C++ Banking program for beginners π°
Summary
TLDRThis video script outlines the creation of a basic banking program in C++ for educational purposes. It covers the development of functions for depositing, withdrawing, and displaying account balances. The script guides viewers through setting up a user interface with a switch statement to handle different banking options, validating user inputs to prevent negative deposits and overdrafts, and managing the input buffer to avoid errors. The tutorial is designed to help learners understand fundamental programming concepts through a practical banking application example.
Takeaways
- π The video script is a tutorial for creating a basic banking program in C++ for practice purposes.
- πΌ It covers functions for depositing, withdrawing money, and displaying the account balance.
- π The main function initializes a bank account balance to zero and uses a switch statement to handle user choices.
- π’ The 'show balance' function displays the current balance with two decimal places using 'setprecision' and 'fixed' from the iomanip library.
- π° The 'deposit' function prompts the user to enter an amount to deposit and checks for valid positive input before updating the balance.
- π« The 'withdraw' function prevents overdrafts by checking if the withdrawal amount exceeds the current balance and validates against negative inputs.
- π The program uses a do-while loop to continuously prompt the user until they choose to exit.
- π To prevent invalid inputs from breaking the program, it includes input validation and clears the input buffer after each user choice.
- π The script includes prompts and output statements to guide the user through the program's functionality.
- π The tutorial mentions that the complete code will be provided in the comments section for those interested.
- π οΈ The banking program is designed for educational purposes to help practice basic C++ programming concepts.
Q & A
What is the main purpose of the banking program discussed in the script?
-The main purpose of the banking program is for practice, allowing users to deposit money, withdraw money, and show their account balance.
How many parameters does the 'showBalance' function have?
-The 'showBalance' function has one parameter, which is of type double representing the bank account balance.
What is the return type of the 'deposit' function?
-The 'deposit' function does not have any parameters and its return type is double, which represents the amount of money deposited.
What is the role of the 'choice' variable in the main function?
-The 'choice' variable is of type int and is used to determine the user's selection among options like showing balance, making a deposit, making a withdrawal, or exiting the program.
What is the significance of the switch statement in the program?
-The switch statement is used to handle different user choices by examining the 'choice' variable against various cases such as showing balance, depositing money, withdrawing money, or exiting.
How does the program handle user input for the 'deposit' function?
-The program prompts the user to enter the amount to be deposited, reads the input, and then checks if the amount is greater than zero before allowing the deposit to proceed.
What is the purpose of the 'setPrecision' function call in the 'showBalance' function?
-The 'setPrecision' function is used to format the balance output to show two decimal places, ensuring the display of cents along with the dollar amount.
How does the program prevent users from making negative deposits?
-The program includes an if statement in the 'deposit' function that checks if the entered amount is greater than zero; if not, it outputs an error message and returns zero, thus preventing negative deposits.
What is the condition used for the do-while loop in the program?
-The condition for the do-while loop is that the 'choice' should not equal four, as four is used to exit the program.
How does the program handle invalid or non-numeric user input?
-The program clears the input buffer using 'clear' and 'flush' functions after the user's choice to ensure that non-numeric or invalid input does not cause the program to malfunction.
What is the final step added to the program to handle unexpected input?
-The final step added to the program is to clear the input buffer after the user's choice to handle unexpected input like characters or strings, preventing the program from breaking.
