GCSE Computer Science Python #2 - Inputs, Outputs and Operators
Summary
TLDRThis tutorial introduces the basics of programming with Python, focusing on inputs, outputs, and operators. It demonstrates how to take user inputs, process them to calculate values (like the area of a room), and display results using print statements. The video covers essential Python functions like 'input' and 'print' and explains how to handle data types, including converting input strings to integers. Additionally, it explores mathematical operators (addition, subtraction, multiplication, division) and comparison operators (equal, not equal, greater than, etc.), finishing with logical operators ('and' and 'or') for more complex conditions.
Takeaways
- 😀 User input in Python is taken using the built-in 'input' function, which retrieves data as strings.
- 😀 To perform calculations with user input, the input data must be converted from string to integer using the 'int()' function.
- 😀 Operators in Python, such as multiplication ('*'), addition ('+'), subtraction ('-'), and division ('/'), are used to perform basic mathematical operations.
- 😀 The 'print()' function in Python allows the output of results, such as calculated values, to the user.
- 😀 The area of a room can be calculated by multiplying the length by the breadth, which are taken as inputs from the user.
- 😀 Mathematical operations like division can result in decimal values, while integer division ('//') rounds the result down to the nearest whole number.
- 😀 The modulus operator ('%') in Python returns the remainder after division, useful for determining if a number is divisible by another.
- 😀 Python comparison operators include '==', '!=', '>', '<', '>=', and '<=' to compare values and return boolean results (True or False).
- 😀 The logical operators 'and' and 'or' are used to combine multiple conditions in comparisons, with 'and' requiring all conditions to be true, and 'or' requiring at least one condition to be true.
- 😀 It's essential to practice and experiment with these operators and functions in a Python editor to fully understand their behavior and applications.
Q & A
What is the purpose of the input function in Python?
-The input function in Python is used to take input from the user, which can then be used for further processing or calculations within the program.
Why do we need to convert user input to an integer for calculations?
-By default, the input function retrieves the input as a string. Since mathematical operations require numerical values, we need to convert the input into an integer using the 'int()' function.
What is the role of the 'print' function in this script?
-The 'print' function is used to output the result of a calculation or to display any information to the user. In this case, it is used to show the area of the room after the calculation.
What does the 'asterisk (*)' operator represent in Python?
-In Python, the asterisk (*) is used as the multiplication operator. It multiplies two values together.
What does the division operator ('/') return when used in Python?
-The division operator ('/') returns the exact result of division, including decimal places. For example, 5 divided by 2 would return 2.5.
What is integer division in Python and how does it work?
-Integer division, represented by the '//' operator, performs division and rounds the result down to the nearest whole number. For instance, 5 divided by 2 would result in 2.
How does the modulo operator (%) work in Python?
-The modulo operator (%) calculates the remainder of a division. For example, 5 divided by 2 gives a remainder of 1, so 5 % 2 returns 1.
What is the difference between '==' and '!=' in comparison operators?
-'==' checks if two values are equal, while '!=' checks if two values are not equal. The result is a Boolean value (True or False) based on the comparison.
How does the 'and' logical operator work in Python?
-The 'and' logical operator requires both conditions in the expression to be true for the entire expression to evaluate as true. If one condition is false, the result will be false.
What is the function of the 'or' logical operator in Python?
-The 'or' logical operator allows an expression to evaluate as true if at least one of the conditions is true. It only evaluates to false if both conditions are false.
