C++ programming to calculate area and perimeter, with functions.
Summary
TLDRThis tutorial demonstrates how to structure a program to calculate the area and perimeter of a rectangle using modular functions. The program begins by taking user inputs for length and width, then calculates the area and perimeter in a separate function. Results are displayed, and a loop is included to allow users to perform multiple calculations. The key takeaway is the modularization of the program, which enhances clarity, reusability, and scalability, making it easier to debug and extend. The video emphasizes the importance of organizing code into distinct sections to improve functionality and maintainability.
Takeaways
- 😀 The program calculates the area and perimeter of a rectangle based on user input for length and width.
- 😀 The program is modularized by separating it into functions for input, processing, and display to improve readability and reuse.
- 😀 Functions use references or pointers to share memory locations, meaning changes in one variable affect others (e.g., length and width).
- 😀 A dedicated `get_input` function is used to obtain the length and width from the user, with shared memory locations for efficient management.
- 😀 The `calculate_area_perimeter` function computes the area and perimeter, which are passed by reference as output values.
- 😀 The `display` function is used to print the area and perimeter, separating concerns and making the code more modular.
- 😀 The program includes a `do-while` loop, allowing users to repeat the calculation process without restarting the program.
- 😀 The `do-while` loop asks the user if they wish to repeat the calculation after each run, enabling repeated execution.
- 😀 The program is structured in such a way that reusable functions can be utilized in other programs or parts of the same project.
- 😀 The professor emphasizes using quick actions in the IDE for refactoring and organizing code for better clarity and ease of understanding.
Q & A
What is the main objective of the program discussed in the transcript?
-The main objective of the program is to calculate the area and perimeter of a rectangle based on user input for the length and width.
How is the program structured?
-The program is structured into three parts: Input (to get the length and width), Processing (to calculate the area and perimeter), and Output (to display the results).
What role do functions play in this program?
-Functions are used to modularize the program by separating the input, calculation, and output into distinct parts. This improves code organization and reusability.
Why is memory management important in this program, and how is it handled?
-Memory management is important to ensure that the program correctly handles the input values and modifies the output values. The program uses shared memory by passing values by reference, allowing the area and perimeter to be calculated and stored directly in the provided variables.
What is the purpose of using 'call by reference' in this program?
-'Call by reference' is used to pass the area and perimeter variables to the function so that the function can directly modify their values in memory, allowing the calculated results to be returned to the main function.
How does the do-while loop improve the program?
-The do-while loop allows the user to repeat the process of inputting values and calculating the area and perimeter without restarting the program. It prompts the user to continue or exit based on their input.
What would happen if 'call by value' was used instead of 'call by reference' for area and perimeter?
-If 'call by value' were used, the area and perimeter would be copied to the function, and any changes made within the function would not affect the original variables in the main function. This would require additional logic to return values from the function.
How does modularizing the program benefit the user and the programmer?
-Modularizing the program makes it easier to maintain, understand, and update. Functions can be reused in different parts of the program, and the code is less complex, which simplifies debugging and future enhancements.
What is the significance of structuring the program with input, process, and output sections?
-Structuring the program into distinct input, process, and output sections enhances readability and organization. It ensures that each part of the program has a clear and specific role, which improves code clarity and maintainability.
What improvements could be made to handle invalid inputs from the user?
-The program could include error handling to check for invalid input, such as ensuring that the user enters numeric values for length and width. This could involve using input validation techniques like checking the input type or using try-catch blocks.