Outlines
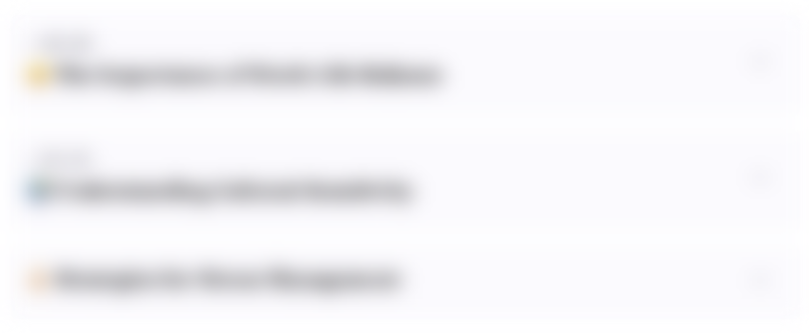
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
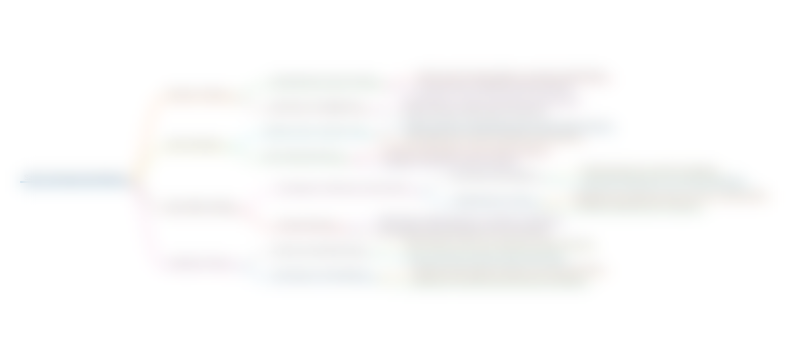
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
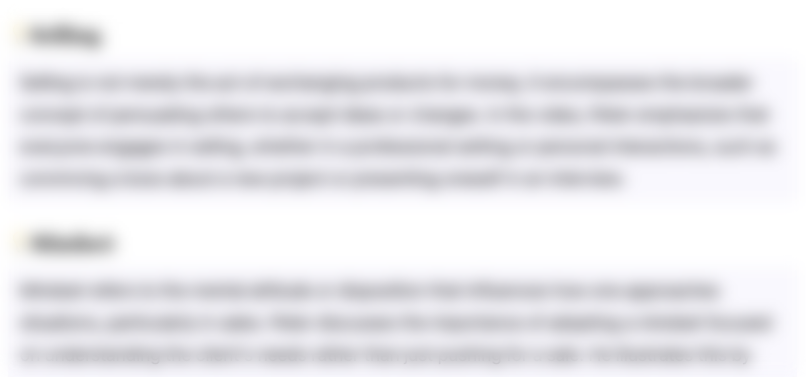
