Intro to Arrays
Summary
TLDRThis tutorial provides a clear introduction to arrays in Java, focusing on their definition, initialization, and usage. It explains how arrays are indexed structures that store multiple values of the same type, and how they can be initialized either explicitly with predefined values or by defining their size. The script covers array element access and modification, the use of loops to iterate through arrays, and the importance of handling array boundaries correctly to avoid exceptions. This foundational lesson is ideal for beginners learning to work with arrays in Java.
Takeaways
- 😀 Arrays are indexed structures that store multiple values of the same type, with indices starting at 0.
- 😀 An array can store primitive data types (e.g., int, double) or objects (e.g., String), but all elements must be of the same type.
- 😀 Arrays can be initialized in two main ways: with predefined values or by specifying the size when the values are unknown.
- 😀 When initializing an array with known values, you specify the type, use square brackets, and separate the values with commas in curly braces.
- 😀 When initializing an array without predefined values, you define the size of the array and fill it with default values (e.g., 0 for ints, null for objects).
- 😀 In Java, once the size of an array is set, it cannot be changed. This differs from ArrayLists, which can grow or shrink dynamically.
- 😀 To access individual elements in an array, use their index (e.g., array[0] for the first element).
- 😀 Arrays have a fixed length, and you can access this length using the `.length` attribute (e.g., array.length).
- 😀 To print the content of an array, you can use `Arrays.toString()` to convert the array into a printable string.
- 😀 When using loops to iterate through arrays, ensure the loop condition checks that the index is less than `array.length` to avoid `IndexOutOfBoundsException`.
- 😀 It's essential to remember that array indices in Java start at 0, and the last valid index is `array.length - 1`.
Q & A
What is an array in Java?
-An array is an indexed data structure that holds multiple values of the same type. These values can either be primitives (e.g., integers or doubles) or objects (e.g., strings), and they are accessed using their index, which starts from 0.
What does 'index' mean in the context of an array?
-An index refers to the position of an element within an array, starting at 0. Each element in an array has a unique index, and you can access elements by specifying their index.
Can arrays hold different types of data?
-No, arrays in Java can only hold elements of the same type. You cannot mix primitive types or different object types within a single array.
How do you initialize an array when you know its values?
-You can initialize an array with known values by specifying the type, using square brackets, and enclosing the values within curly braces. For example: `int[] grades = {91, 78, 87, 88};`
How do you initialize an array when the values are unknown but the size is known?
-If you know the size but not the values, you can create an empty array by specifying the type and size. For example: `String[] names = new String[4];` This creates an array of size 4, with default values (null for object types).
What happens when you access an array element using an invalid index?
-Accessing an array element with an invalid index (either negative or greater than or equal to the array length) results in an `ArrayIndexOutOfBoundsException`.
What is the role of the `length` property in arrays?
-The `length` property provides the number of elements in an array. It is used to control loops and prevent out-of-bounds errors. For example: `array.length` gives the number of elements in the array.
What is the difference between `length` in arrays and `length()` in strings?
-The `length` property in arrays is a variable that returns the size of the array, while `length()` in strings is a method that returns the number of characters in a string. Arrays use `length` without parentheses, but strings use `length()` with parentheses.
How do you print the contents of an array in Java?
-To print the contents of an array, you can use `Arrays.toString()` to convert the array to a printable string format. For example: `System.out.println(Arrays.toString(array));`
Why is it important to initialize array elements immediately after declaring the array?
-Initializing array elements immediately helps avoid issues related to default values that may be confusing or unreliable. It ensures that the array contains valid data when accessed, preventing errors that arise from uninitialized values.
