Python Intermediate Tutorial #6 - Queues
Summary
TLDRIn this Python tutorial for intermediate learners, the focus is on understanding and using queues in multi-threaded programming. The video explains how queues help manage data flow when multiple threads access shared data. It covers three main types of queues: FIFO (First In, First Out), LIFO (Last In, First Out), and priority queues, demonstrating their use with Python's `queue` module. Practical examples show how threads can safely process data from a queue without conflicts, ensuring an organized, structured approach to handling multiple tasks simultaneously. The tutorial is perfect for those looking to explore threading and data synchronization in Python.
Takeaways
- 😀 Queues in Python are essential for managing the order of data, especially in multi-threaded programs.
- 😀 The primary reason to use queues is to prevent race conditions when multiple threads need to access shared data.
- 😀 A queue operates in a structured manner where the first element inserted is the first one to be processed (FIFO).
- 😀 Python's `queue.Queue()` class is used for FIFO behavior, ensuring thread-safe element retrieval in order.
- 😀 LIFO (Last In, First Out) queues allow the last element inserted to be the first one processed, using `queue.LifoQueue()`.
- 😀 A priority queue in Python allows you to assign a priority to each element, and elements with the lowest priority number are processed first.
- 😀 Python's `queue.PriorityQueue()` class supports priority queues, which are useful in applications like task scheduling.
- 😀 In multi-threaded applications, using a queue ensures that no element is processed more than once and that elements are accessed sequentially.
- 😀 The `put()` method adds elements to the queue, while `get()` retrieves the next available element, ensuring the correct order of processing.
- 😀 The tutorial also highlights practical applications, such as using queues for building a threaded port scanner to handle multiple connections simultaneously.
- 😀 The video encourages viewers to explore further topics like socket programming and network communication, building on the knowledge of multithreading and queues.
Q & A
What is the main advantage of using queues in Python for threading?
-Queues in Python provide a structured way to manage data shared between multiple threads, ensuring that elements are processed in a controlled and predictable order without conflicts like skipping or duplicating data.
What is the difference between a FIFO queue and a LIFO queue?
-A FIFO (First In, First Out) queue processes elements in the order they were added, whereas a LIFO (Last In, First Out) queue processes the most recently added elements first.
How does a priority queue differ from a FIFO and LIFO queue?
-A priority queue allows elements to be assigned a priority, with the queue always processing elements with the highest priority first, based on a numerical value. In contrast, FIFO and LIFO queues don’t consider priorities when processing elements.
Why is it important to use queues when multiple threads are involved?
-When multiple threads are accessing shared data, queues provide a safe and structured method of data handling. They prevent issues like data duplication or skipping by ensuring that threads process elements in a predictable order.
Can we manually set the priority of elements in a Python priority queue?
-Yes, in a Python priority queue, elements can be assigned a priority by using a tuple, where the first element of the tuple is the priority value. Lower numbers represent higher priorities.
What does the 'q.put()' method do in a queue?
-'q.put()' is used to add an element to the queue. The element will be added at the appropriate position based on the type of queue (FIFO, LIFO, or priority).
What happens when we call 'q.get()' on a queue?
-Calling 'q.get()' removes and returns the next element from the queue, following the order defined by the type of queue being used (FIFO, LIFO, or priority).
What type of queue would you use if you wanted the most recent task to be processed first?
-You would use a LIFO (Last In, First Out) queue, which processes the most recent element added to the queue before older ones.
How do you ensure that each thread processes a unique task from the queue?
-By using a queue, each thread retrieves the next available task using 'q.get()', ensuring that no task is processed more than once, and threads cannot skip tasks. Once a task is processed, it is removed from the queue.
What is a potential problem that queues help prevent in multithreaded applications?
-Queues help prevent the problem of race conditions, where multiple threads might attempt to access or modify the same data simultaneously, leading to errors such as skipping or duplicating tasks.
