NW LAB 1. Basics of Socket Programming in C : TCP and UDP - Program Demo
Summary
TLDRThis video tutorial delves into the fundamentals of socket programming in C, showcasing TCP and UDP implementations. It explains sockets as endpoints for network communication, detailing their structure with IP addresses and port numbers. The video distinguishes between datagram and stream sockets, utilizing UDP and TCP respectively, and touches on raw sockets for security applications. It proceeds to illustrate the Berkeley Socket API, covering essential socket functions and their usage in client-server models. The script also includes practical examples of TCP and UDP server-client communication, demonstrating the process from socket creation to data transfer and closure.
Takeaways
- 🔌 A socket is an endpoint for two-way communication between programs on a network, providing a bi-directional FIFO communication facility.
- 📍 Each socket has a unique address composed of an IP address and a port number, crucial for establishing connections in client-server applications.
- 🛠️ There are three main types of sockets: datagram (UDP), stream (TCP), and raw sockets, each with distinct transport layer protocols and use cases.
- 🔗 TCP provides a connection-oriented, reliable, and bi-directional protocol with acknowledgements and retransmissions, ensuring data integrity.
- 🌐 UDP is a connectionless protocol without the guarantees of order, acknowledgements, or duplicate protection, offering less overhead.
- 🔍 Raw sockets allow for direct IP packet manipulation, used in security and diagnostic tools like Nmap, IGMP, and ICMP utilities.
- 📚 Berkeley sockets, the focus of the script, use the Berkeley Socket API, which is demonstrated through various socket functions in the script.
- 🔧 Key socket functions include socket creation, binding, listening (for servers), connecting (for clients), and data transfer via send/receive or sendto/recvfrom.
- 📡 The script explains the importance of network byte order for port and address specifications in socket functions, contrasting with host byte order.
- 🔄 Functions like htons and inet_addr are used for converting host data to network byte order and IP addresses to a format usable in socket operations.
- 📝 The script outlines a step-by-step implementation of both TCP and UDP server and client programs, detailing the process of creating sockets, handling connections, and data transfer.
- 🖥️ The video concludes with demonstrations of running TCP and UDP server and client programs, showing practical application of the discussed concepts.
Q & A
What is a socket in the context of network programming?
-A socket is one endpoint of a two-way communication link between two programs running on a network, providing a bi-directional FIFO (First In, First Out) communication facility over the network.
What is the purpose of the 'socket' function in C?
-The 'socket' function in C is used to create an endpoint for communication and returns a file descriptor that refers to that endpoint.
What are the two main types of sockets used in transport layer protocols?
-The two main types of sockets used in transport layer protocols are datagram sockets, which use UDP, and stream sockets, which use TCP.
What is the difference between TCP and UDP protocols?
-TCP (Transmission Control Protocol) is a connection-oriented protocol that is reliable and bi-directional, ensuring data integrity with acknowledgements and retransmissions. UDP (User Datagram Protocol) is a connectionless protocol that does not guarantee data delivery, allowing packets to arrive out of order and duplicates to occur.
What is a raw socket and what is it used for?
-A raw socket allows direct sending and receiving of IP packets without any protocol-specific transport layer formatting. It is used in security-related applications like network scanners (e.g., nmap) and in routing protocols like IGMP and ICMP.
What are the three arguments of the 'bind' function in socket programming?
-The 'bind' function has three arguments: the socket descriptor, a pointer to the 'sockaddr_in' structure, and the length of that structure.
What is the purpose of the 'listen' function in TCP socket programming?
-The 'listen' function marks a socket as a passive socket that will be used to accept incoming connection requests, with a specified maximum length for the queue of pending connections.
How does the 'connect' function in socket programming work?
-The 'connect' function attempts to establish a connection to a specified socket. If successful, it returns 0; otherwise, it returns -1 indicating an error.
What is the role of the 'accept' function in server-side TCP socket programming?
-The 'accept' function is used on the server side to extract the first connection request from the queue of pending connections for the listening socket and create a new connected socket, returning a new file descriptor for the connection.