Outlines
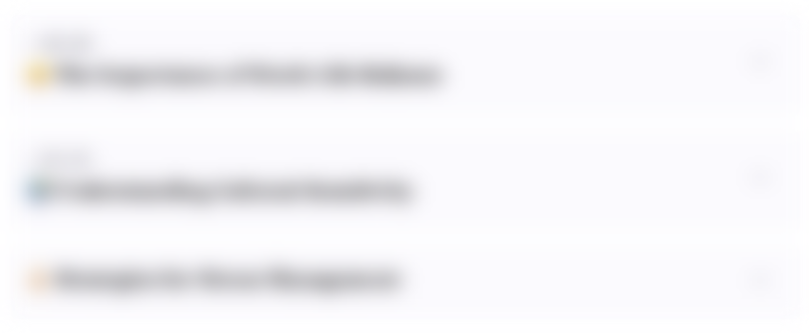
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
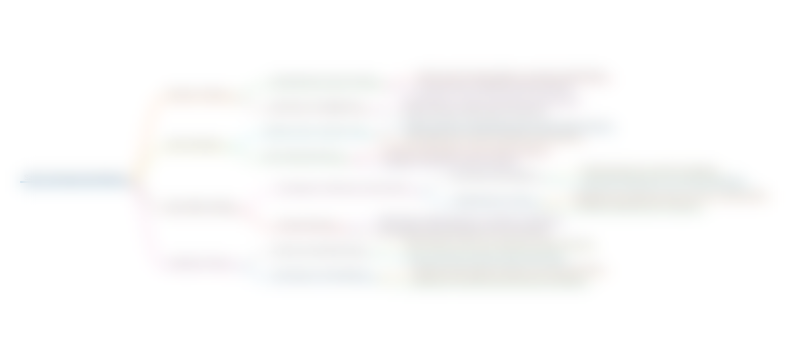
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