Outlines
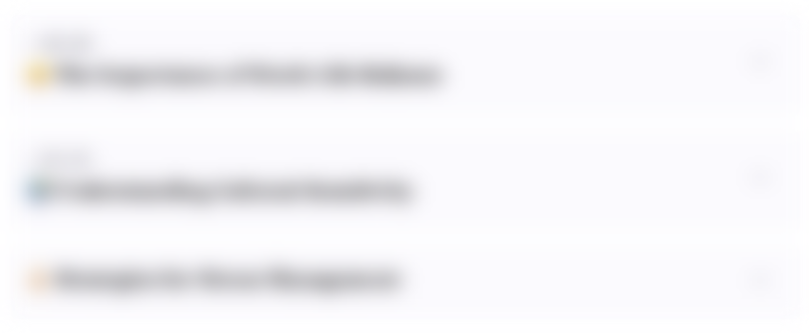
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
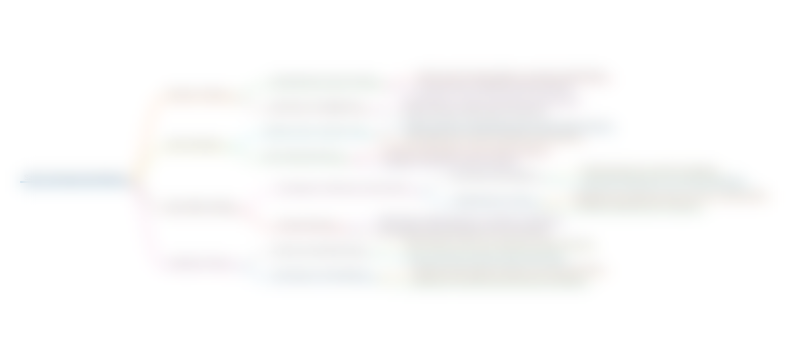
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
