Elements, Children and Re-renders - Advanced React course, Episode 2
Summary
TLDRIn this Advanced React course episode, we explore how to improve a lagging scrolling experience using composition techniques. The video walks through the process of implementing a smooth, performant floating block in a React app by optimizing state management and preventing unnecessary re-renders. By leveraging React's reconciliation and diffing algorithms, we learn how to pass heavy components as props without triggering re-renders, thus improving scroll performance. Key concepts covered include React elements, components, shallow comparisons, and the special 'children' prop. This lesson is essential for developers aiming to create fast, responsive UIs.
Takeaways
- 😀 Efficient floating blocks can improve user scrolling experience, making it smoother and more responsive.
- 😀 React's state changes on scroll can trigger unnecessary re-renders, which degrade performance.
- 😀 The second scrolling experience (slowly moving floating blocks) is the desired behavior, and it’s easier to implement than the first.
- 😀 To avoid unnecessary re-renders, state should be moved to smaller, more specific components within the render tree.
- 😀 When a component's state changes, it triggers re-renders in React, but we can optimize this by controlling state more effectively.
- 😀 React uses a shallow comparison to decide if a component should re-render when state changes, which can be leveraged for performance optimization.
- 😀 React Elements are objects that describe a component or DOM element, and React uses them to build the render tree.
- 😀 The diffing and reconciliation algorithm in React helps minimize unnecessary re-renders by comparing Elements and their 'type' properties.
- 😀 Props in React are just part of the Element object, and React will skip re-renders if props do not change.
- 😀 Using 'children' as a prop instead of 'content' has no functional difference, but the 'children' prop has special syntax in JSX that makes it easier to manage nested components.
Q & A
What problem does the floating block feature solve?
-The floating block solves the issue of inefficient re-renders during scrolling. It provides a smooth experience by avoiding unnecessary re-renders of heavy components as the user scrolls the page.
Why does the floating block experience lag initially?
-The initial lag occurs because every scroll event triggers a state change, which causes React to re-render all the components in the application, including the heavy ones inside the scrollable div.
What is the solution to the performance issue with the floating block?
-The solution is to move the state that tracks the scroll position into a smaller component lower down the render tree. This prevents unnecessary re-renders of the entire app and improves performance.
How does React's reconciliation algorithm work to improve performance?
-React's reconciliation algorithm compares the current and previous state of elements using shallow comparison. If no changes are detected, React skips re-rendering components, which improves performance by reducing unnecessary updates.
What role do props play in preventing unnecessary re-renders?
-Props, particularly the `children` prop, are passed down from parent to child components. When elements are passed as props and not recreated with every render, React can skip re-rendering those components if the props haven't changed, enhancing performance.
What is the difference between a React Element and a React Component?
-A React Element is an object that describes a component or a DOM element. A React Component, on the other hand, is a function that returns a React Element. Components can be used to build a tree of elements that React renders to the DOM.
Why does React re-render components when state changes?
-React re-renders components when state changes to reflect the new data in the UI. This ensures that the displayed content matches the current state of the application.
What is the significance of the `children` prop in React?
-The `children` prop is a special prop in React that allows you to pass nested elements to a component. It is often used to render dynamic content or components inside other components, and it can be optimized to prevent unnecessary re-renders.
How can you optimize rendering for heavy components in React?
-You can optimize rendering by passing heavy components as props, especially through the `children` prop. This way, React can skip re-rendering these components if the state or props don't change, resulting in better performance.
What happens when React performs a shallow comparison of elements?
-During shallow comparison, React compares the references of objects (elements) rather than their values. If the references are the same, React skips the re-render. If the references are different, React will proceed with re-rendering the component.
