Balanced Binary Tree - Leetcode 110 - Python
Summary
TLDRIn this educational coding video, the presenter tackles the problem of determining if a binary tree is height-balanced. The script explains the concept of a balanced binary tree, where the height difference between left and right subtrees for every node is at most one. It discusses a naive approach using recursive depth-first search (DFS) to check balance, which results in a time complexity of O(n^2). The presenter then introduces an optimized bottom-up approach, which eliminates redundant work and reduces the time complexity to O(n). The code implementation involves a nested recursive function that returns both a boolean indicating balance and the tree's height, ensuring efficiency and correctness.
Takeaways
- 📚 The video discusses solving the problem of determining if a binary tree is height-balanced.
- 🔍 A height-balanced binary tree is defined as a tree where the difference in height between the left and right subtrees of any node is at most one.
- 👀 The script provides an example of a balanced tree and an example of an unbalanced tree to illustrate the concept.
- 🤔 The naive approach to solving the problem involves recursive depth-first search (DFS) on each subtree, which results in a time complexity of O(n^2).
- 🔄 The script introduces an optimized approach that eliminates repeated work by checking subtrees from the bottom up, resulting in a time complexity of O(n).
- 🛠️ The optimized solution uses a nested recursive function that returns two values: a boolean indicating balance and the height of the subtree.
- 📈 The base case for the recursive function is when the tree is empty (null root), which is considered balanced with a height of zero.
- 🔢 The recursive function calculates the height of the tree as one plus the maximum height of the left and right subtrees.
- 🔄 The script emphasizes that the balance of the entire tree is determined by both the balance of the subtrees and the balance from the root node.
- 👌 The final result of the function is a boolean indicating whether the entire tree is balanced, which is the product of all subtrees being balanced and the root node being balanced.
- 💻 The script concludes with a demonstration of the code working correctly, emphasizing its efficiency.
Q & A
What is a balanced binary tree?
-A balanced binary tree is a tree where, for every node, the height of its left and right subtrees differ by at most one.
How does the height of a binary tree affect its balance?
-The balance of a binary tree is determined by comparing the heights of the left and right subtrees of every node; the difference in height should be no more than one.
What is the naive approach to check if a binary tree is balanced?
-The naive approach involves performing a recursive depth-first search (DFS) on each subtree to determine its height and then comparing the heights of the left and right subtrees at each node.
Why is the naive approach inefficient for checking a binary tree's balance?
-The naive approach is inefficient because it requires visiting every node in the tree multiple times, leading to a time complexity of O(n^2), where n is the number of nodes.
What is the optimized approach to determine if a binary tree is balanced?
-The optimized approach is a bottom-up recursive method that checks subtree balance and height simultaneously, ensuring each node is visited at most once, resulting in a time complexity of O(n).
How does the optimized approach eliminate repeated work?
-The optimized approach eliminates repeated work by checking subtree balance and height during the same recursive call, storing these values to avoid recalculating them when returning to parent nodes.
What are the two values returned by the recursive function in the optimized approach?
-The recursive function returns a pair of values: a boolean indicating whether the subtree is balanced and an integer representing the height of the subtree.
What is the base case for the recursive function in the optimized approach?
-The base case for the recursive function is when the root is null, indicating an empty tree, which is considered balanced with a height of zero.
How does the optimized approach determine the height of a subtree?
-The height of a subtree is determined by taking the maximum height of its left and right subtrees and adding one (for the root node).
What is the final result of the outer function in the optimized approach?
-The outer function returns the boolean value indicating whether the entire binary tree is balanced, based on the results of the recursive depth-first search.