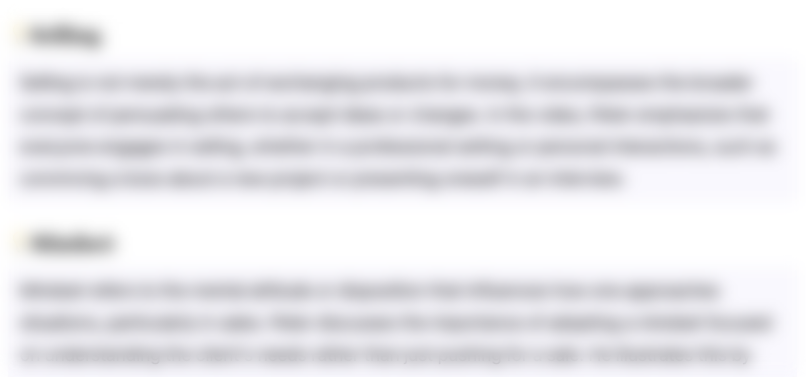
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
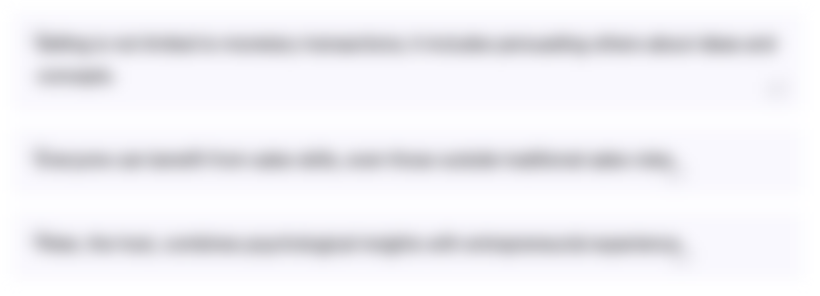
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
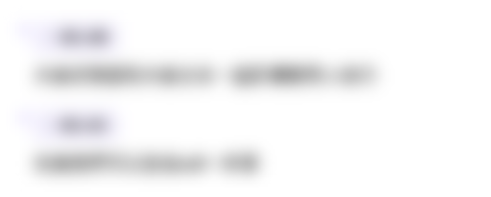
