SUPER() in Python explained! 🔴
Summary
TLDRThis video script explains the concept and utility of the 'super' function in Python, which is instrumental in a child class to invoke methods from a parent class. It demonstrates the function's role in avoiding code redundancy by utilizing inheritance, showcasing how to define constructors and methods in a 'Shape' parent class and its child classes like 'Circle', 'Square', and 'Triangle'. The script also illustrates method overriding and extending functionality of inherited methods using 'super', providing practical examples of object instantiation and method calls to clarify these concepts.
Takeaways
- 📚 The 'super()' function in Python is used to call methods from a parent class within a child class, promoting code reusability.
- 👨👧 The child class is often referred to as a 'subclass', and the parent class as the 'superclass', which is the origin of the 'super' function's name.
- 🔄 'super()' allows for extending the functionality of inherited methods, which can be useful for method overriding or extending.
- 📐 The script provides an example using shape classes like Circle, Square, and Triangle, demonstrating how attributes like color and fill status can be inherited.
- 🔧 The use of 'super()' eliminates the need to manually assign common attributes in each child class constructor, streamlining the code.
- 🏗️ By placing shared attributes in a parent class, any changes to these attributes need to be made only once, reducing the potential for errors.
- 📝 The script illustrates how to use 'super()' to call the parent class constructor, passing necessary arguments to initialize child class objects.
- 🎨 It demonstrates object instantiation with attributes like color, fill status, and specific shape dimensions, using the 'super()' function for clarity.
- 📈 The 'super()' function can also be used to extend the functionality of a method, as shown with the 'describe' method in the shape classes.
- 🛠️ Method overriding is shown where a child class has a similar method to the parent class, and the child's version is used instead of the parent's.
- 📚 The script concludes by emphasizing the utility of 'super()' in both constructors for attribute assignment and in methods for functionality extension.
Q & A
What is the purpose of the 'super' function in Python?
-The 'super' function in Python is used within a child class to call methods from a parent class. It allows for the extension of functionality of inherited methods and helps in reusing code to avoid redundancy.
What is the term used for the class that inherits from another class?
-The class that inherits from another is called a 'subclass' or 'child class', while the class from which it inherits is referred to as the 'superclass' or 'parent class'.
What are some attributes that might be common among different shape classes like Circle, Square, and Triangle?
-Common attributes among shape classes could include 'color' and 'is filled', which are shared by all shapes regardless of their specific geometric properties.
How does the script suggest handling attributes that are unique to each shape class?
-The script suggests defining unique attributes like 'radius' for Circle, 'width' for Square, and 'width' and 'height' for Triangle within their respective class constructors after calling the superclass constructor with 'super'.
What is the advantage of using 'super' in the constructors of child classes?
-Using 'super' in the constructors of child classes allows the child class to inherit the initialization of common attributes from the parent class, reducing the need for repetitive code and potential for errors.
Can you provide an example of how to instantiate a Circle object with the given script?
-A Circle object can be instantiated by calling `Circle(color='red', is_filled=True, radius=5)`, which passes the color, fill status, and radius to the Circle class constructor.
What is the purpose of the 'describe' method in the script?
-The 'describe' method is used to print out the attributes of a shape, such as its color and whether it is filled or not. It can also be overridden or extended in child classes to include additional information specific to the shape type.
How does method overwriting work in the context of the script?
-Method overwriting occurs when a child class defines a method with the same name as a method in the parent class. In such cases, the child class's version of the method is used instead of the parent's.
What is the significance of using 'super' to extend the functionality of a method in a child class?
-Using 'super' to extend the functionality of a method allows the child class to call the parent class's method and then add additional functionality on top of it, combining the behaviors of both methods.
Can you explain how the script demonstrates the concept of inheritance and method overriding with the shape classes?
-The script demonstrates inheritance by having Circle, Square, and Triangle classes inherit common attributes from a Shape parent class. Method overriding is shown by redefining the 'describe' method in each child class to include specific attributes like area calculations, while still being able to call the parent class's 'describe' method using 'super'.
What is the practical application of using 'super' for both constructors and methods in Python classes?
-Using 'super' for constructors ensures that common attributes are initialized properly across all subclasses, while using it for methods allows for extending or modifying inherited behavior without losing the functionality of the parent class, promoting code reusability and maintainability.
