How Rendering Works: The Render Phase | Lecture 124 | React.JS π₯
Summary
TLDRThis lecture delves into the intricate workings of React's rendering process, unveiling the mechanics behind the scenes. While initially seeming daunting, it promises a fascinating exploration for the curious mind. The virtual DOM, a JavaScript object representation, is demystified, and the reconciliation process is unveiled as the heart of React's efficiency. The Fiber reconciler, with its mutable Fiber tree and asynchronous rendering capabilities, takes center stage. Through practical examples, the script illustrates how React determines what to update, reuse, or remove from the DOM, culminating in a list of effects to be applied during the commit phase.
Takeaways
- π The lecture covers the complex and fascinating topic of how React performs rendering, which is essential to understanding React's inner workings.
- π€ React does not actually discard and replace the entire component view or DOM during re-renders, contrary to initial explanations.
- π³ The virtual DOM is a tree of React elements representing the entire component tree, which is relatively cheap and fast to create.
- π When a component is re-rendered, all of its child components are also re-rendered, regardless of whether their props have changed or not.
- β‘ React uses a process called reconciliation to efficiently update the DOM by reusing as much of the existing DOM tree as possible.
- π§ The reconciler, called Fiber, is the engine of React and allows updates without directly touching the DOM.
- π² The Fiber tree is a mutable data structure that keeps track of component state, props, effects, and more, and is mutated during reconciliation.
- β±οΈ Fiber can perform work asynchronously, enabling features like Suspense and improving performance by not blocking the JavaScript engine.
- π Reconciliation involves diffing, which compares elements step-by-step based on their position in the tree to determine necessary DOM mutations.
- π The final result of the render phase is an updated Fiber tree and a list of effects (DOM operations) to be executed in the commit phase.
Q & A
What is the purpose of this lecture?
-The lecture aims to explain the inner workings of the rendering process in React, specifically focusing on the render phase and how reconciliation is performed.
What is the virtual DOM in React?
-The virtual DOM, also known as the React element tree, is a tree of all React elements created from all instances in the component tree. It is a JavaScript object representation of the UI, relatively cheap and fast to create.
Why does React re-render child components when a parent component is updated?
-React re-renders child components of an updated component because it doesn't know beforehand whether the update in the parent component will affect the child components or not. By default, React prefers to play it safe and re-render everything.
What is the purpose of reconciliation in React?
-Reconciliation is the process of deciding which DOM elements need to be inserted, deleted, or updated in order to reflect the latest state changes. It allows React to efficiently update the DOM by reusing as much of the existing DOM tree as possible.
What is the Fiber reconciler in React?
-The Fiber reconciler, also known as just 'Fiber,' is the engine of React. It is responsible for performing the reconciliation process and building the Fiber tree, which is an internal tree representing the component instances and DOM elements in the application.
What is the Fiber tree in React?
-The Fiber tree is a special internal tree where, for each component instance and DOM element in the app, there is one so-called 'Fiber.' Fibers are mutable data structures that keep track of things like component state, props, side effects, and hooks. The Fiber tree is never destroyed, but rather mutated during reconciliation.
How does the Fiber reconciler handle asynchronous rendering?
-The Fiber reconciler can perform work asynchronously, splitting the rendering process into chunks, prioritizing tasks, and pausing or resuming work as needed. This allows rendering to pause and resume later, preventing it from blocking the browser's JavaScript engine with long renders.
What is the 'diffing' process in React's reconciliation?
-Diffing is the process of comparing elements step-by-step, based on their position in the tree, to analyze what needs to change between the current Fiber tree and the updated Fiber tree based on the new virtual DOM.
What is the 'list of effects' in React's rendering process?
-The list of effects is a list of DOM mutations (insertions, deletions, or updates) that need to be performed, which is the result of the reconciliation process. This list will be used in the commit phase to actually mutate the DOM.
What is the next phase after the render phase in React's rendering process?
-The next phase after the render phase is the commit phase, where React uses the list of effects generated during the render phase to actually mutate the DOM and apply the changes to the UI.