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
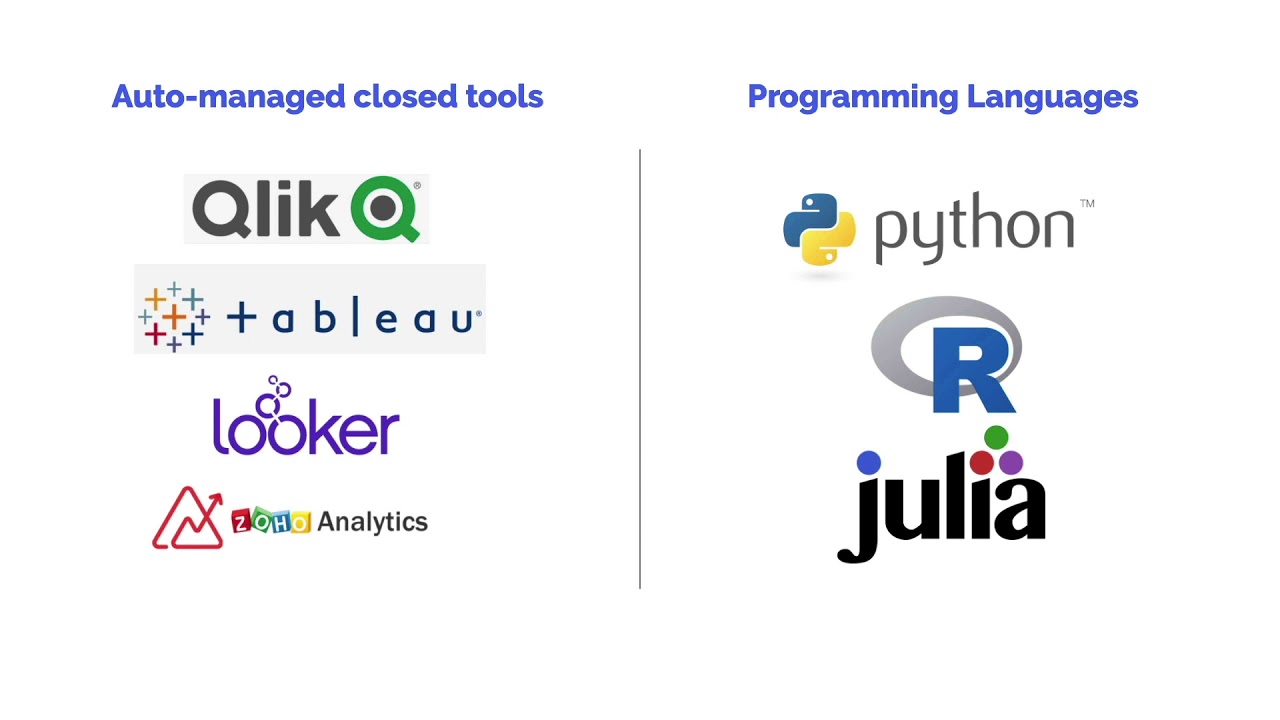
Introduction - Data Analysis with Python
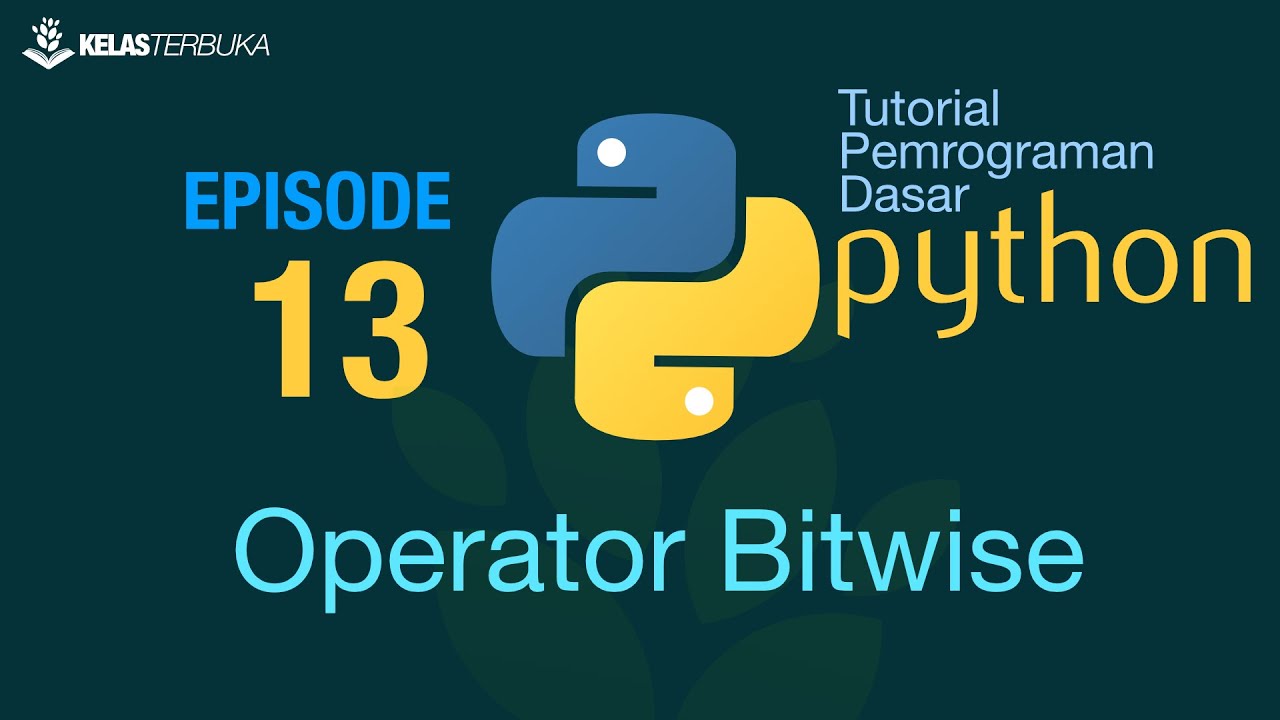
