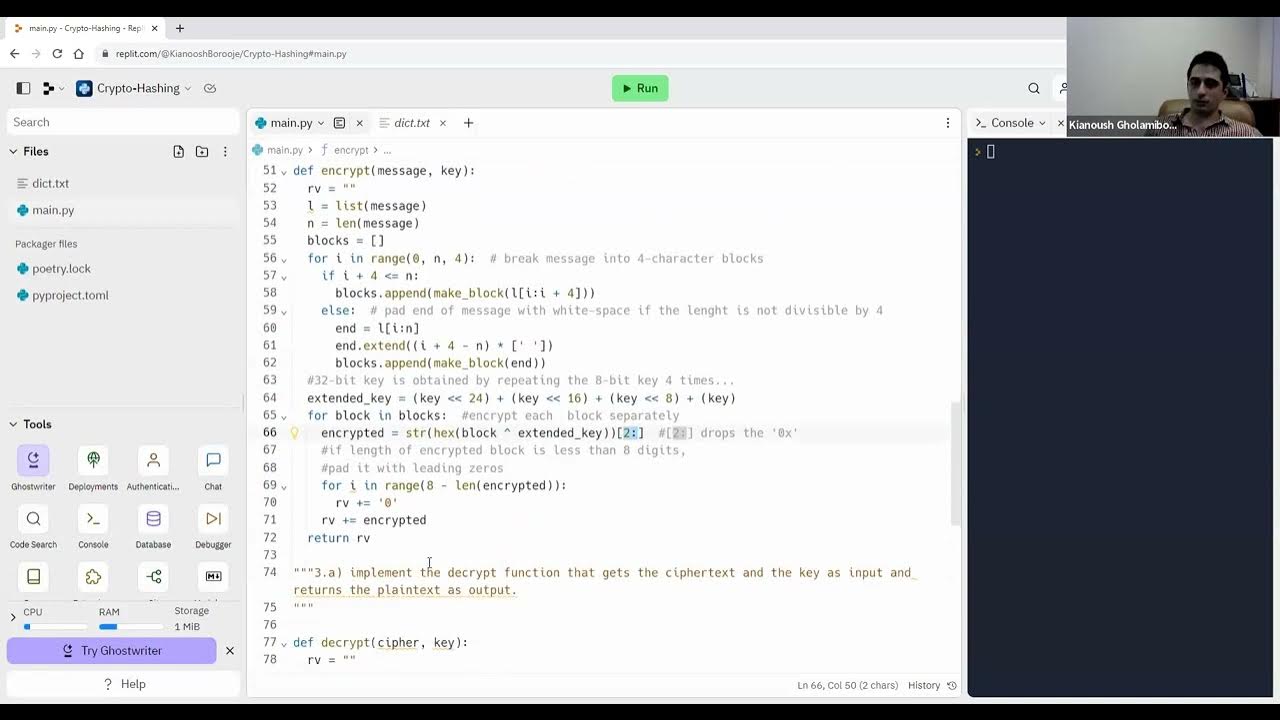
SHA256 and Symmetric Encryption Examples
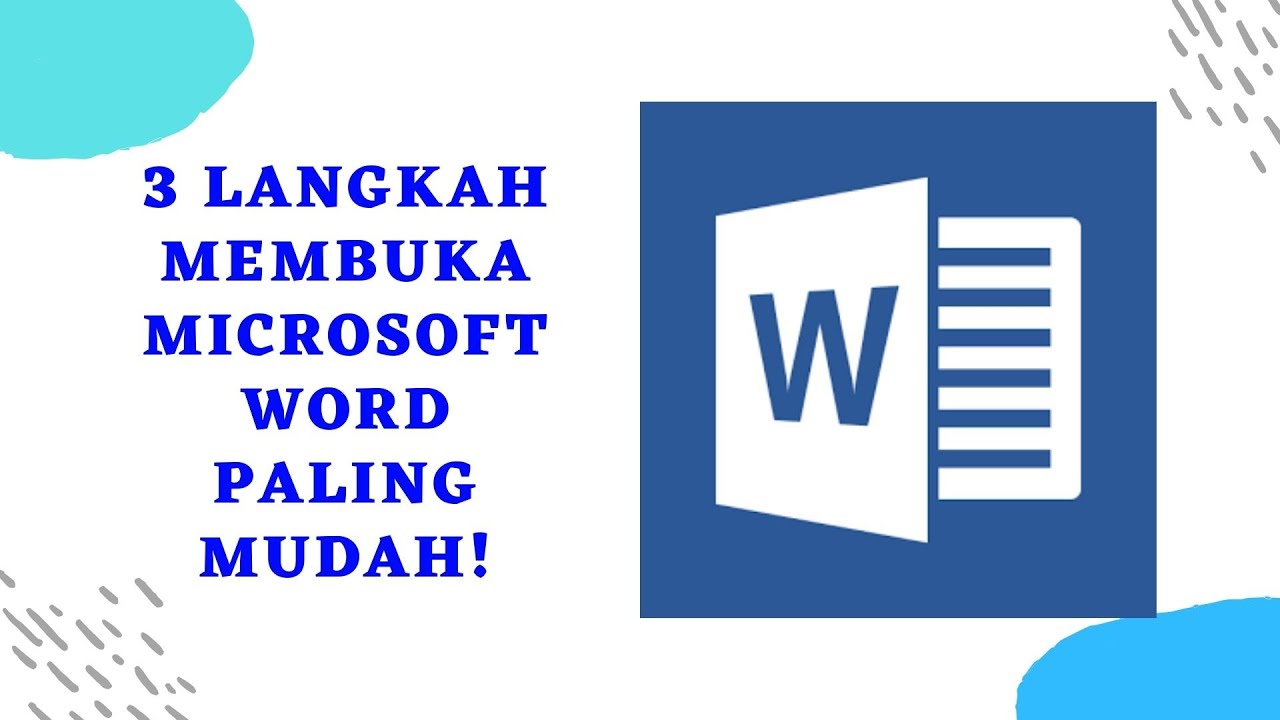
Sangat Mudah Sekali! Langkah