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
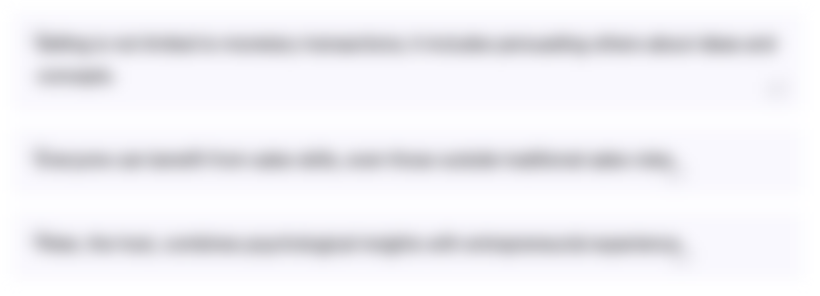
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
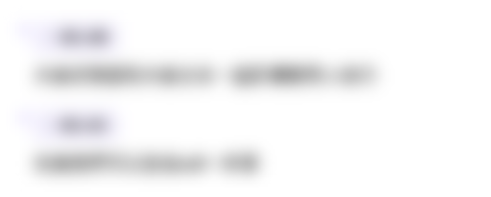
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
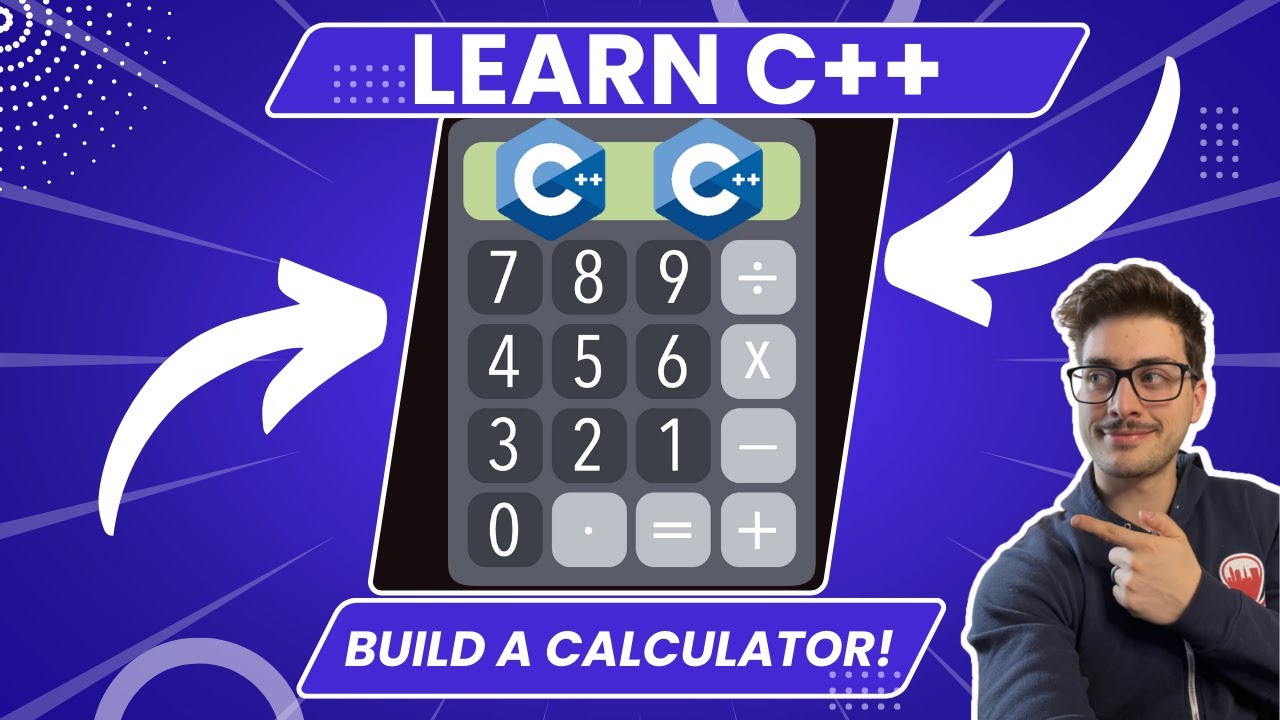
How to Program A Calculator in C++! (Great C++ Microsoft Visual Studio Beginner Project)
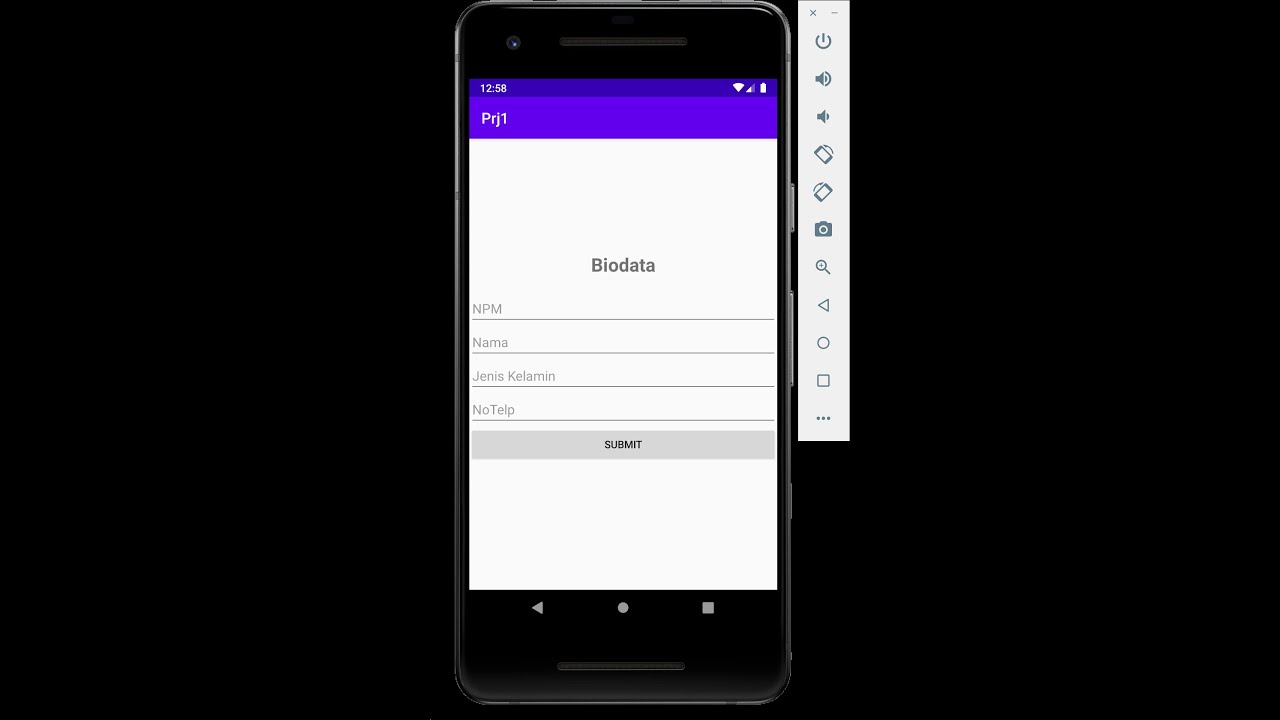
Edit Text, Button, dan Textview Android Studio