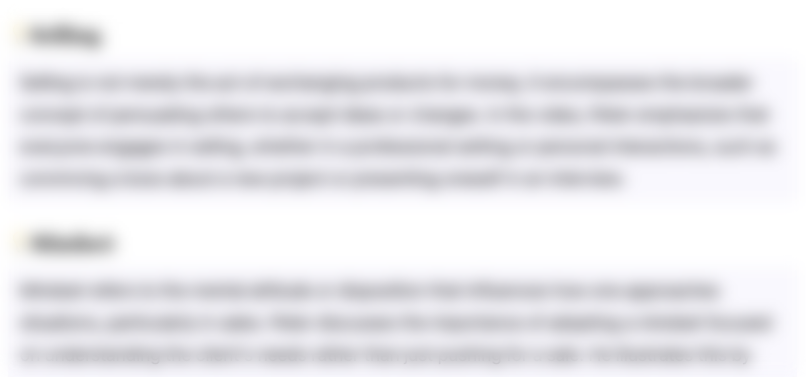
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
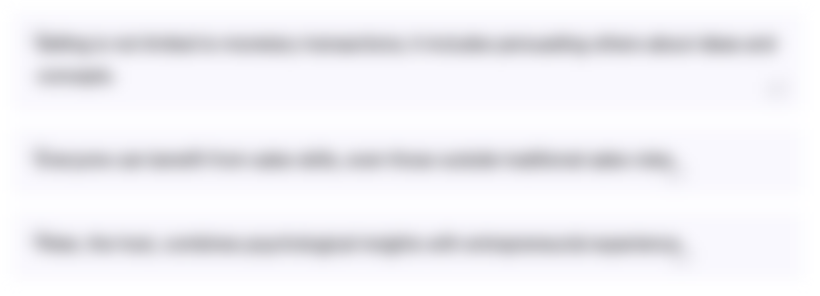
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
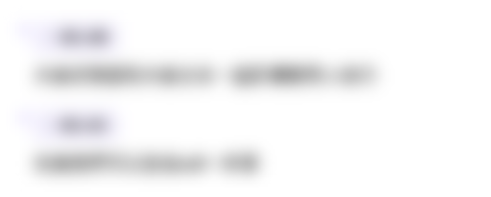