langkah membuka microsoft word │Cocok untuk pemula!
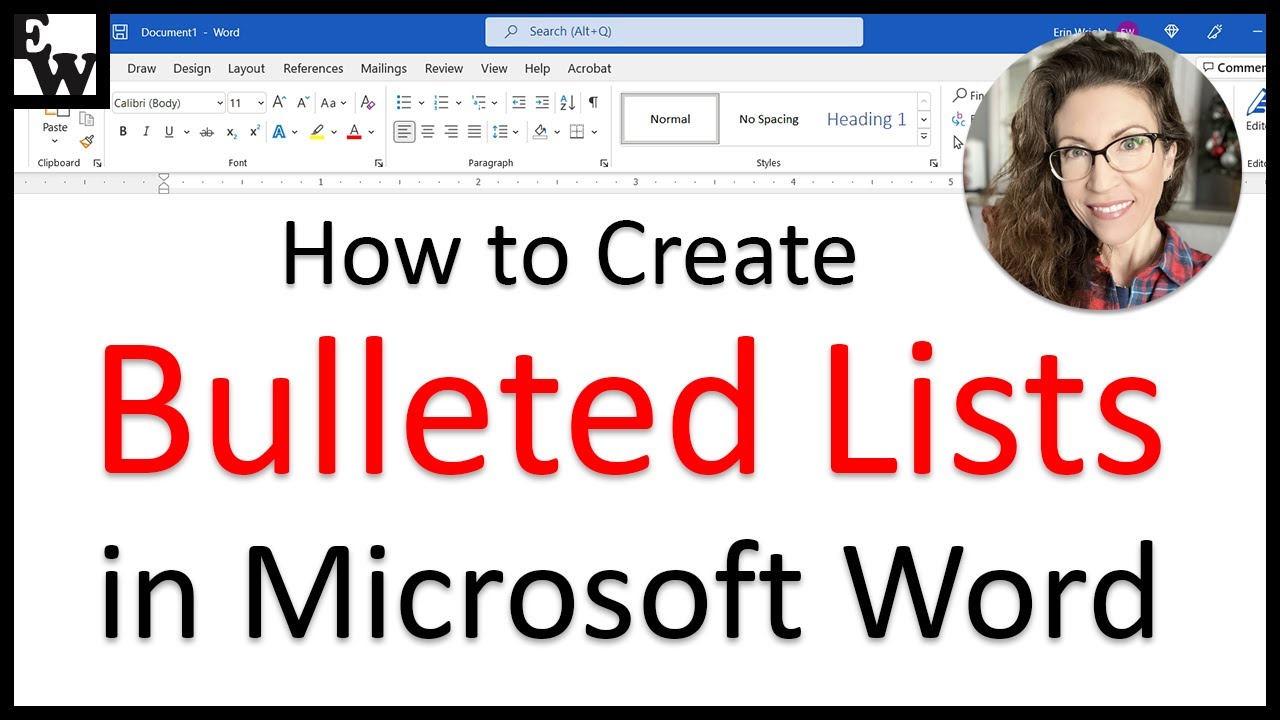
How to Create Bulleted Lists in Microsoft Word
5.0 / 5 (0 votes)