Outlines
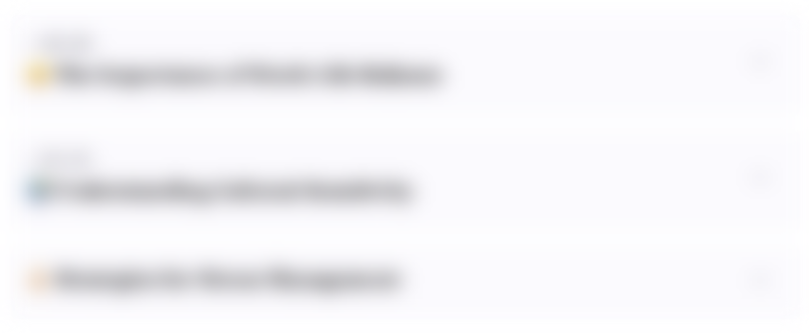
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
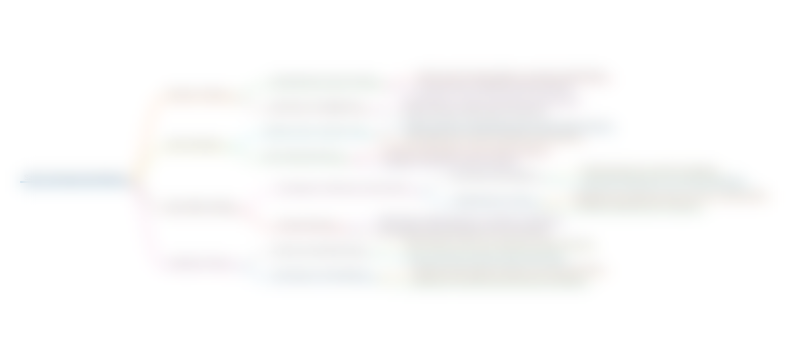
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
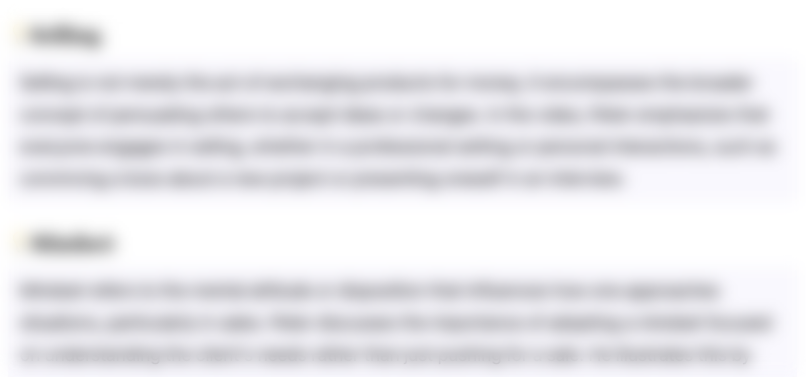
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
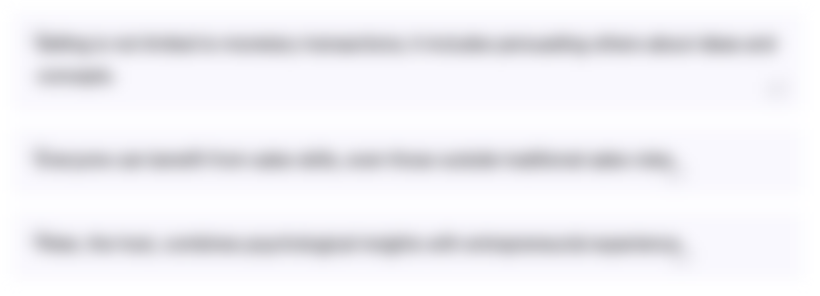