Outlines
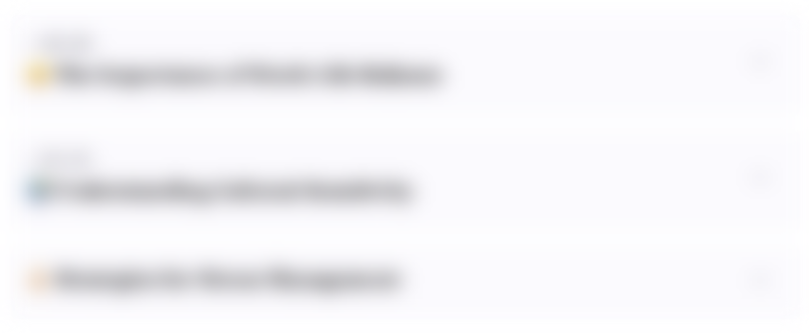
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
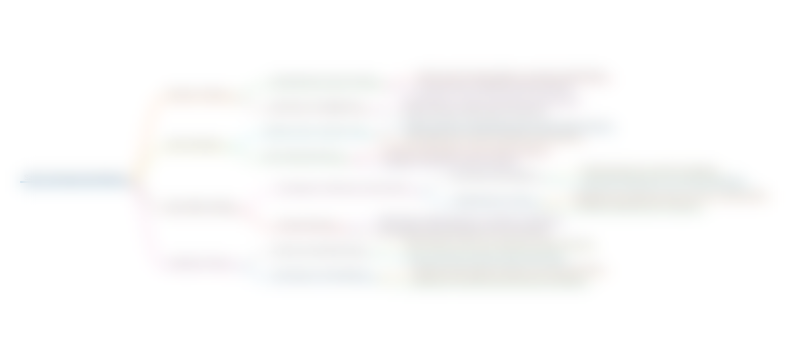
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
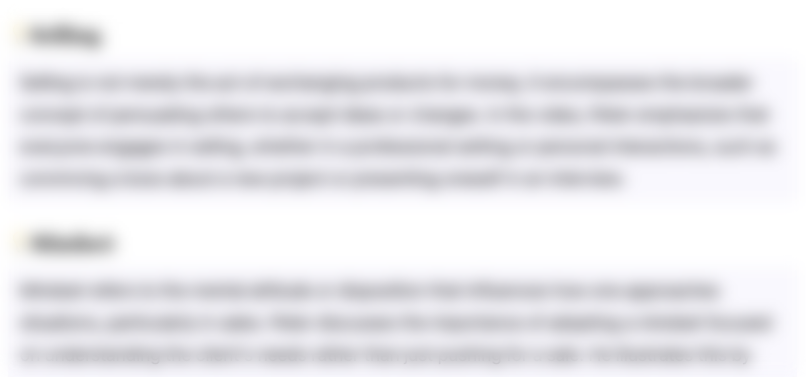
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
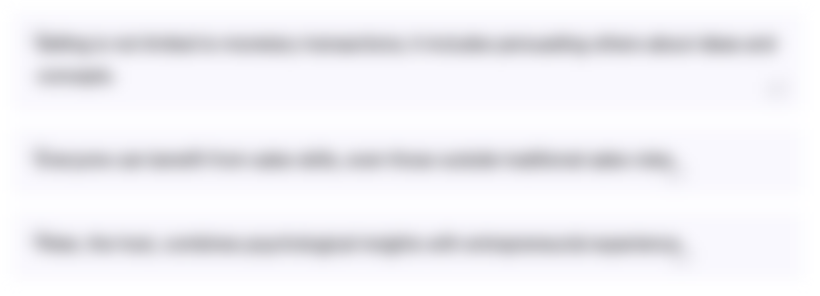