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
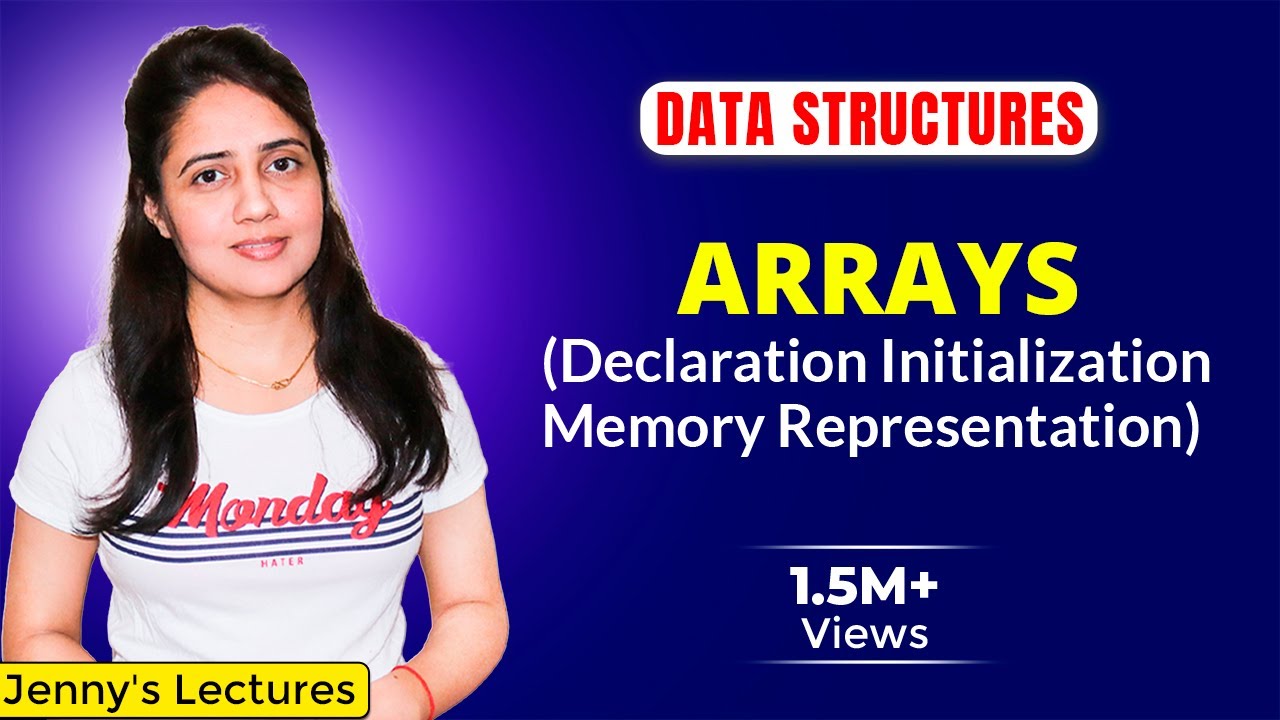
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation
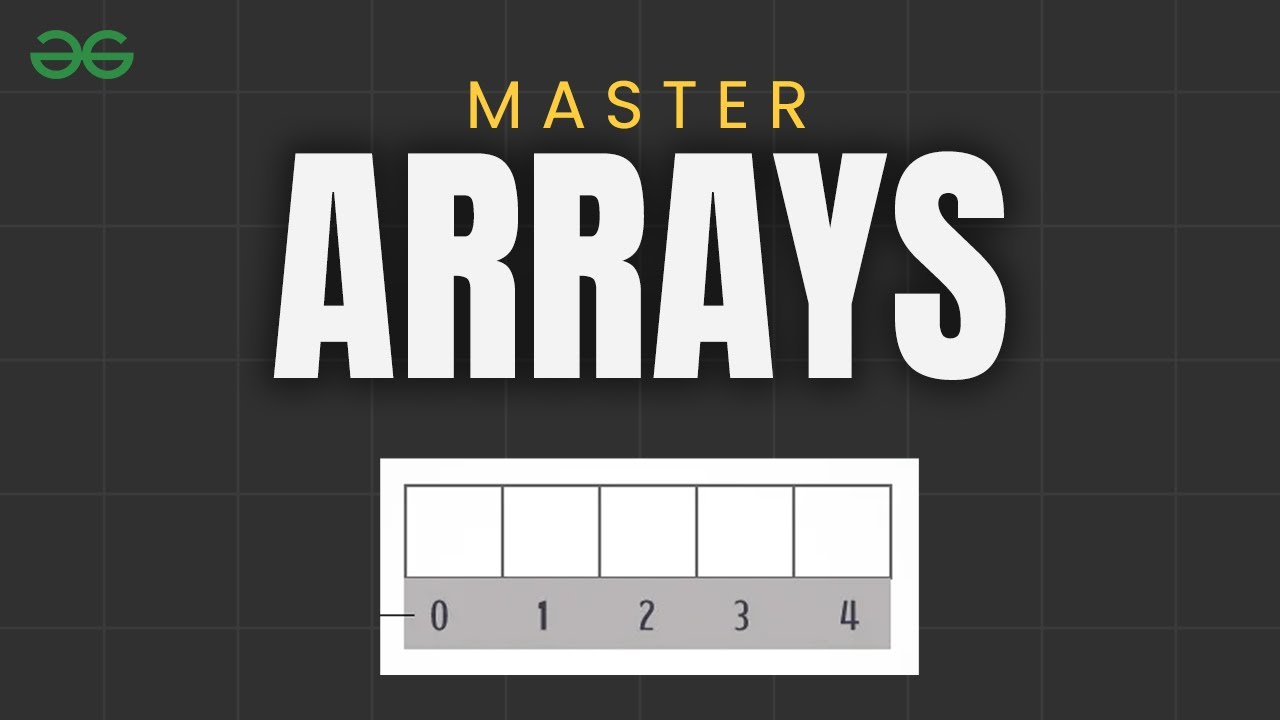