Outlines
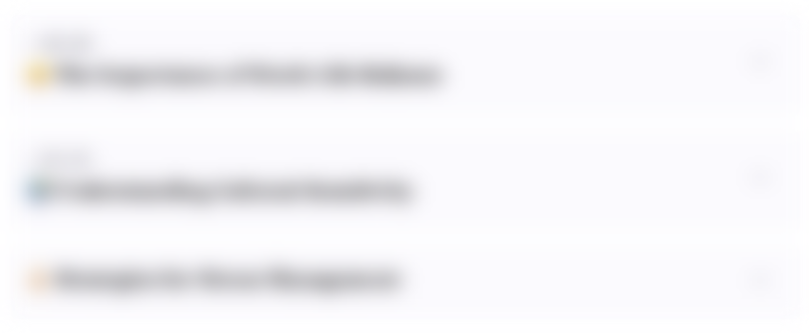
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
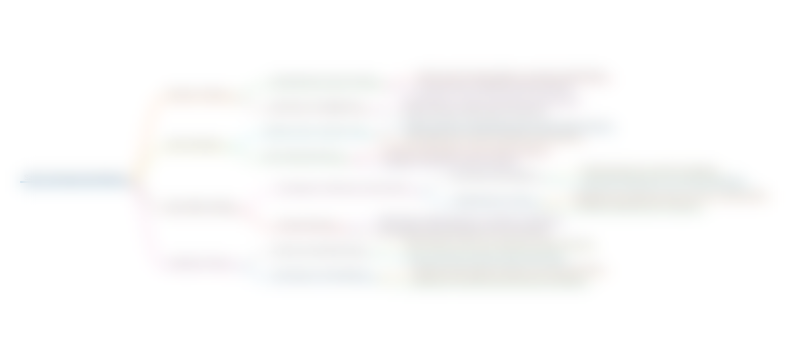
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
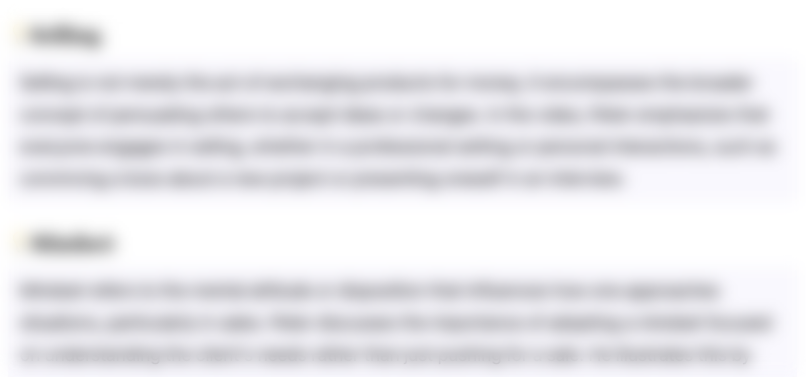
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
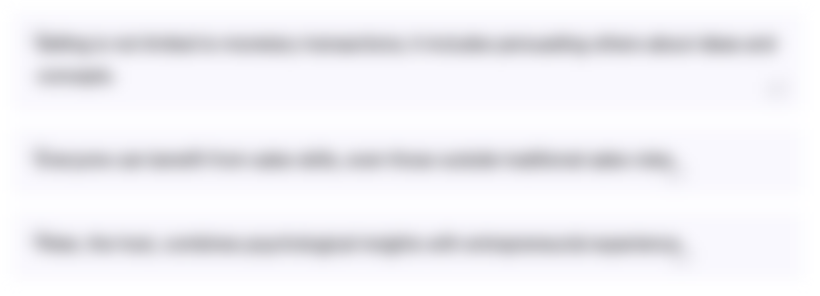