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
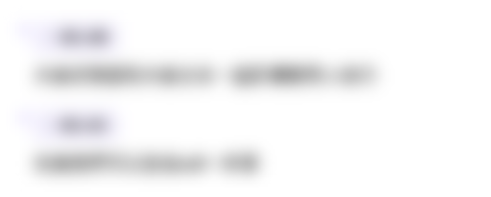
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Learn useRef in 11 Minutes

Understanding memo | Lecture 242 | React.JS 🔥
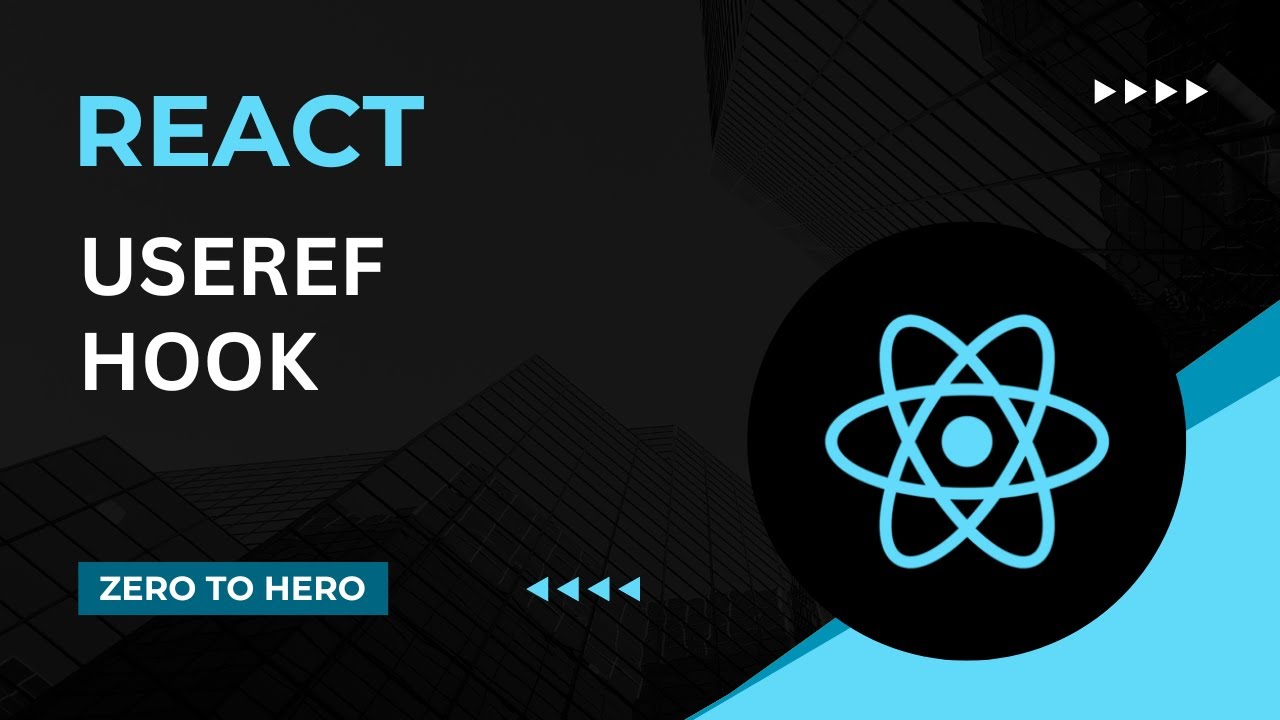
useRef Hook | Mastering React: An In-Depth Zero to Hero Video Series