What are the two flags mentioned for the 'send' function in TCP socket programming?
-The two flags mentioned for the 'send' function are 'MSG_MORE', which indicates that the caller has more data to send, and 'MSG_EOF', which is used for terminating a record.
What is the difference between the 'send' and 'sendto' functions in socket programming?
-The 'send' function is used in connection-oriented protocols like TCP, while 'sendto' is used in connectionless protocols like UDP. 'sendto' requires additional parameters for the destination address and its length.
What is the purpose of the 'inet_addr' function in socket programming?
-The 'inet_addr' function converts a string in standard IPv4 dotted decimal notation to an integer value suitable for use as an Internet address.
What is the significance of network byte order in socket programming?
-Network byte order is a method of sorting bytes that is independent of specific machine architecture and is used to ensure that data is transmitted in a consistent format across different systems. It is always defined to be big endian.
What is the role of the 'close' function in socket programming?
-The 'close' function is used to deallocate the file descriptor associated with a socket. If executed successfully, it returns 0; otherwise, it returns -1 indicating an error.
Outlines
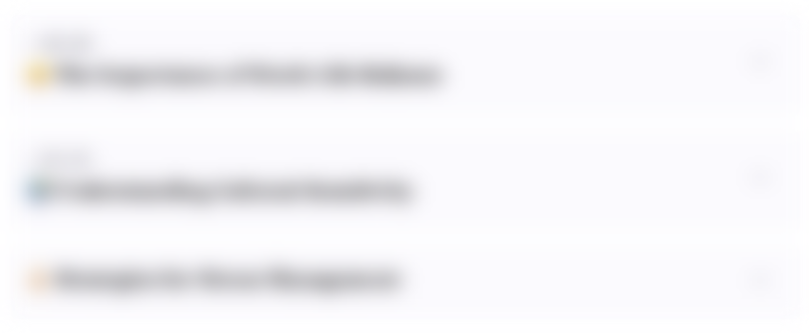
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
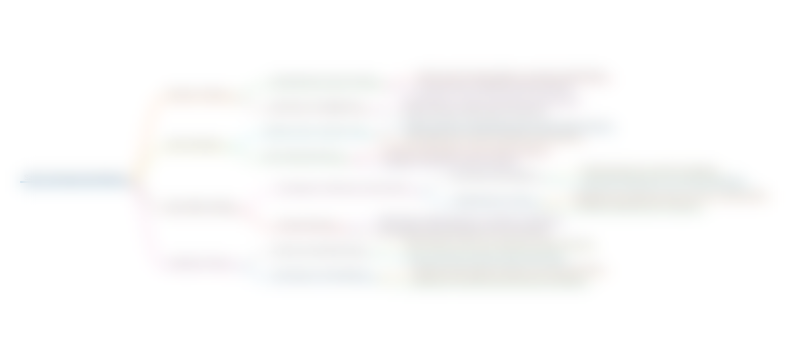
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