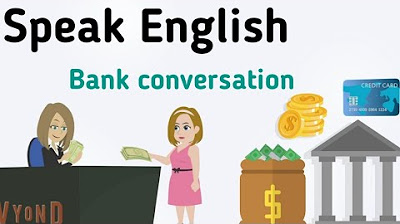
At the bank English conversation | Daily English conversation | Bank vocabulary
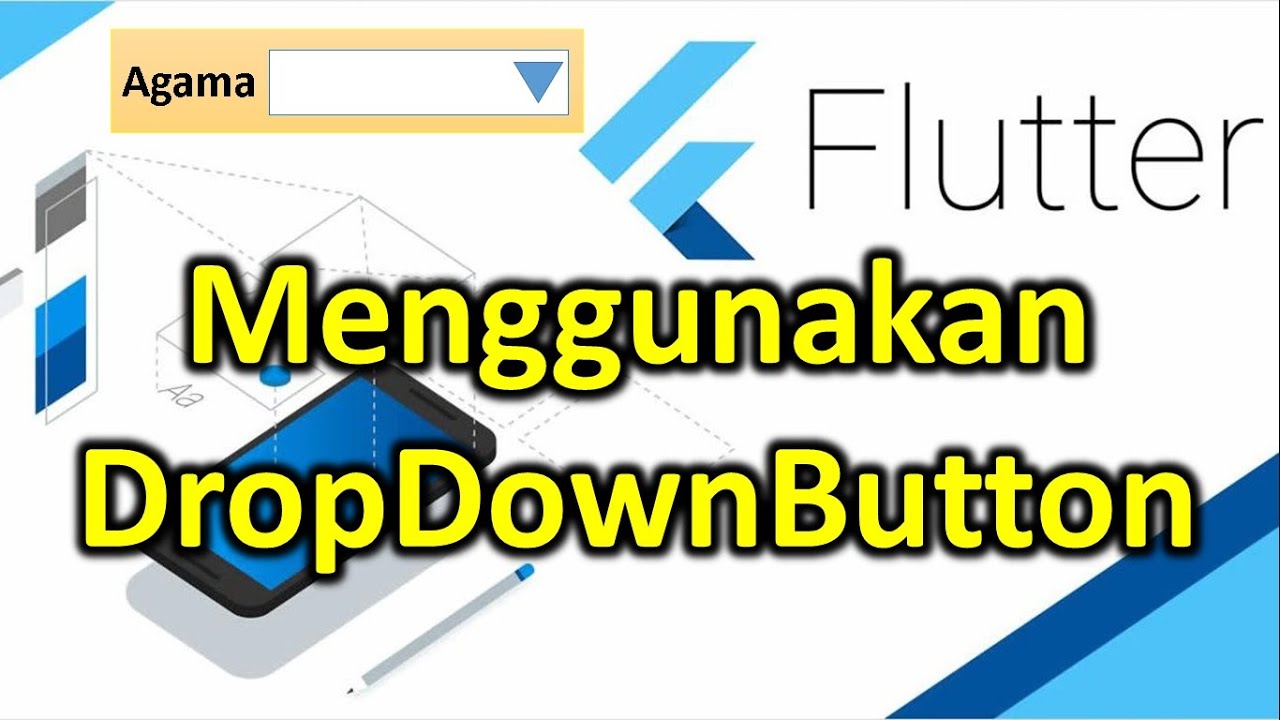
Use Penggunaan Drop Down Button in Flutter | DropDownButton in Flutter