Outlines
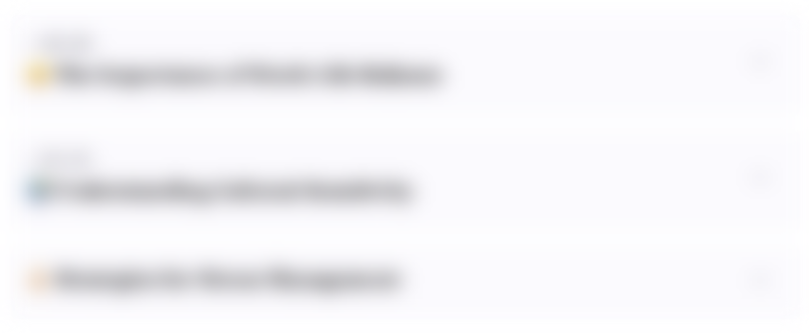
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
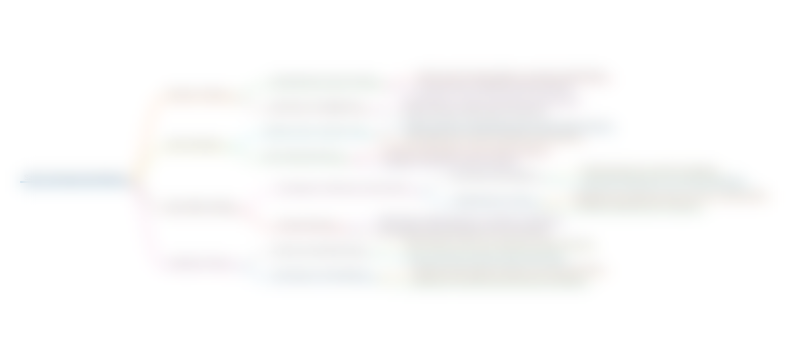
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
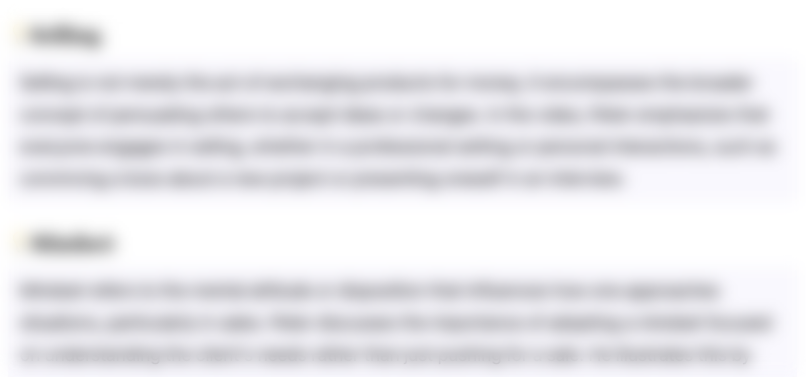
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
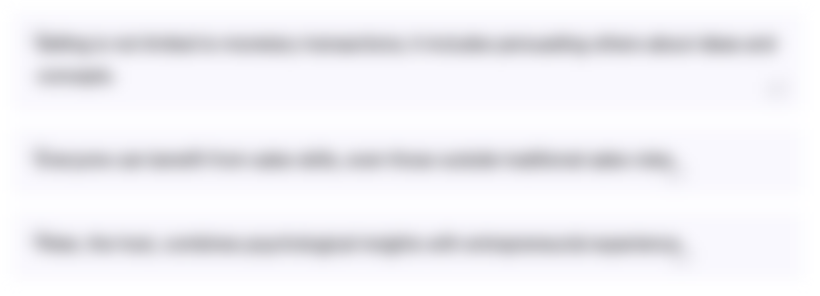