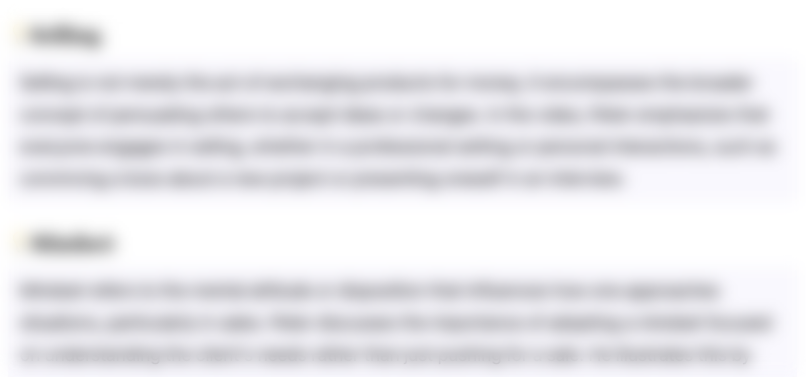
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
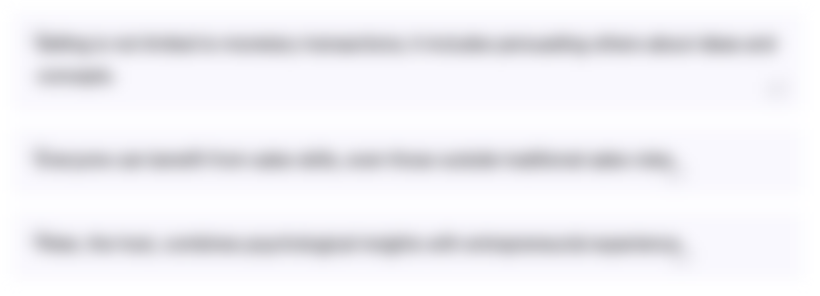
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
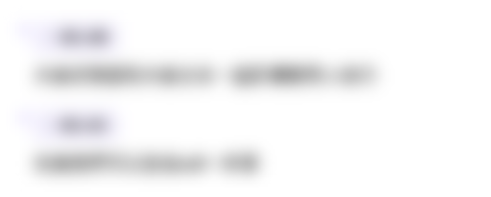
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Sockets Tutorial with Python 3 part 1 - sending and receiving data

Advanced Networking - #7 TCP/IP & UDP/IP [EN]

Introduction to IP - CompTIA A+ 220-1001 - 2.1
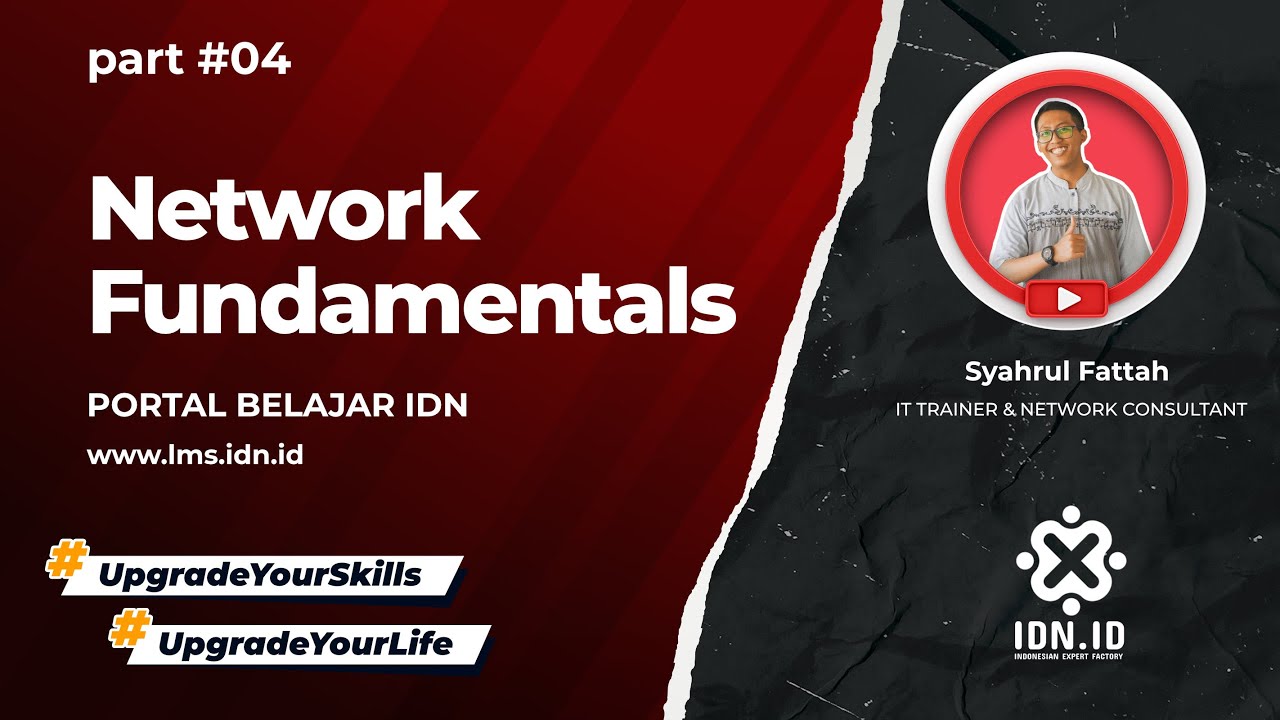
Belajar Dasar Jaringan Komputer dari nol - Part 4/8 | Protocol dan Port
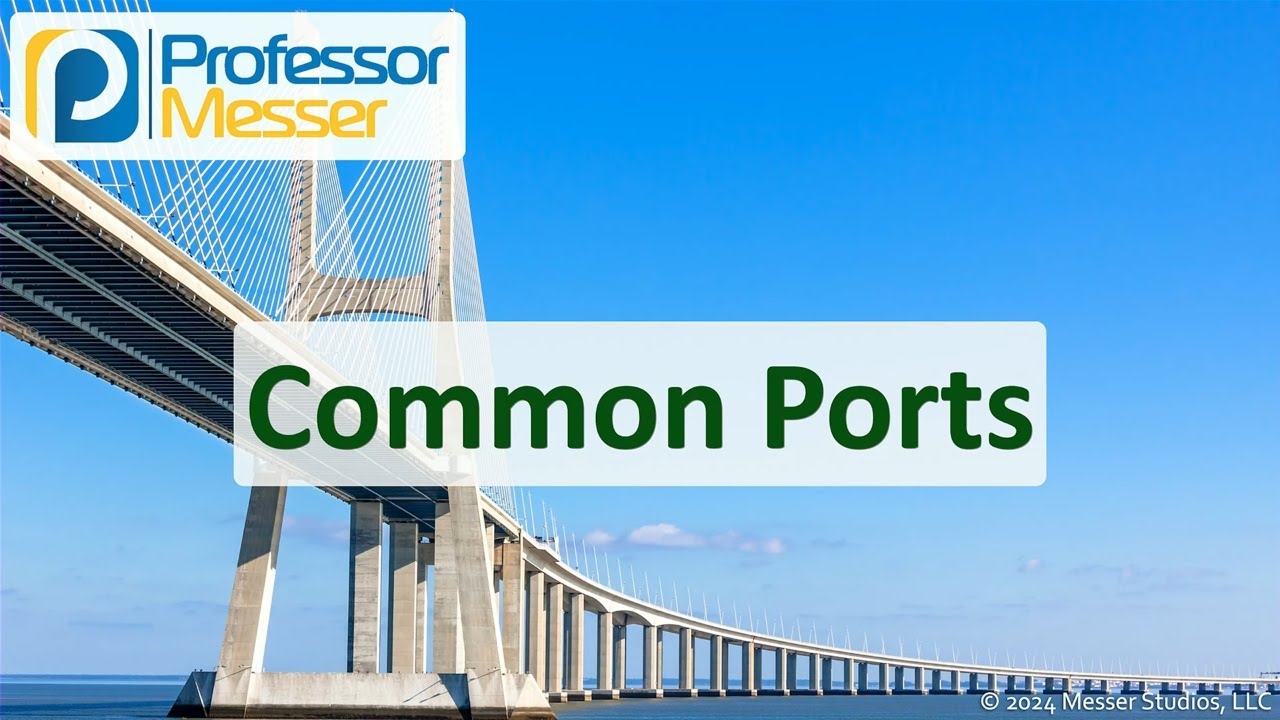
Common Ports - CompTIA Network+ N10-009 - 1.4
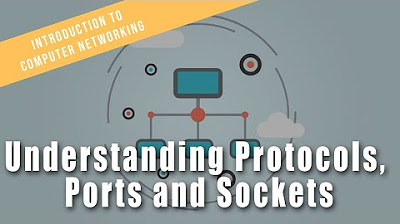
Understanding Protocols, Ports and Sockets | Intro to Computer Networks Course Preview
5.0 / 5 (0 votes)