Outlines
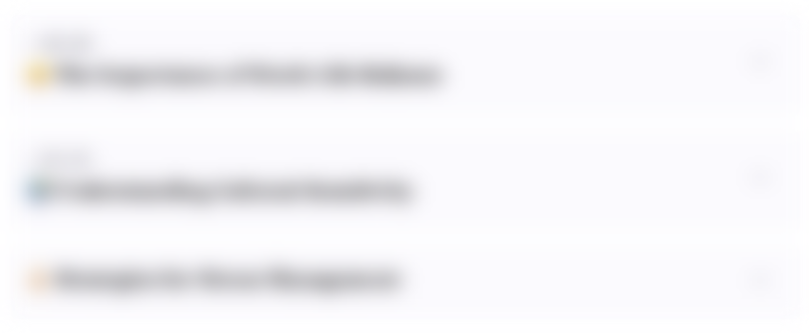
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
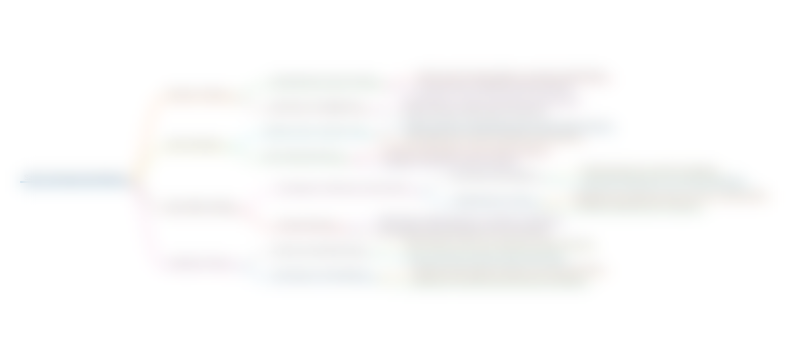
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
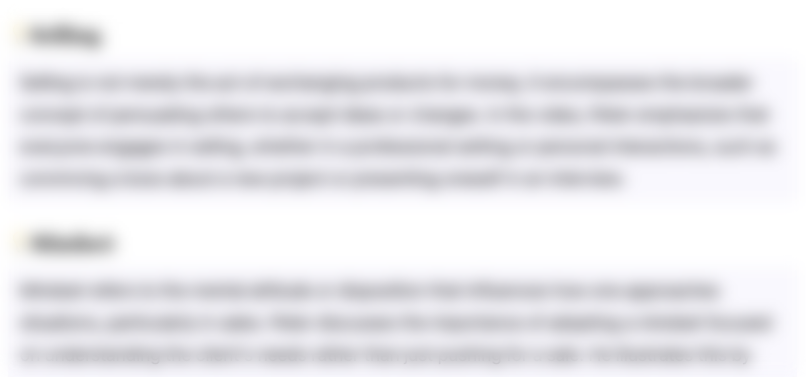
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
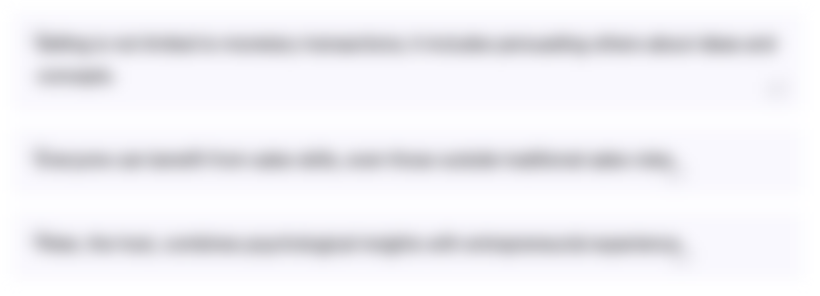
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
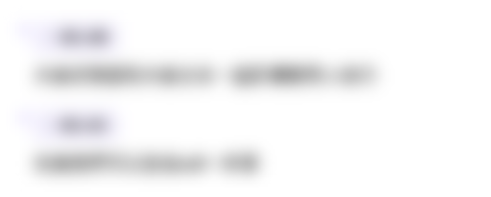
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)