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
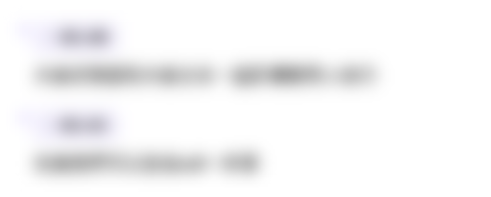
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
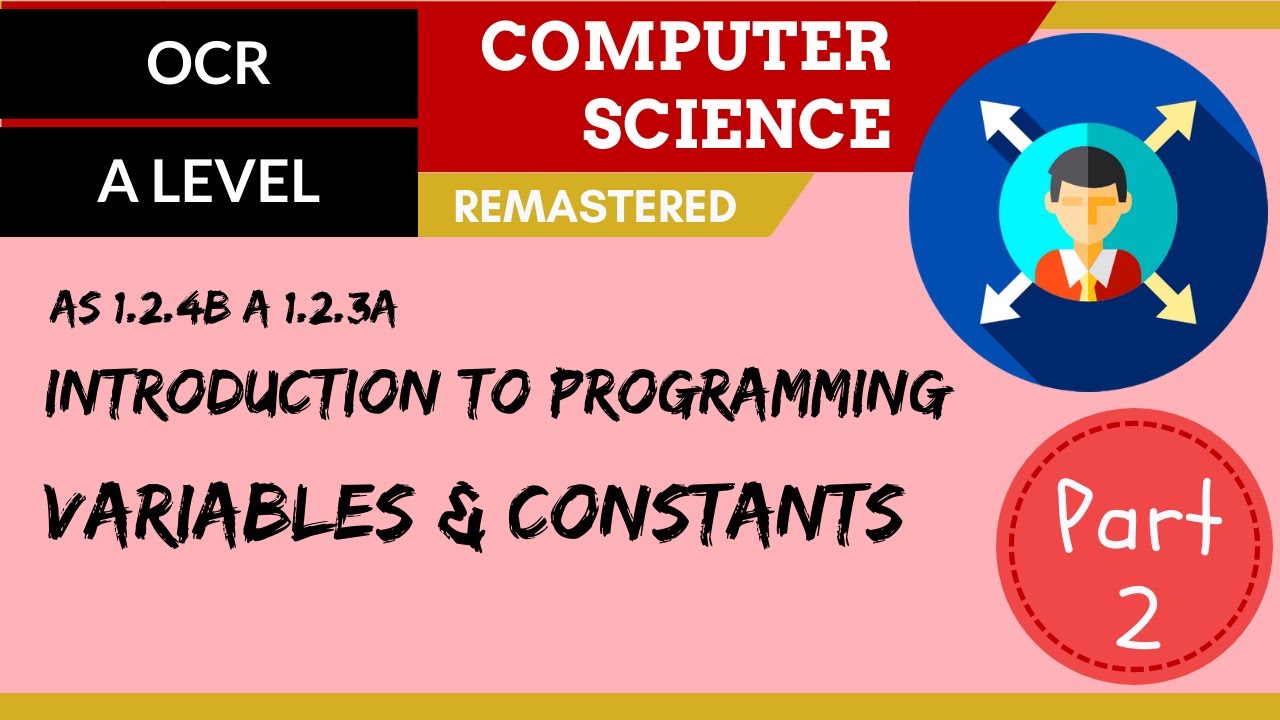
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
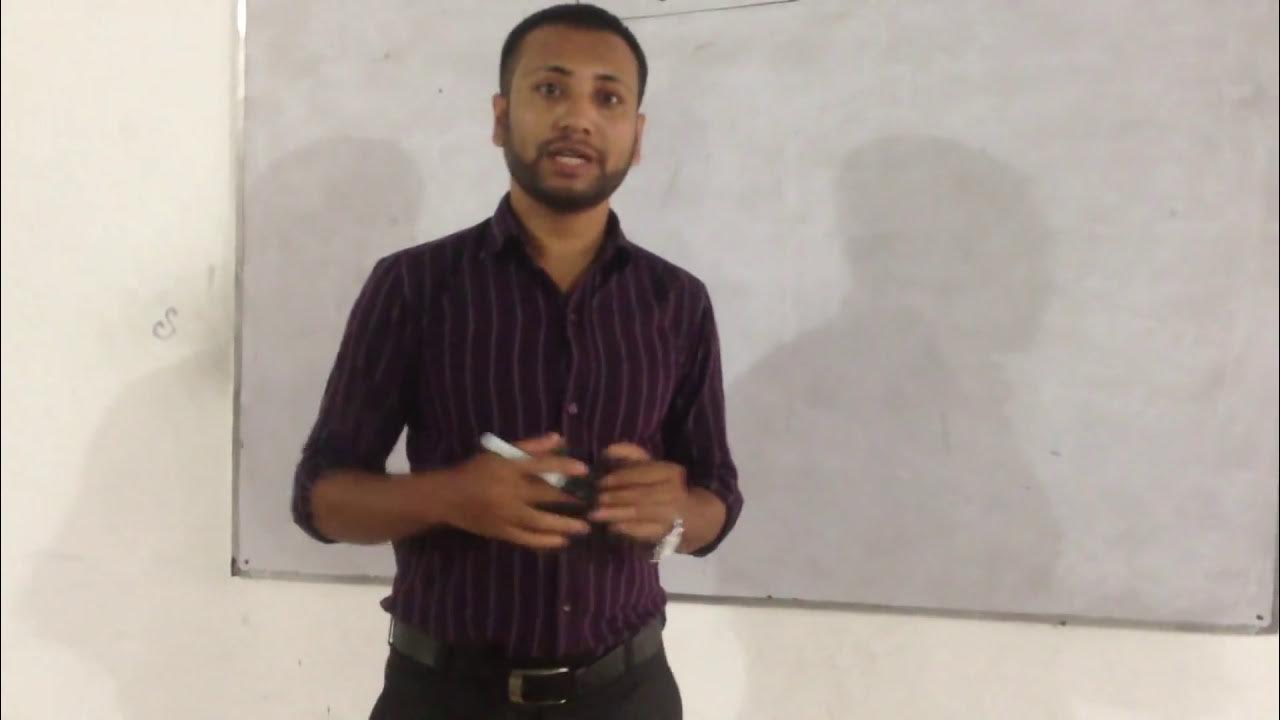
C programming Bangla Tutorial 5.2 : কিভাবে সি প্রোগ্রামিং পড়বেন / পড়াবেন ?