WHAT IS ARRAY? | Array Data Structures | DSA Course | GeeksforGeeks
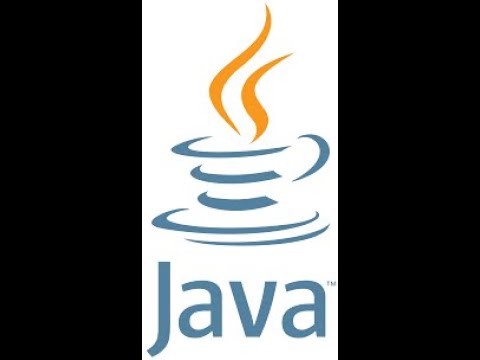
Java programming - Introduction of Array Concept and Algorithm
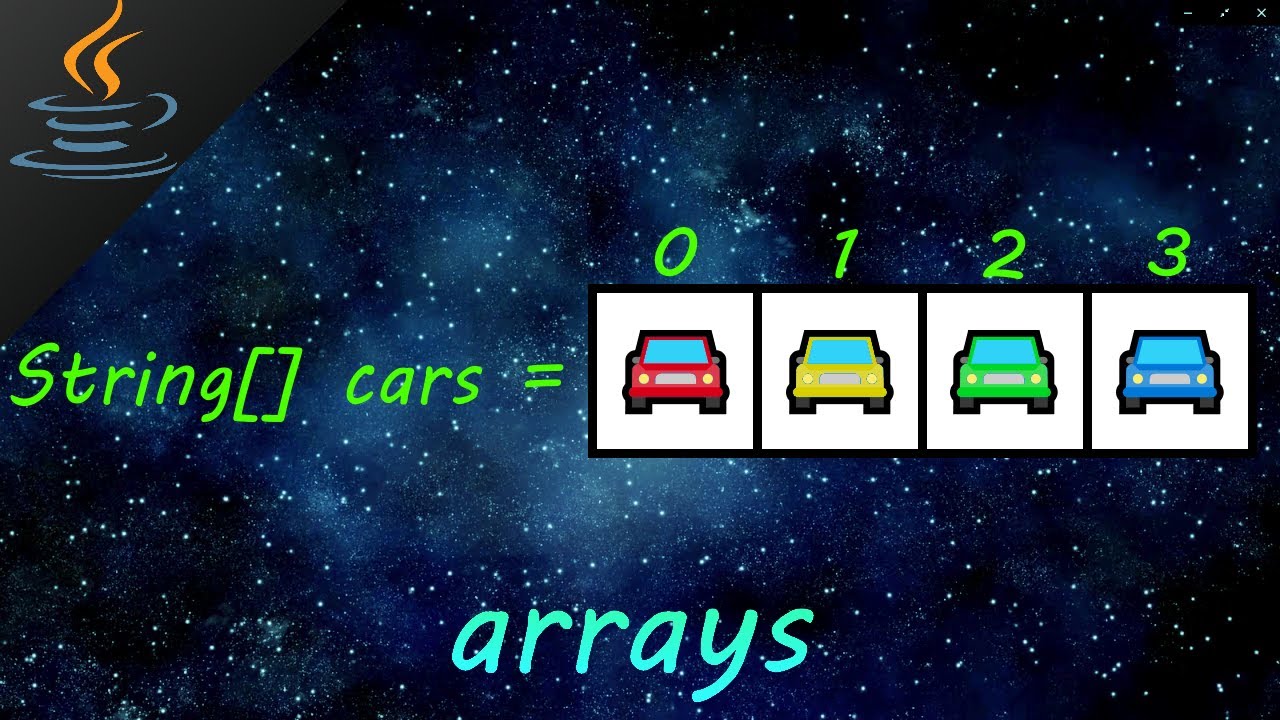
Java arrays 🚗