Outlines
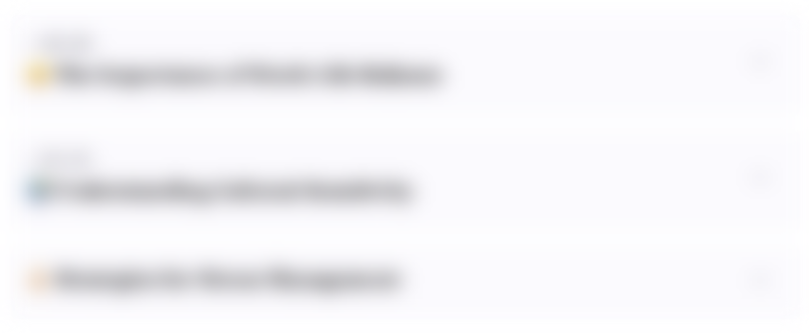
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
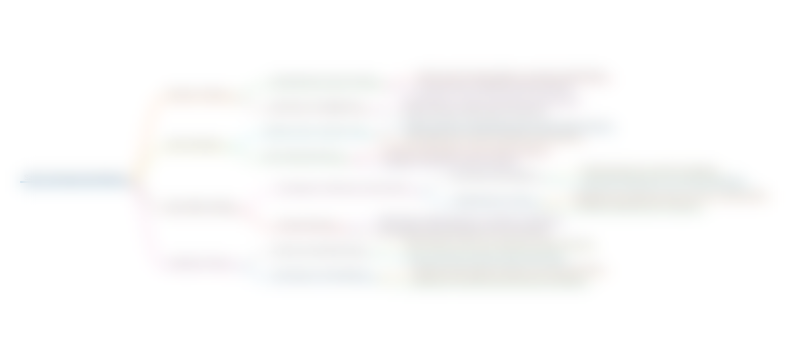
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
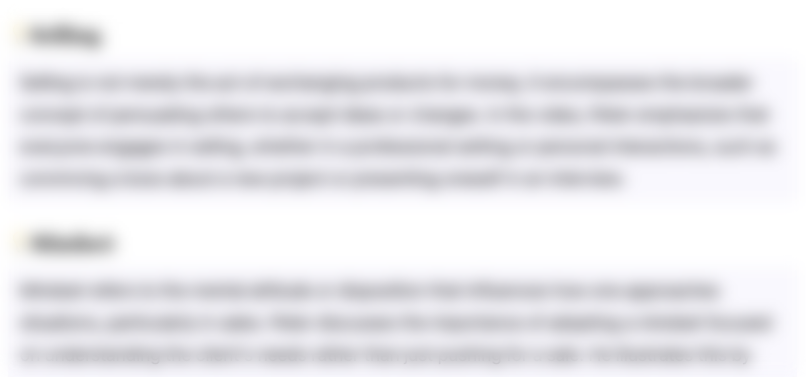
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
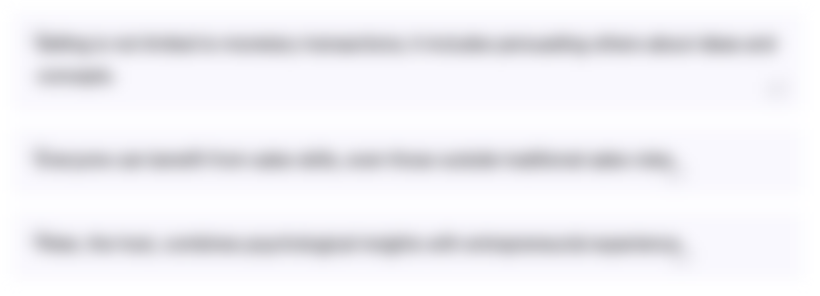
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
