Two Sum - Leetcode 1 - HashMap - Python
Takeaways
- 😀 Дано массив чисел и целевое число, необходимо найти два значения в массиве, сумма которых равна целевому числу.
- 😀 Возвращаем индексы этих двух значений.
- 😀 Имеется гарантированное одно решение, не нужно беспокоиться о множественных решениях.
- 😀 Базовое решение - проверка всех возможных пар значений, что приводит к сложности O(N^2).
- 😀 Более эффективное решение - использование хеш-таблицы для хранения значений и их индексов.
- 😀 При использовании хеш-таблицы проверяется, существует ли нужное значение (разница между целевым числом и текущим значением) в хеш-таблице.
- 😀 Если нужное значение найдено в хеш-таблице, возвращаются индексы двух значений.
- 😀 Проход по массиву выполняется за один раз, добавляя значения в хеш-таблицу по мере их обхода.
- 😀 Алгоритм имеет временную сложность O(N) и требует дополнительной памяти O(N) для хеш-таблицы.
- 😀 Код решения состоит из создания хеш-таблицы, итерации по массиву, проверки наличия нужного значения в хеш-таблице и возврата индексов.
Q & A
Какова основная цель задачи, обсуждаемой в видео?
-Найти два значения в заданном массиве, которые в сумме дают целевое значение (в данном случае 9), и вернуть их индексы.
Какой самый интуитивный способ решения этой задачи?
-Самый интуитивный способ решения - это перебор всех возможных комбинаций двух значений и проверка, дают ли они в сумме целевое значение.
Какова временная сложность алгоритма полного перебора?
-Временная сложность алгоритма полного перебора составляет O(n^2), где n - длина массива.
Какой более эффективный способ решения предложен в видео?
-Более эффективный способ решения - использовать хэш-таблицу (hash map) для хранения значений и их индексов, чтобы проверять наличие необходимого значения за константное время.
Какую структуру данных рекомендуется использовать для улучшения эффективности алгоритма?
-Рекомендуется использовать хэш-таблицу (hash map).
Какова временная сложность предложенного в видео алгоритма с использованием хэш-таблицы?
-Временная сложность предложенного алгоритма составляет O(n), где n - длина массива.
Какова пространственная сложность алгоритма с использованием хэш-таблицы?
-Пространственная сложность алгоритма составляет O(n), так как в хэш-таблицу может быть добавлено до n элементов.
Почему хэш-таблицу можно изначально оставить пустой?
-Хэш-таблицу можно оставить пустой, так как элементы добавляются в неё по мере их обработки, и нужные пары будут найдены в процессе одной итерации по массиву.
Как хэш-таблица помогает избежать повторного использования одного и того же элемента?
-Хэш-таблица помогает, так как при поиске пары для текущего элемента проверяется, существует ли разность между целевым значением и текущим элементом в хэш-таблице, но при этом текущий элемент ещё не добавлен в неё.
Каковы основные шаги алгоритма с использованием хэш-таблицы?
-1. Инициализация пустой хэш-таблицы. 2. Проход по массиву. 3. Для каждого элемента проверка, есть ли в хэш-таблице элемент, равный разности между целевым значением и текущим элементом. 4. Если такой элемент найден, вернуть индексы. 5. Если не найден, добавить текущий элемент в хэш-таблицу.
Outlines
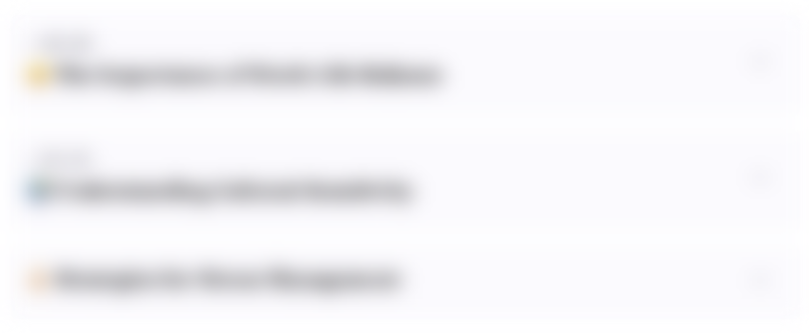
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
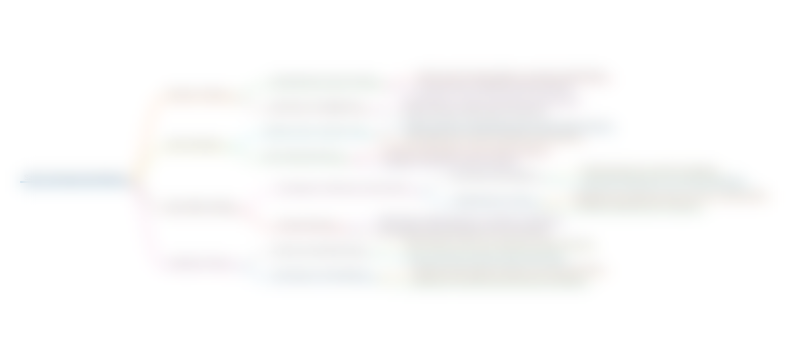
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
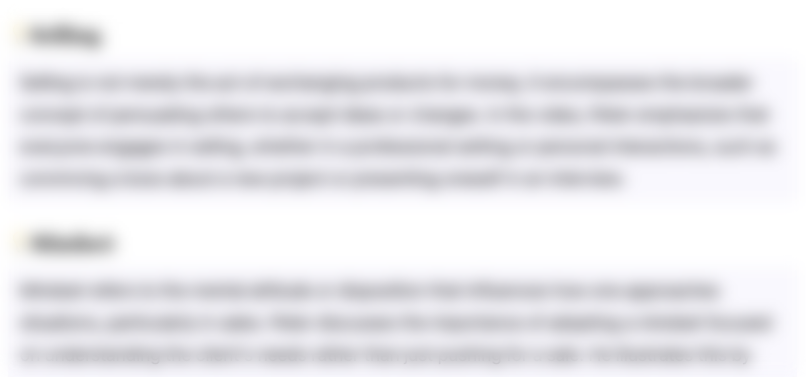
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
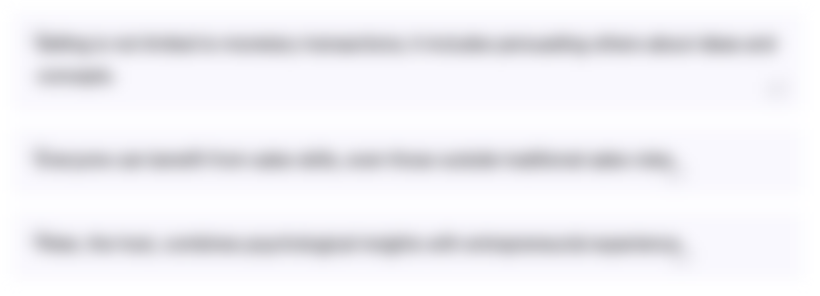
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
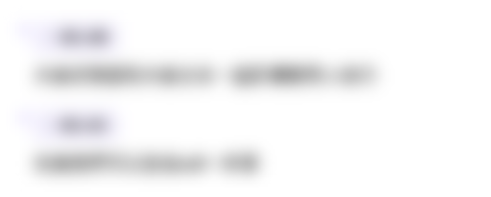
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)