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
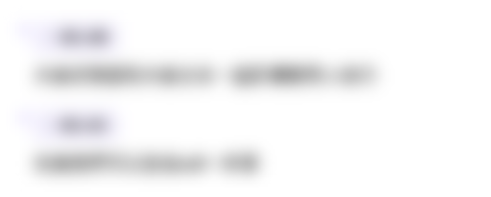
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

How Rendering Works: Overview | Lecture 123 | React.JS π₯
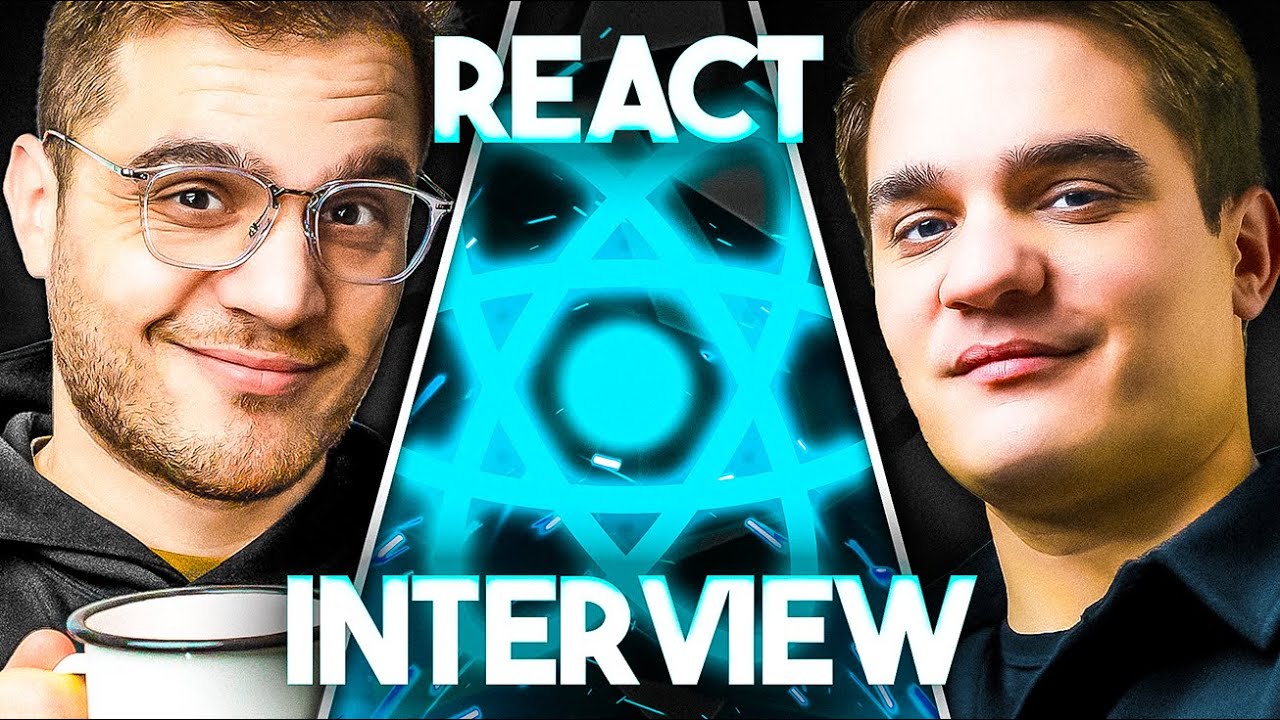
React Interview Questions Senior Level (React Fiber, Reconciliation, Virtual DOM)