Belajar Python [Dasar] - 13 - Operator Bitwise
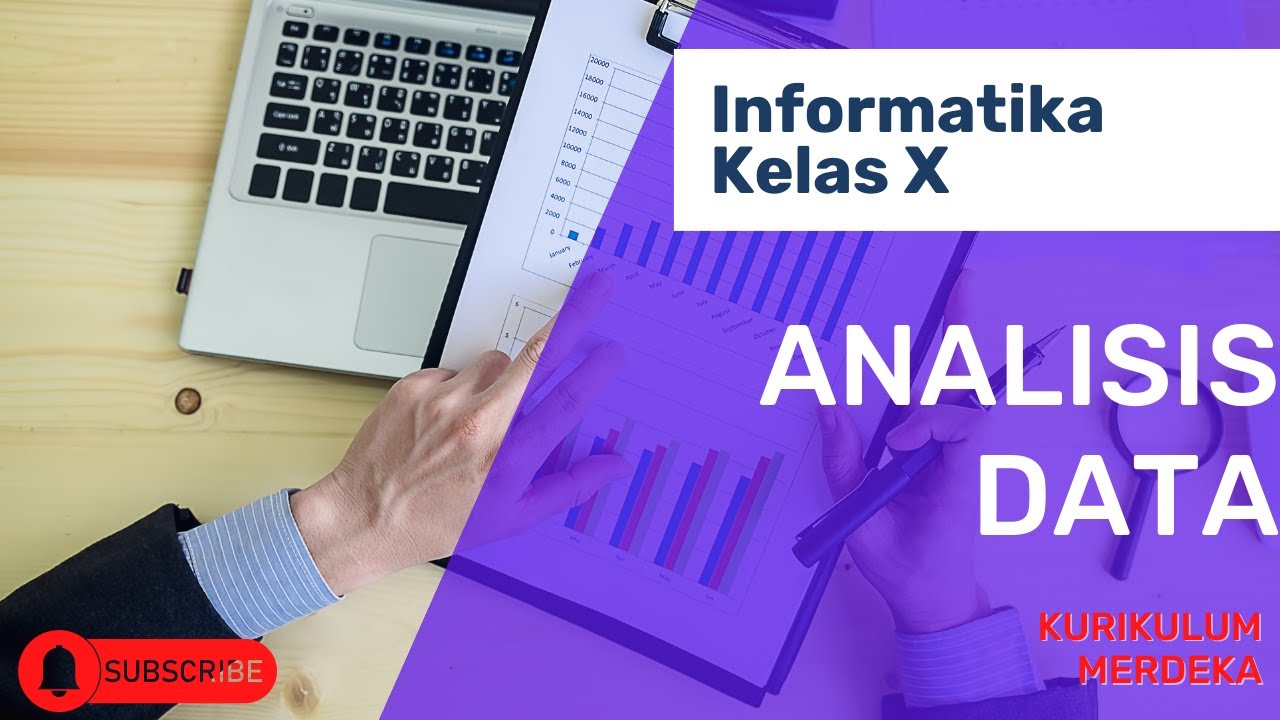
Analisis Data | Informatika Kelas X
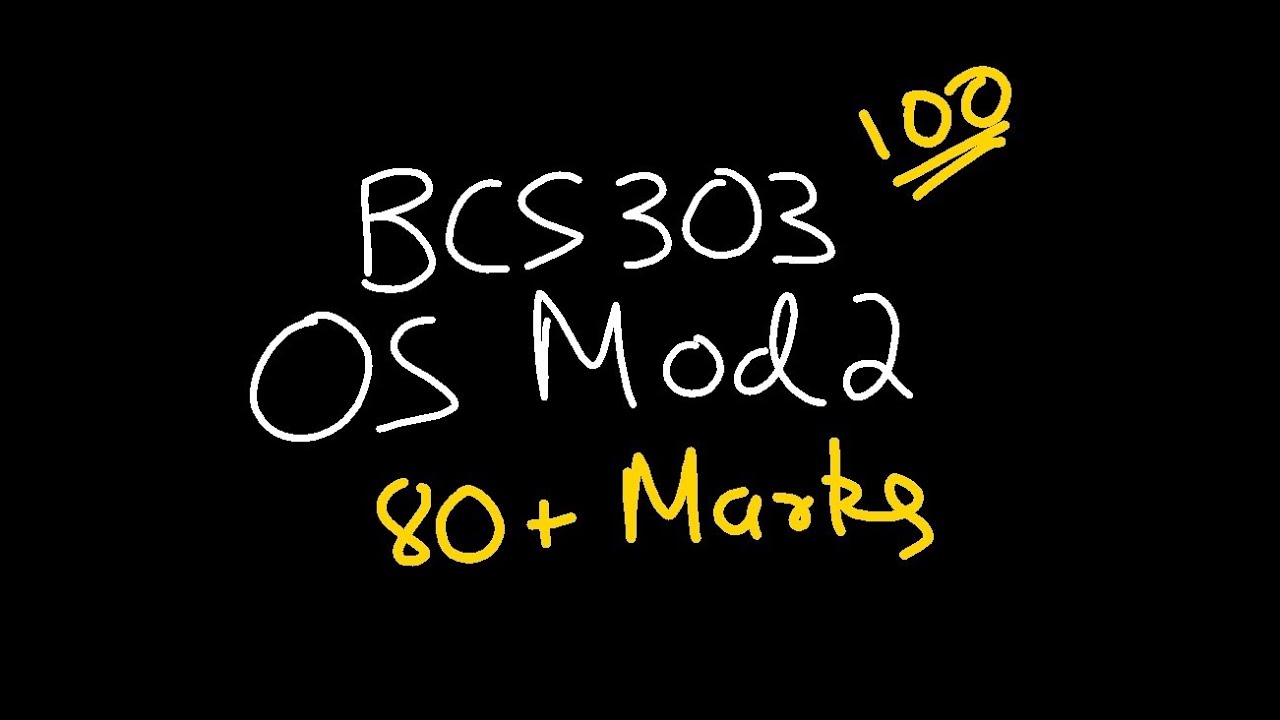
OS MODULE 2 BCS303 Operating System | 22 Scheme VTU 3rd SEM CSE