Performance Optimization and Wasted Renders | Lecture 239 | React.JS 🔥
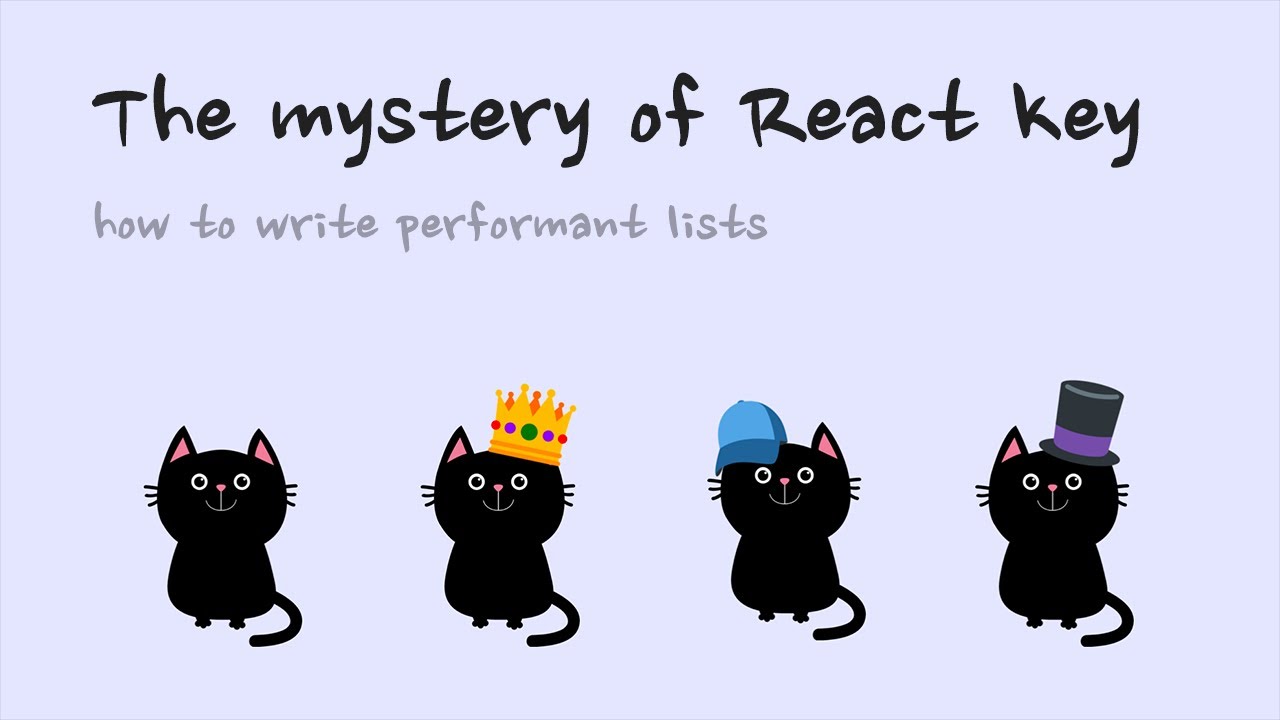
The mystery of React key: how to write performant lists
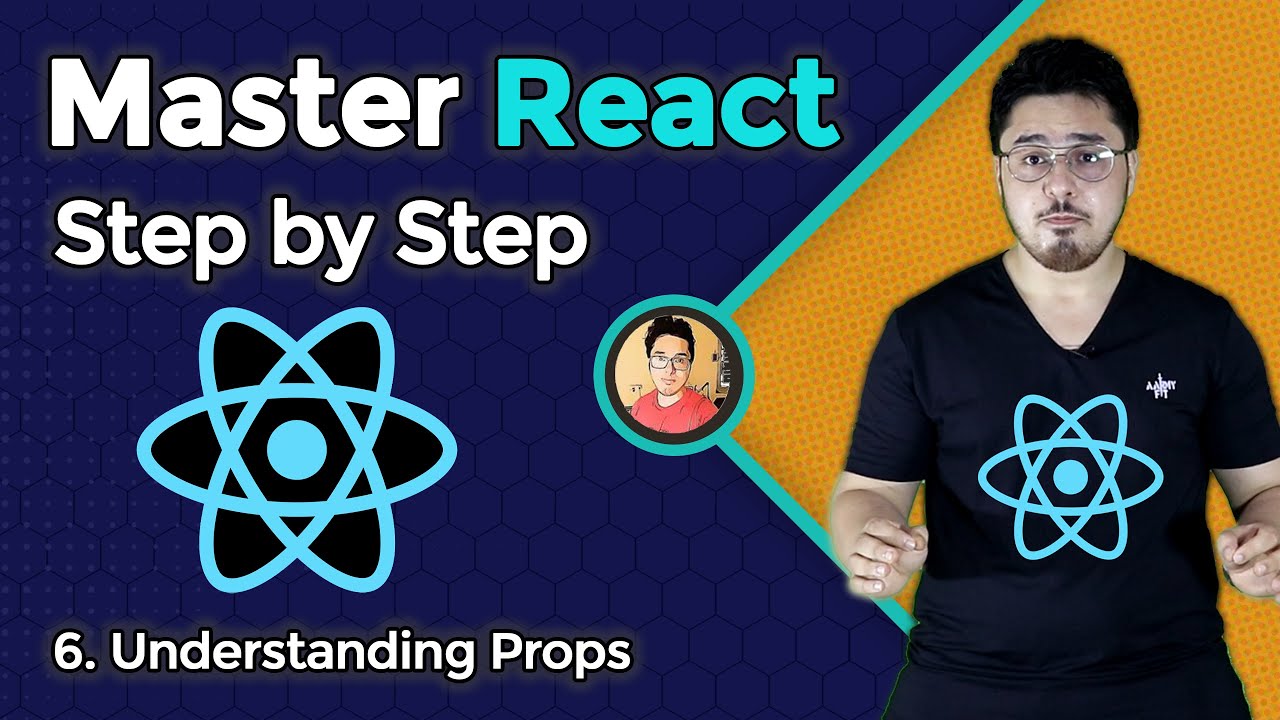
Understanding Props and PropTypes in React | Complete React Course in Hindi #6
5.0 / 5 (0 votes)