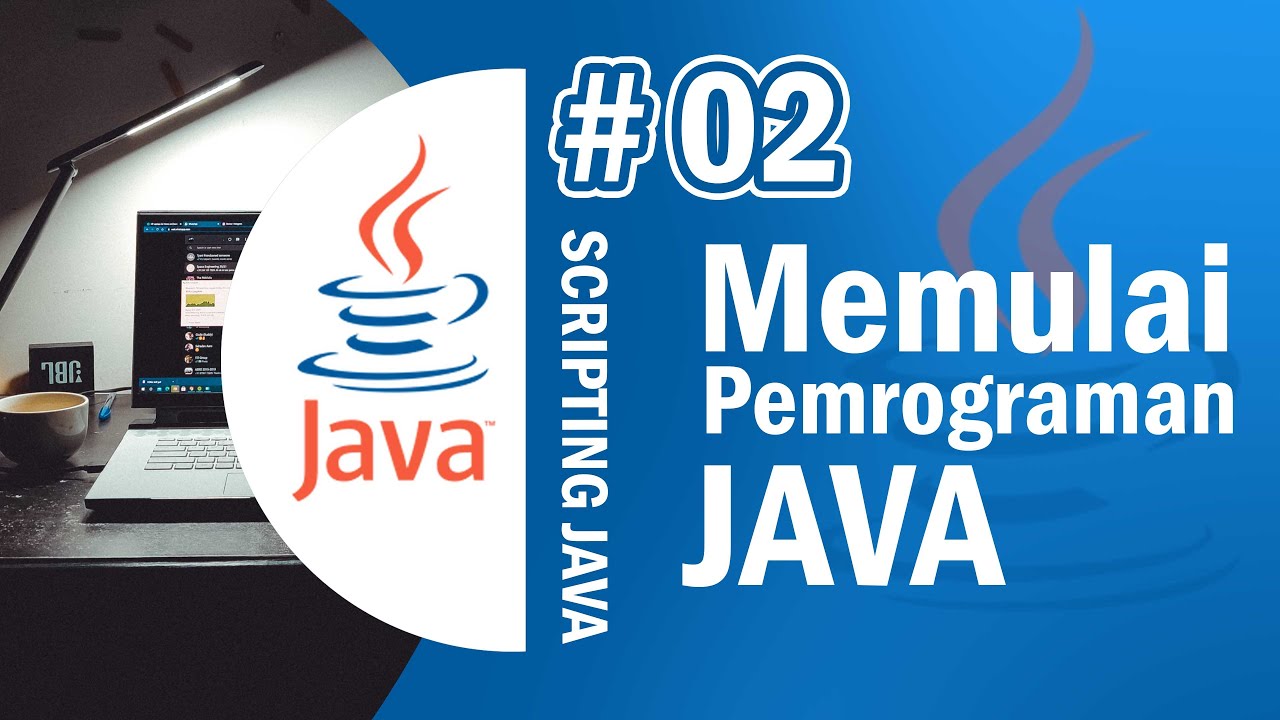
Java 02 - Memulai Pemrograman Java dengan IDE Netbeans - Tutorial Java Netbeans Indonesia
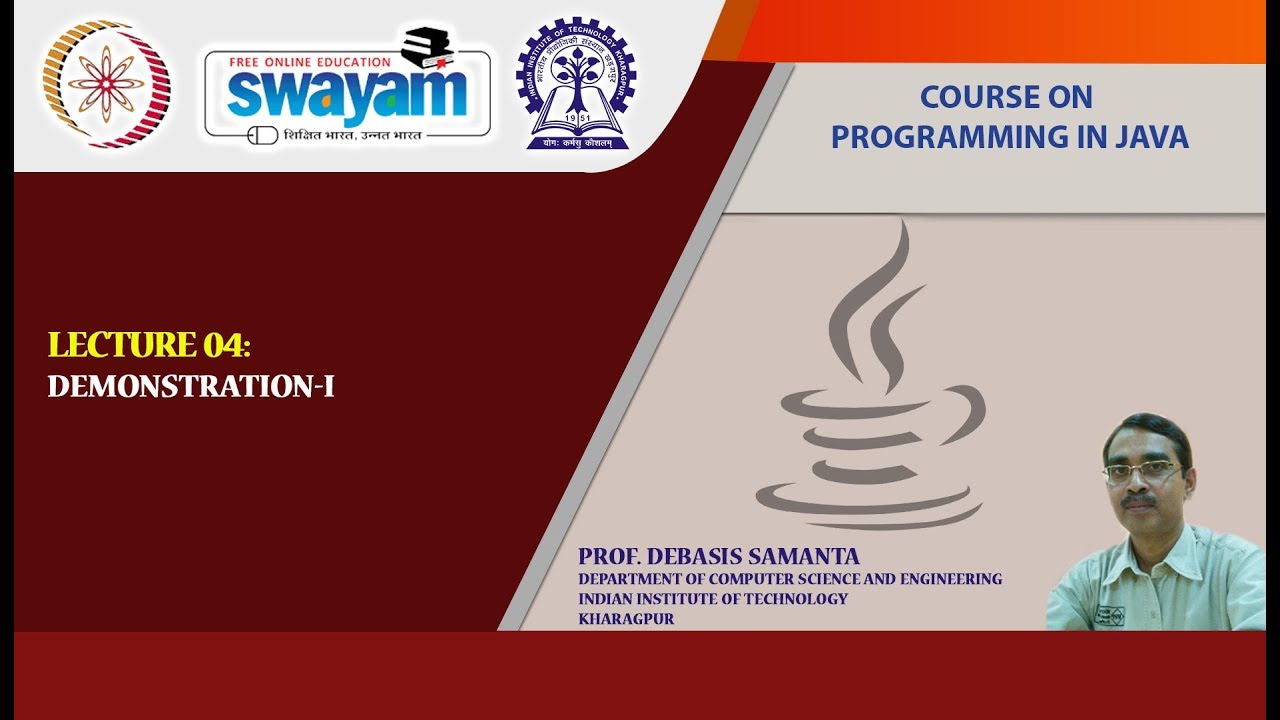
Lecture 04: Demonstration - I
5.0 / 5 (0 votes)