How Events Work in React | Lecture 134 | React.JS π₯

State Update Batching | Lecture 132 | React.JS π₯
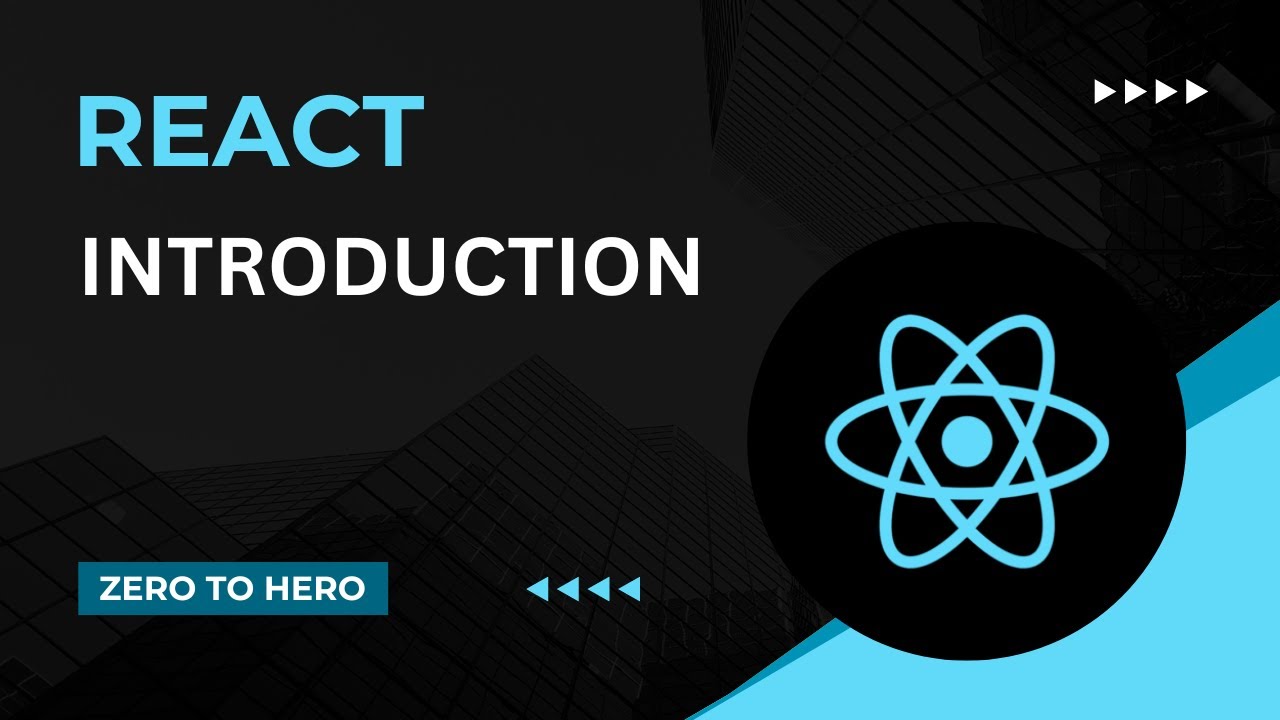
Course Introduction | Mastering React: An In-Depth Zero to Hero Video Series
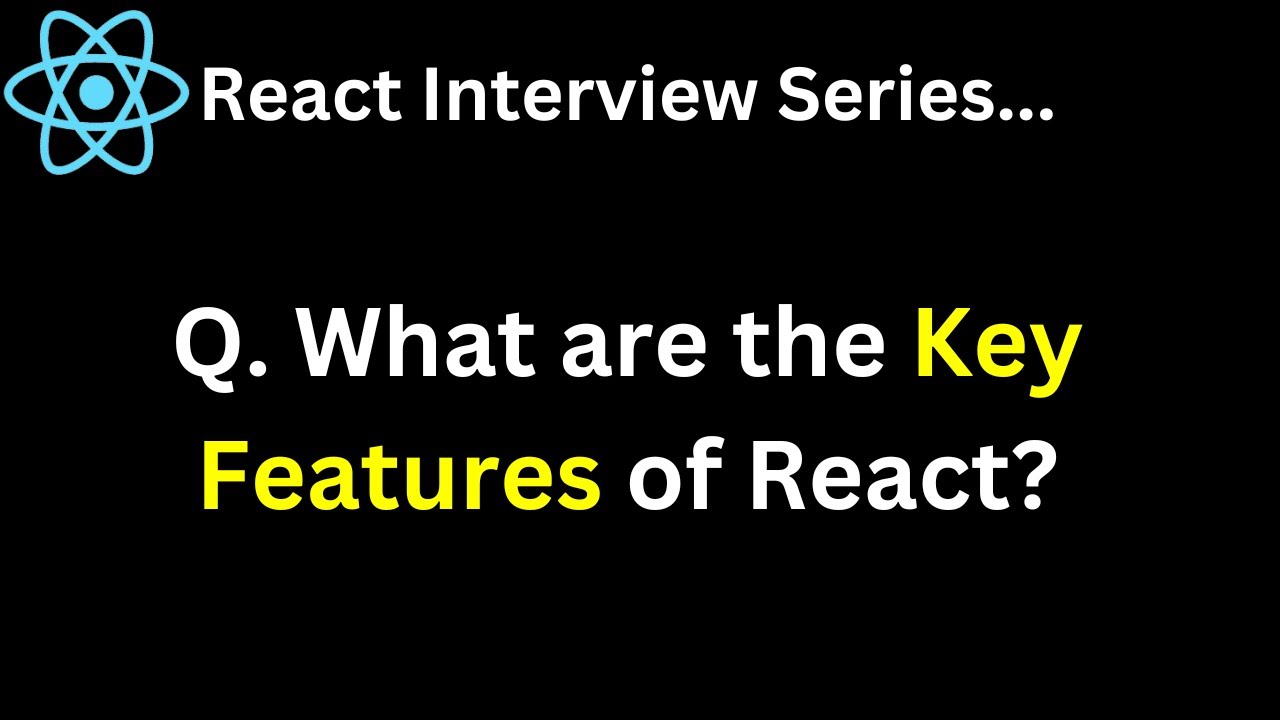
Q. What are the Key Features of React ?
5.0 / 5 (0 votes)