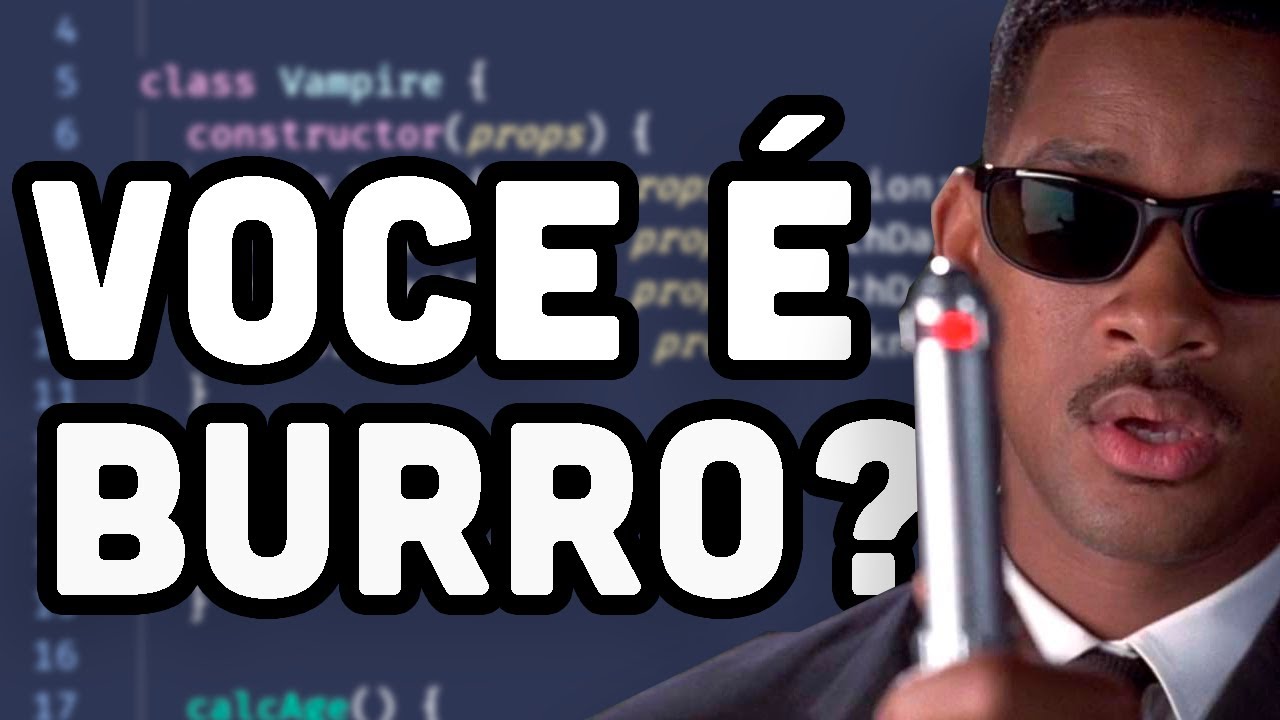
Se nao aprender PROGRAMAÇÃO com esse video. - ̗̀ DESISTE ̖́-
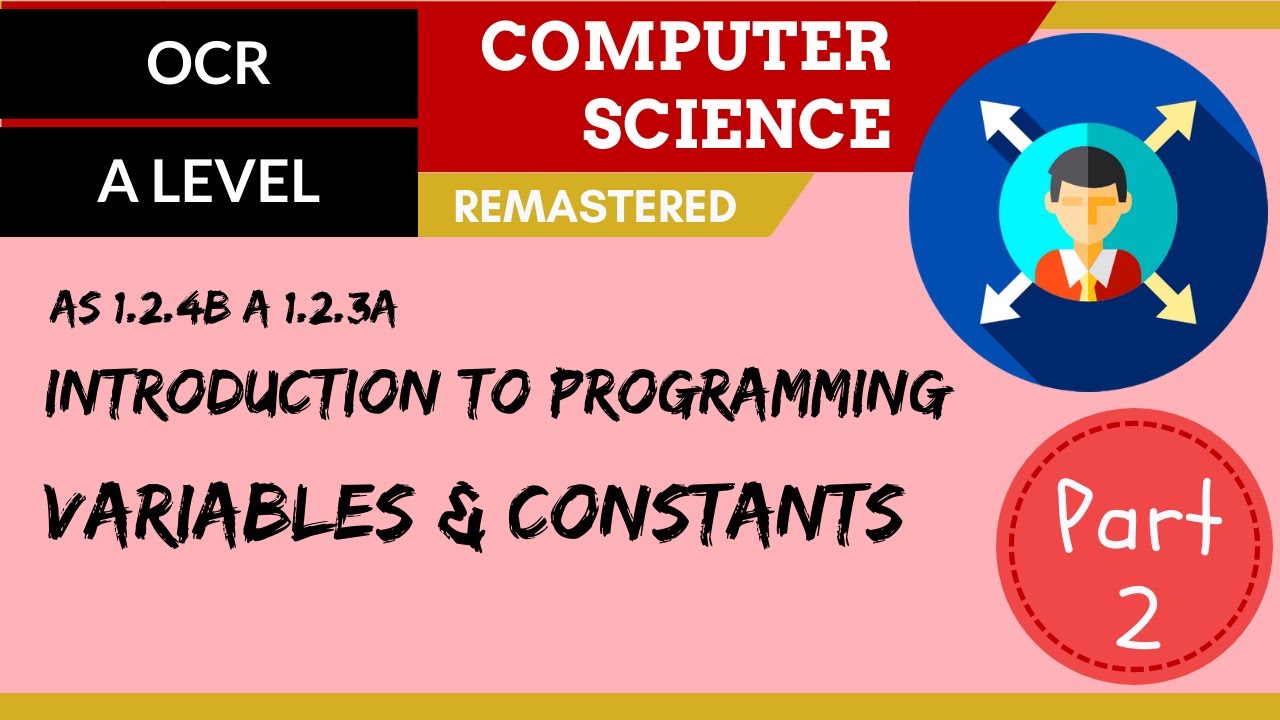
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
5.0 / 5 (0 votes)