Power of Two LeetCode Solution in Java | Optimized O(1) Approach | Placement Interview Questions
Summary
TLDRIn this video, the presenter tackles the 'Power of Two' problem, a popular interview question often asked by companies like Amazon, Google, and Microsoft. The task is to determine if a given number is a power of two in constant time. The video walks through an optimized solution using bitwise operators, explaining how the binary representation of powers of two leads to a quick check using the `n & (n - 1)` operation. The solution is demonstrated in Java, with a focus on efficiency and clarity, offering viewers a solid approach for interview preparation.
Takeaways
- 😀 The 'Power of Two' problem is a common coding interview question, especially asked by top companies like Amazon, Google, and Microsoft.
- 😀 The main challenge in solving this problem is to find an efficient solution with constant time complexity (O(1)).
- 😀 A brute force approach to solve the problem may take O(n) time, which is not optimal for interview scenarios.
- 😀 Bitwise operators (AND, OR, XOR, etc.) can be used to solve the 'Power of Two' problem in constant time.
- 😀 Powers of two in binary have a unique pattern: they contain only one '1' bit, and all other bits are '0'.
- 😀 For a number to be a power of two, performing the bitwise operation `n & (n - 1)` should result in zero.
- 😀 Example binary numbers for powers of two include: 2 (00000010), 4 (00000100), 8 (00001000), etc.
- 😀 The bitwise AND operation works by comparing individual bits, and for powers of two, it yields zero when performed on the number and its decremented value.
- 😀 The Java code implementation uses the `Scanner` class to take input from the user and checks if a number is a power of two using the condition `num & (num - 1) == 0`.
- 😀 The solution is efficient, with a time complexity of O(1) due to the constant time nature of bitwise operations.
- 😀 The video also promotes a DSA with Java course, which covers over 200 placement questions and coding exercises for further practice.
Q & A
What is the main problem discussed in the video?
-The main problem discussed is determining if a given number is a power of two using an optimized approach, specifically in constant time using bitwise operators.
Why is solving the problem in constant time important?
-Solving the problem in constant time (O(1)) is important because it provides a more efficient solution, particularly for large input values, and is often required in coding interviews with top tech companies like Amazon, Google, and Microsoft.
What are some examples of numbers that are powers of two?
-Examples of numbers that are powers of two include 2, 4, 8, 16, 32, 64, etc. These can be represented as 2^1, 2^2, 2^3, 2^4, 2^5, etc.
How can bitwise operators be used to check if a number is a power of two?
-Bitwise operators can be used by performing a bitwise AND between the number and the number minus one (i.e., `n & (n - 1)`). If the result is 0, then the number is a power of two.
What is the binary representation of a number that is a power of two?
-In binary, numbers that are powers of two have a single '1' followed by '0's. For example, 4 is represented as '100', and 8 is represented as '1000'.
What is the significance of using `n & (n - 1)` in the solution?
-The operation `n & (n - 1)` works because for powers of two, the number and its previous value in binary have no overlapping '1' bits. This means the result will be 0 if the number is a power of two.
Can you explain why `n & (n - 1)` results in zero for powers of two?
-For powers of two, the binary representation has a single '1' followed by '0's. Subtracting 1 from a power of two flips the bits after the '1', so performing the AND operation between the number and its previous value will result in zero because there are no common '1' bits.
What are the practical benefits of using bitwise operators in this problem?
-Bitwise operators are efficient and work directly with the individual bits of a number, which allows the solution to run in constant time (O(1)), making it much faster and more suitable for larger input sizes compared to other methods.
What was the approach for writing the program in Java, as explained in the video?
-The approach was to write a simple Java program that uses a Scanner class to take an integer input, then checks if the number is a power of two by applying the `n & (n - 1)` condition, and outputs the result accordingly.
What is the importance of practicing coding questions, as mentioned in the video?
-Practicing coding questions is crucial because it helps you recognize patterns and determine the most efficient approaches for solving problems. As the speaker mentions, with enough practice, you'll develop an instinct for when to apply specific techniques, such as bitwise operators.