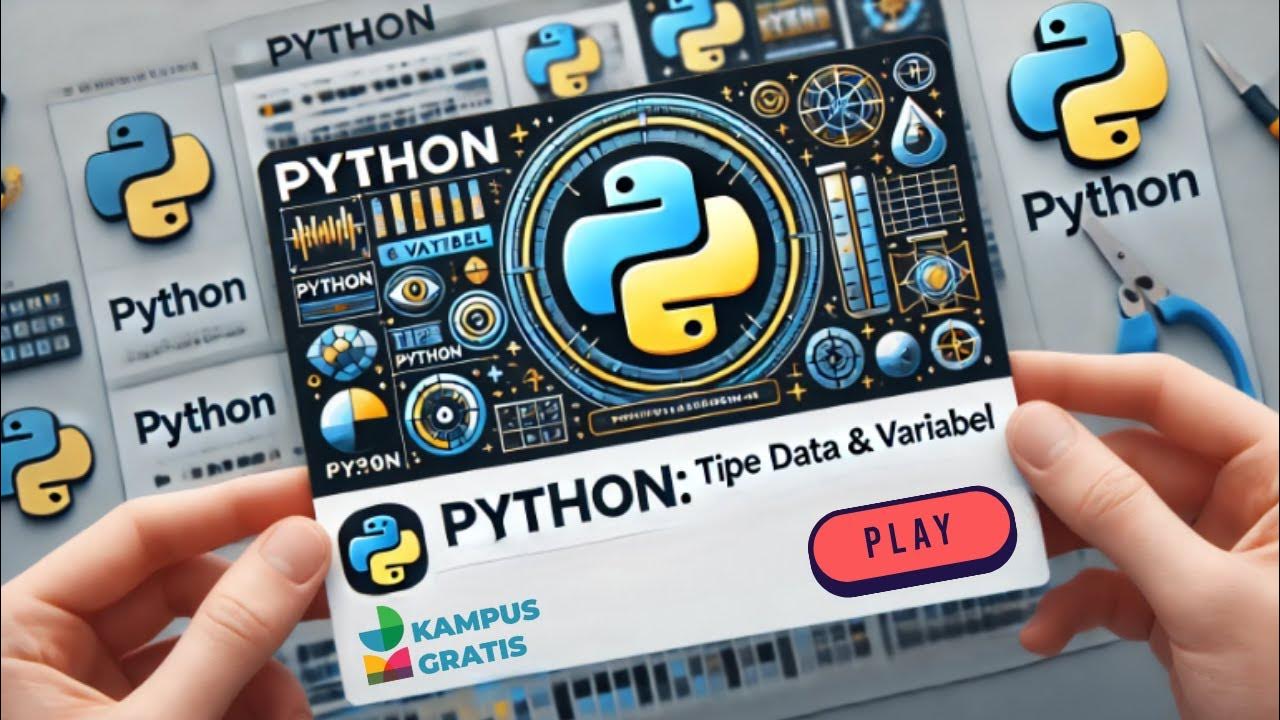
BAID MK1 P2
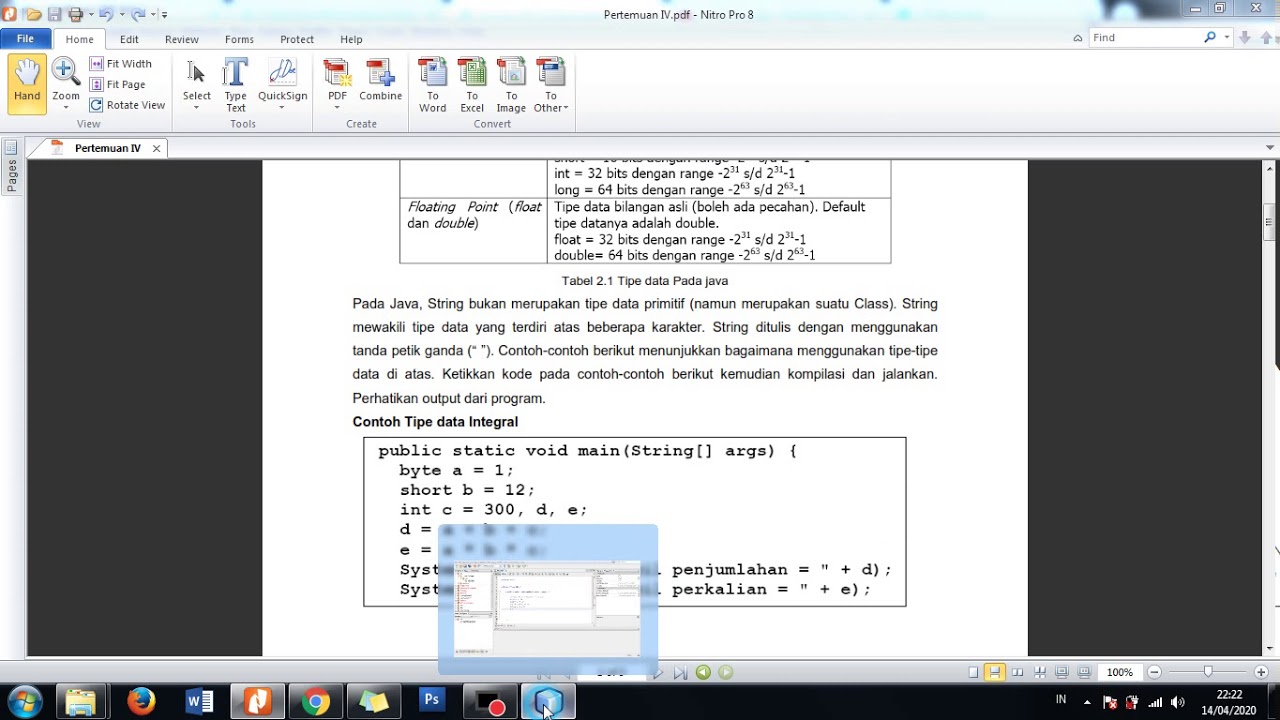
Praktek 2