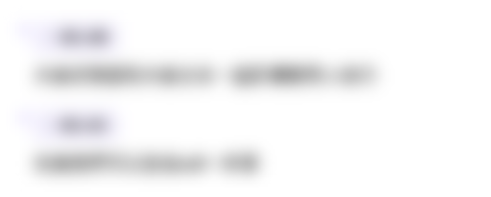
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
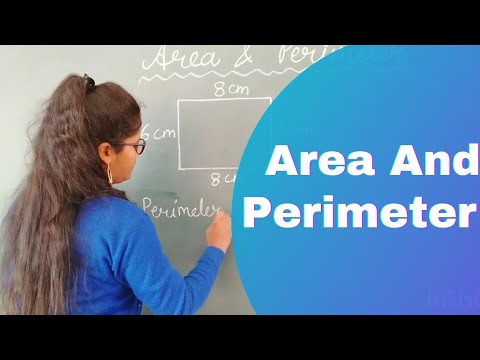
How to find out Area and Perimeter of a shape #maths
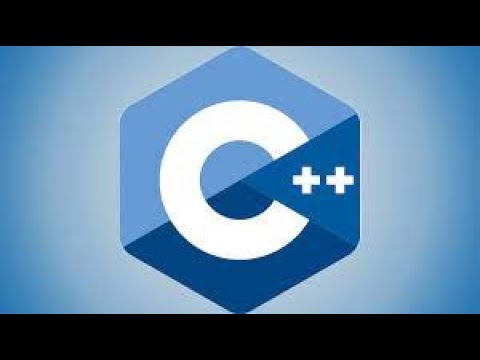
C++ programming, Void function, get input by reference, call function by value and by reference
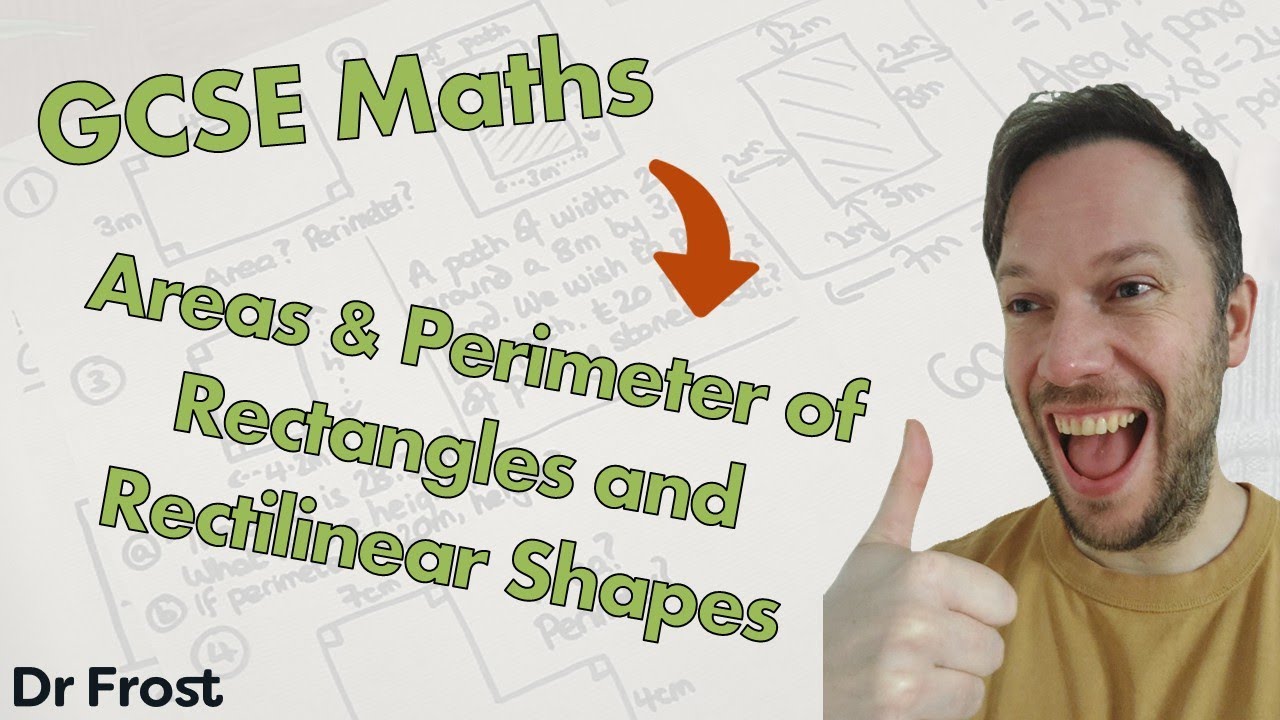