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
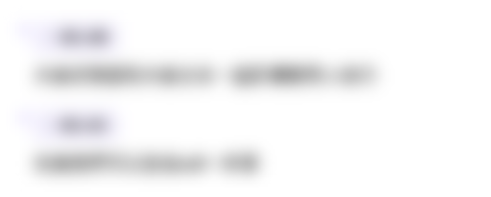
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Lecture 25 : Binary Search Tree (BST) Sort

LeetCode Problem: 226. Invert Binary Tree | Java Solution
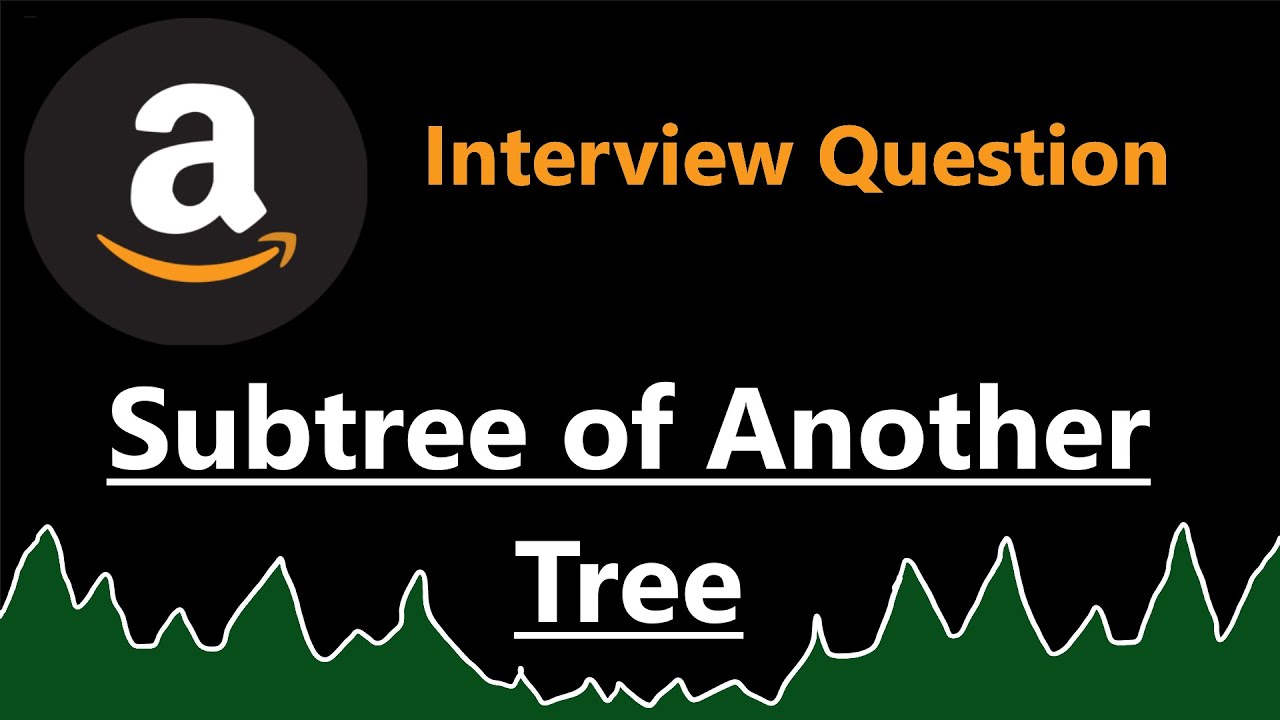