Outlines
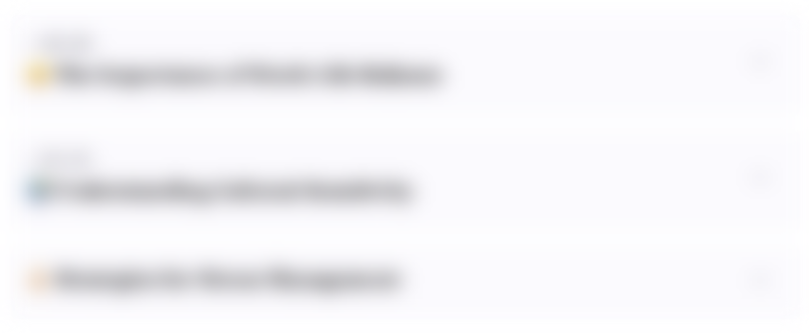
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
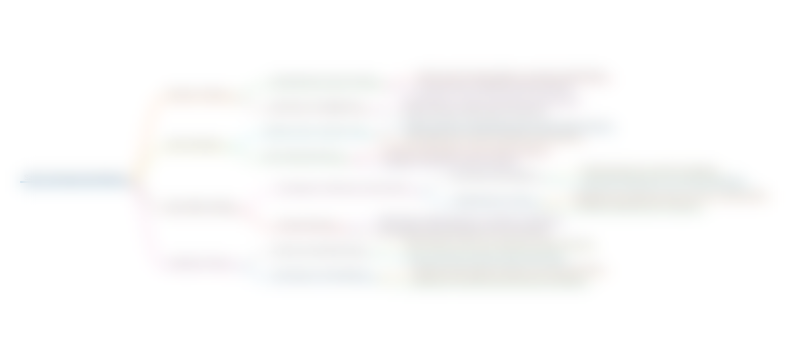
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
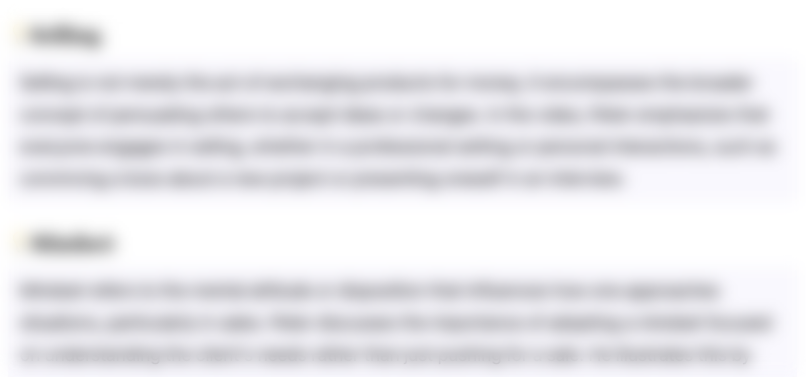
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
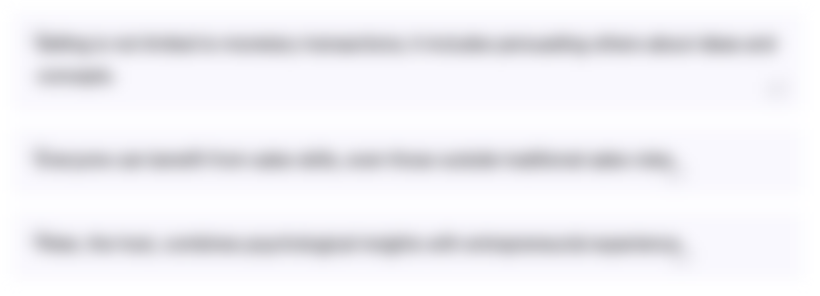
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
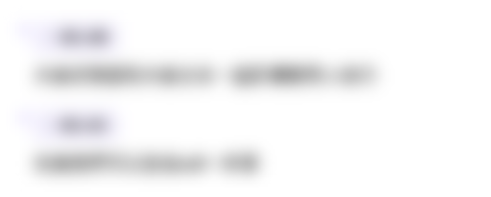
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