Areas & Perimeter of Rectangles and Rectilinear Shapes

ÁREA E PERÍMETRO | RESOLUÇÃO DE PROBLEMAS | EXERCÍCIOS
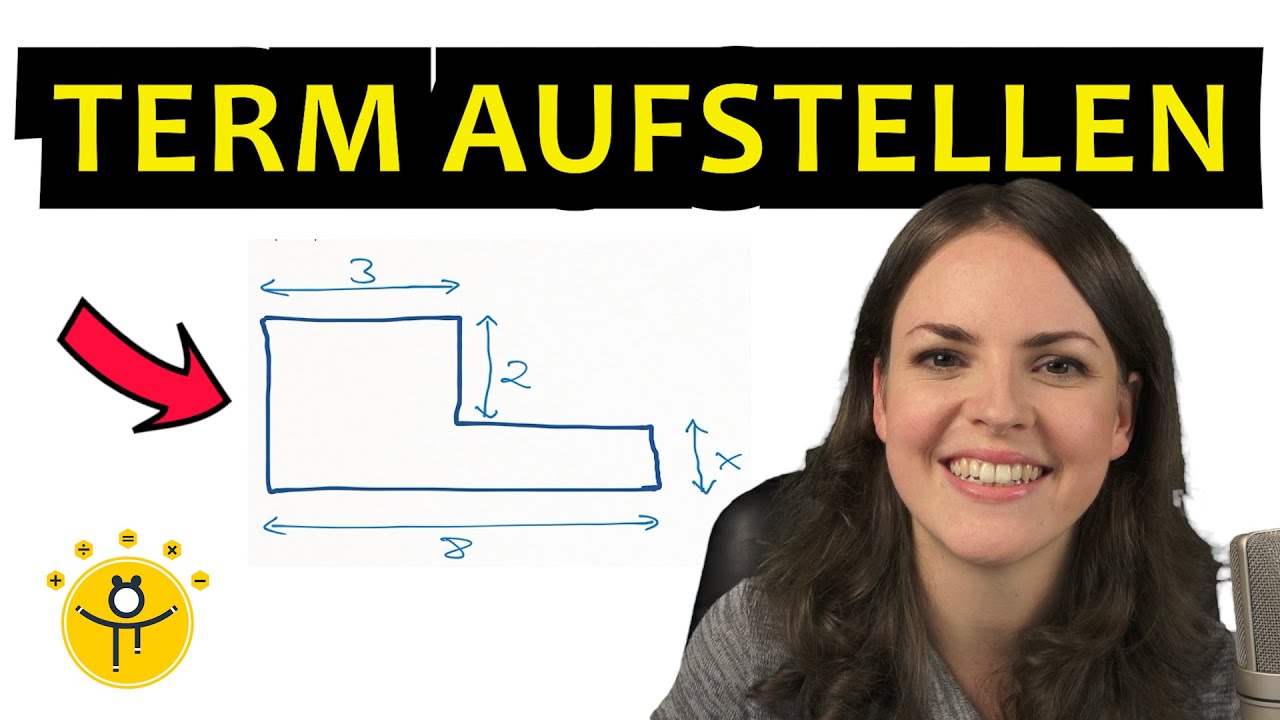
TERM AUFSTELLEN – für Umfang und Flächeninhalt, mit Variablen, Rechteck Figur
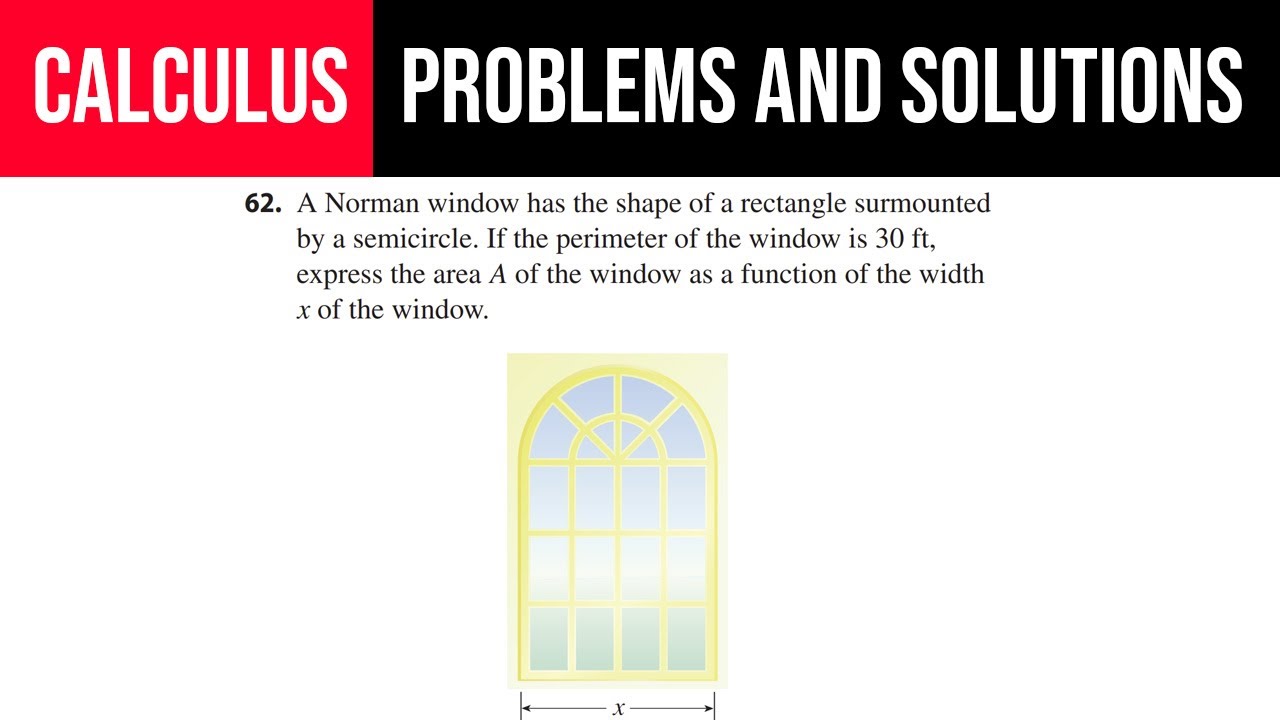
62. A Norman window has the shape of a rectangle surmounted by a semicircle. If the perimeter of...
5.0 / 5 (0 votes)