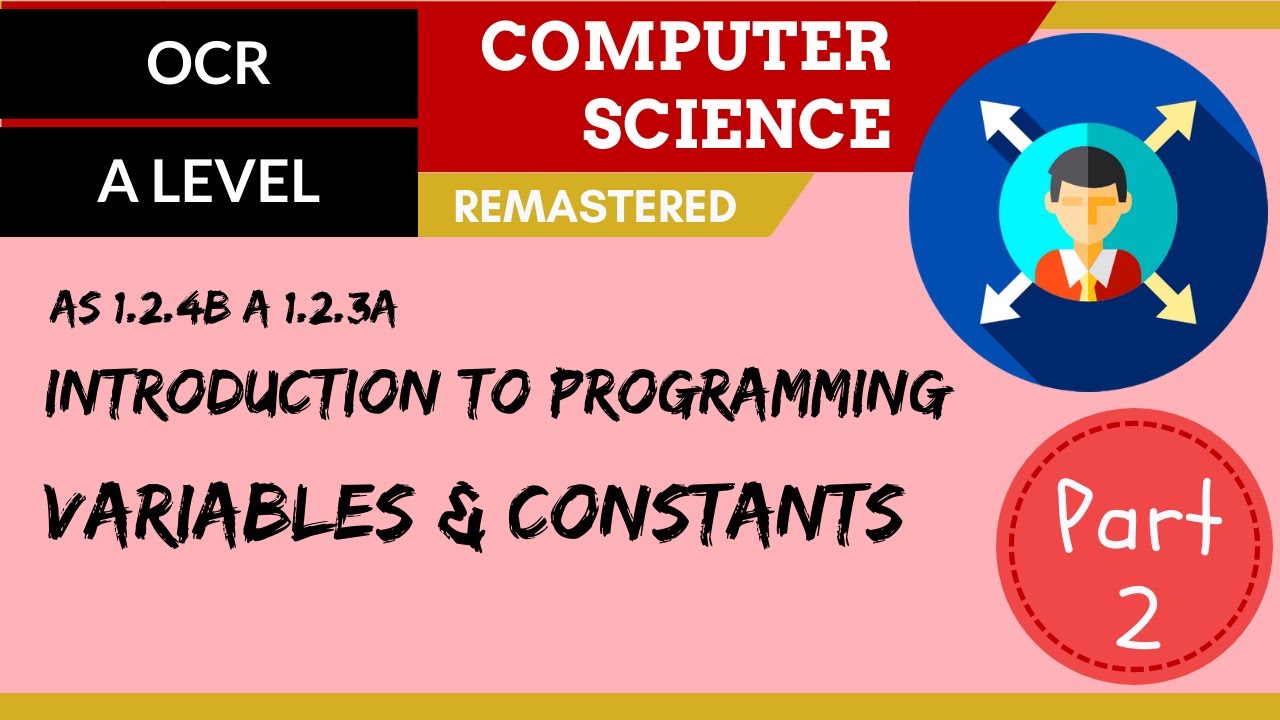
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
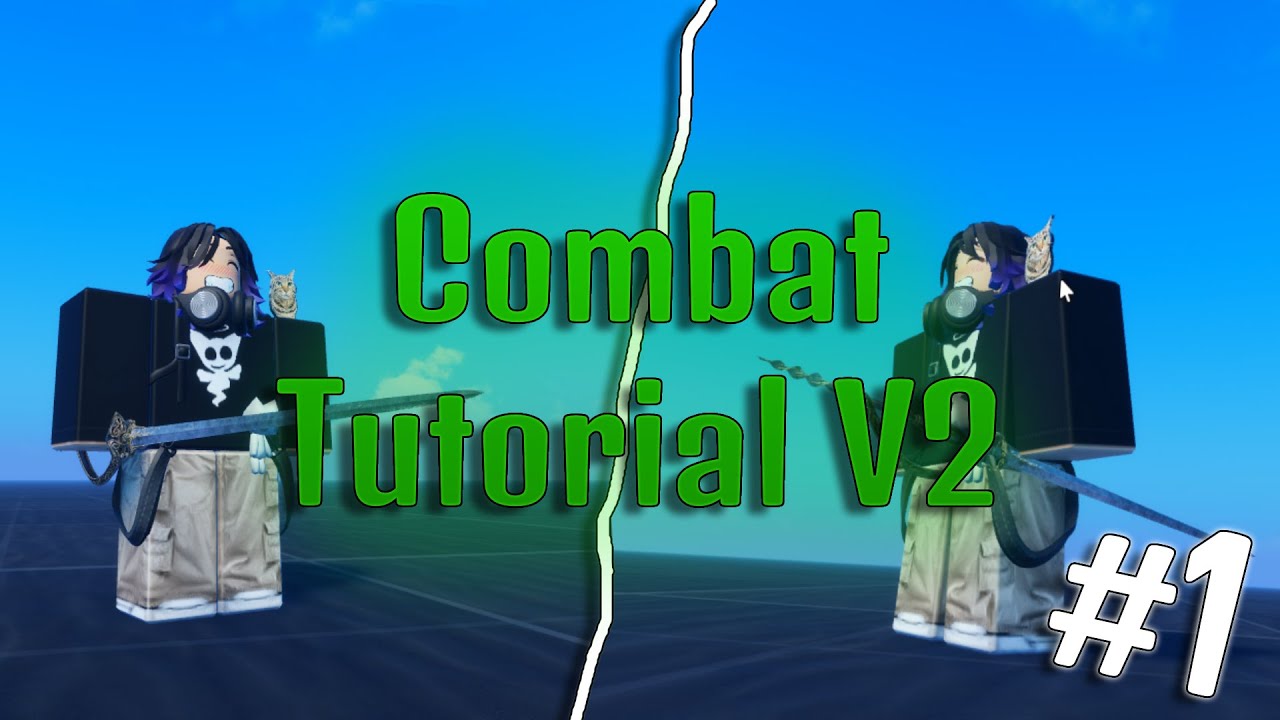
Combat Tutorial V2 #1 - Equipping | Roblox Studio [TUTORIAL]
5.0 / 5 (0 votes)