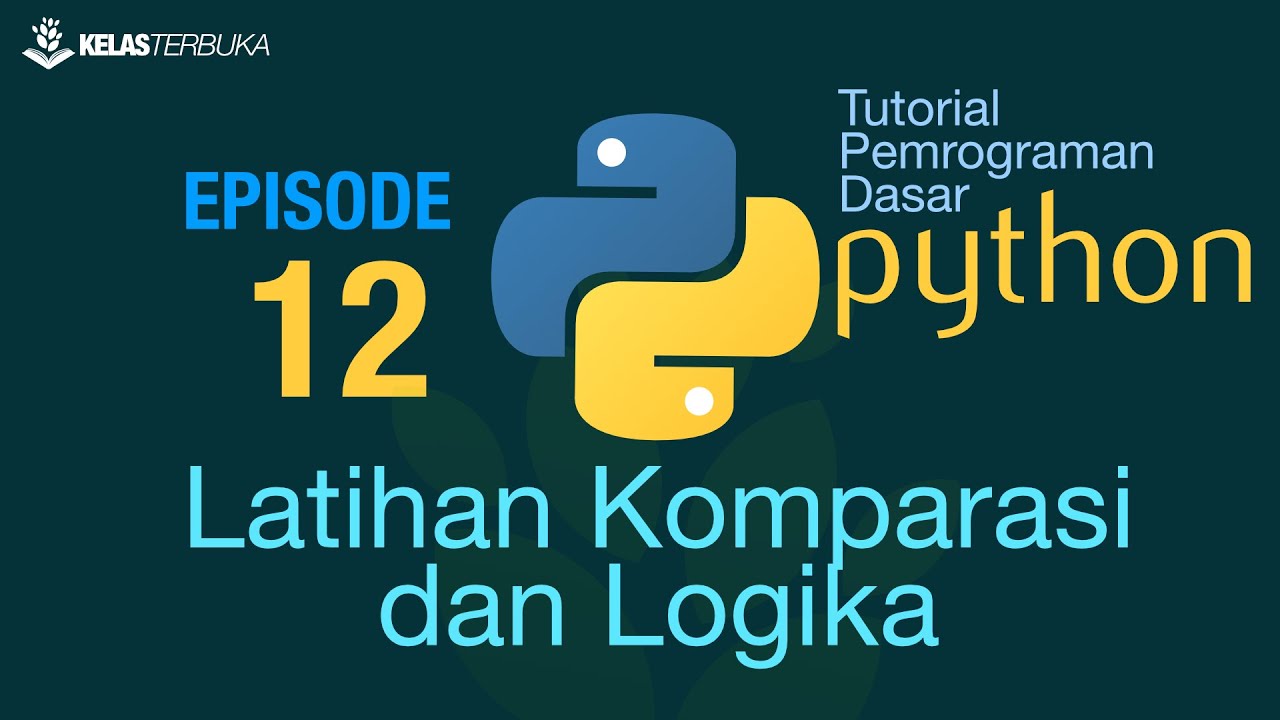
Belajar Python [Dasar] - 12 - Latihan Komparasi dan Logika
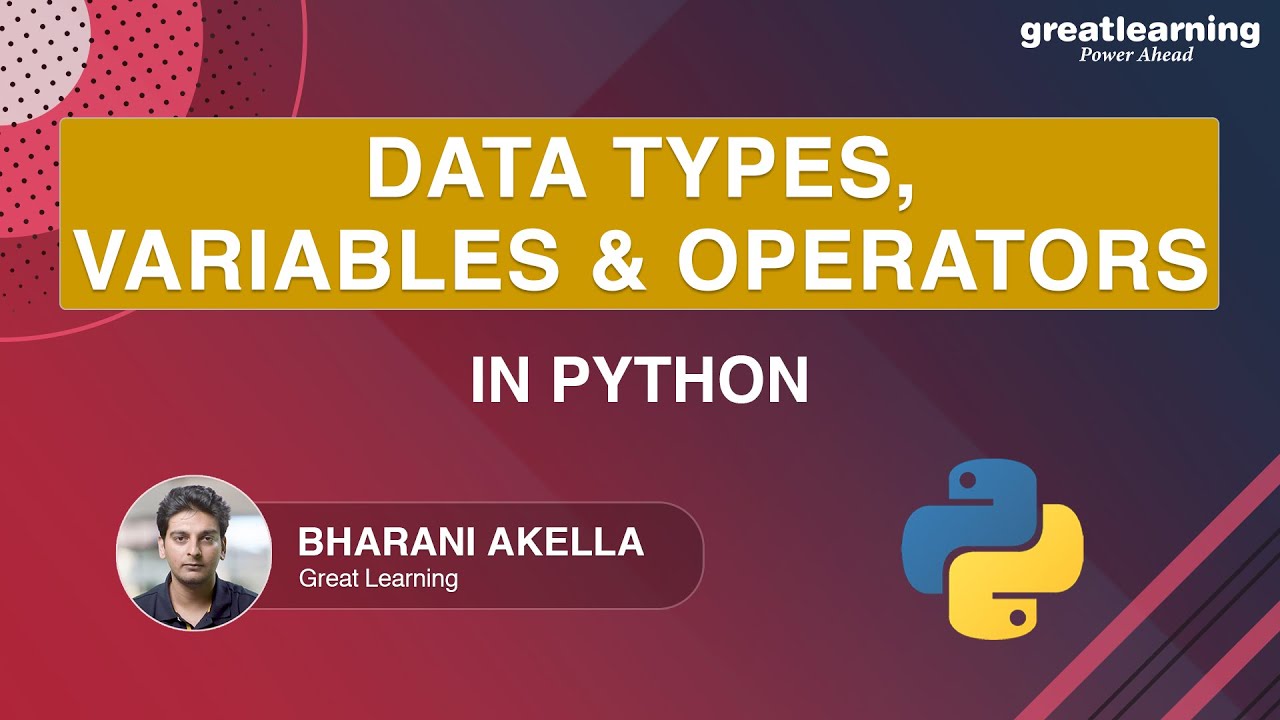
Data Types Variables And Operators In Python | Python Fundamentals | Great Learning
5.0 / 5 (0 votes)