Subtree of Another Tree - Leetcode 572 - Python
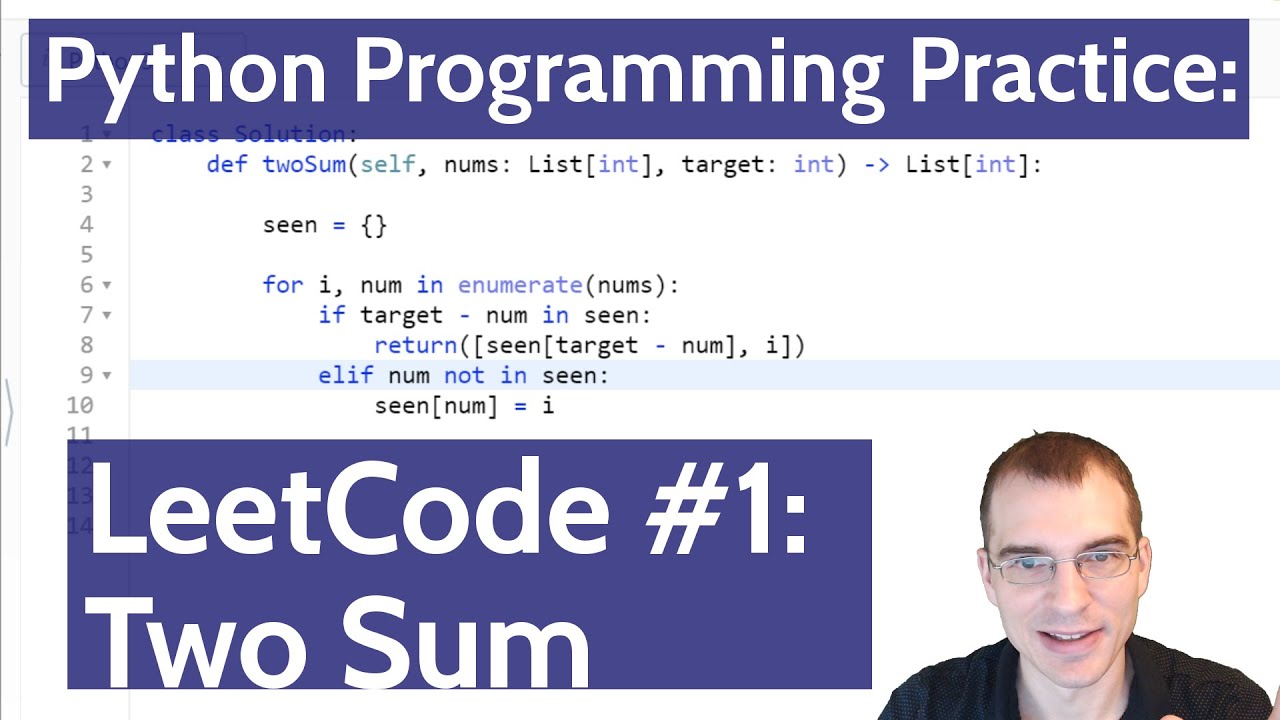
Python Programming Practice: LeetCode #1 -- Two Sum
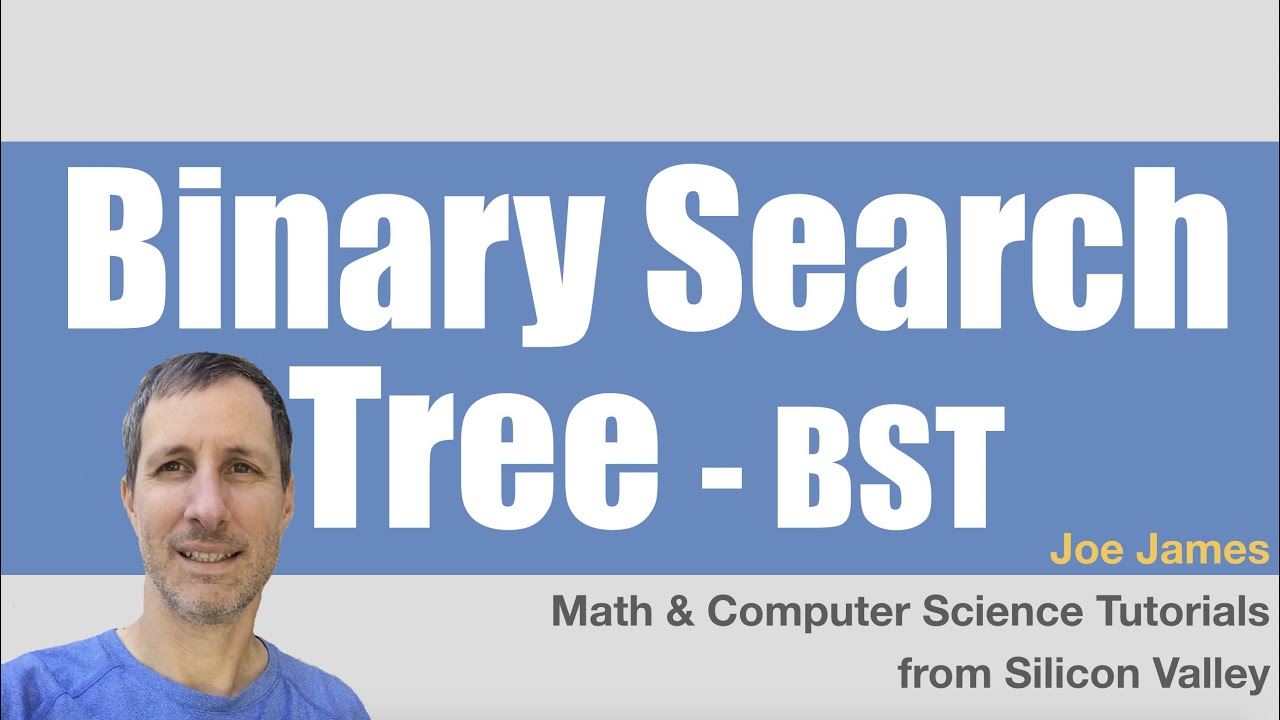
Binary Search Trees (BST) Explained in Animated Demo

8.2 Searching in Arrays | Linear and Binary Search | C++ Placement Course |
5.0 / 5 (0 votes)