The Top 10 Product Manager Interview Questions (And How To Answer Them)

How to Identify Patterns in DSA Problems? | 6 Type of Interval Problems Asked in FAANG Interviews
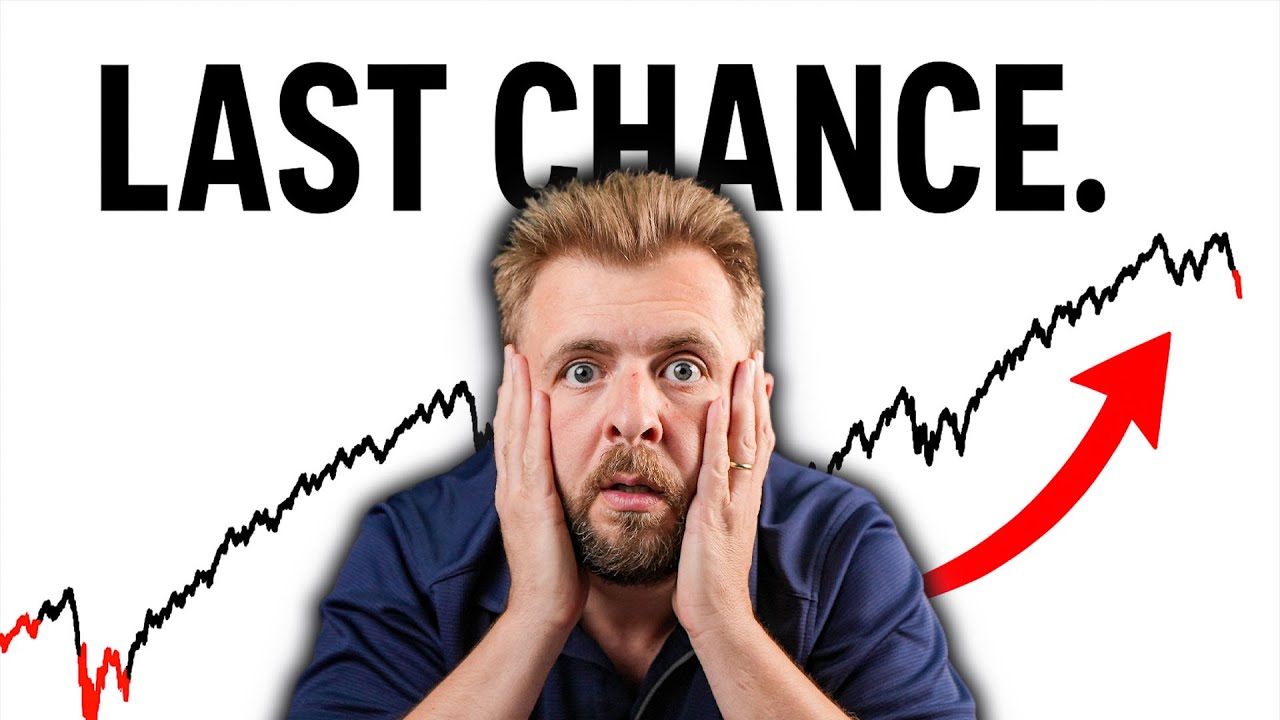
An Opportunity Like This Won't Come Again... (Emergency Update)

THIS Leetcode Strategy Got Me Multiple Big Tech Offers.
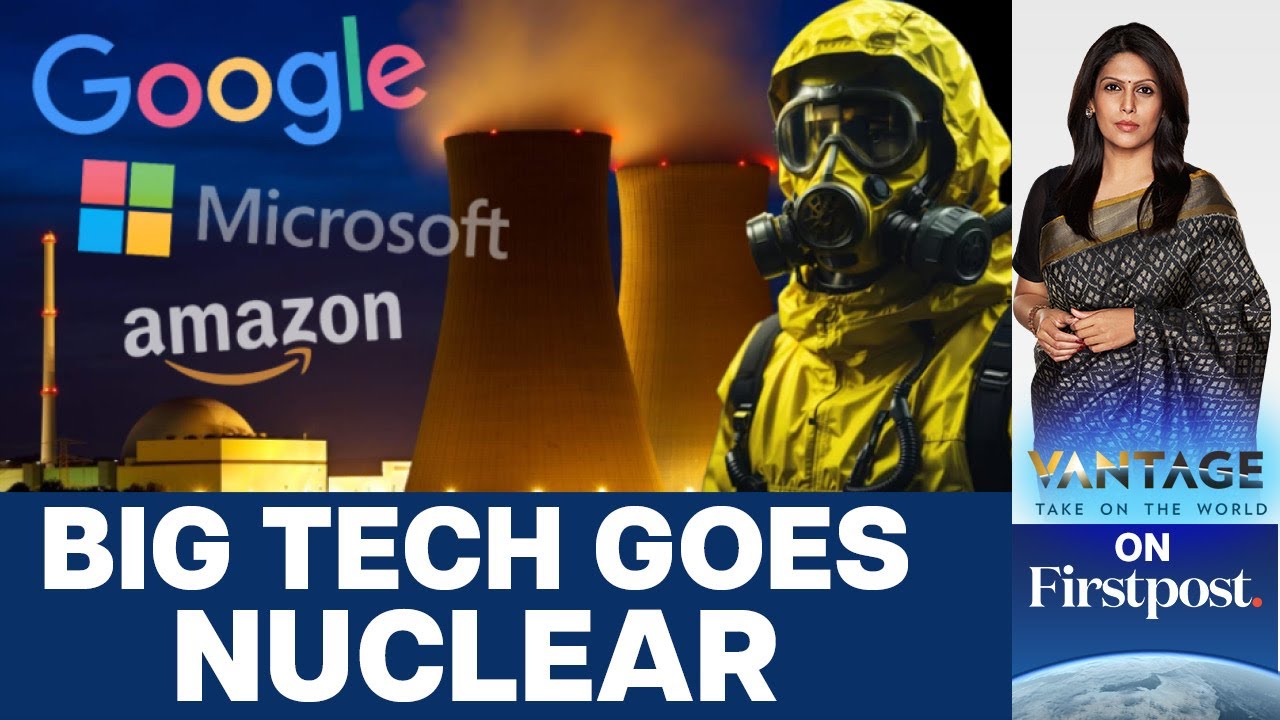
Why Tech Giants are Turning to Nuclear Energy to Power AI | Vantage with Palki Sharma

TECNOFEUDALISMO: O CAPITALISMO JÁ FOI SUPERADO?
5.0 / 5 (0 votes)